Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / Command / KeyBinding.cs / 1 / KeyBinding.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyBinding class is used by the developer to create Keyboard Input Bindings // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // //* KeyBinding class serves the purpose of Input Bindings for Keyboard Device. // // History: // 06/01/2003 : chandras - Created // 05/01/2004 : chandra - changed to accommodate new design // ( http://avalon/coreui/Specs/Commanding(new).mht ) //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.ComponentModel; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// KeyBinding - Implements InputBinding (generic InputGesture-Command map) /// KeyBinding acts like a map for KeyGesture and Commands. /// Most of the logic is in InputBinding and KeyGesture, this only /// facilitates user to add Key/Modifiers directly without going in /// KeyGesture path. Also it provides the KeyGestureTypeConverter /// on the Gesture property to have KeyGesture, like Ctrl+X, Alt+V /// defined in Markup as Gesture="Ctrl+X" working /// public class KeyBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor ////// Constructor /// public KeyBinding() : base() { } ////// Constructor /// /// Command associated /// KeyGesture associated public KeyBinding(ICommand command, KeyGesture gesture) : base(command, gesture) { } ////// Constructor /// /// /// modifiers /// key public KeyBinding(ICommand command, Key key, ModifierKeys modifiers) : base(command, new KeyGesture(key, modifiers)) { } #endregion Constructor //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// KeyGesture Override, to ensure type-safety and provide a /// TypeConverter for KeyGesture /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as KeyGesture; } set { if (value is KeyGesture) { base.Gesture = value; } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(KeyGesture))); } } } ////// Modifier /// public ModifierKeys Modifiers { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Modifiers; } return ModifierKeys.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture(Key.None, (ModifierKeys)value, /*validateGesture = */ false); } else { Gesture = new KeyGesture(((KeyGesture)Gesture).Key, value, /*validateGesture = */ false); } } } } ////// Key /// public Key Key { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Key; } return Key.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture((Key)value, ModifierKeys.None, /*validateGesture = */ false); } else { Gesture = new KeyGesture(value, ((KeyGesture)Gesture).Modifiers, /*validateGesture = */ false); } } } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyBinding class is used by the developer to create Keyboard Input Bindings // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // //* KeyBinding class serves the purpose of Input Bindings for Keyboard Device. // // History: // 06/01/2003 : chandras - Created // 05/01/2004 : chandra - changed to accommodate new design // ( http://avalon/coreui/Specs/Commanding(new).mht ) //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.ComponentModel; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// KeyBinding - Implements InputBinding (generic InputGesture-Command map) /// KeyBinding acts like a map for KeyGesture and Commands. /// Most of the logic is in InputBinding and KeyGesture, this only /// facilitates user to add Key/Modifiers directly without going in /// KeyGesture path. Also it provides the KeyGestureTypeConverter /// on the Gesture property to have KeyGesture, like Ctrl+X, Alt+V /// defined in Markup as Gesture="Ctrl+X" working /// public class KeyBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor ////// Constructor /// public KeyBinding() : base() { } ////// Constructor /// /// Command associated /// KeyGesture associated public KeyBinding(ICommand