Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / internal / materialization / recordstatefactory.cs / 1 / recordstatefactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Linq; using System.Linq.Expressions; namespace System.Data.Common.Internal.Materialization { ////// An immutable class used to generate new RecordStates, which are used /// at runtime to produce value-layer (aka DataReader) results. /// /// Contains static information collected by the Translator visitor. The /// expressions produced by the Translator are compiled. The RecordStates /// will refer to this object for all static information. /// /// This class is cached in the query cache as part of the CoordinatorFactory. /// internal class RecordStateFactory { #region state ////// Indicates which state slot in the Shaper.State is expected to hold the /// value for this record state. Each unique record shape has it's own state /// slot. /// internal readonly int StateSlotNumber; ////// How many column values we have to reserve space for in this record. /// internal readonly int ColumnCount; ////// The DataRecordInfo we must return for this record. If the record represents /// an entity, this will be used to construct a unique EntityRecordInfo with the /// EntityKey and EntitySet for the entity. /// internal readonly DataRecordInfo DataRecordInfo; ////// A function that will gather the data for the row and store it on the record state. /// internal readonly FuncGatherData; /// /// Collection of nested records for this record, such as a complex type that is /// part of an entity. This does not include records that are part of a nested /// collection, however. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionNestedRecordStateFactories; /// /// The name for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionColumnNames; /// /// The type usage information for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionTypeUsages; /// /// Tracks which columns might need special handling (nested readers/records) /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionIsColumnNested; /// /// Tracks whether there are ANY columns that need special handling. /// internal readonly bool HasNestedColumns; ////// A helper class to make the translation from name->ordinal. /// internal readonly FieldNameLookup FieldNameLookup; ////// Description of this RecordStateFactory, used for debugging only; while this /// is not needed in retail code, it is pretty important because it's the only /// description we'll have once we compile the Expressions; debugging a problem /// with retail bits would be pretty hard without this. /// private readonly string Description; #endregion #region constructor public RecordStateFactory(int stateSlotNumber, int columnCount, RecordStateFactory[] nestedRecordStateFactories, DataRecordInfo dataRecordInfo, Expression gatherData, string[] propertyNames, TypeUsage[] typeUsages) { this.StateSlotNumber = stateSlotNumber; this.ColumnCount = columnCount; this.NestedRecordStateFactories = new System.Collections.ObjectModel.ReadOnlyCollection(nestedRecordStateFactories); this.DataRecordInfo = dataRecordInfo; this.GatherData = Translator.Compile (gatherData); this.Description = gatherData.ToString(); this.ColumnNames = new System.Collections.ObjectModel.ReadOnlyCollection (propertyNames); this.TypeUsages = new System.Collections.ObjectModel.ReadOnlyCollection (typeUsages); this.FieldNameLookup = new FieldNameLookup(this.ColumnNames, -1); // pre-compute the nested objects from typeUsage, for performance bool[] isColumnNested = new bool[columnCount]; for (int ordinal = 0; ordinal < columnCount; ordinal++) { switch (typeUsages[ordinal].EdmType.BuiltInTypeKind) { case BuiltInTypeKind.EntityType: case BuiltInTypeKind.ComplexType: case BuiltInTypeKind.RowType: case BuiltInTypeKind.CollectionType: isColumnNested[ordinal] = true; this.HasNestedColumns = true; break; default: isColumnNested[ordinal] = false; break; } } this.IsColumnNested = new System.Collections.ObjectModel.ReadOnlyCollection (isColumnNested); } #endregion #region "public" surface area /// /// It's GO time, create the record state. /// internal RecordState Create(CoordinatorFactory coordinatorFactory) { return new RecordState(this, coordinatorFactory); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Linq; using System.Linq.Expressions; namespace System.Data.Common.Internal.Materialization { ////// An immutable class used to generate new RecordStates, which are used /// at runtime to produce value-layer (aka DataReader) results. /// /// Contains static information collected by the Translator visitor. The /// expressions produced by the Translator are compiled. The RecordStates /// will refer to this object for all static information. /// /// This class is cached in the query cache as part of the CoordinatorFactory. /// internal class RecordStateFactory { #region state ////// Indicates which state slot in the Shaper.State is expected to hold the /// value for this record state. Each unique record shape has it's own state /// slot. /// internal readonly int StateSlotNumber; ////// How many column values we have to reserve space for in this record. /// internal readonly int ColumnCount; ////// The DataRecordInfo we must return for this record. If the record represents /// an entity, this will be used to construct a unique EntityRecordInfo with the /// EntityKey and EntitySet for the entity. /// internal readonly DataRecordInfo DataRecordInfo; ////// A function that will gather the data for the row and store it on the record state. /// internal readonly FuncGatherData; /// /// Collection of nested records for this record, such as a complex type that is /// part of an entity. This does not include records that are part of a nested /// collection, however. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionNestedRecordStateFactories; /// /// The name for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionColumnNames; /// /// The type usage information for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionTypeUsages; /// /// Tracks which columns might need special handling (nested readers/records) /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionIsColumnNested; /// /// Tracks whether there are ANY columns that need special handling. /// internal readonly bool HasNestedColumns; ////// A helper class to make the translation from name->ordinal. /// internal readonly FieldNameLookup FieldNameLookup; ////// Description of this RecordStateFactory, used for debugging only; while this /// is not needed in retail code, it is pretty important because it's the only /// description we'll have once we compile the Expressions; debugging a problem /// with retail bits would be pretty hard without this. /// private readonly string Description; #endregion #region constructor public RecordStateFactory(int stateSlotNumber, int columnCount, RecordStateFactory[] nestedRecordStateFactories, DataRecordInfo dataRecordInfo, Expression gatherData, string[] propertyNames, TypeUsage[] typeUsages) { this.StateSlotNumber = stateSlotNumber; this.ColumnCount = columnCount; this.NestedRecordStateFactories = new System.Collections.ObjectModel.ReadOnlyCollection(nestedRecordStateFactories); this.DataRecordInfo = dataRecordInfo; this.GatherData = Translator.Compile (gatherData); this.Description = gatherData.ToString(); this.ColumnNames = new System.Collections.ObjectModel.ReadOnlyCollection (propertyNames); this.TypeUsages = new System.Collections.ObjectModel.ReadOnlyCollection (typeUsages); this.FieldNameLookup = new FieldNameLookup(this.ColumnNames, -1); // pre-compute the nested objects from typeUsage, for performance bool[] isColumnNested = new bool[columnCount]; for (int ordinal = 0; ordinal < columnCount; ordinal++) { switch (typeUsages[ordinal].EdmType.BuiltInTypeKind) { case BuiltInTypeKind.EntityType: case BuiltInTypeKind.ComplexType: case BuiltInTypeKind.RowType: case BuiltInTypeKind.CollectionType: isColumnNested[ordinal] = true; this.HasNestedColumns = true; break; default: isColumnNested[ordinal] = false; break; } } this.IsColumnNested = new System.Collections.ObjectModel.ReadOnlyCollection (isColumnNested); } #endregion #region "public" surface area /// /// It's GO time, create the record state. /// internal RecordState Create(CoordinatorFactory coordinatorFactory) { return new RecordState(this, coordinatorFactory); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
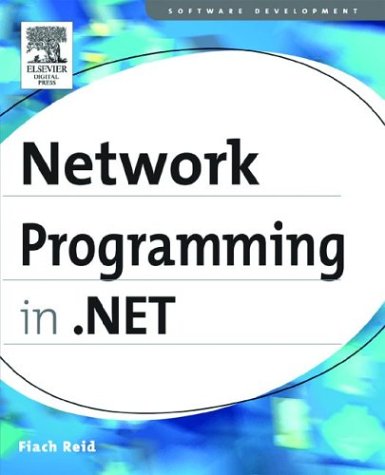
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextParagraphCache.cs
- RtType.cs
- ValidateNames.cs
- DataServiceResponse.cs
- ValidationPropertyAttribute.cs
- DataRecordInfo.cs
- DetailsViewModeEventArgs.cs
- BlobPersonalizationState.cs
- NumberFormatter.cs
- DataGridViewCellStyleBuilderDialog.cs
- DataGridViewCellCancelEventArgs.cs
- ByeMessageCD1.cs
- BuiltInPermissionSets.cs
- RequiredAttributeAttribute.cs
- SimpleBitVector32.cs
- ProfileSection.cs
- LocatorGroup.cs
- TemplateComponentConnector.cs
- PackagingUtilities.cs
- SeparatorAutomationPeer.cs
- XomlCompilerHelpers.cs
- TextEditorCharacters.cs
- UiaCoreApi.cs
- XmlUTF8TextReader.cs
- ResXResourceReader.cs
- RowBinding.cs
- AuthorizationSection.cs
- TableCellAutomationPeer.cs
- SocketPermission.cs
- SingleAnimationUsingKeyFrames.cs
- StringStorage.cs
- ScrollChrome.cs
- InternalDispatchObject.cs
- InvokeMemberBinder.cs
- ListViewCancelEventArgs.cs
- ComponentCollection.cs
- RelationshipConverter.cs
- WeakEventManager.cs
- XamlNamespaceHelper.cs
- DataPointer.cs
- BindingContext.cs
- TypeListConverter.cs
- VisualStyleTypesAndProperties.cs
- Clock.cs
- EdmSchemaAttribute.cs
- Hash.cs
- CharConverter.cs
- SqlMultiplexer.cs
- MessageBodyDescription.cs
- DetailsViewDesigner.cs
- KeyboardNavigation.cs
- WindowsScrollBarBits.cs
- JulianCalendar.cs
- PropertyPathConverter.cs
- AttributeTableBuilder.cs
- MasterPageBuildProvider.cs
- RuntimeIdentifierPropertyAttribute.cs
- BinaryWriter.cs
- COM2IProvidePropertyBuilderHandler.cs
- DoubleCollectionConverter.cs
- AutomationIdentifier.cs
- BamlResourceSerializer.cs
- ServiceModelConfiguration.cs
- ToolStripPanel.cs
- GridViewSortEventArgs.cs
- XmlException.cs
- ACE.cs
- GridErrorDlg.cs
- AutomationPeer.cs
- CodeAttachEventStatement.cs
- WorkflowOperationInvoker.cs
- _TimerThread.cs
- WindowsSpinner.cs
- PropertyInfoSet.cs
- ResXDataNode.cs
- ListViewSortEventArgs.cs
- NumberFormatInfo.cs
- Pair.cs
- SortedDictionary.cs
- XPathNodeIterator.cs
- DataBindingHandlerAttribute.cs
- StrokeRenderer.cs
- FontCacheUtil.cs
- ChangeConflicts.cs
- KeyFrames.cs
- ComponentManagerBroker.cs
- SiteMapPath.cs
- basevalidator.cs
- JsonReaderWriterFactory.cs
- GeneralTransformCollection.cs
- CqlQuery.cs
- RadioButtonPopupAdapter.cs
- MarkupCompiler.cs
- XmlTextEncoder.cs
- TheQuery.cs
- XmlSchemaChoice.cs
- FixedSOMSemanticBox.cs
- WebEncodingValidatorAttribute.cs
- RSAPKCS1KeyExchangeFormatter.cs
- StylusPlugin.cs