Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / IO / Packaging / ResponseStream.cs / 1 / ResponseStream.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: ResponseStream.cs // // Description: Exists so that the gc lifetime for the container // and the webresponse are shared. // // This wrapper is returned for any PackWebResponse satisified // with a container. It ensures that the container lives until // the stream is closed because we are unaware of the lifetime of // the stream and the client is unaware of the existence of the // container. // // Container is never closed because it may be used by other // responses. // // History: 11/17/03 - brucemac - created // 12/11/03 - brucemac - adapted from ResponseStream // 15/10/04 - brucemac - adapted from ContainerResponseStream //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.IO.Packaging; // for PackWebResponse using MS.Utility; using System.Windows; namespace MS.Internal.IO.Packaging { ////// Wrap returned stream so we can release the webresponse container when the stream is closed /// internal class ResponseStream : Stream { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Wraps PackWebResponse to ensure correct lifetime handling and stream length functionality /// /// stream to read from (baseStream) /// response /// stream under the package /// container to hold on to internal ResponseStream(Stream s, PackWebResponse response, Stream owningStream, Package container) { Debug.Assert(container != null, "Logic error: use other constructor for full package request streams"); Debug.Assert(owningStream != null, "Logic error: use other constructor for full package request streams"); Init(s, response, owningStream, container); } ////// Wraps stream returned by PackWebResponse to ensure correct lifetime handlingy /// /// stream to read from (baseStream) /// webresponse to close when we close internal ResponseStream(Stream s, PackWebResponse response) { Init(s, response, null, null); } ////// Wraps PackWebResponse to ensure correct lifetime handling and stream length functionality /// /// stream to read from (baseStream) /// stream under the container /// response /// container to hold on to private void Init(Stream s, PackWebResponse response, Stream owningStream, Package container) { Debug.Assert(s != null, "Logic error: base stream cannot be null"); Debug.Assert(response != null, "Logic error: response cannot be null"); _innerStream = s; _response = response; _owningStream = owningStream; _container = container; } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Return the bytes requested /// /// destination buffer /// offset to write into that buffer /// how many bytes requested ///how many bytes were written into buffer ////// Blocks until data is available. /// The read semantics, and in particular the restoration of the position in case of an /// exception, is implemented by the inner stream, i.e. the stream returned by PackWebResponse. /// public override int Read(byte[] buffer, int offset, int count) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.DRXREADSTREAMGUID), EventType.StartEvent, count); } CheckDisposed(); int rslt = _innerStream.Read(buffer, offset, count); if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.DRXREADSTREAMGUID), EventType.EndEvent, rslt); } return rslt; } ////// Seek /// /// only zero is supported /// only SeekOrigin.Begin is supported ///zero public override long Seek(long offset, SeekOrigin origin) { CheckDisposed(); return _innerStream.Seek(offset, origin); } ////// SetLength /// ///not supported public override void SetLength(long newLength) { CheckDisposed(); _innerStream.SetLength(newLength); } ////// Write /// ///not supported public override void Write(byte[] buf, int offset, int count) { CheckDisposed(); _innerStream.Write(buf, offset, count); } ////// Flush /// ///not supported public override void Flush() { CheckDisposed(); _innerStream.Flush(); } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ ////// Is stream readable? /// public override bool CanRead { get { return (!_closed && _innerStream.CanRead); } } ////// Is stream seekable? /// ///We MUST support seek as this is used to implement ILockBytes.ReadAt() public override bool CanSeek { get { return (!_closed && _innerStream.CanSeek); } } ////// Is stream writeable? /// public override bool CanWrite { get { return (!_closed && _innerStream.CanWrite); } } ////// Logical byte position in this stream /// public override long Position { get { CheckDisposed(); return _innerStream.Position; } set { CheckDisposed(); _innerStream.Position = value; } } ////// Length /// public override long Length { get { CheckDisposed(); // inner stream should always know its length because it's based on a local file // or because it's on a NetStream that can fake this return _innerStream.Length; } } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && !_closed) { #if DEBUG if (PackWebRequestFactory._traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation("ContainerResponseStream.Dispose(bool)"); #endif _container = null; // close the Part or NetStream _innerStream.Close(); if (_owningStream != null) { // in this case, the innerStream was the part so this is the NetStream _owningStream.Close(); } } } finally { _innerStream = null; _owningStream = null; _response = null; _closed = true; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { if (_closed) throw new ObjectDisposedException("ResponseStream"); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private bool _closed; // prevent recursion private Stream _innerStream; // stream we are emulating private Package _container; // container to release when we are closed private Stream _owningStream; // stream under the _innerStream when opening a Part private PackWebResponse _response; // packWebResponse we can consult for reliable length } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: ResponseStream.cs // // Description: Exists so that the gc lifetime for the container // and the webresponse are shared. // // This wrapper is returned for any PackWebResponse satisified // with a container. It ensures that the container lives until // the stream is closed because we are unaware of the lifetime of // the stream and the client is unaware of the existence of the // container. // // Container is never closed because it may be used by other // responses. // // History: 11/17/03 - brucemac - created // 12/11/03 - brucemac - adapted from ResponseStream // 15/10/04 - brucemac - adapted from ContainerResponseStream //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.IO.Packaging; // for PackWebResponse using MS.Utility; using System.Windows; namespace MS.Internal.IO.Packaging { ////// Wrap returned stream so we can release the webresponse container when the stream is closed /// internal class ResponseStream : Stream { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Wraps PackWebResponse to ensure correct lifetime handling and stream length functionality /// /// stream to read from (baseStream) /// response /// stream under the package /// container to hold on to internal ResponseStream(Stream s, PackWebResponse response, Stream owningStream, Package container) { Debug.Assert(container != null, "Logic error: use other constructor for full package request streams"); Debug.Assert(owningStream != null, "Logic error: use other constructor for full package request streams"); Init(s, response, owningStream, container); } ////// Wraps stream returned by PackWebResponse to ensure correct lifetime handlingy /// /// stream to read from (baseStream) /// webresponse to close when we close internal ResponseStream(Stream s, PackWebResponse response) { Init(s, response, null, null); } ////// Wraps PackWebResponse to ensure correct lifetime handling and stream length functionality /// /// stream to read from (baseStream) /// stream under the container /// response /// container to hold on to private void Init(Stream s, PackWebResponse response, Stream owningStream, Package container) { Debug.Assert(s != null, "Logic error: base stream cannot be null"); Debug.Assert(response != null, "Logic error: response cannot be null"); _innerStream = s; _response = response; _owningStream = owningStream; _container = container; } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Return the bytes requested /// /// destination buffer /// offset to write into that buffer /// how many bytes requested ///how many bytes were written into buffer ////// Blocks until data is available. /// The read semantics, and in particular the restoration of the position in case of an /// exception, is implemented by the inner stream, i.e. the stream returned by PackWebResponse. /// public override int Read(byte[] buffer, int offset, int count) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.DRXREADSTREAMGUID), EventType.StartEvent, count); } CheckDisposed(); int rslt = _innerStream.Read(buffer, offset, count); if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.DRXREADSTREAMGUID), EventType.EndEvent, rslt); } return rslt; } ////// Seek /// /// only zero is supported /// only SeekOrigin.Begin is supported ///zero public override long Seek(long offset, SeekOrigin origin) { CheckDisposed(); return _innerStream.Seek(offset, origin); } ////// SetLength /// ///not supported public override void SetLength(long newLength) { CheckDisposed(); _innerStream.SetLength(newLength); } ////// Write /// ///not supported public override void Write(byte[] buf, int offset, int count) { CheckDisposed(); _innerStream.Write(buf, offset, count); } ////// Flush /// ///not supported public override void Flush() { CheckDisposed(); _innerStream.Flush(); } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ ////// Is stream readable? /// public override bool CanRead { get { return (!