Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / __Filters.cs / 1 / __Filters.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // __Filters.cs // // This class defines the delegate methods for the COM+ implemented filters. // // // namespace System { using System; using System.Reflection; using System.Globalization; [Serializable()] internal class __Filters { // Filters... // The following are the built in filters defined for this class. These // should really be defined as static methods. They are used in as delegates // which currently doesn't support static methods. We will change this // once the compiler supports delegates. // FilterAttribute // This method will search for a member based upon the attribute passed in. // filterCriteria -- an Int32 representing the attribute internal virtual bool FilterAttribute(MemberInfo m,Object filterCriteria) { // Check that the criteria object is an Integer object if (filterCriteria == null) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); switch (m.MemberType) { case MemberTypes.Constructor: case MemberTypes.Method: { MethodAttributes criteria = 0; try { int i = (int) filterCriteria; criteria = (MethodAttributes) i; } catch { throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); } MethodAttributes attr; if (m.MemberType == MemberTypes.Method) attr = ((MethodInfo) m).Attributes; else attr = ((ConstructorInfo) m).Attributes; if (((criteria & MethodAttributes.MemberAccessMask) != 0) && (attr & MethodAttributes.MemberAccessMask) != (criteria & MethodAttributes.MemberAccessMask)) return false; if (((criteria & MethodAttributes.Static) != 0) && (attr & MethodAttributes.Static) == 0) return false; if (((criteria & MethodAttributes.Final) != 0) && (attr & MethodAttributes.Final) == 0) return false; if (((criteria & MethodAttributes.Virtual) != 0) && (attr & MethodAttributes.Virtual) == 0) return false; if (((criteria & MethodAttributes.Abstract) != 0) && (attr & MethodAttributes.Abstract) == 0) return false; if (((criteria & MethodAttributes.SpecialName) != 0) && (attr & MethodAttributes.SpecialName) == 0) return false; return true; } case MemberTypes.Field: { FieldAttributes criteria = 0; try { int i = (int) filterCriteria; criteria = (FieldAttributes) i; } catch { throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); } FieldAttributes attr = ((FieldInfo) m).Attributes; if (((criteria & FieldAttributes.FieldAccessMask) != 0) && (attr & FieldAttributes.FieldAccessMask) != (criteria & FieldAttributes.FieldAccessMask)) return false; if (((criteria & FieldAttributes.Static) != 0) && (attr & FieldAttributes.Static) == 0) return false; if (((criteria & FieldAttributes.InitOnly) != 0) && (attr & FieldAttributes.InitOnly) == 0) return false; if (((criteria & FieldAttributes.Literal) != 0) && (attr & FieldAttributes.Literal) == 0) return false; if (((criteria & FieldAttributes.NotSerialized) != 0) && (attr & FieldAttributes.NotSerialized) == 0) return false; if (((criteria & FieldAttributes.PinvokeImpl) != 0) && (attr & FieldAttributes.PinvokeImpl) == 0) return false; return true; } } return false; } // FilterName // This method will filter based upon the name. A partial wildcard // at the end of the string is supported. // filterCriteria -- This is the string name internal virtual bool FilterName(MemberInfo m,Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritString")); // At the moment this fails if its done on a single line.... String