Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripRenderEventArgs.cs / 1 / ToolStripRenderEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Drawing; ////// /// ToolStripRenderEventArgs /// public class ToolStripRenderEventArgs : EventArgs { private ToolStrip toolStrip = null; private Graphics graphics = null; private Rectangle affectedBounds = Rectangle.Empty; private Color backColor = Color.Empty; ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip) { this.toolStrip = toolStrip; this.graphics = g; this.affectedBounds = new Rectangle(Point.Empty, toolStrip.Size); } ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip, Rectangle affectedBounds, Color backColor) { this.toolStrip = toolStrip; this.affectedBounds = affectedBounds; this.graphics = g; this.backColor = backColor; } ////// /// the bounds to draw in /// public Rectangle AffectedBounds { get { return affectedBounds; } } ////// /// the back color to draw with. /// public Color BackColor { get { if (backColor == Color.Empty) { // get the user specified color backColor = toolStrip.RawBackColor; if (backColor == Color.Empty) { if (toolStrip is ToolStripDropDown) { backColor = SystemColors.Menu; } else if (toolStrip is MenuStrip) { backColor = SystemColors.MenuBar; } else { backColor = SystemColors.Control; } } } return backColor; } } ////// /// the graphics object to draw with /// public Graphics Graphics { get { return graphics; } } ////// /// Represents which toolStrip was affected by the click /// public ToolStrip ToolStrip { get { return toolStrip; } } ///public Rectangle ConnectedArea { get { ToolStripDropDown dropDown = toolStrip as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem ownerItem = dropDown.OwnerItem as ToolStripDropDownItem; if (ownerItem is MdiControlStrip.SystemMenuItem) { // there's no connected rect between a system menu item and a dropdown. return Rectangle.Empty; } if (ownerItem !=null && ownerItem.ParentInternal != null && !ownerItem.IsOnDropDown) { // translate the item into our coordinate system. Rectangle itemBounds = new Rectangle(toolStrip.PointToClient(ownerItem.TranslatePoint(Point.Empty, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords)), ownerItem.Size); Rectangle bounds = ToolStrip.Bounds; Rectangle overlap = ToolStrip.ClientRectangle; overlap.Inflate(1,1); if (overlap.IntersectsWith(itemBounds)) { switch (ownerItem.DropDownDirection) { case ToolStripDropDownDirection.AboveLeft: case ToolStripDropDownDirection.AboveRight: // Consider... the shadow effect interferes with the connected area. return Rectangle.Empty; // return new Rectangle(itemBounds.X+1, bounds.Height -2, itemBounds.Width -2, 2); case ToolStripDropDownDirection.BelowRight: case ToolStripDropDownDirection.BelowLeft: overlap.Intersect(itemBounds); if (overlap.Height == 2) { return new Rectangle(itemBounds.X+1, 0, itemBounds.Width -2, 2); } // if its overlapping more than one pixel, this means we've pushed it to obscure // the menu item. in this case pretend it's not connected. return Rectangle.Empty; case ToolStripDropDownDirection.Right: case ToolStripDropDownDirection.Left: return Rectangle.Empty; // Consider... the shadow effect interferes with the connected area. // return new Rectangle(bounds.Width-2, 1, 2, itemBounds.Height-2); } } } } return Rectangle.Empty; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Drawing; ////// /// ToolStripRenderEventArgs /// public class ToolStripRenderEventArgs : EventArgs { private ToolStrip toolStrip = null; private Graphics graphics = null; private Rectangle affectedBounds = Rectangle.Empty; private Color backColor = Color.Empty; ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip) { this.toolStrip = toolStrip; this.graphics = g; this.affectedBounds = new Rectangle(Point.Empty, toolStrip.Size); } ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip, Rectangle affectedBounds, Color backColor) { this.toolStrip = toolStrip; this.affectedBounds = affectedBounds; this.graphics = g; this.backColor = backColor; } ////// /// the bounds to draw in /// public Rectangle AffectedBounds { get { return affectedBounds; } } ////// /// the back color to draw with. /// public Color BackColor { get { if (backColor == Color.Empty) { // get the user specified color backColor = toolStrip.RawBackColor; if (backColor == Color.Empty) { if (toolStrip is ToolStripDropDown) { backColor = SystemColors.Menu; } else if (toolStrip is MenuStrip) { backColor = SystemColors.MenuBar; } else { backColor = SystemColors.Control; } } } return backColor; } } ////// /// the graphics object to draw with /// public Graphics Graphics { get { return graphics; } } ////// /// Represents which toolStrip was affected by the click /// public ToolStrip ToolStrip { get { return toolStrip; } } ///public Rectangle ConnectedArea { get { ToolStripDropDown dropDown = toolStrip as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem ownerItem = dropDown.OwnerItem as ToolStripDropDownItem; if (ownerItem is MdiControlStrip.SystemMenuItem) { // there's no connected rect between a system menu item and a dropdown. return Rectangle.Empty; } if (ownerItem !