Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / ButtonColumn.cs / 2 / ButtonColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ButtonColumn : DataGridColumn { private PropertyDescriptor textFieldDesc; ///Creates a column with a set of ////// controls. /// public ButtonColumn() { } ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), DefaultValue(ButtonColumnType.LinkButton), WebSysDescriptionAttribute(SR.ButtonColumn_ButtonType) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } [ DefaultValue(false), WebSysDescriptionAttribute(SR.ButtonColumn_CausesValidation) ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return false; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Gets or sets the type of button to render in the /// column. ////// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescriptionAttribute(SR.WebControl_CommandName) ] public virtual string CommandName { get { object o = ViewState["CommandName"]; if (o != null) return(string)o; return string.Empty; } set { ViewState["CommandName"] = value; OnColumnChanged(); } } ///Gets or sets the command to perform when this ////// is clicked. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_DataTextField) ] public virtual string DataTextField { get { object o = ViewState["DataTextField"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextField"] = value; OnColumnChanged(); } } ///Gets or sets the field name from the data model that is /// bound to the ///property of the button in this column. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_DataTextFormatString) ] public virtual string DataTextFormatString { get { object o = ViewState["DataTextFormatString"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the string used to format the data bound to /// the ///property of the button. /// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_Text) ] public virtual string Text { get { object o = ViewState["Text"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["Text"] = value; OnColumnChanged(); } } [ DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_ValidationGroup) ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } ///Gets or sets the caption text displayed on the ////// in this column. /// protected virtual string FormatDataTextValue(object dataTextValue) { string formattedTextValue = String.Empty; if (!DataBinder.IsNull(dataTextValue)) { string formatting = DataTextFormatString; if (formatting.Length == 0) { formattedTextValue = dataTextValue.ToString(); } else { formattedTextValue = String.Format(CultureInfo.CurrentCulture, formatting, dataTextValue); } } return formattedTextValue; } ////// public override void Initialize() { base.Initialize(); textFieldDesc = null; } ////// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { WebControl buttonControl = null; if (ButtonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); button.Text = Text; button.CommandName = CommandName; button.CausesValidation = CausesValidation; button.ValidationGroup = ValidationGroup; buttonControl = button; } else { Button button = new Button(); button.Text = Text; button.CommandName = CommandName; button.CausesValidation = CausesValidation; button.ValidationGroup = ValidationGroup; buttonControl = button; } if (DataTextField.Length != 0) { buttonControl.DataBinding += new EventHandler(this.OnDataBindColumn); } cell.Controls.Add(buttonControl); } } ///Initializes a cell in the ///. /// private void OnDataBindColumn(object sender, EventArgs e) { Debug.Assert(DataTextField.Length != 0, "Shouldn't be DataBinding without a DataTextField"); Control boundControl = (Control)sender; DataGridItem item = (DataGridItem)boundControl.NamingContainer; object dataItem = item.DataItem; if (textFieldDesc == null) { string dataField = DataTextField; textFieldDesc = TypeDescriptor.GetProperties(dataItem).Find(dataField, true); if ((textFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, dataField)); } } string dataValue; if (textFieldDesc != null) { object data = textFieldDesc.GetValue(dataItem); dataValue = FormatDataTextValue(data); } else { Debug.Assert(DesignMode == true); dataValue = SR.GetString(SR.Sample_Databound_Text); } if (boundControl is LinkButton) { ((LinkButton)boundControl).Text = dataValue; } else { Debug.Assert(boundControl is Button, "Expected the bound control to be a Button"); ((Button)boundControl).Text = dataValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ButtonColumn : DataGridColumn { private PropertyDescriptor textFieldDesc; ///Creates a column with a set of ////// controls. /// public ButtonColumn() { } ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), DefaultValue(ButtonColumnType.LinkButton), WebSysDescriptionAttribute(SR.ButtonColumn_ButtonType) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } [ DefaultValue(false), WebSysDescriptionAttribute(SR.ButtonColumn_CausesValidation) ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return false; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Gets or sets the type of button to render in the /// column. ////// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescriptionAttribute(SR.WebControl_CommandName) ] public virtual string CommandName { get { object o = ViewState["CommandName"]; if (o != null) return(string)o; return string.Empty; } set { ViewState["CommandName"] = value; OnColumnChanged(); } } ///Gets or sets the command to perform when this ////// is clicked. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_DataTextField) ] public virtual string DataTextField { get { object o = ViewState["DataTextField"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextField"] = value; OnColumnChanged(); } } ///Gets or sets the field name from the data model that is /// bound to the ///property of the button in this column. