Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / PointAnimationClockResource.cs / 1305600 / PointAnimationClockResource.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; namespace System.Windows.Media.Animation { ////// PointAnimationClockResource class. /// AnimationClockResource classes refer to an AnimationClock and a base /// value. They implement DUCE.IResource, and thus can be used to produce /// a render-side resource which represents the current value of this /// AnimationClock. /// They subscribe to the Changed event on the AnimationClock and ensure /// that the resource's current value is up to date. /// internal class PointAnimationClockResource: AnimationClockResource, DUCE.IResource { ////// Constructor for public PointAnimationClockResource. /// This constructor accepts the base value and AnimationClock. /// Note that since there is no current requirement that we be able to set or replace either the /// base value or the AnimationClock, this is the only way to initialize an instance of /// PointAnimationClockResource. /// Also, we currently Assert that the resource is non-null, since without mutability /// such a resource isn't needed. /// We can easily extend this class if/when new requirements arise. /// /// Point - The base value. /// AnimationClock - cannot be null. public PointAnimationClockResource( Point baseValue, AnimationClock animationClock ): base( animationClock ) { _baseValue = baseValue; } #region Public Properties ////// BaseValue Property - typed accessor for BaseValue. /// public Point BaseValue { get { return _baseValue; } } ////// CurrentValue Property - typed accessor for CurrentValue /// public Point CurrentValue { get { if (_animationClock != null) { // No handoff for DrawingContext animations so we use the // BaseValue as the defaultOriginValue and the // defaultDestinationValue. We call the Timeline's GetCurrentValue // directly to avoid boxing return ((PointAnimationBase)(_animationClock.Timeline)).GetCurrentValue( _baseValue, // defaultOriginValue _baseValue, // defaultDesinationValue _animationClock); // clock } else { return _baseValue; } } } #endregion Public Properties #region DUCE // // Method which returns the DUCE type of this class. // The base class needs this type when calling CreateOrAddRefOnChannel. // By providing this via a virtual, we avoid a per-instance storage cost. // protected override DUCE.ResourceType ResourceType { get { return DUCE.ResourceType.TYPE_POINTRESOURCE; } } ////// UpdateResource - This method is called to update the render-thread /// resource on a given channel. /// /// The DUCE.ResourceHandle for this resource on this channel. /// The channel on which to update the render-thread resource. ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels can handle bad pointers and will not affect other appdomains or processes /// [SecurityCritical,SecurityTreatAsSafe] protected override void UpdateResource( DUCE.ResourceHandle handle, DUCE.Channel channel) { DUCE.MILCMD_POINTRESOURCE cmd = new DUCE.MILCMD_POINTRESOURCE(); cmd.Type = MILCMD.MilCmdPointResource; cmd.Handle = handle; cmd.Value = CurrentValue; unsafe { channel.SendCommand( (byte*)&cmd, sizeof(DUCE.MILCMD_POINTRESOURCE)); } // Validate this resource IsResourceInvalid = false; } #endregion DUCE private Point _baseValue; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; namespace System.Windows.Media.Animation { ////// PointAnimationClockResource class. /// AnimationClockResource classes refer to an AnimationClock and a base /// value. They implement DUCE.IResource, and thus can be used to produce /// a render-side resource which represents the current value of this /// AnimationClock. /// They subscribe to the Changed event on the AnimationClock and ensure /// that the resource's current value is up to date. /// internal class PointAnimationClockResource: AnimationClockResource, DUCE.IResource { ////// Constructor for public PointAnimationClockResource. /// This constructor accepts the base value and AnimationClock. /// Note that since there is no current requirement that we be able to set or replace either the /// base value or the AnimationClock, this is the only way to initialize an instance of /// PointAnimationClockResource. /// Also, we currently Assert that the resource is non-null, since without mutability /// such a resource isn't needed. /// We can easily extend this class if/when new requirements arise. /// /// Point - The base value. /// AnimationClock - cannot be null. public