Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / SQLTypes / SqlCharStream.cs / 1 / SqlCharStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlStreamChars.cs // // Create by: JunFang // // Description: // // Notes: // // History: // // 04/17/01 JunFang Created. // // @EndHeader@ //************************************************************************* namespace System.Data.SqlTypes { using System; using System.IO; using System.Runtime.InteropServices; using System.Data.SqlTypes; #if WINFSInternalOnly public #else internal #endif abstract class SqlStreamChars: System.Data.SqlTypes.INullable, IDisposable { public abstract bool IsNull { get; } public abstract bool CanRead { get; } public abstract bool CanSeek { get; } public abstract bool CanWrite { get; } public abstract long Length { get; } public abstract long Position { get; set; } // -------------------------------------------------------------- // Public methods // ------------------------------------------------------------- public abstract int Read (char[] buffer, int offset, int count); public abstract void Write (char[] buffer, int offset, int count); public abstract long Seek (long offset, SeekOrigin origin); public abstract void SetLength (long value); public abstract void Flush (); public virtual void Close(){ Dispose(true); } void IDisposable.Dispose() { Dispose(true); } protected virtual void Dispose(bool disposing) { } public virtual int ReadChar() { // Reads one char from the stream by calling Read(char[], int, int). // Will return an char cast to an int or -1 on end of stream. // The performance of the default implementation on Stream is bad, // and any subclass with an internal buffer should override this method. char[] oneCharArray = new char[1]; int r = Read(oneCharArray, 0, 1); if (r==0) return -1; return oneCharArray[0]; } public virtual void WriteChar(char value) { // Writes one char from the stream by calling Write(char[], int, int). // The performance of the default implementation on Stream is bad, // and any subclass with an internal buffer should override this method. char[] oneCharArray = new char[1]; oneCharArray[0] = value; Write(oneCharArray, 0, 1); } // Private class: the Null SqlStreamChars private class NullSqlStreamChars : SqlStreamChars { // -------------------------------------------------------------- // Constructor(s) // -------------------------------------------------------------- internal NullSqlStreamChars() { } // ------------------------------------------------------------- // Public properties // -------------------------------------------------------------- public override bool IsNull { get { return true; } } public override bool CanRead { get { return false; } } public override bool CanSeek { get { return false; } } public override bool CanWrite { get { return false; } } public override long Length { get { throw new SqlNullValueException(); } } public override long Position { get { throw new SqlNullValueException(); } set { throw new SqlNullValueException(); } } // ------------------------------------------------------------- // Public methods // ------------------------------------------------------------- public override int Read (char[] buffer, int offset, int count) { throw new SqlNullValueException(); } public override void Write (char[] buffer, int offset, int count) { throw new SqlNullValueException(); } public override long Seek (long offset, SeekOrigin origin) { throw new SqlNullValueException(); } public override void SetLength (long value) { throw new SqlNullValueException(); } public override void Flush () { throw new SqlNullValueException(); } public override void Close () { } } // class NullSqlStreamChars // The Null instance public static SqlStreamChars Null { get { return new NullSqlStreamChars(); } } } // class SqlStreamChars } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
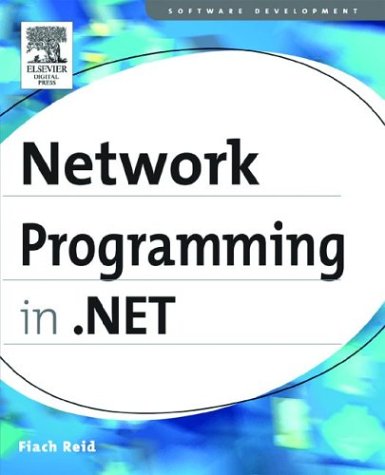
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SharedStatics.cs
- SchemaElementLookUpTable.cs
- XsdBuildProvider.cs
- RegionData.cs
- SourceChangedEventArgs.cs
- MatrixTransform.cs
- CacheEntry.cs
- ClientSession.cs
- BufferedReceiveElement.cs
- IntegrationExceptionEventArgs.cs
- ZipIOExtraFieldPaddingElement.cs
- HyperLinkStyle.cs
- CodeDirectiveCollection.cs
- RoleGroup.cs
- FillErrorEventArgs.cs
- HtmlTextArea.cs
- Model3D.cs
- MarkupExtensionParser.cs
- SystemWebSectionGroup.cs
- Margins.cs
- InternalConfigHost.cs
- PackageRelationshipCollection.cs
- CodeTypeMemberCollection.cs
- SqlSelectStatement.cs
- SqlConnectionHelper.cs
- SchemaElement.cs
- DataGridRow.cs
- HostingPreferredMapPath.cs
- Image.cs
- MenuItemAutomationPeer.cs
- XmlAnyAttributeAttribute.cs
- CodeTypeMemberCollection.cs
- InputManager.cs
- ContentPlaceHolderDesigner.cs
- RadioButton.cs
- XhtmlBasicPanelAdapter.cs
- Camera.cs
- WebHeaderCollection.cs
- RepeaterItemCollection.cs
- ImageSource.cs
- SchemaAttDef.cs
- XmlAutoDetectWriter.cs
- ProvidersHelper.cs
- RegexCharClass.cs
- SqlMetaData.cs
- TemplateBindingExtensionConverter.cs
- HeaderedContentControl.cs
- AssemblyFilter.cs
- SR.cs
- DefaultTraceListener.cs
- LineProperties.cs
- DataRowCollection.cs
- Literal.cs
- Dynamic.cs
- QueryPageSettingsEventArgs.cs
- InputScopeNameConverter.cs
- NonParentingControl.cs
- MetadataPropertyCollection.cs
- TypeToken.cs
- ControlBindingsCollection.cs
- Assign.cs
- SaveFileDialog.cs
- ProcessExitedException.cs
- PresentationSource.cs
- DataGridViewButtonCell.cs
- SourceLineInfo.cs
- COM2IDispatchConverter.cs
- _CacheStreams.cs
- OleDbMetaDataFactory.cs
- AspCompat.cs
- SelectingProviderEventArgs.cs
- ListBox.cs
- EntityDataSourceContextCreatingEventArgs.cs
- DataTrigger.cs
- ObjectMemberMapping.cs
- SqlUserDefinedTypeAttribute.cs
- FullTextState.cs
- Query.cs
- PingOptions.cs
- ObjectAssociationEndMapping.cs
- DriveInfo.cs
- Label.cs
- IsolatedStorageException.cs
- ContractType.cs
- ComboBoxAutomationPeer.cs
- ToolStripDropDownButton.cs
- ConfigurationValidatorBase.cs
- Base64Encoding.cs
- ObjectDataSourceView.cs
- TypeDependencyAttribute.cs
- ArraySegment.cs
- TableLayoutColumnStyleCollection.cs
- ImageSource.cs
- RegisteredDisposeScript.cs
- CodeArgumentReferenceExpression.cs
- GlyphInfoList.cs
- DataGridViewCellValueEventArgs.cs
- DrawingVisual.cs
- PagedDataSource.cs
- CodeDefaultValueExpression.cs