=null && ownerItem.ParentInternal != null && !ownerItem.IsOnDropDown) { // translate the item into our coordinate system. Rectangle itemBounds = new Rectangle(toolStrip.PointToClient(ownerItem.TranslatePoint(Point.Empty, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords)), ownerItem.Size); Rectangle bounds = ToolStrip.Bounds; Rectangle overlap = ToolStrip.ClientRectangle; overlap.Inflate(1,1); if (overlap.IntersectsWith(itemBounds)) { switch (ownerItem.DropDownDirection) { case ToolStripDropDownDirection.AboveLeft: case ToolStripDropDownDirection.AboveRight: // Consider... the shadow effect interferes with the connected area. return Rectangle.Empty; // return new Rectangle(itemBounds.X+1, bounds.Height -2, itemBounds.Width -2, 2); case ToolStripDropDownDirection.BelowRight: case ToolStripDropDownDirection.BelowLeft: overlap.Intersect(itemBounds); if (overlap.Height == 2) { return new Rectangle(itemBounds.X+1, 0, itemBounds.Width -2, 2); } // if its overlapping more than one pixel, this means we've pushed it to obscure // the menu item. in this case pretend it's not connected. return Rectangle.Empty; case ToolStripDropDownDirection.Right: case ToolStripDropDownDirection.Left: return Rectangle.Empty; // Consider... the shadow effect interferes with the connected area. // return new Rectangle(bounds.Width-2, 1, 2, itemBounds.Height-2); } } } } return Rectangle.Empty; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
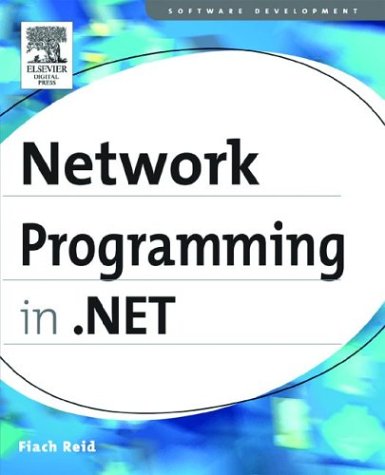
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- figurelengthconverter.cs
- Matrix.cs
- WasHttpModulesInstallComponent.cs
- SystemIPv6InterfaceProperties.cs
- control.ime.cs
- XmlMapping.cs
- ItemDragEvent.cs
- EdmTypeAttribute.cs
- PropertyGridEditorPart.cs
- MetadataCollection.cs
- ScriptResourceInfo.cs
- followingsibling.cs
- WsrmMessageInfo.cs
- Keywords.cs
- HttpStaticObjectsCollectionBase.cs
- RowUpdatedEventArgs.cs
- DbConnectionPool.cs
- tooltip.cs
- MessageSecurityProtocolFactory.cs
- URLAttribute.cs
- PhysicalAddress.cs
- ApplicationSecurityInfo.cs
- TreePrinter.cs
- AccessedThroughPropertyAttribute.cs
- Executor.cs
- SoapIgnoreAttribute.cs
- TraceContextEventArgs.cs
- SafeCertificateContext.cs
- MarkupWriter.cs
- ControlParameter.cs
- DispatchOperation.cs
- TripleDESCryptoServiceProvider.cs
- Column.cs
- WebPartsPersonalizationAuthorization.cs
- SortableBindingList.cs
- ReferentialConstraint.cs
- UrlAuthorizationModule.cs
- ContractCodeDomInfo.cs
- ObjectQuery_EntitySqlExtensions.cs
- sqlinternaltransaction.cs
- XmlDataLoader.cs
- SecureUICommand.cs
- MessageBox.cs
- DbProviderFactory.cs
- RequestTimeoutManager.cs
- DeclarationUpdate.cs
- LookupNode.cs
- Table.cs
- LicenseException.cs
- DiagnosticStrings.cs
- SystemWebCachingSectionGroup.cs
- XmlReaderDelegator.cs
- StrokeNodeOperations2.cs
- XmlTextReaderImplHelpers.cs
- PagedDataSource.cs
- ProcessingInstructionAction.cs
- EventManager.cs
- FilteredSchemaElementLookUpTable.cs
- StylusEditingBehavior.cs
- httpserverutility.cs
- SecurityTraceRecordHelper.cs
- URLAttribute.cs
- Wrapper.cs
- SqlLiftIndependentRowExpressions.cs
- XmlResolver.cs
- MgmtConfigurationRecord.cs
- XPathException.cs
- WaitHandleCannotBeOpenedException.cs
- ThreadNeutralSemaphore.cs
- LinqMaximalSubtreeNominator.cs
- SharedStatics.cs
- ParagraphResult.cs
- UnlockInstanceAsyncResult.cs
- ContractComponent.cs
- PropertyItemInternal.cs
- Lease.cs
- OrderedHashRepartitionEnumerator.cs
- externdll.cs
- TypeTypeConverter.cs
- EdmItemCollection.cs
- ActivityExecutorOperation.cs
- ServicePoint.cs
- TreeView.cs
- EdmProviderManifest.cs
- DataGridColumn.cs
- Mappings.cs
- NameObjectCollectionBase.cs
- SocketAddress.cs
- RowToFieldTransformer.cs
- DataPointer.cs
- ConfigXmlWhitespace.cs
- ElementHostPropertyMap.cs
- PageContent.cs
- MobileResource.cs
- KeyedCollection.cs
- DiscoveryVersionConverter.cs
- SupportsEventValidationAttribute.cs
- MailBnfHelper.cs
- BindingOperations.cs
- DataGridViewComboBoxColumnDesigner.cs