PointAnimationClockResource( Point baseValue, AnimationClock animationClock ): base( animationClock ) { _baseValue = baseValue; } #region Public Properties ////// BaseValue Property - typed accessor for BaseValue. /// public Point BaseValue { get { return _baseValue; } } ////// CurrentValue Property - typed accessor for CurrentValue /// public Point CurrentValue { get { if (_animationClock != null) { // No handoff for DrawingContext animations so we use the // BaseValue as the defaultOriginValue and the // defaultDestinationValue. We call the Timeline's GetCurrentValue // directly to avoid boxing return ((PointAnimationBase)(_animationClock.Timeline)).GetCurrentValue( _baseValue, // defaultOriginValue _baseValue, // defaultDesinationValue _animationClock); // clock } else { return _baseValue; } } } #endregion Public Properties #region DUCE // // Method which returns the DUCE type of this class. // The base class needs this type when calling CreateOrAddRefOnChannel. // By providing this via a virtual, we avoid a per-instance storage cost. // protected override DUCE.ResourceType ResourceType { get { return DUCE.ResourceType.TYPE_POINTRESOURCE; } } ////// UpdateResource - This method is called to update the render-thread /// resource on a given channel. /// /// The DUCE.ResourceHandle for this resource on this channel. /// The channel on which to update the render-thread resource. ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels can handle bad pointers and will not affect other appdomains or processes /// [SecurityCritical,SecurityTreatAsSafe] protected override void UpdateResource( DUCE.ResourceHandle handle, DUCE.Channel channel) { DUCE.MILCMD_POINTRESOURCE cmd = new DUCE.MILCMD_POINTRESOURCE(); cmd.Type = MILCMD.MilCmdPointResource; cmd.Handle = handle; cmd.Value = CurrentValue; unsafe { channel.SendCommand( (byte*)&cmd, sizeof(DUCE.MILCMD_POINTRESOURCE)); } // Validate this resource IsResourceInvalid = false; } #endregion DUCE private Point _baseValue; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
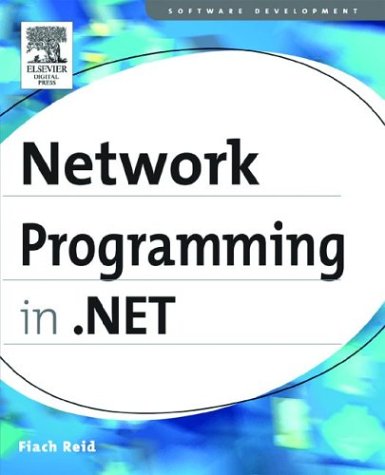
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeConverter.cs
- PositiveTimeSpanValidatorAttribute.cs
- PermissionSetEnumerator.cs
- ClientTargetSection.cs
- BufferAllocator.cs
- SerializationSectionGroup.cs
- SqlNotificationEventArgs.cs
- ScriptingSectionGroup.cs
- SafeFileMapViewHandle.cs
- NamespaceList.cs
- ObjectViewFactory.cs
- QueryRewriter.cs
- TextTreeText.cs
- TargetFrameworkAttribute.cs
- LoadedOrUnloadedOperation.cs
- WorkItem.cs
- Resources.Designer.cs
- UnauthorizedAccessException.cs
- MDIWindowDialog.cs
- _BaseOverlappedAsyncResult.cs
- SoapSchemaMember.cs
- StaticResourceExtension.cs
- SqlTopReducer.cs
- CombinedTcpChannel.cs
- XmlSchemaImport.cs
- WebPartZoneCollection.cs
- DefaultProxySection.cs
- TypeConverterHelper.cs
- ArgumentOutOfRangeException.cs
- SwitchLevelAttribute.cs
- ConnectionManagementElementCollection.cs
- TextElement.cs
- CodeIdentifier.cs
- StickyNoteAnnotations.cs
- SubqueryRules.cs
- DetectEofStream.cs
- XmlILAnnotation.cs
- ArrangedElement.cs
- VideoDrawing.cs
- SHA1Managed.cs
- LocatorManager.cs
- Main.cs
- EditCommandColumn.cs
- Enum.cs
- HtmlValidationSummaryAdapter.cs
- CryptoConfig.cs
- URI.cs
- SizeF.cs
- NoClickablePointException.cs
- CodeSubDirectory.cs
- NavigationExpr.cs
- EntityStoreSchemaFilterEntry.cs
- ToolStripDropTargetManager.cs
- AnnotationService.cs
- ResizeGrip.cs
- CodeTypeDeclarationCollection.cs
- LockCookie.cs
- httpserverutility.cs
- DelimitedListTraceListener.cs
- SwitchLevelAttribute.cs
- MultipleFilterMatchesException.cs
- XPathDocumentIterator.cs
- AsymmetricAlgorithm.cs
- SynchronizingStream.cs
- PenLineCapValidation.cs
- GroupQuery.cs
- OdbcCommandBuilder.cs
- QilIterator.cs
- ScrollableControl.cs
- ContentPlaceHolder.cs
- PreservationFileReader.cs
- XmlSchemaGroup.cs
- EpmSourceTree.cs
- MsdtcClusterUtils.cs
- BitmapDownload.cs
- BamlLocalizer.cs
- AccessDataSource.cs
- HostedNamedPipeTransportManager.cs
- ClientUrlResolverWrapper.cs
- ExtendLockAsyncResult.cs
- TitleStyle.cs
- SafeTokenHandle.cs
- SpanIndex.cs
- DependencyPropertyChangedEventArgs.cs
- ResourceExpressionBuilder.cs
- SqlDataSourceQuery.cs
- XamlWrappingReader.cs
- RemotingSurrogateSelector.cs
- StylusButton.cs
- CodeGroup.cs
- CodeSnippetExpression.cs
- PingOptions.cs
- WeakEventManager.cs
- TdsParameterSetter.cs
- XmlStreamStore.cs
- NamespaceInfo.cs
- DocumentPageTextView.cs
- X509UI.cs
- XhtmlBasicValidationSummaryAdapter.cs
- SafeNativeMethods.cs