Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / ParallelTimeline.cs / 1 / ParallelTimeline.cs
//---------------------------------------------------------------------------- //// Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; namespace System.Windows.Media.Animation { ////// This class represents a group of Timelines where the children /// become active according to the value of their Begin property rather /// than their specific order in the Children collection. Children /// are also able to overlap and run in parallel with each other. /// public partial class ParallelTimeline : TimelineGroup { #region Constructors ////// Creates a ParallelTimeline with default properties. /// public ParallelTimeline() : base() { } ////// Creates a ParallelTimeline with the specified BeginTime. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime) : base(beginTime) { } ////// Creates a ParallelTimeline with the specified begin time and duration. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration) : base(beginTime, duration) { } ////// Creates a ParallelTimeline with the specified BeginTime, Duration and RepeatBehavior. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// /// /// The RepeatBehavior for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration, RepeatBehavior repeatBehavior) : base(beginTime, duration, repeatBehavior) { } #endregion #region Methods ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { Duration simpleDuration = TimeSpan.Zero; ClockGroup clockGroup = clock as ClockGroup; if (clockGroup != null) { Listchildren = clockGroup.InternalChildren; // The container ends when all of its children have ended at least // one of their active periods. if (children != null) { bool hasChildWithUnresolvedDuration = false; for (int childIndex = 0; childIndex < children.Count; childIndex++) { Duration childEndOfActivePeriod = children[childIndex].EndOfActivePeriod; if (childEndOfActivePeriod == Duration.Forever) { // If we have even one child with a duration of forever // our resolved duration will also be forever. It doesn't // matter if other children have unresolved durations. return Duration.Forever; } else if (childEndOfActivePeriod == Duration.Automatic) { hasChildWithUnresolvedDuration = true; } else if (childEndOfActivePeriod > simpleDuration) { simpleDuration = childEndOfActivePeriod; } } // We've iterated through all our children. We know that at this // point none of them have a duration of Forever or we would have // returned already. If any of them still have unresolved // durations then our duration is also still unresolved and we // will return automatic. Otherwise, we'll fall out of the 'if' // block and return the simpleDuration as our final resolved // duration. if (hasChildWithUnresolvedDuration) { return Duration.Automatic; } } } return simpleDuration; } #endregion #region SlipBehavior Property /// /// SlipBehavior Property /// public static readonly DependencyProperty SlipBehaviorProperty = DependencyProperty.Register( "SlipBehavior", typeof(SlipBehavior), typeof(ParallelTimeline), new PropertyMetadata( SlipBehavior.Grow, new PropertyChangedCallback(ParallelTimeline_PropertyChangedFunction)), new ValidateValueCallback(ValidateSlipBehavior)); private static bool ValidateSlipBehavior(object value) { return TimeEnumHelper.IsValidSlipBehavior((SlipBehavior)value); } ////// Returns the SlipBehavior for this ClockGroup /// [DefaultValue(SlipBehavior.Grow)] public SlipBehavior SlipBehavior { get { return (SlipBehavior)GetValue(SlipBehaviorProperty); } set { SetValue(SlipBehaviorProperty, value); } } internal static void ParallelTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((ParallelTimeline)d).PropertyChanged(e.Property); } #endregion // SlipBehavior Property } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- //// Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; namespace System.Windows.Media.Animation { ////// This class represents a group of Timelines where the children /// become active according to the value of their Begin property rather /// than their specific order in the Children collection. Children /// are also able to overlap and run in parallel with each other. /// public partial class ParallelTimeline : TimelineGroup { #region Constructors ////// Creates a ParallelTimeline with default properties. /// public ParallelTimeline() : base() { } ////// Creates a ParallelTimeline with the specified BeginTime. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime) : base(beginTime) { } ////// Creates a ParallelTimeline with the specified begin time and duration. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration) : base(beginTime, duration) { } ////// Creates a ParallelTimeline with the specified BeginTime, Duration and RepeatBehavior. