Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / Vector3D.cs / 1 / Vector3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { [Serializable] [TypeConverter(typeof(Vector3DConverter))] [ValueSerializer(typeof(Vector3DValueSerializer))] // Used by MarkupWriter partial struct Vector3D : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Vector3D instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Vector3D instances are exactly equal, false otherwise /// /// The first Vector3D to compare /// The second Vector3D to compare public static bool operator == (Vector3D vector1, Vector3D vector2) { return vector1.X == vector2.X && vector1.Y == vector2.Y && vector1.Z == vector2.Z; } ////// Compares two Vector3D instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Vector3D instances are exactly unequal, false otherwise /// /// The first Vector3D to compare /// The second Vector3D to compare public static bool operator != (Vector3D vector1, Vector3D vector2) { return !(vector1 == vector2); } ////// Compares two Vector3D instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Vector3D instances are exactly equal, false otherwise /// /// The first Vector3D to compare /// The second Vector3D to compare public static bool Equals (Vector3D vector1, Vector3D vector2) { return vector1.X.Equals(vector2.X) && vector1.Y.Equals(vector2.Y) && vector1.Z.Equals(vector2.Z); } ////// Equals - compares this Vector3D with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Vector3D and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Vector3D)) { return false; } Vector3D value = (Vector3D)o; return Vector3D.Equals(this,value); } ////// Equals - compares this Vector3D with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Vector3D to compare to "this" public bool Equals(Vector3D value) { return Vector3D.Equals(this, value); } ////// Returns the HashCode for this Vector3D /// ////// int - the HashCode for this Vector3D /// public override int GetHashCode() { // Perform field-by-field XOR of HashCodes return X.GetHashCode() ^ Y.GetHashCode() ^ Z.GetHashCode(); } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Vector3D data /// public static Vector3D Parse(string source) { IFormatProvider formatProvider = CultureInfo.GetCultureInfo("en-us"); TokenizerHelper th = new TokenizerHelper(source, formatProvider); Vector3D value; String firstToken = th.NextTokenRequired(); value = new Vector3D( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// X - double. Default value is 0. /// public double X { get { return _x; } set { _x = value; } } ////// Y - double. Default value is 0. /// public double Y { get { return _y; } set { _y = value; } } ////// Z - double. Default value is 0. /// public double Z { get { return _z; } set { _z = value; } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}", separator, _x, _y, _z); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _x; internal double _y; internal double _z; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { [Serializable] [TypeConverter(typeof(Vector3DConverter))] [ValueSerializer(typeof(Vector3DValueSerializer))] // Used by MarkupWriter partial struct Vector3D : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Vector3D instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Vector3D instances are exactly equal, false otherwise /// /// The first Vector3D to compare /// The second Vector3D to compare public static bool operator == (Vector3D vector1, Vector3D vector2) { return vector1.X == vector2.X && vector1.Y == vector2.Y && vector1.Z == vector2.Z; } ////// Compares two Vector3D instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Vector3D instances are exactly unequal, false otherwise /// /// The first Vector3D to compare /// The second Vector3D to compare public static bool operator != (Vector3D vector1, Vector3D vector2) { return !