str = ((String) filterCriteria); str = str.Trim(); String name = m.Name; // Get the nested class name only, as opposed to the mangled one if (m.MemberType == MemberTypes.NestedType) name = name.Substring(name.LastIndexOf('+') + 1); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return (name.StartsWith(str, StringComparison.Ordinal)); } return (name.Equals(str)); } // FilterIgnoreCase // This delegate will do a name search but does it with the // ignore case specified. internal virtual bool FilterIgnoreCase(MemberInfo m,Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; str = str.Trim(); String name = m.Name; // Get the nested class name only, as opposed to the mangled one if (m.MemberType == MemberTypes.NestedType) name = name.Substring(name.LastIndexOf('+') + 1); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return (String.Compare(name,0,str,0,str.Length,StringComparison.OrdinalIgnoreCase)==0); } return (String.Compare(str,name, StringComparison.OrdinalIgnoreCase) == 0); } } }
Link Menu
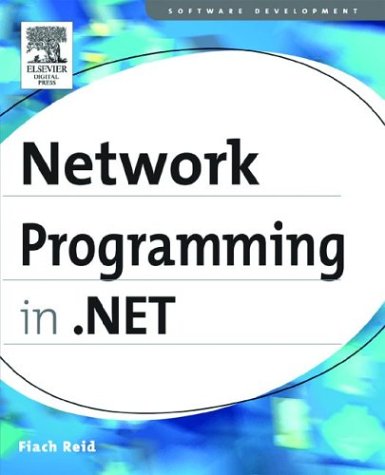
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmbeddedMailObject.cs
- ValueUtilsSmi.cs
- IndexerNameAttribute.cs
- QilList.cs
- TemplateXamlTreeBuilder.cs
- _DigestClient.cs
- InheritanceAttribute.cs
- WebHttpBindingElement.cs
- ObjectReferenceStack.cs
- WebServiceData.cs
- PermissionAttributes.cs
- ScrollChrome.cs
- DynamicILGenerator.cs
- ValidatedControlConverter.cs
- AttributeXamlType.cs
- TypefaceMetricsCache.cs
- NativeStructs.cs
- RichTextBox.cs
- ImageFormat.cs
- SafeNativeMethods.cs
- TextContainerHelper.cs
- ObjectListCommandEventArgs.cs
- Completion.cs
- WindowsAuthenticationModule.cs
- SiteMapDataSource.cs
- URLString.cs
- IListConverters.cs
- MissingMethodException.cs
- CodeRemoveEventStatement.cs
- LocalizabilityAttribute.cs
- UriSection.cs
- ColumnTypeConverter.cs
- DataSetUtil.cs
- XmlDownloadManager.cs
- HotSpot.cs
- SubpageParagraph.cs
- util.cs
- SectionRecord.cs
- TemplateControl.cs
- MouseOverProperty.cs
- ParentQuery.cs
- UnsafeNativeMethods.cs
- localization.cs
- CancellationState.cs
- MsmqAuthenticationMode.cs
- PerformanceCounterLib.cs
- VersionValidator.cs
- SplashScreenNativeMethods.cs
- ScrollChrome.cs
- ExpandCollapsePattern.cs
- WindowsPrincipal.cs
- DateTimeSerializationSection.cs
- NumberSubstitution.cs
- FragmentQueryKB.cs
- DataListItemCollection.cs
- CertificateManager.cs
- SelectiveScrollingGrid.cs
- Axis.cs
- BaseValidator.cs
- DataGridViewCellPaintingEventArgs.cs
- CanonicalXml.cs
- StateDesigner.CommentLayoutGlyph.cs
- MouseEvent.cs
- Grammar.cs
- CounterSampleCalculator.cs
- HtmlUtf8RawTextWriter.cs
- TextServicesContext.cs
- MailWebEventProvider.cs
- SaveFileDialog.cs
- DocumentPageViewAutomationPeer.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- AnimationClock.cs
- DataGridSortCommandEventArgs.cs
- ForwardPositionQuery.cs
- CacheOutputQuery.cs
- DefaultShape.cs
- DataGridViewSelectedCellCollection.cs
- DefaultValidator.cs
- validationstate.cs
- OutputCacheProfileCollection.cs
- RowParagraph.cs
- XPathBuilder.cs
- TypeTypeConverter.cs
- WizardStepBase.cs
- UrlPath.cs
- DrawingContextWalker.cs
- ComboBoxItem.cs
- MemberCollection.cs
- DefaultTraceListener.cs
- ContractMapping.cs
- ServiceDescriptions.cs
- EventDescriptorCollection.cs
- SqlLiftWhereClauses.cs
- BitmapSizeOptions.cs
- MaskedTextBox.cs
- SeekableReadStream.cs
- DataTable.cs
- TextTreeObjectNode.cs
- DynamicRenderer.cs
- OracleParameterBinding.cs