Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Generated / GeometryConverter.cs / 1 / GeometryConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { ////// GeometryConverter - Converter class for converting instances of other types to and from Geometry instances /// public sealed class GeometryConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for some instances if (context != null && context.Instance != null) { if (!(context.Instance is Geometry)) { throw new ArgumentException(SR.Get(SRID.General_Expected_Type, "Geometry"), "context.Instance"); } Geometry value = (Geometry)context.Instance; #pragma warning suppress 6506 // value is obviously not null return value.CanSerializeToString(); } return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Geometry from the given object. /// ////// The Geometry which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Geometry. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Geometry. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Geometry.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Geometry to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Geometry, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Geometry instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Geometry) { Geometry instance = (Geometry)value; if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for some instances if (context != null && context.Instance != null) { #pragma warning suppress 6506 // instance is obviously not null if (!instance.CanSerializeToString()) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertToNotSupported)); } } // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { ////// GeometryConverter - Converter class for converting instances of other types to and from Geometry instances /// public sealed class GeometryConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for some instances if (context != null && context.Instance != null) { if (!(context.Instance is Geometry)) { throw new ArgumentException(SR.Get(SRID.General_Expected_Type, "Geometry"), "context.Instance"); } Geometry value = (Geometry)context.Instance; #pragma warning suppress 6506 // value is obviously not null return value.CanSerializeToString(); } return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Geometry from the given object. /// ////// The Geometry which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Geometry. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Geometry. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Geometry.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Geometry to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Geometry, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Geometry instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Geometry) { Geometry instance = (Geometry)value; if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for some instances if (context != null && context.Instance != null) { #pragma warning suppress 6506 // instance is obviously not null if (!instance.CanSerializeToString()) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertToNotSupported)); } } // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
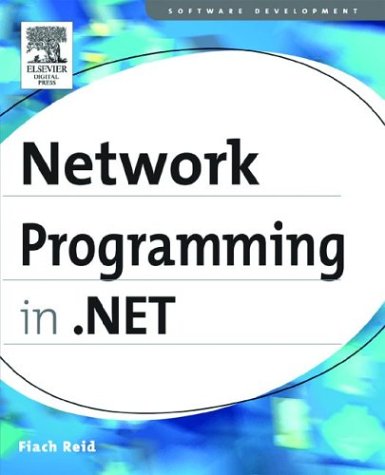
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompatibleIComparer.cs
- AuthenticationService.cs
- RuntimeIdentifierPropertyAttribute.cs
- DropSourceBehavior.cs
- UpdateTranslator.cs
- PropertyIDSet.cs
- HwndSourceParameters.cs
- RuntimeResourceSet.cs
- OperationFormatter.cs
- HttpInputStream.cs
- CapabilitiesRule.cs
- XmlToDatasetMap.cs
- WindowsFont.cs
- ClosableStream.cs
- StrokeNodeEnumerator.cs
- ArrayList.cs
- FileSystemWatcher.cs
- EventDescriptor.cs
- basevalidator.cs
- DbBuffer.cs
- FlowchartSizeFeature.cs
- TextFindEngine.cs
- ResourceIDHelper.cs
- RoleGroupCollection.cs
- DateTimeFormatInfoScanner.cs
- BitmapImage.cs
- PrimitiveOperationFormatter.cs
- ToolStripSplitStackLayout.cs
- Iis7Helper.cs
- DoubleIndependentAnimationStorage.cs
- Asn1IntegerConverter.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- WebBrowserContainer.cs
- SHA512.cs
- InheritanceContextChangedEventManager.cs
- FontCollection.cs
- ReadWriteObjectLock.cs
- XmlSchemaCollection.cs
- NumberAction.cs
- ScriptComponentDescriptor.cs
- WebBrowser.cs
- RC2CryptoServiceProvider.cs
- DataViewSettingCollection.cs
- PrimaryKeyTypeConverter.cs
- DataQuery.cs
- ValueTable.cs
- SqlMultiplexer.cs
- EventLogInternal.cs
- SQLMoney.cs
- SystemMulticastIPAddressInformation.cs
- MeshGeometry3D.cs
- TransformerInfoCollection.cs
- InstanceKeyCollisionException.cs
- DecimalKeyFrameCollection.cs
- CircleHotSpot.cs
- QilStrConcatenator.cs
- SecurityElementBase.cs
- DataGridViewTopLeftHeaderCell.cs
- EncoderFallback.cs
- WindowShowOrOpenTracker.cs
- Delegate.cs
- returneventsaver.cs
- RuleAttributes.cs
- Peer.cs
- HttpHandlersSection.cs
- UserControlBuildProvider.cs
- MarkupExtensionReturnTypeAttribute.cs
- StandardRuntimeEnumValidatorAttribute.cs
- querybuilder.cs
- NameNode.cs
- SamlAuthorityBinding.cs
- ModuleElement.cs
- ProcessModule.cs
- SerializationUtilities.cs
- SerializationSectionGroup.cs
- FormParameter.cs
- CommonDialog.cs
- ProcessDesigner.cs
- login.cs
- BitmapDownload.cs
- DataGridViewColumnCollection.cs
- TransformConverter.cs
- OperationBehaviorAttribute.cs
- DataDocumentXPathNavigator.cs
- HtmlGenericControl.cs
- Timeline.cs
- AlternateViewCollection.cs
- odbcmetadatafactory.cs
- ProcessModule.cs
- Visual.cs
- CodeDomLoader.cs
- TransportSecurityProtocol.cs
- FileDialog.cs
- DBAsyncResult.cs
- DbConnectionClosed.cs
- DeriveBytes.cs
- BufferCache.cs
- DataGridLinkButton.cs
- PeerCollaboration.cs
- ClickablePoint.cs