Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Advanced / PointF.cs / 2 / PointF.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Diagnostics; using System.Drawing; using System.ComponentModel; using System; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a point in 2D coordinate space * (float precision floating-point coordinates) */ ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct PointF { ////// /// public static readonly PointF Empty = new PointF(); private float x; private float y; /** * Create a new Point object at the given location */ ////// Creates a new instance of the ///class /// with member data left uninitialized. /// /// /// public PointF(float x, float y) { this.x = x; this.y = y; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// [Browsable(false)] public bool IsEmpty { get { return x == 0f && y == 0f; } } ////// Gets a value indicating whether this ///is empty. /// /// /// public float X { get { return x; } set { x = value; } } ////// Gets the x-coordinate of this ///. /// /// /// public float Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static PointF operator +(PointF pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static PointF operator -(PointF pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// public static PointF operator +(PointF pt, SizeF sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// public static PointF operator -(PointF pt, SizeF sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(PointF left, PointF right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(PointF left, PointF right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static PointF Add(PointF pt, Size sz) { return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, Size sz) { return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// public static PointF Add(PointF pt, SizeF sz){ return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, SizeF sz){ return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// public override bool Equals(object obj) { if (!(obj is PointF)) return false; PointF comp = (PointF)obj; return comp.X == this.X && comp.Y == this.Y && comp.GetType().Equals(this.GetType()); } ///[To be supplied.] ////// /// public override int GetHashCode() { return base.GetHashCode(); } ///[To be supplied.] ///public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "{{X={0}, Y={1}}}", x, y); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Diagnostics; using System.Drawing; using System.ComponentModel; using System; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a point in 2D coordinate space * (float precision floating-point coordinates) */ ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct PointF { ////// /// public static readonly PointF Empty = new PointF(); private float x; private float y; /** * Create a new Point object at the given location */ ////// Creates a new instance of the ///class /// with member data left uninitialized. /// /// /// public PointF(float x, float y) { this.x = x; this.y = y; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// [Browsable(false)] public bool IsEmpty { get { return x == 0f && y == 0f; } } ////// Gets a value indicating whether this ///is empty. /// /// /// public float X { get { return x; } set { x = value; } } ////// Gets the x-coordinate of this ///. /// /// /// public float Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static PointF operator +(PointF pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static PointF operator -(PointF pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// public static PointF operator +(PointF pt, SizeF sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// public static PointF operator -(PointF pt, SizeF sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(PointF left, PointF right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(PointF left, PointF right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static PointF Add(PointF pt, Size sz) { return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, Size sz) { return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// public static PointF Add(PointF pt, SizeF sz){ return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, SizeF sz){ return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// public override bool Equals(object obj) { if (!(obj is PointF)) return false; PointF comp = (PointF)obj; return comp.X == this.X && comp.Y == this.Y && comp.GetType().Equals(this.GetType()); } ///[To be supplied.] ////// /// public override int GetHashCode() { return base.GetHashCode(); } ///[To be supplied.] ///public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "{{X={0}, Y={1}}}", x, y); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
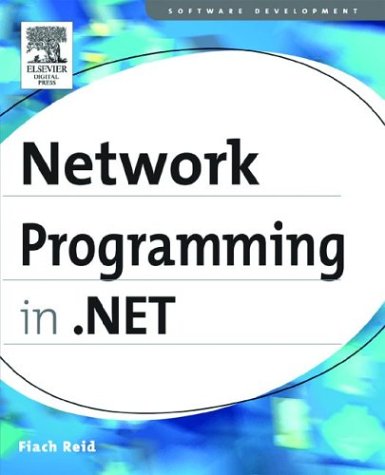
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeAssignStatement.cs
- IdentityHolder.cs
- EntityViewContainer.cs
- AttachmentCollection.cs
- handlecollector.cs
- ExceptionList.cs
- sqlinternaltransaction.cs
- CompositeControl.cs
- CompressEmulationStream.cs
- DefaultAsyncDataDispatcher.cs
- JournalEntry.cs
- MergePropertyDescriptor.cs
- Composition.cs
- DBAsyncResult.cs
- ImageEditor.cs
- CodeMethodMap.cs
- SystemColorTracker.cs
- NotSupportedException.cs
- Utility.cs
- EnumCodeDomSerializer.cs
- ObjectKeyFrameCollection.cs
- ResourceManager.cs
- HtmlInputSubmit.cs
- TTSVoice.cs
- ScrollViewerAutomationPeer.cs
- SchemaConstraints.cs
- GradientStopCollection.cs
- CacheOutputQuery.cs
- querybuilder.cs
- GuidConverter.cs
- SafeTimerHandle.cs
- SmiMetaDataProperty.cs
- DbProviderSpecificTypePropertyAttribute.cs
- GAC.cs
- DataGridItemCollection.cs
- LinqDataSourceContextEventArgs.cs
- Types.cs
- VersionedStream.cs
- Char.cs
- MessageParameterAttribute.cs
- PanelDesigner.cs
- DetailsViewUpdatedEventArgs.cs
- DeploymentExceptionMapper.cs
- Semaphore.cs
- InputScope.cs
- _Semaphore.cs
- SHA1Managed.cs
- CollectionViewProxy.cs
- MissingMethodException.cs
- Point4D.cs
- odbcmetadatafactory.cs
- PolicyManager.cs
- HostExecutionContextManager.cs
- SharedStatics.cs
- UserControlParser.cs
- XmlSchemaSimpleTypeRestriction.cs
- SafeBuffer.cs
- HtmlShimManager.cs
- WebPartTracker.cs
- ChtmlMobileTextWriter.cs
- ApplicationInfo.cs
- ViewStateException.cs
- StringHandle.cs
- HttpServerVarsCollection.cs
- ImageUrlEditor.cs
- AssociationTypeEmitter.cs
- TagMapInfo.cs
- RepeaterCommandEventArgs.cs
- TextBox.cs
- SqlRowUpdatingEvent.cs
- XmlLoader.cs
- WebPermission.cs
- Missing.cs
- InfoCard.cs
- ValidatorCompatibilityHelper.cs
- XmlName.cs
- CollectionBase.cs
- AutomationPattern.cs
- PropertyEmitterBase.cs
- XamlToRtfParser.cs
- DBAsyncResult.cs
- MetadataItem_Static.cs
- Pair.cs
- TextOptions.cs
- PathParser.cs
- ConfigXmlText.cs
- AutoGeneratedField.cs
- AsyncPostBackTrigger.cs
- TimeStampChecker.cs
- MessagePropertyDescriptionCollection.cs
- StylusDownEventArgs.cs
- AutomationElement.cs
- ReadOnlyDictionary.cs
- ValueOfAction.cs
- EditingMode.cs
- RepeaterItemEventArgs.cs
- BaseResourcesBuildProvider.cs
- XmlQueryStaticData.cs
- SimpleHandlerBuildProvider.cs
- TileModeValidation.cs