_closed && _innerStream.CanRead); } } ////// Is stream seekable? /// ///We MUST support seek as this is used to implement ILockBytes.ReadAt() public override bool CanSeek { get { return (!_closed && _innerStream.CanSeek); } } ////// Is stream writeable? /// public override bool CanWrite { get { return (!_closed && _innerStream.CanWrite); } } ////// Logical byte position in this stream /// public override long Position { get { CheckDisposed(); return _innerStream.Position; } set { CheckDisposed(); _innerStream.Position = value; } } ////// Length /// public override long Length { get { CheckDisposed(); // inner stream should always know its length because it's based on a local file // or because it's on a NetStream that can fake this return _innerStream.Length; } } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && !_closed) { #if DEBUG if (PackWebRequestFactory._traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation("ContainerResponseStream.Dispose(bool)"); #endif _container = null; // close the Part or NetStream _innerStream.Close(); if (_owningStream != null) { // in this case, the innerStream was the part so this is the NetStream _owningStream.Close(); } } } finally { _innerStream = null; _owningStream = null; _response = null; _closed = true; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { if (_closed) throw new ObjectDisposedException("ResponseStream"); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private bool _closed; // prevent recursion private Stream _innerStream; // stream we are emulating private Package _container; // container to release when we are closed private Stream _owningStream; // stream under the _innerStream when opening a Part private PackWebResponse _response; // packWebResponse we can consult for reliable length } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
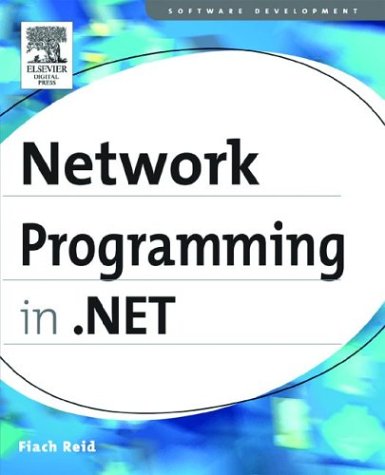
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringInfo.cs
- DataControlFieldCollection.cs
- ExtensionSimplifierMarkupObject.cs
- SchemaConstraints.cs
- CDSCollectionETWBCLProvider.cs
- ChangeProcessor.cs
- WindowAutomationPeer.cs
- XsdSchemaFileEditor.cs
- ReadOnlyKeyedCollection.cs
- PropertyInformationCollection.cs
- DSASignatureDeformatter.cs
- XmlSiteMapProvider.cs
- typedescriptorpermissionattribute.cs
- DataTableMappingCollection.cs
- TreeWalker.cs
- Win32MouseDevice.cs
- TextOutput.cs
- ConsoleCancelEventArgs.cs
- TiffBitmapEncoder.cs
- DrawingContextDrawingContextWalker.cs
- ListControlConvertEventArgs.cs
- BaseDataList.cs
- FlatButtonAppearance.cs
- InputScopeConverter.cs
- TextPointerBase.cs
- OdbcConnectionOpen.cs
- _CacheStreams.cs
- RedirectionProxy.cs
- _TLSstream.cs
- DataGridViewCellValidatingEventArgs.cs
- ListBoxAutomationPeer.cs
- ChtmlTextWriter.cs
- AttachedPropertyBrowsableAttribute.cs
- HttpException.cs
- LockedBorderGlyph.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- QueryServiceConfigHandle.cs
- WinInetCache.cs
- WebHeaderCollection.cs
- EdmToObjectNamespaceMap.cs
- BaseWebProxyFinder.cs
- SqlClientWrapperSmiStream.cs
- WorkflowTransactionService.cs
- SchemaTableColumn.cs
- DropTarget.cs
- TreeNodeStyle.cs
- DataObjectMethodAttribute.cs
- SmtpException.cs
- DiagnosticsConfiguration.cs
- PnrpPermission.cs
- Pair.cs
- RuntimeResourceSet.cs
- ConnectionPoint.cs
- _CommandStream.cs
- LinqDataSourceContextData.cs
- Storyboard.cs
- SizeChangedInfo.cs
- KnownTypesProvider.cs
- TemplateGroupCollection.cs
- ContentPathSegment.cs
- SineEase.cs
- TemplatePartAttribute.cs
- ImageMap.cs
- Operator.cs
- SiteMapDataSource.cs
- LoginDesigner.cs
- InternalCache.cs
- CodeEntryPointMethod.cs
- Util.cs
- OleAutBinder.cs
- ListControl.cs
- MessageLogTraceRecord.cs
- MetadataSource.cs
- HttpRawResponse.cs
- Sql8ExpressionRewriter.cs
- BridgeDataRecord.cs
- VisualStyleRenderer.cs
- CurrencyWrapper.cs
- DataErrorValidationRule.cs
- AssociatedControlConverter.cs
- ExpressionVisitorHelpers.cs
- MobileCategoryAttribute.cs
- ApplicationHost.cs
- AuthenticationService.cs
- CriticalExceptions.cs
- XmlNavigatorStack.cs
- GacUtil.cs
- DesignDataSource.cs
- KeyPressEvent.cs
- userdatakeys.cs
- XslException.cs
- XmlnsCompatibleWithAttribute.cs
- SqlUtils.cs
- RestClientProxyHandler.cs
- WebBrowsableAttribute.cs
- EventLogPermissionEntryCollection.cs
- DataError.cs
- TextDpi.cs
- FontFamily.cs
- ProxyHelper.cs