Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / EventHandlersStore.cs / 1 / EventHandlersStore.cs
using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using MS.Utility; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Container for the event handlers /// ////// EventHandlersStore is a hashtable /// of handlers for a given /// EventPrivateKey or RoutedEvent /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.LinkDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] [FriendAccessAllowed] // Built into Core, also used by Framework. internal class EventHandlersStore { #region Construction ////// Constructor for EventHandlersStore /// public EventHandlersStore() { _entries = new FrugalMap(); } ////// Copy constructor for EventHandlersStore /// public EventHandlersStore(EventHandlersStore source) { _entries = source._entries; } #endregion Construction #region ExternalAPI ////// Adds a Clr event handler for the /// given EventPrivateKey to the store /// /// /// Private key for the event /// /// /// Event handler /// public void Add(EventPrivateKey key, Delegate handler) { if (key == null) { throw new ArgumentNullException("key"); } if (handler == null) { throw new ArgumentNullException("handler"); } // Get the entry corresponding to the given key Delegate existingDelegate = (Delegate)this[key]; if (existingDelegate == null) { _entries[key.GlobalIndex] = handler; } else { _entries[key.GlobalIndex] = Delegate.Combine(existingDelegate, handler); } } ////// Removes an instance of the specified /// Clr event handler for the given /// EventPrivateKey from the store /// /// /// Private key for the event /// /// /// Event handler /// ////// NOTE: This method does nothing if no /// matching handler instances are found /// in the store /// public void Remove(EventPrivateKey key, Delegate handler) { if (key == null) { throw new ArgumentNullException("key"); } if (handler == null) { throw new ArgumentNullException("handler"); } // Get the entry corresponding to the given key Delegate existingDelegate = (Delegate) this[key]; if (existingDelegate != null) { existingDelegate = Delegate.Remove(existingDelegate, handler); if (existingDelegate == null) { // last handler for this event was removed -- reclaim space in // underlying FrugalMap by setting value to DependencyProperty.UnsetValue _entries[key.GlobalIndex] = DependencyProperty.UnsetValue; } else { _entries[key.GlobalIndex] = existingDelegate; } } } ////// Gets all the handlers for the given EventPrivateKey /// /// /// Private key for the event /// ////// Combined delegate or null if no match found /// ////// This method is not exposing a security risk for the reason /// that the EventPrivateKey for the events will themselves be /// private to the declaring class. This will be enforced via fxcop rules. /// public Delegate Get(EventPrivateKey key) { if (key == null) { throw new ArgumentNullException("key"); } // Return the handlers corresponding to the given key return (Delegate)this[key]; } #endregion ExternalAPI #region Operations ////// Adds a routed event handler for the given /// RoutedEvent to the store /// public void AddRoutedEventHandler( RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } // Create a new RoutedEventHandler RoutedEventHandlerInfo routedEventHandlerInfo = new RoutedEventHandlerInfo(handler, handledEventsToo); // Get the entry corresponding to the given RoutedEvent FrugalObjectListhandlers = (FrugalObjectList )this[routedEvent]; if (handlers == null) { _entries[routedEvent.GlobalIndex] = handlers = new FrugalObjectList (1); } // Add the RoutedEventHandlerInfo to the list handlers.Add(routedEventHandlerInfo); } /// /// Removes an instance of the specified /// routed event handler for the given /// RoutedEvent from the store /// ////// NOTE: This method does nothing if no /// matching handler instances are found /// in