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// /// /// The RepeatBehavior for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration, RepeatBehavior repeatBehavior) : base(beginTime, duration, repeatBehavior) { } #endregion #region Methods ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { Duration simpleDuration = TimeSpan.Zero; ClockGroup clockGroup = clock as ClockGroup; if (clockGroup != null) { Listchildren = clockGroup.InternalChildren; // The container ends when all of its children have ended at least // one of their active periods. if (children != null) { bool hasChildWithUnresolvedDuration = false; for (int childIndex = 0; childIndex < children.Count; childIndex++) { Duration childEndOfActivePeriod = children[childIndex].EndOfActivePeriod; if (childEndOfActivePeriod == Duration.Forever) { // If we have even one child with a duration of forever // our resolved duration will also be forever. It doesn't // matter if other children have unresolved durations. return Duration.Forever; } else if (childEndOfActivePeriod == Duration.Automatic) { hasChildWithUnresolvedDuration = true; } else if (childEndOfActivePeriod > simpleDuration) { simpleDuration = childEndOfActivePeriod; } } // We've iterated through all our children. We know that at this // point none of them have a duration of Forever or we would have // returned already. If any of them still have unresolved // durations then our duration is also still unresolved and we // will return automatic. Otherwise, we'll fall out of the 'if' // block and return the simpleDuration as our final resolved // duration. if (hasChildWithUnresolvedDuration) { return Duration.Automatic; } } } return simpleDuration; } #endregion #region SlipBehavior Property /// /// SlipBehavior Property /// public static readonly DependencyProperty SlipBehaviorProperty = DependencyProperty.Register( "SlipBehavior", typeof(SlipBehavior), typeof(ParallelTimeline), new PropertyMetadata( SlipBehavior.Grow, new PropertyChangedCallback(ParallelTimeline_PropertyChangedFunction)), new ValidateValueCallback(ValidateSlipBehavior)); private static bool ValidateSlipBehavior(object value) { return TimeEnumHelper.IsValidSlipBehavior((SlipBehavior)value); } ////// Returns the SlipBehavior for this ClockGroup /// [DefaultValue(SlipBehavior.Grow)] public SlipBehavior SlipBehavior { get { return (SlipBehavior)GetValue(SlipBehaviorProperty); } set { SetValue(SlipBehaviorProperty, value); } } internal static void ParallelTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((ParallelTimeline)d).PropertyChanged(e.Property); } #endregion // SlipBehavior Property } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
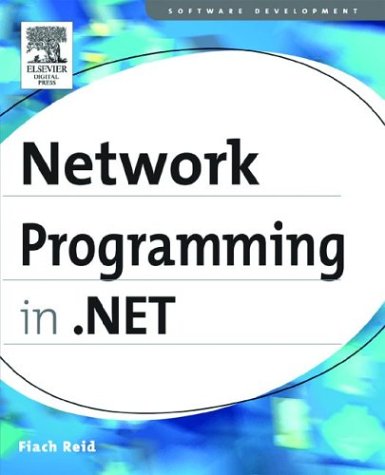
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EFColumnProvider.cs
- CodeCommentStatementCollection.cs
- BitmapSizeOptions.cs
- ConstraintManager.cs
- ByteBufferPool.cs
- BuildDependencySet.cs
- XamlContextStack.cs
- XmlNamespaceDeclarationsAttribute.cs
- WebPartUtil.cs
- MetadataSerializer.cs
- CompiledRegexRunnerFactory.cs
- ForceCopyBuildProvider.cs
- Win32SafeHandles.cs
- SchemaInfo.cs
- Splitter.cs
- WebRequestModulesSection.cs
- InfoCardRSACryptoProvider.cs
- DeclarationUpdate.cs
- Signature.cs
- MenuItemStyle.cs
- AuthorizationSection.cs
- LinkUtilities.cs
- HttpApplicationFactory.cs
- Misc.cs
- GacUtil.cs
- UrlPath.cs
- EmptyEnumerator.cs
- MatrixUtil.cs
- Stacktrace.cs
- controlskin.cs
- ISAPIApplicationHost.cs
- ComboBoxRenderer.cs
- PersonalizationProviderCollection.cs
- RootAction.cs
- BoolLiteral.cs
- Errors.cs
- Animatable.cs
- TransformedBitmap.cs
- XmlAutoDetectWriter.cs
- RectAnimationUsingKeyFrames.cs
- ScrollBar.cs
- ModelUIElement3D.cs
- XmlIncludeAttribute.cs
- TypedTableGenerator.cs
- DocumentPageTextView.cs
- ClassHandlersStore.cs
- TabControlDesigner.cs
- ObjectStateManager.cs
- PlanCompiler.cs
- ServiceControllerDesigner.cs
- BitmapEffectInput.cs
- SystemInfo.cs
- OutputScope.cs
- ExcludeFromCodeCoverageAttribute.cs
- ActiveDocumentEvent.cs
- MsmqHostedTransportManager.cs
- IIS7WorkerRequest.cs
- MD5Cng.cs
- ReadOnlyCollection.cs
- TypeConverterHelper.cs
- StoragePropertyMapping.cs
- BuiltInExpr.cs
- StylusDownEventArgs.cs
- Baml2006KnownTypes.cs
- InputScope.cs
- VirtualPathUtility.cs
- PenThreadPool.cs
- CopyOnWriteList.cs
- OneOf.cs
- FunctionQuery.cs
- SubMenuStyleCollection.cs
- SafeCertificateStore.cs
- PublisherIdentityPermission.cs
- _NtlmClient.cs
- DerivedKeySecurityTokenStub.cs
- PartialCachingAttribute.cs
- cryptoapiTransform.cs
- TreeViewItem.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- GlyphRun.cs
- DetailsViewModeEventArgs.cs
- mansign.cs
- WebPartZoneBaseDesigner.cs
- FontFamilyConverter.cs
- CorePropertiesFilter.cs
- HttpClientCertificate.cs
- WmpBitmapEncoder.cs
- FilteredDataSetHelper.cs
- PropertyConverter.cs
- TemplateControl.cs
- ProcessDesigner.cs
- SafeThreadHandle.cs
- ContextMenu.cs
- DBCommandBuilder.cs
- QuerySelectOp.cs
- EventLogger.cs
- DescendentsWalker.cs
- BitmapEffectGroup.cs
- XmlRawWriter.cs
- ObjectAnimationBase.cs