Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / ListControlDesigner.cs / 1 / ListControlDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls { using System; using System.Design; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.IO; using System.Data; using System.Web.UI; using System.Web.UI.Design; using System.Web.UI.Design.Util; using System.Web.UI.WebControls; using System.Windows.Forms.Design; using DialogResult = System.Windows.Forms.DialogResult; using AttributeCollection = System.ComponentModel.AttributeCollection; using DataBinding = System.Web.UI.DataBinding; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] [SupportsPreviewControl(true)] public class ListControlDesigner : DataBoundControlDesigner { ////// This is the base class for all ////// designers. /// /// /// public ListControlDesigner() { } ////// Initializes a new instance of ////// . /// /// /// Adds designer actions to the ActionLists collection. /// public override DesignerActionListCollection ActionLists { get { DesignerActionListCollection actionLists = new DesignerActionListCollection(); actionLists.AddRange(base.ActionLists); actionLists.Add(new ListControlActionList(this, DataSourceDesigner)); return actionLists; } } ////// /// public string DataValueField { get { return ((ListControl)Component).DataValueField; } set { ((ListControl)Component).DataValueField = value; } } ////// /// Retrieves the HTML to be used for the design time representation of the control runtime. /// public string DataTextField { get { return ((ListControl)Component).DataTextField; } set { ((ListControl)Component).DataTextField = value; } } ////// /// Used to determine whether the control should render its default action lists, /// containing a DataSourceID dropdown and related tasks. /// protected override bool UseDataSourcePickerActionList { get { return false; } } internal void ConnectToDataSourceAction() { InvokeTransactedChange(Component, new TransactedChangeCallback(ConnectToDataSourceCallback), null, SR.GetString(SR.ListControlDesigner_ConnectToDataSource)); } private bool ConnectToDataSourceCallback(object context) { ListControlConnectToDataSourceDialog dialog = new ListControlConnectToDataSourceDialog(this); DialogResult result = UIServiceHelper.ShowDialog(Component.Site, dialog); return (result == DialogResult.OK); } ////// /// DataBinds the given control to get design time rendering /// protected override void DataBind(BaseDataBoundControl dataBoundControl) { // We don't need to databind here because we just added items to the items collection return; } ////// Starts collection editor for List Items /// internal void EditItems() { PropertyDescriptor descriptor = TypeDescriptor.GetProperties(Component)["Items"]; Debug.Assert(descriptor != null, "Expected to find Items property on ListControl"); InvokeTransactedChange(Component, new TransactedChangeCallback(EditItemsCallback), descriptor, SR.GetString(SR.ListControlDesigner_EditItems), descriptor); } ////// Transacted callback to invoke the Edit Items dialog. /// private bool EditItemsCallback(object context) { IDesignerHost designerHost = (IDesignerHost)GetService(typeof(IDesignerHost)); Debug.Assert(designerHost != null); PropertyDescriptor descriptor = (PropertyDescriptor)context; ListItemsCollectionEditor editor = new ListItemsCollectionEditor(typeof(ListItemCollection)); editor.EditValue(new TypeDescriptorContext(designerHost, descriptor, Component), new WindowsFormsEditorServiceHelper(this), descriptor.GetValue(Component)); return true; } ////// /// public override string GetDesignTimeHtml() { try { ListControl listControl = (ListControl)ViewControl; ListItemCollection items = listControl.Items; bool isDataBound = IsDataBound(); if (items.Count == 0 || isDataBound) { if (isDataBound) { items.Clear(); items.Add(SR.GetString(SR.Sample_Databound_Text)); } else { items.Add(SR.GetString(SR.Sample_Unbound_Text)); } } return base.GetDesignTimeHtml(); } catch (Exception e) { return GetErrorDesignTimeHtml(e); } } ///Gets the HTML to be used for the design time representation of the control runtime. ////// public IEnumerable GetResolvedSelectedDataSource() { return ((IDataSourceProvider)this).GetResolvedSelectedDataSource(); } ////// public object GetSelectedDataSource() { return ((IDataSourceProvider)this).GetSelectedDataSource(); } ////// /// public override void Initialize(IComponent