the store /// public void RemoveRoutedEventHandler(RoutedEvent routedEvent, Delegate handler) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } // Get the entry corresponding to the given RoutedEvent FrugalObjectListhandlers = (FrugalObjectList )this[routedEvent]; if (handlers != null && handlers.Count > 0) { if ((handlers.Count == 1) && (handlers[0].Handler == handler)) { // this is the only handler for this event and it's being removed // reclaim space in underlying FrugalMap by setting value to // DependencyProperty.UnsetValue _entries[routedEvent.GlobalIndex] = DependencyProperty.UnsetValue; } else { // When a matching instance is found remove it for (int i = 0; i < handlers.Count; i++) { if (handlers[i].Handler == handler) { handlers.RemoveAt(i); break; } } } } } /// /// Determines whether the given /// RoutedEvent exists in the store. /// /// /// the RoutedEvent of the event. /// public bool Contains(RoutedEvent routedEvent) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } FrugalObjectListhandlers = (FrugalObjectList )this[routedEvent]; return handlers != null && handlers.Count != 0; } private static void OnEventHandlersIterationCallback(ArrayList list, int key, object value) { RoutedEvent routedEvent = GlobalEventManager.EventFromGlobalIndex(key) as RoutedEvent; if (routedEvent != null && ((FrugalObjectList )value).Count > 0) { list.Add(routedEvent); } } /// /// Get all the event handlers in this store for the given routed event /// public RoutedEventHandlerInfo[] GetRoutedEventHandlers(RoutedEvent routedEvent) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } FrugalObjectListhandlers = this[routedEvent]; if (handlers != null) { return handlers.ToArray(); } return null; } // Returns Handlers for the given key internal FrugalObjectList this[RoutedEvent key] { get { Debug.Assert(key != null, "Search key cannot be null"); object list = _entries[key.GlobalIndex]; if (list == DependencyProperty.UnsetValue) { return null; } else { return (FrugalObjectList )list; } } } internal Delegate this[EventPrivateKey key] { get { Debug.Assert(key != null, "Search key cannot be null"); object existingDelegate = _entries[key.GlobalIndex]; if (existingDelegate == DependencyProperty.UnsetValue) { return null; } else { return (Delegate)existingDelegate; } } } internal int Count { get { return _entries.Count; } } #endregion Operations #region Data // Map of EventPrivateKey/RoutedEvent to Delegate/FrugalObjectList (respectively) private FrugalMap _entries; private static FrugalMapIterationCallback _iterationCallback = new FrugalMapIterationCallback(OnEventHandlersIterationCallback); #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using MS.Utility; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { /// /// Container for the event handlers /// ////// EventHandlersStore is a hashtable /// of handlers for a given /// EventPrivateKey or RoutedEvent /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.LinkDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] [FriendAccessAllowed] // Built into Core, also used by Framework. internal class EventHandlersStore { #region Construction ////// Constructor for EventHandlersStore /// public EventHandlersStore() { _entries = new FrugalMap(); } ////// Copy constructor for EventHandlersStore /// public EventHandlersStore(EventHandlersStore source) { _entries = source._entries; } #endregion Construction #region ExternalAPI ////// Adds a Clr event handler for the /// given EventPrivateKey to the store /// /// /// Private key for the event /// /// /// Event handler /// public void Add(EventPrivateKey key, Delegate handler) { if (key == null) { throw new ArgumentNullException("key"); } if (handler == null) { throw new ArgumentNullException("handler"); } // Get the entry corresponding to the given key Delegate existingDelegate = (Delegate)this[key]; if (existingDelegate == null) { _entries[key.GlobalIndex] = handler; } else { _entries[key.GlobalIndex] = Delegate.Combine(existingDelegate, handler); } } ////// Removes an instance of the specified /// Clr event handler for the given /// EventPrivateKey from the store /// /// /// Private key for the event /// /// /// Event handler /// ////// NOTE: This method does nothing if no /// matching handler instances are found /// in the store /// public void Remove(EventPrivateKey key, Delegate handler) { if (key == null) { throw new ArgumentNullException("key"); } if (handler == null) { throw new ArgumentNullException("handler"); } // Get the entry corresponding to the given key Delegate existingDelegate = (Delegate) this[key]; if (existingDelegate != null) { existingDelegate = Delegate.Remove(existingDelegate, handler); if (existingDelegate == null) { // last handler for this event was removed -- reclaim space in // underlying FrugalMap by setting value to DependencyProperty.UnsetValue _entries[key.GlobalIndex] = DependencyProperty.UnsetValue; } else { _entries[key.GlobalIndex] = existingDelegate; } } } ////// Gets all the handlers for the given EventPrivateKey /// /// /// Private key for the event /// ////// Combined delegate or null if no match found /// ////// This method is not exposing a security risk for the reason /// that the EventPrivateKey for the events will themselves be /// private to the declaring class. This will be enforced via fxcop rules. /// public Delegate Get(EventPrivateKey key) { if (key == null) { throw new ArgumentNullException("key"); } // Return the handlers corresponding to the given key return (Delegate)this[key]; } #endregion ExternalAPI #region Operations ////// Adds a routed event handler for the given /// RoutedEvent to the store /// public void AddRoutedEventHandler( RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } // Create a new RoutedEventHandler RoutedEventHandlerInfo routedEventHandlerInfo = new RoutedEventHandlerInfo(handler, handledEventsToo); // Get the entry corresponding to the given RoutedEvent FrugalObjectListhandlers = (FrugalObjectList )this[routedEvent]; if (handlers == null) { _entries[routedEvent.GlobalIndex] = handlers = new FrugalObjectList (1); } // Add the RoutedEventHandlerInfo to the list handlers.Add(routedEventHandlerInfo); } /// /// Removes an instance of the specified /// routed event handler for the given /// RoutedEvent from the store /// ////// NOTE: This method does nothing if no /// matching handler instances are found /// in the store /// public void RemoveRoutedEventHandler(RoutedEvent routedEvent, Delegate handler) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } // Get the entry corresponding to the given RoutedEvent FrugalObjectListhandlers = (FrugalObjectList )this[routedEvent]; if (handlers != null && handlers.Count > 0) { if ((handlers.Count == 1) && (handlers[0].Handler == handler)) { // this is the only handler for this event and it's being removed // reclaim space in underlying FrugalMap by setting value to // DependencyProperty.UnsetValue _entries[routedEvent.GlobalIndex] = DependencyProperty.UnsetValue; } else { // When a matching instance is found remove it for (int i = 0; i < handlers.Count; i++) { if (handlers[i].Handler == handler) { handlers.RemoveAt(i); break; } } } } } /// /// Determines whether the given /// RoutedEvent exists in the store. /// /// /// the RoutedEvent of the event. /// public bool Contains(RoutedEvent routedEvent) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } FrugalObjectListhandlers = (FrugalObjectList )this[routedEvent]; return handlers != null && handlers.Count != 0; } private static void OnEventHandlersIterationCallback(ArrayList list, int key, object value) { RoutedEvent routedEvent = GlobalEventManager.EventFromGlobalIndex(key) as RoutedEvent; if (routedEvent != null && ((FrugalObjectList )value).Count > 0) { list.Add(routedEvent); } } /// /// Get all the event handlers in this store for the given routed event /// public RoutedEventHandlerInfo[] GetRoutedEventHandlers(RoutedEvent routedEvent) { if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } FrugalObjectListhandlers = this[routedEvent]; if (handlers != null) { return handlers.ToArray(); } return null; } // Returns Handlers for the given key internal FrugalObjectList this[RoutedEvent key] { get { Debug.Assert(key != null, "Search key cannot be null"); object list = _entries[key.GlobalIndex]; if (list == DependencyProperty.UnsetValue) { return null; } else { return (FrugalObjectList )list; } } } internal Delegate this[EventPrivateKey key] { get { Debug.Assert(key != null, "Search key cannot be null"); object existingDelegate = _entries[key.GlobalIndex]; if (existingDelegate == DependencyProperty.UnsetValue) { return null; } else { return (Delegate)existingDelegate; } } } internal int Count { get { return _entries.Count; } } #endregion Operations #region Data // Map of EventPrivateKey/RoutedEvent to Delegate/FrugalObjectList (respectively) private FrugalMap _entries; private static FrugalMapIterationCallback _iterationCallback = new FrugalMapIterationCallback(OnEventHandlersIterationCallback); #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
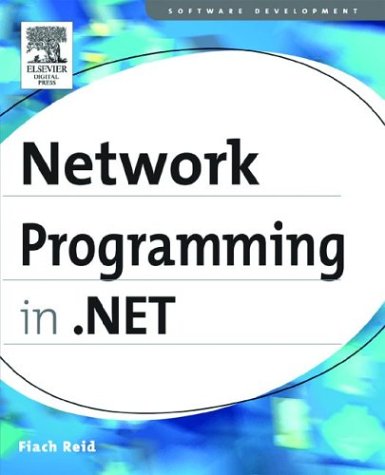
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuleSettings.cs
- SessionIDManager.cs
- ImageMapEventArgs.cs
- HttpPostProtocolReflector.cs
- RotateTransform3D.cs
- TaiwanLunisolarCalendar.cs
- XmlNode.cs
- WorkflowServiceNamespace.cs
- DependentTransaction.cs
- MasterPageBuildProvider.cs
- XpsFilter.cs
- SystemIcmpV4Statistics.cs
- XmlAutoDetectWriter.cs
- XmlStringTable.cs
- Configuration.cs
- HttpsHostedTransportConfiguration.cs
- EditableTreeList.cs
- RelatedImageListAttribute.cs
- XmlSchemaIdentityConstraint.cs
- TextServicesDisplayAttributePropertyRanges.cs
- RegistrySecurity.cs
- EasingQuaternionKeyFrame.cs
- AddInEnvironment.cs
- SQLInt32Storage.cs
- ActivityIdHeader.cs
- ProxyAttribute.cs
- DataBindingCollectionEditor.cs
- TemplateControl.cs
- BackgroundWorker.cs
- SectionInformation.cs
- TextEditorCharacters.cs
- EventlogProvider.cs
- AutoGeneratedFieldProperties.cs
- NavigationExpr.cs
- TrackingServices.cs
- Perspective.cs
- DataGridViewRowHeaderCell.cs
- Type.cs
- BatchWriter.cs
- KeySpline.cs
- MatrixConverter.cs
- InvalidAsynchronousStateException.cs
- ClientSettingsStore.cs
- IssuanceTokenProviderState.cs
- AbstractExpressions.cs
- CryptoStream.cs
- BuildResultCache.cs
- GeneralTransformGroup.cs
- SuppressIldasmAttribute.cs
- Clock.cs
- Point3D.cs
- ObjectDataSourceEventArgs.cs
- TextHidden.cs
- VectorKeyFrameCollection.cs
- SmiContextFactory.cs
- ChannelManager.cs
- ExpanderAutomationPeer.cs
- ClientBuildManagerCallback.cs
- NavigationPropertyAccessor.cs
- VisualTreeHelper.cs
- SimplePropertyEntry.cs
- CodeConstructor.cs
- TreeViewBindingsEditorForm.cs
- XmlReaderDelegator.cs
- SecurityTokenProvider.cs
- ToolTipAutomationPeer.cs
- ClientSettingsProvider.cs
- DataTable.cs
- InfoCardRSACryptoProvider.cs
- CommentEmitter.cs
- Decoder.cs
- ProcessThread.cs
- ScriptControlManager.cs
- ServicesUtilities.cs
- ConfigurationLockCollection.cs
- WindowsScrollBar.cs
- MD5HashHelper.cs
- SamlSerializer.cs
- DbException.cs
- SizeIndependentAnimationStorage.cs
- HtmlInputText.cs
- Transform.cs
- ExpressionBuilder.cs
- ComponentEditorPage.cs
- SystemIPv4InterfaceProperties.cs
- SQLUtility.cs
- CursorConverter.cs
- DataGridViewRowHeaderCell.cs
- __FastResourceComparer.cs
- PathBox.cs
- AppLevelCompilationSectionCache.cs
- SHA512.cs
- UDPClient.cs
- XmlCharCheckingReader.cs
- WS2007HttpBindingElement.cs
- DisplayNameAttribute.cs
- InputMethodStateChangeEventArgs.cs
- Configuration.cs
- XmlWellformedWriter.cs
- PropertyCollection.cs