Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Serialization / XmlSerializerFactory.cs / 1 / XmlSerializerFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System.Reflection; using System.Collections; using System.IO; using System.Xml.Schema; using System; using System.Text; using System.Threading; using System.Globalization; using System.Security.Permissions; using System.Security.Policy; using System.Xml.Serialization.Configuration; using System.Diagnostics; using System.CodeDom.Compiler; ////// /// public class XmlSerializerFactory { static TempAssemblyCache cache = new TempAssemblyCache(); ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides, Type[] extraTypes, XmlRootAttribute root, string defaultNamespace) { return CreateSerializer(type, overrides, extraTypes, root, defaultNamespace, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlRootAttribute root) { return CreateSerializer(type, null, new Type[0], root, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, Type[] extraTypes) { return CreateSerializer(type, null, extraTypes, null, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides) { return CreateSerializer(type, overrides, new Type[0], null, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(XmlTypeMapping xmlTypeMapping) { TempAssembly tempAssembly = XmlSerializer.GenerateTempAssembly(xmlTypeMapping); return (XmlSerializer)tempAssembly.Contract.TypedSerializers[xmlTypeMapping.Key]; } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type) { return CreateSerializer(type, (string)null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, string defaultNamespace) { if (type == null) throw new ArgumentNullException("type"); TempAssembly tempAssembly = cache[defaultNamespace, type]; XmlTypeMapping mapping = null; if (tempAssembly == null) { lock (cache) { tempAssembly = cache[defaultNamespace, type]; if (tempAssembly == null) { XmlSerializerImplementation contract; Assembly assembly = TempAssembly.LoadGeneratedAssembly(type, defaultNamespace, out contract); if (assembly == null) { // need to reflect and generate new serialization assembly XmlReflectionImporter importer = new XmlReflectionImporter(defaultNamespace); mapping = importer.ImportTypeMapping(type, null, defaultNamespace); tempAssembly = XmlSerializer.GenerateTempAssembly(mapping, type, defaultNamespace); } else { tempAssembly = new TempAssembly(contract); } cache.Add(defaultNamespace, type, tempAssembly); } } } if (mapping == null) { mapping = XmlReflectionImporter.GetTopLevelMapping(type, defaultNamespace); } return (XmlSerializer)tempAssembly.Contract.GetSerializer(type); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides, Type[] extraTypes, XmlRootAttribute root, string defaultNamespace, string location, Evidence evidence) { if (type == null) throw new ArgumentNullException("type"); XmlReflectionImporter importer = new XmlReflectionImporter(overrides, defaultNamespace); for (int i = 0; i < extraTypes.Length; i++) importer.IncludeType(extraTypes[i]); XmlTypeMapping mapping = importer.ImportTypeMapping(type, root, defaultNamespace); TempAssembly tempAssembly = XmlSerializer.GenerateTempAssembly(mapping, type, defaultNamespace, location, evidence); return (XmlSerializer)tempAssembly.Contract.TypedSerializers[mapping.Key]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System.Reflection; using System.Collections; using System.IO; using System.Xml.Schema; using System; using System.Text; using System.Threading; using System.Globalization; using System.Security.Permissions; using System.Security.Policy; using System.Xml.Serialization.Configuration; using System.Diagnostics; using System.CodeDom.Compiler; ////// /// public class XmlSerializerFactory { static TempAssemblyCache cache = new TempAssemblyCache(); ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides, Type[] extraTypes, XmlRootAttribute root, string defaultNamespace) { return CreateSerializer(type, overrides, extraTypes, root, defaultNamespace, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlRootAttribute root) { return CreateSerializer(type, null, new Type[0], root, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, Type[] extraTypes) { return CreateSerializer(type, null, extraTypes, null, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides) { return CreateSerializer(type, overrides, new Type[0], null, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(XmlTypeMapping xmlTypeMapping) { TempAssembly tempAssembly = XmlSerializer.GenerateTempAssembly(xmlTypeMapping); return (XmlSerializer)tempAssembly.Contract.TypedSerializers[xmlTypeMapping.Key]; } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type) { return CreateSerializer(type, (string)null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, string defaultNamespace) { if (type == null) throw new ArgumentNullException("type"); TempAssembly tempAssembly = cache[defaultNamespace, type]; XmlTypeMapping mapping = null; if (tempAssembly == null) { lock (cache) { tempAssembly = cache[defaultNamespace, type]; if (tempAssembly == null) { XmlSerializerImplementation contract; Assembly assembly = TempAssembly.LoadGeneratedAssembly(type, defaultNamespace, out contract); if (assembly == null) { // need to reflect and generate new serialization assembly XmlReflectionImporter importer = new XmlReflectionImporter(defaultNamespace); mapping = importer.ImportTypeMapping(type, null, defaultNamespace); tempAssembly = XmlSerializer.GenerateTempAssembly(mapping, type, defaultNamespace); } else { tempAssembly = new TempAssembly(contract); } cache.Add(defaultNamespace, type, tempAssembly); } } } if (mapping == null) { mapping = XmlReflectionImporter.GetTopLevelMapping(type, defaultNamespace); } return (XmlSerializer)tempAssembly.Contract.GetSerializer(type); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides, Type[] extraTypes, XmlRootAttribute root, string defaultNamespace, string location, Evidence evidence) { if (type == null) throw new ArgumentNullException("type"); XmlReflectionImporter importer = new XmlReflectionImporter(overrides, defaultNamespace); for (int i = 0; i < extraTypes.Length; i++) importer.IncludeType(extraTypes[i]); XmlTypeMapping mapping = importer.ImportTypeMapping(type, root, defaultNamespace); TempAssembly tempAssembly = XmlSerializer.GenerateTempAssembly(mapping, type, defaultNamespace, location, evidence); return (XmlSerializer)tempAssembly.Contract.TypedSerializers[mapping.Key]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
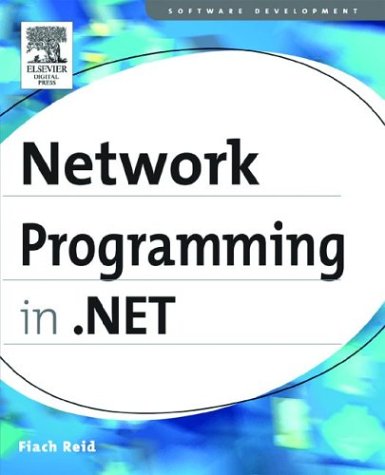
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionsZone.cs
- FrameworkElementAutomationPeer.cs
- JapaneseCalendar.cs
- ModuleBuilderData.cs
- Camera.cs
- SimplePropertyEntry.cs
- TypeConverterHelper.cs
- DocumentOrderQuery.cs
- PrimarySelectionGlyph.cs
- DbXmlEnabledProviderManifest.cs
- CompressedStack.cs
- XsdBuilder.cs
- WhereaboutsReader.cs
- Models.cs
- ListBindingHelper.cs
- OleDbWrapper.cs
- XmlElement.cs
- CheckBoxList.cs
- PocoEntityKeyStrategy.cs
- OutputCacheProviderCollection.cs
- Cursor.cs
- CodeAccessSecurityEngine.cs
- SignatureConfirmations.cs
- AnnotationAuthorChangedEventArgs.cs
- SchemaCollectionPreprocessor.cs
- ServicePointManagerElement.cs
- SafeNativeMethods.cs
- HttpCookiesSection.cs
- SpellerStatusTable.cs
- XmlAnyElementAttributes.cs
- WebPartHeaderCloseVerb.cs
- DBAsyncResult.cs
- AbandonedMutexException.cs
- AxisAngleRotation3D.cs
- SqlDataSourceCommandEventArgs.cs
- XamlValidatingReader.cs
- DataGridViewColumnDesigner.cs
- ProfilePropertyNameValidator.cs
- Point3DKeyFrameCollection.cs
- WebPartConnectionsConfigureVerb.cs
- Queue.cs
- ClientSettingsProvider.cs
- XamlPointCollectionSerializer.cs
- SectionXmlInfo.cs
- BezierSegment.cs
- WmlObjectListAdapter.cs
- RemotingConfigParser.cs
- BindingSourceDesigner.cs
- InfoCardSymmetricAlgorithm.cs
- PageEventArgs.cs
- SchemaElement.cs
- _FtpControlStream.cs
- EventWaitHandle.cs
- PerformanceCounterPermissionEntry.cs
- GuidelineCollection.cs
- ViewKeyConstraint.cs
- mansign.cs
- SignedXml.cs
- grammarelement.cs
- Encoding.cs
- ProcessHostConfigUtils.cs
- HyperLinkColumn.cs
- PlainXmlDeserializer.cs
- XmlExtensionFunction.cs
- QualifiedCellIdBoolean.cs
- KeyEventArgs.cs
- HierarchicalDataBoundControl.cs
- TextRangeEdit.cs
- METAHEADER.cs
- AssemblyHash.cs
- Geometry3D.cs
- BrowserCapabilitiesCompiler.cs
- ChannelServices.cs
- AdapterSwitches.cs
- OdbcError.cs
- ServiceOperationUIEditor.cs
- ConstructorNeedsTagAttribute.cs
- RedistVersionInfo.cs
- Positioning.cs
- IntPtr.cs
- EditingCommands.cs
- ViewBase.cs
- SystemUdpStatistics.cs
- PropertyChangedEventArgs.cs
- TcpHostedTransportConfiguration.cs
- SupportingTokenDuplexChannel.cs
- FilterException.cs
- SQLBinaryStorage.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- UrlRoutingHandler.cs
- NameValueConfigurationCollection.cs
- CrossAppDomainChannel.cs
- CompoundFileStreamReference.cs
- CalendarAutoFormat.cs
- SettingsPropertyValue.cs
- RegistrationServices.cs
- ReturnType.cs
- RegistrationServices.cs
- AppDomainShutdownMonitor.cs
- FlowDocumentView.cs