component) { VerifyInitializeArgument(component, typeof(ListControl)); base.Initialize(component); } ////// Initializes the component for design. /// ////// /// Return true if the control is databound. /// private bool IsDataBound() { DataBinding dataSourceBinding = DataBindings["DataSource"]; return (dataSourceBinding != null || DataSourceID.Length > 0); } ////// /// This method is called when the data source the control is /// connected to changes. Override this method to perform any /// additional actions that your designer requires. Make sure to call /// the base implementation as well. /// This method exists because it shipped in V1. Removing it creates a breaking change. /// Then call the base class' implementation. /// public virtual void OnDataSourceChanged() { // Call the base protected implementation to inherit the default behavior // of BaseDataBoundControlDesigner. base.OnDataSourceChanged(true); } ////// Called by data bound control designers to raise the data source /// changed event so that listeners can be notified. /// protected override void OnDataSourceChanged(bool forceUpdateView) { // Call the public OnDataSourceChanged method without parameters introduced in this class, // which exists for back-compat. This method is new in V2. // Derived classes overrode OnDataSourceChanged(), so make sure that gets called. this.OnDataSourceChanged(); } ////// /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop; Attribute[] fieldPropAttrs = new Attribute[] { new TypeConverterAttribute(typeof(DataFieldConverter)) }; prop = (PropertyDescriptor)properties["DataTextField"]; Debug.Assert(prop != null); prop = TypeDescriptor.CreateProperty(this.GetType(), prop, fieldPropAttrs); properties["DataTextField"] = prop; prop = (PropertyDescriptor)properties["DataValueField"]; Debug.Assert(prop != null); prop = TypeDescriptor.CreateProperty(this.GetType(), prop, fieldPropAttrs); properties["DataValueField"] = prop; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Filters the properties to replace the runtime DataSource property /// descriptor with the designer's. /// ///
Link Menu
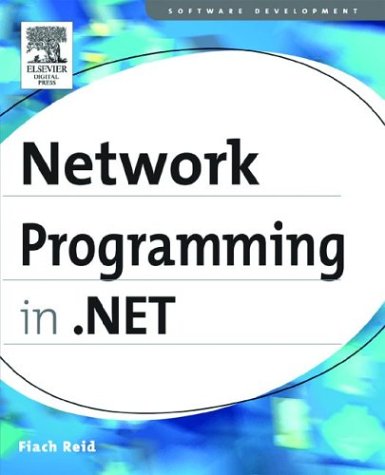
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IOException.cs
- MILUtilities.cs
- CompositeFontFamily.cs
- TransformedBitmap.cs
- WebServiceData.cs
- Quad.cs
- LinkedList.cs
- Matrix3DStack.cs
- AtomServiceDocumentSerializer.cs
- CodeVariableDeclarationStatement.cs
- ViewGenResults.cs
- DataTableTypeConverter.cs
- AssemblyBuilder.cs
- sqlpipe.cs
- Themes.cs
- ControlUtil.cs
- RectangleHotSpot.cs
- WebPartDisplayMode.cs
- AppDomain.cs
- TransformProviderWrapper.cs
- ToolboxCategoryItems.cs
- PolicyLevel.cs
- DataGridViewLinkCell.cs
- AnchoredBlock.cs
- MetadataUtil.cs
- MemberHolder.cs
- ItemPager.cs
- PointAnimationClockResource.cs
- GenericIdentity.cs
- RoleService.cs
- HttpEncoder.cs
- NodeFunctions.cs
- ProjectedSlot.cs
- TextSelectionProcessor.cs
- RadioButtonAutomationPeer.cs
- SchemaLookupTable.cs
- InputBuffer.cs
- ListItemsCollectionEditor.cs
- GroupBox.cs
- IFlowDocumentViewer.cs
- DataControlFieldHeaderCell.cs
- TableRowGroup.cs
- WrappedIUnknown.cs
- RadioButtonFlatAdapter.cs
- StringValueSerializer.cs
- ButtonColumn.cs
- SimpleWebHandlerParser.cs
- BufferModeSettings.cs
- TokenBasedSet.cs
- MethodRental.cs
- BrushConverter.cs
- Hex.cs
- ProxyHwnd.cs
- DbDataAdapter.cs
- UriTemplateClientFormatter.cs
- Activator.cs
- CryptoConfig.cs
- GenericIdentity.cs
- TdsParserHelperClasses.cs
- WindowsStatusBar.cs
- QuaternionConverter.cs
- odbcmetadatafactory.cs
- AttachedPropertyDescriptor.cs
- DataTemplateKey.cs
- PropertyHelper.cs
- DBSchemaTable.cs
- FacetValues.cs
- TextContainerHelper.cs
- CharacterHit.cs
- Splitter.cs
- TracedNativeMethods.cs
- Attribute.cs
- WebSysDescriptionAttribute.cs
- WebServiceHandler.cs
- DecimalKeyFrameCollection.cs
- DictionaryEntry.cs
- ScrollProperties.cs
- BasicExpressionVisitor.cs
- SpnegoTokenAuthenticator.cs
- SetStateEventArgs.cs
- XamlToRtfParser.cs
- ResourceManager.cs
- Compensation.cs
- EndGetFileNameFromUserRequest.cs
- SplitterDesigner.cs
- IconConverter.cs
- NativeMethods.cs
- PropertySegmentSerializer.cs
- CodePageUtils.cs
- elementinformation.cs
- PrePrepareMethodAttribute.cs
- Int16Converter.cs
- InfoCardSchemas.cs
- HttpProfileGroupBase.cs
- SourceItem.cs
- DataGridHelper.cs
- XmlSubtreeReader.cs
- InternalTypeHelper.cs
- QilReference.cs
- WebDisplayNameAttribute.cs