Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlNavigatorStack.cs / 1 / XmlNavigatorStack.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; namespace System.Xml.Xsl.Runtime { ////// A dynamic stack of IXmlNavigators. /// internal struct XmlNavigatorStack { private XPathNavigator[] stkNav; // Stack of XPathNavigators private int sp; // Stack pointer (size of stack) #if DEBUG private const int InitialStackSize = 2; #else private const int InitialStackSize = 8; #endif ////// Push a navigator onto the stack /// public void Push(XPathNavigator nav) { if (this.stkNav == null) { this.stkNav = new XPathNavigator[InitialStackSize]; } else { if (this.sp >= this.stkNav.Length) { // Resize the stack XPathNavigator[] stkOld = this.stkNav; this.stkNav = new XPathNavigator[2 * this.sp]; Array.Copy(stkOld, this.stkNav, this.sp); } } this.stkNav[this.sp++] = nav; } ////// Pop the topmost navigator and return it /// public XPathNavigator Pop() { Debug.Assert(!IsEmpty); return this.stkNav[--this.sp]; } ////// Returns the navigator at the top of the stack without adjusting the stack pointer /// public XPathNavigator Peek() { Debug.Assert(!IsEmpty); return this.stkNav[this.sp - 1]; } ////// Remove all navigators from the stack /// public void Reset() { this.sp = 0; } ////// Returns true if there are no navigators in the stack /// public bool IsEmpty { get { return this.sp == 0; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; namespace System.Xml.Xsl.Runtime { ////// A dynamic stack of IXmlNavigators. /// internal struct XmlNavigatorStack { private XPathNavigator[] stkNav; // Stack of XPathNavigators private int sp; // Stack pointer (size of stack) #if DEBUG private const int InitialStackSize = 2; #else private const int InitialStackSize = 8; #endif ////// Push a navigator onto the stack /// public void Push(XPathNavigator nav) { if (this.stkNav == null) { this.stkNav = new XPathNavigator[InitialStackSize]; } else { if (this.sp >= this.stkNav.Length) { // Resize the stack XPathNavigator[] stkOld = this.stkNav; this.stkNav = new XPathNavigator[2 * this.sp]; Array.Copy(stkOld, this.stkNav, this.sp); } } this.stkNav[this.sp++] = nav; } ////// Pop the topmost navigator and return it /// public XPathNavigator Pop() { Debug.Assert(!IsEmpty); return this.stkNav[--this.sp]; } ////// Returns the navigator at the top of the stack without adjusting the stack pointer /// public XPathNavigator Peek() { Debug.Assert(!IsEmpty); return this.stkNav[this.sp - 1]; } ////// Remove all navigators from the stack /// public void Reset() { this.sp = 0; } ////// Returns true if there are no navigators in the stack /// public bool IsEmpty { get { return this.sp == 0; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
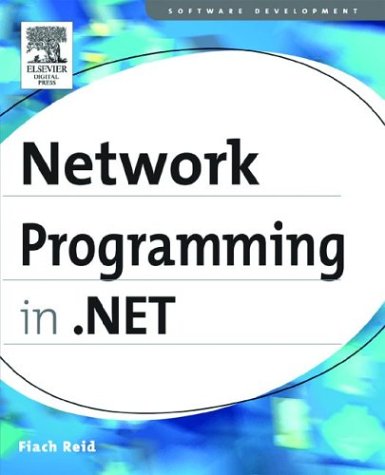
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataMemberConverter.cs
- CodeDelegateCreateExpression.cs
- SqlCaseSimplifier.cs
- KeyValueSerializer.cs
- SystemSounds.cs
- DataTrigger.cs
- Funcletizer.cs
- HijriCalendar.cs
- ObjectQueryExecutionPlan.cs
- DecimalAnimation.cs
- RootBrowserWindowAutomationPeer.cs
- XamlTemplateSerializer.cs
- ping.cs
- PageTheme.cs
- FormatConvertedBitmap.cs
- HtmlShimManager.cs
- PrivateFontCollection.cs
- TextProperties.cs
- _AutoWebProxyScriptWrapper.cs
- SingleKeyFrameCollection.cs
- DockPanel.cs
- EntityDataSourceColumn.cs
- UshortList2.cs
- QueryResults.cs
- ContentPropertyAttribute.cs
- LinkedResourceCollection.cs
- FileUtil.cs
- Types.cs
- DetailsViewUpdateEventArgs.cs
- VerificationException.cs
- TextViewSelectionProcessor.cs
- StdRegProviderWrapper.cs
- UserControlBuildProvider.cs
- WebPartMovingEventArgs.cs
- BufferedStream.cs
- InvalidPrinterException.cs
- HttpCapabilitiesEvaluator.cs
- MultilineStringConverter.cs
- ImageMap.cs
- ValidationSummary.cs
- CrossContextChannel.cs
- BindingManagerDataErrorEventArgs.cs
- BasicExpressionVisitor.cs
- _ContextAwareResult.cs
- templategroup.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- PassportAuthentication.cs
- Relationship.cs
- UIAgentCrashedException.cs
- BinaryConverter.cs
- BypassElementCollection.cs
- XmlDataSourceView.cs
- Rect.cs
- ModuleElement.cs
- JournalEntry.cs
- XamlReader.cs
- LinkArea.cs
- MessageOperationFormatter.cs
- HtmlTableRowCollection.cs
- DoubleAnimation.cs
- Matrix3D.cs
- DataGridGeneralPage.cs
- XmlSchemaType.cs
- ExclusiveTcpListener.cs
- Cursor.cs
- WCFServiceClientProxyGenerator.cs
- SafeHandles.cs
- SafeNativeMemoryHandle.cs
- bidPrivateBase.cs
- SqlConnection.cs
- RegexTypeEditor.cs
- Point3DCollection.cs
- PrtCap_Base.cs
- HandlerElementCollection.cs
- AuthenticationModuleElementCollection.cs
- MetafileHeader.cs
- DirectoryRootQuery.cs
- NotifyIcon.cs
- XappLauncher.cs
- localization.cs
- TypeValidationEventArgs.cs
- XmlIlTypeHelper.cs
- SByte.cs
- MsmqProcessProtocolHandler.cs
- WindowClosedEventArgs.cs
- SplitterPanel.cs
- UInt64Converter.cs
- SafeCryptoHandles.cs
- WindowsPen.cs
- FamilyCollection.cs
- ArrangedElementCollection.cs
- ToolStripDropDownItemDesigner.cs
- sqlcontext.cs
- WebPartTransformer.cs
- ForeignKeyConstraint.cs
- DocumentOrderComparer.cs
- SingleStorage.cs
- XmlAttributeAttribute.cs
- GridViewSortEventArgs.cs
- CompositeKey.cs