Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / TreePrinter.cs / 1305376 / TreePrinter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Text; namespace System.Data.Common.Utils { ////// Represents a node in a hierarchical collection of information strings. /// Intended as a common way mechanism to represent tree structures for debugging (using the TreePrinter class). /// A node consists of a string (represented as a StringBuilder), its collection of child nodes, and an optional Tag value. /// internal class TreeNode { private StringBuilder _text; private List_children = new List (); private int _position; // Default constructor internal TreeNode() { _text = new StringBuilder(); } /// /// Constructs a new TreeNode with the specified text, tag value and child nodes /// /// The initial value of the new node's text /// An optional list of initial child nodes internal TreeNode(string text, params TreeNode[] children) { if (string.IsNullOrEmpty(text)) { _text = new StringBuilder(); } else { _text = new StringBuilder(text); } if (children != null) { _children.AddRange(children); } } // IEnumerable convenience constructors internal TreeNode(string text, Listchildren) : this(text) { if (children != null) { _children.AddRange(children); } } // 'public' properties /// /// The current text of this node. /// internal StringBuilder Text { get { return _text; } } ////// The collection of child nodes for this node, which may be empty. /// internal IListChildren { get { return _children; } } // Used only by the TreePrinter when generating the output string internal int Position { get { return _position; } set { _position = value; } } } /// /// Generates a formatted string from a hierarchy of tree nodes. Derived types may override /// the PreProcess, Before/AfterAppend, Print, PrintNode and PrintChildren methods to add /// specific functionality at particular points in process of building the string. /// internal abstract class TreePrinter { #region Private Instance Members private List_scopes = new List (); private bool _showLines = true; private char _horizontals = '_'; private char _verticals = '|'; #endregion #region 'Public' API /// /// Entry point method for the TreePrinter /// /// The TreeNode instance that is the root of the tree to be printed ///A string representation of the specified tree internal virtual string Print(TreeNode node) { this.PreProcess(node); StringBuilder text = new StringBuilder(); PrintNode(text, node); return text.ToString(); } #endregion #region 'Protected' API // 'protected' constructor internal TreePrinter() { } // 'protected' API that may be overriden to customize printing ////// Called once on the root of the tree before printing begins /// /// The TreeNode that is the root of the tree internal virtual void PreProcess(TreeNode node) { } ////// Called once for every node after indentation, connecting lines and the node's text value /// have been added to the output but before the line suffix (if any) has been added. /// /// The current node /// The StringBuilder into which the tree is being printed internal virtual void AfterAppend(TreeNode node, StringBuilder text) { } ////// Called once for every node immediately after the line prefix (if any) and appropriate /// indentation and connecting lines have been added to the output but before the node's /// text value has been added. /// /// The current node /// The StringBuilder into which the tree is being printed internal virtual void BeforeAppend(TreeNode node, StringBuilder text) { } ////// The recursive step of the printing process, called once for each TreeNode in the tree /// /// The StringBuilder into which the tree is being printed /// The current node that should be printed to the StringBuilder internal virtual void PrintNode(StringBuilder text, TreeNode node) { IndentLine(text); this.BeforeAppend(node, text); text.Append(node.Text.ToString()); this.AfterAppend(node, text); PrintChildren(text, node); } ////// Called to recursively visit the child nodes of the current TreeNode. /// /// The StringBuilder into which the tree is being printed /// The current node internal virtual void PrintChildren(StringBuilder text, TreeNode node) { _scopes.Add(node); node.Position = 0; foreach (TreeNode childNode in node.Children) { text.AppendLine(); node.Position++; PrintNode(text, childNode); } _scopes.RemoveAt(_scopes.Count - 1); } #endregion #region Private Implementation private void IndentLine(StringBuilder text) { int idx = 0; for (int scopeIdx = 0; scopeIdx < _scopes.Count; scopeIdx++) { TreeNode parentScope = _scopes[scopeIdx]; if (!