(vector1 == vector2); } ////// Compares two Vector3D instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Vector3D instances are exactly equal, false otherwise /// /// The first Vector3D to compare /// The second Vector3D to compare public static bool Equals (Vector3D vector1, Vector3D vector2) { return vector1.X.Equals(vector2.X) && vector1.Y.Equals(vector2.Y) && vector1.Z.Equals(vector2.Z); } ////// Equals - compares this Vector3D with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Vector3D and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Vector3D)) { return false; } Vector3D value = (Vector3D)o; return Vector3D.Equals(this,value); } ////// Equals - compares this Vector3D with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Vector3D to compare to "this" public bool Equals(Vector3D value) { return Vector3D.Equals(this, value); } ////// Returns the HashCode for this Vector3D /// ////// int - the HashCode for this Vector3D /// public override int GetHashCode() { // Perform field-by-field XOR of HashCodes return X.GetHashCode() ^ Y.GetHashCode() ^ Z.GetHashCode(); } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Vector3D data /// public static Vector3D Parse(string source) { IFormatProvider formatProvider = CultureInfo.GetCultureInfo("en-us"); TokenizerHelper th = new TokenizerHelper(source, formatProvider); Vector3D value; String firstToken = th.NextTokenRequired(); value = new Vector3D( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// X - double. Default value is 0. /// public double X { get { return _x; } set { _x = value; } } ////// Y - double. Default value is 0. /// public double Y { get { return _y; } set { _y = value; } } ////// Z - double. Default value is 0. /// public double Z { get { return _z; } set { _z = value; } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}", separator, _x, _y, _z); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _x; internal double _y; internal double _z; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
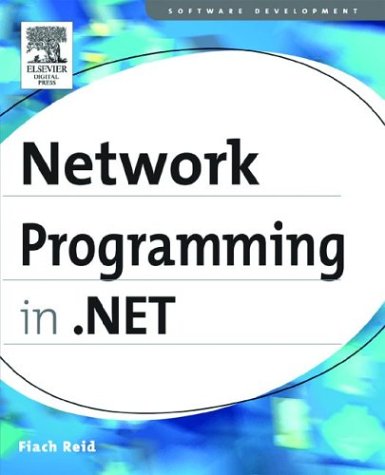
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewComboBoxEditingControl.cs
- CloseCollectionAsyncResult.cs
- TextRangeProviderWrapper.cs
- ResourceAssociationSet.cs
- CustomErrorsSection.cs
- ToolStripScrollButton.cs
- HTMLTagNameToTypeMapper.cs
- ToolStripDropDownItem.cs
- WindowsSlider.cs
- WorkflowRuntimeServicesBehavior.cs
- GeneralTransform.cs
- WebPartEditVerb.cs
- DispatcherExceptionFilterEventArgs.cs
- SelectedPathEditor.cs
- FileDialog_Vista.cs
- HostSecurityManager.cs
- RadioButton.cs
- SpeechAudioFormatInfo.cs
- TableCellCollection.cs
- TripleDES.cs
- ObjectDataSourceStatusEventArgs.cs
- DataColumnMappingCollection.cs
- DynamicQueryableWrapper.cs
- CategoryEditor.cs
- InkCollectionBehavior.cs
- IconBitmapDecoder.cs
- SQLInt16.cs
- DataErrorValidationRule.cs
- FormsAuthenticationCredentials.cs
- AttributeCollection.cs
- InputReferenceExpression.cs
- WorkflowMarkupSerializationException.cs
- ItemsControl.cs
- SpellerStatusTable.cs
- DataSourceExpression.cs
- FilterEventArgs.cs
- CounterCreationDataCollection.cs
- IdentityHolder.cs
- RenderContext.cs
- AutomationPropertyChangedEventArgs.cs
- FragmentQueryKB.cs
- Exceptions.cs
- EntityTypeBase.cs
- NegationPusher.cs
- Pen.cs
- RunClient.cs
- CodeAttributeArgumentCollection.cs
- _FtpDataStream.cs
- RSACryptoServiceProvider.cs
- UidManager.cs
- DataTablePropertyDescriptor.cs
- ProfileInfo.cs
- DBConnectionString.cs
- Cursor.cs
- Point4D.cs
- RectAnimationClockResource.cs
- Image.cs
- TreeChangeInfo.cs
- TableCellAutomationPeer.cs
- ExpressionEvaluator.cs
- StreamSecurityUpgradeProvider.cs
- TemplateDefinition.cs
- ThreadAttributes.cs
- AllMembershipCondition.cs
- ProxyAttribute.cs
- SortFieldComparer.cs
- TextCollapsingProperties.cs
- HttpHandlersInstallComponent.cs
- ObjectFullSpanRewriter.cs
- LayoutEngine.cs
- CodeAccessSecurityEngine.cs
- XmlILIndex.cs
- Socket.cs
- RequestQueue.cs
- UserInitiatedNavigationPermission.cs
- DomNameTable.cs
- ReachPrintTicketSerializerAsync.cs
- DataServiceClientException.cs
- _Events.cs
- EncoderFallback.cs
- DbConnectionFactory.cs
- DiagnosticTrace.cs
- LinkLabel.cs
- ImageField.cs
- Label.cs
- DBConnectionString.cs
- StorageRoot.cs
- HttpGetProtocolReflector.cs
- SectionUpdates.cs
- LocatorBase.cs
- WebBrowser.cs
- EtwProvider.cs
- Mutex.cs
- SessionEndedEventArgs.cs
- ISFClipboardData.cs
- TypeUsage.cs
- ConfigurationLocationCollection.cs
- DbProviderConfigurationHandler.cs
- DynamicPropertyHolder.cs
- EntityCommandCompilationException.cs