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_DataTextFormatString) ] public virtual string DataTextFormatString { get { object o = ViewState["DataTextFormatString"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the string used to format the data bound to /// the ///property of the button. /// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_Text) ] public virtual string Text { get { object o = ViewState["Text"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["Text"] = value; OnColumnChanged(); } } [ DefaultValue(""), WebSysDescriptionAttribute(SR.ButtonColumn_ValidationGroup) ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } ///Gets or sets the caption text displayed on the ////// in this column. /// protected virtual string FormatDataTextValue(object dataTextValue) { string formattedTextValue = String.Empty; if (!DataBinder.IsNull(dataTextValue)) { string formatting = DataTextFormatString; if (formatting.Length == 0) { formattedTextValue = dataTextValue.ToString(); } else { formattedTextValue = String.Format(CultureInfo.CurrentCulture, formatting, dataTextValue); } } return formattedTextValue; } ////// public override void Initialize() { base.Initialize(); textFieldDesc = null; } ////// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { WebControl buttonControl = null; if (ButtonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); button.Text = Text; button.CommandName = CommandName; button.CausesValidation = CausesValidation; button.ValidationGroup = ValidationGroup; buttonControl = button; } else { Button button = new Button(); button.Text = Text; button.CommandName = CommandName; button.CausesValidation = CausesValidation; button.ValidationGroup = ValidationGroup; buttonControl = button; } if (DataTextField.Length != 0) { buttonControl.DataBinding += new EventHandler(this.OnDataBindColumn); } cell.Controls.Add(buttonControl); } } ///Initializes a cell in the ///. /// private void OnDataBindColumn(object sender, EventArgs e) { Debug.Assert(DataTextField.Length != 0, "Shouldn't be DataBinding without a DataTextField"); Control boundControl = (Control)sender; DataGridItem item = (DataGridItem)boundControl.NamingContainer; object dataItem = item.DataItem; if (textFieldDesc == null) { string dataField = DataTextField; textFieldDesc = TypeDescriptor.GetProperties(dataItem).Find(dataField, true); if ((textFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, dataField)); } } string dataValue; if (textFieldDesc != null) { object data = textFieldDesc.GetValue(dataItem); dataValue = FormatDataTextValue(data); } else { Debug.Assert(DesignMode == true); dataValue = SR.GetString(SR.Sample_Databound_Text); } if (boundControl is LinkButton) { ((LinkButton)boundControl).Text = dataValue; } else { Debug.Assert(boundControl is Button, "Expected the bound control to be a Button"); ((Button)boundControl).Text = dataValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
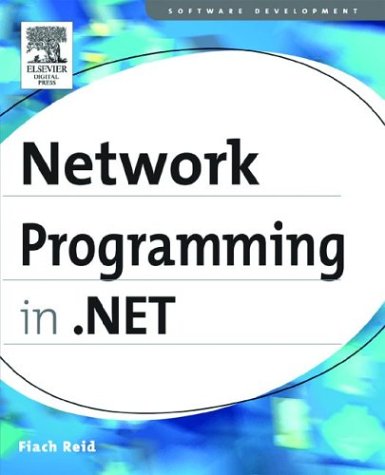
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OracleDateTime.cs
- StringResourceManager.cs
- CodeCatchClauseCollection.cs
- ListMarkerLine.cs
- SqlDuplicator.cs
- MenuItemCollection.cs
- ExceptionHandlerDesigner.cs
- WorkflowDefinitionContext.cs
- EntityProviderServices.cs
- Parser.cs
- DependentTransaction.cs
- Block.cs
- ListViewTableCell.cs
- Transform3D.cs
- DictionaryEntry.cs
- MiniAssembly.cs
- StatusInfoItem.cs
- TextChange.cs
- ProjectionPruner.cs
- StyleTypedPropertyAttribute.cs
- SecurityManager.cs
- LocalFileSettingsProvider.cs
- BaseCollection.cs
- FontCollection.cs
- WebReferencesBuildProvider.cs
- LogSwitch.cs
- DatatypeImplementation.cs
- HostExecutionContextManager.cs
- PageThemeParser.cs
- TimeEnumHelper.cs
- ConcatQueryOperator.cs
- Rectangle.cs
- OrderedEnumerableRowCollection.cs
- CqlParserHelpers.cs
- Transform3DCollection.cs
- MembershipValidatePasswordEventArgs.cs
- SecureEnvironment.cs
- TextServicesPropertyRanges.cs
- TTSEngineProxy.cs
- Set.cs
- PKCS1MaskGenerationMethod.cs
- SafeCryptContextHandle.cs
- ToolStripDropDownMenu.cs
- BasicCellRelation.cs
- DrawListViewItemEventArgs.cs
- CodeStatementCollection.cs
- XmlSchemaObjectCollection.cs
- SourceExpressionException.cs
- ListBase.cs
- SemanticTag.cs
- ProviderConnectionPoint.cs
- TraceUtility.cs
- NameService.cs
- JsonReader.cs
- ConfigXmlComment.cs
- Size.cs
- SelectionEditor.cs
- ArgumentOutOfRangeException.cs
- DetailsViewRow.cs
- DbConnectionOptions.cs
- URL.cs
- BooleanAnimationBase.cs
- Selector.cs
- TableLayoutCellPaintEventArgs.cs
- PrintDialog.cs
- ShaderEffect.cs
- SchemaElementDecl.cs
- typedescriptorpermissionattribute.cs
- SoapEnumAttribute.cs
- UnicodeEncoding.cs
- CodeIdentifiers.cs
- WebPartTransformerAttribute.cs
- ProtocolsConfiguration.cs
- IntegerCollectionEditor.cs
- BitmapEffectState.cs
- TypeContext.cs
- ToolboxItemCollection.cs
- DirectoryNotFoundException.cs
- ProfileBuildProvider.cs
- COM2ICategorizePropertiesHandler.cs
- AspNetCacheProfileAttribute.cs
- TableLayoutStyle.cs
- UnionCqlBlock.cs
- InlinedAggregationOperatorEnumerator.cs
- Wow64ConfigurationLoader.cs
- _Events.cs
- DockProviderWrapper.cs
- PropertyGridEditorPart.cs
- PathSegment.cs
- TransactionFlowProperty.cs
- SystemResourceKey.cs
- NameValuePair.cs
- TreeWalker.cs
- GridViewRowPresenterBase.cs
- SecurityChannel.cs
- LogAppendAsyncResult.cs
- FilterElement.cs
- ProfessionalColorTable.cs
- TableAdapterManagerGenerator.cs
- ReadWriteSpinLock.cs