Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Generated / EllipseGeometry.cs / 1 / EllipseGeometry.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class EllipseGeometry : Geometry { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new EllipseGeometry Clone() { return (EllipseGeometry)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new EllipseGeometry CloneCurrentValue() { return (EllipseGeometry)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void RadiusXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { EllipseGeometry target = ((EllipseGeometry) d); target.PropertyChanged(RadiusXProperty); } private static void RadiusYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { EllipseGeometry target = ((EllipseGeometry) d); target.PropertyChanged(RadiusYProperty); } private static void CenterPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { EllipseGeometry target = ((EllipseGeometry) d); target.PropertyChanged(CenterProperty); } #region Public Properties ////// RadiusX - double. Default value is 0.0. /// public double RadiusX { get { return (double) GetValue(RadiusXProperty); } set { SetValueInternal(RadiusXProperty, value); } } ////// RadiusY - double. Default value is 0.0. /// public double RadiusY { get { return (double) GetValue(RadiusYProperty); } set { SetValueInternal(RadiusYProperty, value); } } ////// Center - Point. Default value is new Point(). /// public Point Center { get { return (Point) GetValue(CenterProperty); } set { SetValueInternal(CenterProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new EllipseGeometry(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hRadiusXAnimations = GetAnimationResourceHandle(RadiusXProperty, channel); DUCE.ResourceHandle hRadiusYAnimations = GetAnimationResourceHandle(RadiusYProperty, channel); DUCE.ResourceHandle hCenterAnimations = GetAnimationResourceHandle(CenterProperty, channel); // Pack & send command packet DUCE.MILCMD_ELLIPSEGEOMETRY data; unsafe { data.Type = MILCMD.MilCmdEllipseGeometry; data.Handle = _duceResource.GetHandle(channel); data.hTransform = hTransform; if (hRadiusXAnimations.IsNull) { data.RadiusX = RadiusX; } data.hRadiusXAnimations = hRadiusXAnimations; if (hRadiusYAnimations.IsNull) { data.RadiusY = RadiusY; } data.hRadiusYAnimations = hRadiusYAnimations; if (hCenterAnimations.IsNull) { data.Center = Center; } data.hCenterAnimations = hCenterAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_ELLIPSEGEOMETRY)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_ELLIPSEGEOMETRY)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // RadiusX // RadiusY // Center // internal override int EffectiveValuesInitialSize { get { return 3; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the EllipseGeometry.RadiusX property. /// public static readonly DependencyProperty RadiusXProperty; ////// The DependencyProperty for the EllipseGeometry.RadiusY property. /// public static readonly DependencyProperty RadiusYProperty; ////// The DependencyProperty for the EllipseGeometry.Center property. /// public static readonly DependencyProperty CenterProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_RadiusX = 0.0; internal const double c_RadiusY = 0.0; internal static Point s_Center = new Point(); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static EllipseGeometry() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(EllipseGeometry); RadiusXProperty = RegisterProperty("RadiusX", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(RadiusXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); RadiusYProperty = RegisterProperty("RadiusY", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(RadiusYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterProperty = RegisterProperty("Center", typeof(Point), typeofThis, new Point(), new PropertyChangedCallback(CenterPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class EllipseGeometry : Geometry { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new EllipseGeometry Clone() { return (EllipseGeometry)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new EllipseGeometry CloneCurrentValue() { return (EllipseGeometry)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void RadiusXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { EllipseGeometry target = ((EllipseGeometry) d); target.PropertyChanged(RadiusXProperty); } private static void RadiusYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { EllipseGeometry target = ((EllipseGeometry) d); target.PropertyChanged(RadiusYProperty); } private static void CenterPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { EllipseGeometry target = ((EllipseGeometry) d); target.PropertyChanged(CenterProperty); } #region Public Properties ////// RadiusX - double. Default value is 0.0. /// public double RadiusX { get { return (double) GetValue(RadiusXProperty); } set { SetValueInternal(RadiusXProperty, value); } } ////// RadiusY - double. Default value is 0.0. /// public double RadiusY { get { return (double) GetValue(RadiusYProperty); } set { SetValueInternal(RadiusYProperty, value); } } ////// Center - Point. Default value is new Point(). /// public Point Center { get { return (Point) GetValue(CenterProperty); } set { SetValueInternal(CenterProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new EllipseGeometry(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hRadiusXAnimations = GetAnimationResourceHandle(RadiusXProperty, channel); DUCE.ResourceHandle hRadiusYAnimations = GetAnimationResourceHandle(RadiusYProperty, channel); DUCE.ResourceHandle hCenterAnimations = GetAnimationResourceHandle(CenterProperty, channel); // Pack & send command packet DUCE.MILCMD_ELLIPSEGEOMETRY data; unsafe { data.Type = MILCMD.MilCmdEllipseGeometry; data.Handle = _duceResource.GetHandle(channel); data.hTransform = hTransform; if (hRadiusXAnimations.IsNull) { data.RadiusX = RadiusX; } data.hRadiusXAnimations = hRadiusXAnimations; if (hRadiusYAnimations.IsNull) { data.RadiusY = RadiusY; } data.hRadiusYAnimations = hRadiusYAnimations; if (hCenterAnimations.IsNull) { data.Center = Center; } data.hCenterAnimations = hCenterAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_ELLIPSEGEOMETRY)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_ELLIPSEGEOMETRY)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // RadiusX // RadiusY // Center // internal override int EffectiveValuesInitialSize { get { return 3; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the EllipseGeometry.RadiusX property. /// public static readonly DependencyProperty RadiusXProperty; ////// The DependencyProperty for the EllipseGeometry.RadiusY property. /// public static readonly DependencyProperty RadiusYProperty; ////// The DependencyProperty for the EllipseGeometry.Center property. /// public static readonly DependencyProperty CenterProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_RadiusX = 0.0; internal const double c_RadiusY = 0.0; internal static Point s_Center = new Point(); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static EllipseGeometry() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(EllipseGeometry); RadiusXProperty = RegisterProperty("RadiusX", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(RadiusXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); RadiusYProperty = RegisterProperty("RadiusY", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(RadiusYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterProperty = RegisterProperty("Center", typeof(Point), typeofThis, new Point(), new PropertyChangedCallback(CenterPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
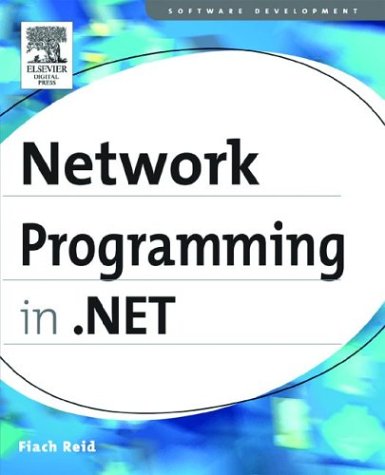
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- listitem.cs
- CharacterHit.cs
- StateBag.cs
- SoapFault.cs
- HtmlInputRadioButton.cs
- PlainXmlDeserializer.cs
- HttpInputStream.cs
- XmlQueryCardinality.cs
- OverflowException.cs
- HostingEnvironment.cs
- WebPartUserCapability.cs
- UnmanagedMemoryStreamWrapper.cs
- TextElementCollectionHelper.cs
- AutoCompleteStringCollection.cs
- SweepDirectionValidation.cs
- DescriptionAttribute.cs
- CharacterBufferReference.cs
- XomlCompilerError.cs
- SettingsPropertyCollection.cs
- SmtpReplyReaderFactory.cs
- AppDomainProtocolHandler.cs
- TimeStampChecker.cs
- DataQuery.cs
- CompilerTypeWithParams.cs
- InProcStateClientManager.cs
- DataFormats.cs
- FixedFlowMap.cs
- WindowsFormsHostPropertyMap.cs
- CollectionBase.cs
- XmlDigitalSignatureProcessor.cs
- BitmapEffectGeneralTransform.cs
- ImpersonateTokenRef.cs
- WebZoneDesigner.cs
- StreamWriter.cs
- ExpressionPrefixAttribute.cs
- StreamGeometry.cs
- AbsoluteQuery.cs
- HtmlForm.cs
- AliasExpr.cs
- StyleSelector.cs
- UnregisterInfo.cs
- MembershipAdapter.cs
- Color.cs
- CharacterShapingProperties.cs
- ByteStorage.cs
- SafeEventLogWriteHandle.cs
- EventManager.cs
- PropertyGridEditorPart.cs
- ButtonFieldBase.cs
- Int32AnimationBase.cs
- WizardStepBase.cs
- Msec.cs
- Command.cs
- DeploymentExceptionMapper.cs
- ResourceDescriptionAttribute.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- SHA384Managed.cs
- FlowPosition.cs
- ReaderContextStackData.cs
- GlyphsSerializer.cs
- XmlIterators.cs
- Renderer.cs
- ProxyHelper.cs
- CheckBoxDesigner.cs
- NetworkAddressChange.cs
- AsyncResult.cs
- MonitoringDescriptionAttribute.cs
- ToolBarButtonClickEvent.cs
- ConvertersCollection.cs
- TiffBitmapEncoder.cs
- DataGridTable.cs
- GradientStopCollection.cs
- BooleanToVisibilityConverter.cs
- ObjectNavigationPropertyMapping.cs
- OleDbCommand.cs
- SqlGenericUtil.cs
- Run.cs
- HttpServerVarsCollection.cs
- XamlTypeWithExplicitNamespace.cs
- EntityClassGenerator.cs
- ExpressionBinding.cs
- Padding.cs
- StreamHelper.cs
- ValueConversionAttribute.cs
- Size.cs
- BulletedList.cs
- EnumValAlphaComparer.cs
- EnumBuilder.cs
- ConnectionString.cs
- FlowLayout.cs
- DbConnectionPoolOptions.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- ReadOnlyHierarchicalDataSourceView.cs
- BaseCAMarshaler.cs
- AnnotationResource.cs
- ObjectHandle.cs
- ValueUnavailableException.cs
- StylusSystemGestureEventArgs.cs
- OpenTypeMethods.cs
- latinshape.cs