command, KeyGesture gesture) : base(command, gesture) { } ////// Constructor /// /// /// modifiers /// key public KeyBinding(ICommand command, Key key, ModifierKeys modifiers) : base(command, new KeyGesture(key, modifiers)) { } #endregion Constructor //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// KeyGesture Override, to ensure type-safety and provide a /// TypeConverter for KeyGesture /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as KeyGesture; } set { if (value is KeyGesture) { base.Gesture = value; } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(KeyGesture))); } } } ////// Modifier /// public ModifierKeys Modifiers { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Modifiers; } return ModifierKeys.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture(Key.None, (ModifierKeys)value, /*validateGesture = */ false); } else { Gesture = new KeyGesture(((KeyGesture)Gesture).Key, value, /*validateGesture = */ false); } } } } ////// Key /// public Key Key { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Key; } return Key.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture((Key)value, ModifierKeys.None, /*validateGesture = */ false); } else { Gesture = new KeyGesture(value, ((KeyGesture)Gesture).Modifiers, /*validateGesture = */ false); } } } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
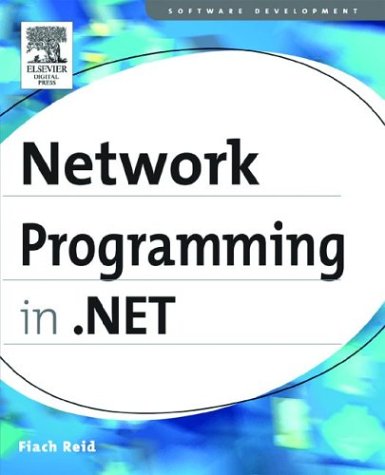
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Win32Interop.cs
- PrintControllerWithStatusDialog.cs
- ProcessHostServerConfig.cs
- ParameterCollection.cs
- WsatTransactionInfo.cs
- TextSchema.cs
- PEFileEvidenceFactory.cs
- shaperfactoryquerycacheentry.cs
- UserPersonalizationStateInfo.cs
- IntegrationExceptionEventArgs.cs
- ModelServiceImpl.cs
- DoubleAnimationUsingKeyFrames.cs
- TextProperties.cs
- WorkflowNamespace.cs
- DbInsertCommandTree.cs
- InvokeProviderWrapper.cs
- StrongNameUtility.cs
- FrameworkElement.cs
- Calendar.cs
- RsaSecurityKey.cs
- CodeAttributeDeclaration.cs
- WebPartEditorOkVerb.cs
- Viewport2DVisual3D.cs
- QilTernary.cs
- Descriptor.cs
- CurrentChangingEventManager.cs
- XmlElement.cs
- StyleBamlTreeBuilder.cs
- DataGridTextBoxColumn.cs
- VersionValidator.cs
- FileNotFoundException.cs
- DirectoryInfo.cs
- UnionExpr.cs
- SplineKeyFrames.cs
- BasePropertyDescriptor.cs
- ExpandCollapsePattern.cs
- DrawingContext.cs
- ImpersonateTokenRef.cs
- MemoryMappedView.cs
- IisTraceWebEventProvider.cs
- thaishape.cs
- DesignerValidatorAdapter.cs
- BulletedListEventArgs.cs
- FixedLineResult.cs
- ManagementObjectSearcher.cs
- ConstantProjectedSlot.cs
- ResourceExpression.cs
- DynamicResourceExtensionConverter.cs
- ImplicitInputBrush.cs
- LocalBuilder.cs
- BitmapSourceSafeMILHandle.cs
- FillBehavior.cs
- SecurityHeaderElementInferenceEngine.cs
- TraceProvider.cs
- OptimizedTemplateContent.cs
- Point.cs
- ReadOnlyObservableCollection.cs
- MultiSelectRootGridEntry.cs
- AesManaged.cs
- Stack.cs
- GeneralTransform3D.cs
- ClientProxyGenerator.cs
- HttpCookieCollection.cs
- Sql8ExpressionRewriter.cs
- SchemaImporterExtensionsSection.cs
- QueryCursorEventArgs.cs
- ContentValidator.cs
- TextBoxBase.cs
- InertiaExpansionBehavior.cs
- DictionarySectionHandler.cs
- NodeFunctions.cs
- AsyncPostBackErrorEventArgs.cs
- ReferenceService.cs
- TrackingCondition.cs
- Token.cs
- MailMessage.cs
- GeometryModel3D.cs
- Mappings.cs
- WebPartVerbsEventArgs.cs
- SelfIssuedSamlTokenFactory.cs
- ProfileManager.cs
- RadioButton.cs
- CapabilitiesAssignment.cs
- GridViewColumnHeaderAutomationPeer.cs
- _ConnectionGroup.cs
- Button.cs
- VideoDrawing.cs
- BooleanAnimationUsingKeyFrames.cs
- FlowPosition.cs
- RuntimeConfigurationRecord.cs
- ToolStripRenderEventArgs.cs
- EventHandlingScope.cs
- UIElement.cs
- CodeTypeParameterCollection.cs
- TextBox.cs
- OleDbStruct.cs
- SqlInternalConnectionSmi.cs
- PageCatalogPart.cs
- ValueChangedEventManager.cs
- DataSetFieldSchema.cs