_showLines || (parentScope.Position == parentScope.Children.Count && scopeIdx != _scopes.Count - 1)) { text.Append(' '); } else { text.Append(_verticals); } idx++; if (_scopes.Count == idx && _showLines) { text.Append(_horizontals); } else { text.Append(' '); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Text; namespace System.Data.Common.Utils { ////// Represents a node in a hierarchical collection of information strings. /// Intended as a common way mechanism to represent tree structures for debugging (using the TreePrinter class). /// A node consists of a string (represented as a StringBuilder), its collection of child nodes, and an optional Tag value. /// internal class TreeNode { private StringBuilder _text; private List_children = new List (); private int _position; // Default constructor internal TreeNode() { _text = new StringBuilder(); } /// /// Constructs a new TreeNode with the specified text, tag value and child nodes /// /// The initial value of the new node's text /// An optional list of initial child nodes internal TreeNode(string text, params TreeNode[] children) { if (string.IsNullOrEmpty(text)) { _text = new StringBuilder(); } else { _text = new StringBuilder(text); } if (children != null) { _children.AddRange(children); } } // IEnumerable convenience constructors internal TreeNode(string text, Listchildren) : this(text) { if (children != null) { _children.AddRange(children); } } // 'public' properties /// /// The current text of this node. /// internal StringBuilder Text { get { return _text; } } ////// The collection of child nodes for this node, which may be empty. /// internal IListChildren { get { return _children; } } // Used only by the TreePrinter when generating the output string internal int Position { get { return _position; } set { _position = value; } } } /// /// Generates a formatted string from a hierarchy of tree nodes. Derived types may override /// the PreProcess, Before/AfterAppend, Print, PrintNode and PrintChildren methods to add /// specific functionality at particular points in process of building the string. /// internal abstract class TreePrinter { #region Private Instance Members private List_scopes = new List (); private bool _showLines = true; private char _horizontals = '_'; private char _verticals = '|'; #endregion #region 'Public' API /// /// Entry point method for the TreePrinter /// /// The TreeNode instance that is the root of the tree to be printed ///A string representation of the specified tree internal virtual string Print(TreeNode node) { this.PreProcess(node); StringBuilder text = new StringBuilder(); PrintNode(text, node); return text.ToString(); } #endregion #region 'Protected' API // 'protected' constructor internal TreePrinter() { } // 'protected' API that may be overriden to customize printing ////// Called once on the root of the tree before printing begins /// /// The TreeNode that is the root of the tree internal virtual void PreProcess(TreeNode node) { } ////// Called once for every node after indentation, connecting lines and the node's text value /// have been added to the output but before the line suffix (if any) has been added. /// /// The current node /// The StringBuilder into which the tree is being printed internal virtual void AfterAppend(TreeNode node, StringBuilder text) { } ////// Called once for every node immediately after the line prefix (if any) and appropriate /// indentation and connecting lines have been added to the output but before the node's /// text value has been added. /// /// The current node /// The StringBuilder into which the tree is being printed internal virtual void BeforeAppend(TreeNode node, StringBuilder text) { } ////// The recursive step of the printing process, called once for each TreeNode in the tree /// /// The StringBuilder into which the tree is being printed /// The current node that should be printed to the StringBuilder internal virtual void PrintNode(StringBuilder text, TreeNode node) { IndentLine(text); this.BeforeAppend(node, text); text.Append(node.Text.ToString()); this.AfterAppend(node, text); PrintChildren(text, node); } ////// Called to recursively visit the child nodes of the current TreeNode. /// /// The StringBuilder into which the tree is being printed /// The current node internal virtual void PrintChildren(StringBuilder text, TreeNode node) { _scopes.Add(node); node.Position = 0; foreach (TreeNode childNode in node.Children) { text.AppendLine(); node.Position++; PrintNode(text, childNode); } _scopes.RemoveAt(_scopes.Count - 1); } #endregion #region Private Implementation private void IndentLine(StringBuilder text) { int idx = 0; for (int scopeIdx = 0; scopeIdx < _scopes.Count; scopeIdx++) { TreeNode parentScope = _scopes[scopeIdx]; if (!_showLines || (parentScope.Position == parentScope.Children.Count && scopeIdx != _scopes.Count - 1)) { text.Append(' '); } else { text.Append(_verticals); } idx++; if (_scopes.Count == idx && _showLines) { text.Append(_horizontals); } else { text.Append(' '); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
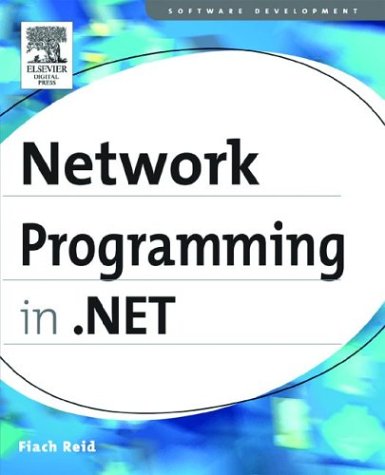
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpResponse.cs
- ReadOnlyMetadataCollection.cs
- HandleRef.cs
- CapabilitiesState.cs
- ErrorReporting.cs
- AsnEncodedData.cs
- RequestBringIntoViewEventArgs.cs
- SyntaxCheck.cs
- TitleStyle.cs
- ErrorLog.cs
- ProtocolViolationException.cs
- ManifestResourceInfo.cs
- RtfControls.cs
- DynamicActionMessageFilter.cs
- GridItemPatternIdentifiers.cs
- XmlSchemaAttributeGroupRef.cs
- Blend.cs
- LogicalExpr.cs
- FlowDocumentReaderAutomationPeer.cs
- SchemaElementDecl.cs
- StylusShape.cs
- XPathNodeList.cs
- CodeExpressionStatement.cs
- MouseButton.cs
- TableRow.cs
- BuildProvider.cs
- FileIOPermission.cs
- FileSystemInfo.cs
- hebrewshape.cs
- BidPrivateBase.cs
- RemotingConfiguration.cs
- _Connection.cs
- DataRowChangeEvent.cs
- XPathDocumentIterator.cs
- ProxyAssemblyNotLoadedException.cs
- IImplicitResourceProvider.cs
- ImpersonationContext.cs
- HierarchicalDataSourceControl.cs
- WebBrowser.cs
- XmlElementCollection.cs
- HtmlUtf8RawTextWriter.cs
- RuntimeResourceSet.cs
- ITextView.cs
- SplitterCancelEvent.cs
- ConnectionsZoneDesigner.cs
- UInt32.cs
- MD5.cs
- DelegateInArgument.cs
- InlinedAggregationOperator.cs
- SqlColumnizer.cs
- TraceHandlerErrorFormatter.cs
- Int64.cs
- Comparer.cs
- ControlCachePolicy.cs
- XPathAncestorIterator.cs
- BindingElement.cs
- SafeNativeMemoryHandle.cs
- SqlCharStream.cs
- XmlSchemaAttribute.cs
- SmiEventSink_Default.cs
- x509store.cs
- DefaultMemberAttribute.cs
- XmlName.cs
- JpegBitmapDecoder.cs
- DbProviderSpecificTypePropertyAttribute.cs
- RSAPKCS1SignatureDeformatter.cs
- MultiPageTextView.cs
- TypeBuilder.cs
- HandlerBase.cs
- XmlSchemaType.cs
- RsaSecurityToken.cs
- MemoryRecordBuffer.cs
- PointConverter.cs
- ExtentCqlBlock.cs
- RecognizerStateChangedEventArgs.cs
- KeyFrames.cs
- CollaborationHelperFunctions.cs
- ByteRangeDownloader.cs
- DummyDataSource.cs
- SHA256.cs
- InvalidDataException.cs
- ScriptManager.cs
- RegexGroupCollection.cs
- MaterializeFromAtom.cs
- Constant.cs
- BeginStoryboard.cs
- CellLabel.cs
- ExpressionPrefixAttribute.cs
- CallbackValidatorAttribute.cs
- GridViewColumnCollection.cs
- ThreadPool.cs
- RuleSetCollection.cs
- Label.cs
- Cursor.cs
- DataGridCaption.cs
- ProfileBuildProvider.cs
- Metadata.cs
- PeerMaintainer.cs
- CompressStream.cs
- NonSerializedAttribute.cs