Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Reflection / Emit / Opcode.cs / 1 / Opcode.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Reflection.Emit { using System; using System.Security.Permissions; [System.Runtime.InteropServices.ComVisible(true)] public struct OpCode { internal String m_stringname; internal StackBehaviour m_pop; internal StackBehaviour m_push; internal OperandType m_operand; internal OpCodeType m_type; internal int m_size; internal byte m_s1; internal byte m_s2; internal FlowControl m_ctrl; // Specifies whether the current instructions causes the control flow to // change unconditionally. internal bool m_endsUncondJmpBlk; // Specifies the stack change that the current instruction causes not // taking into account the operand dependant stack changes. internal int m_stackChange; internal OpCode(String stringname, StackBehaviour pop, StackBehaviour push, OperandType operand, OpCodeType type, int size, byte s1, byte s2, FlowControl ctrl, bool endsjmpblk, int stack) { m_stringname = stringname; m_pop = pop; m_push = push; m_operand = operand; m_type = type; m_size = size; m_s1 = s1; m_s2 = s2; m_ctrl = ctrl; m_endsUncondJmpBlk = endsjmpblk; m_stackChange = stack; } internal bool EndsUncondJmpBlk() { return m_endsUncondJmpBlk; } internal int StackChange() { return m_stackChange; } public OperandType OperandType { get { return (m_operand); } } public FlowControl FlowControl { get { return (m_ctrl); } } public OpCodeType OpCodeType { get { return (m_type); } } public StackBehaviour StackBehaviourPop { get { return (m_pop); } } public StackBehaviour StackBehaviourPush { get { return (m_push); } } public int Size { get { return (m_size); } } public short Value { get { if (m_size == 2) return (short) (m_s1 << 8 | m_s2); return (short) m_s2; } } public String Name { get { return m_stringname; } } public override bool Equals(Object obj) { if (obj is OpCode) return Equals((OpCode)obj); else return false; } public bool Equals(OpCode obj) { return obj.m_s1 == m_s1 && obj.m_s2 == m_s2; } public static bool operator ==(OpCode a, OpCode b) { return a.Equals(b); } public static bool operator !=(OpCode a, OpCode b) { return !(a == b); } public override int GetHashCode() { return this.m_stringname.GetHashCode(); } public override String ToString() { return m_stringname; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Reflection.Emit { using System; using System.Security.Permissions; [System.Runtime.InteropServices.ComVisible(true)] public struct OpCode { internal String m_stringname; internal StackBehaviour m_pop; internal StackBehaviour m_push; internal OperandType m_operand; internal OpCodeType m_type; internal int m_size; internal byte m_s1; internal byte m_s2; internal FlowControl m_ctrl; // Specifies whether the current instructions causes the control flow to // change unconditionally. internal bool m_endsUncondJmpBlk; // Specifies the stack change that the current instruction causes not // taking into account the operand dependant stack changes. internal int m_stackChange; internal OpCode(String stringname, StackBehaviour pop, StackBehaviour push, OperandType operand, OpCodeType type, int size, byte s1, byte s2, FlowControl ctrl, bool endsjmpblk, int stack) { m_stringname = stringname; m_pop = pop; m_push = push; m_operand = operand; m_type = type; m_size = size; m_s1 = s1; m_s2 = s2; m_ctrl = ctrl; m_endsUncondJmpBlk = endsjmpblk; m_stackChange = stack; } internal bool EndsUncondJmpBlk() { return m_endsUncondJmpBlk; } internal int StackChange() { return m_stackChange; } public OperandType OperandType { get { return (m_operand); } } public FlowControl FlowControl { get { return (m_ctrl); } } public OpCodeType OpCodeType { get { return (m_type); } } public StackBehaviour StackBehaviourPop { get { return (m_pop); } } public StackBehaviour StackBehaviourPush { get { return (m_push); } } public int Size { get { return (m_size); } } public short Value { get { if (m_size == 2) return (short) (m_s1 << 8 | m_s2); return (short) m_s2; } } public String Name { get { return m_stringname; } } public override bool Equals(Object obj) { if (obj is OpCode) return Equals((OpCode)obj); else return false; } public bool Equals(OpCode obj) { return obj.m_s1 == m_s1 && obj.m_s2 == m_s2; } public static bool operator ==(OpCode a, OpCode b) { return a.Equals(b); } public static bool operator !=(OpCode a, OpCode b) { return !(a == b); } public override int GetHashCode() { return this.m_stringname.GetHashCode(); } public override String ToString() { return m_stringname; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
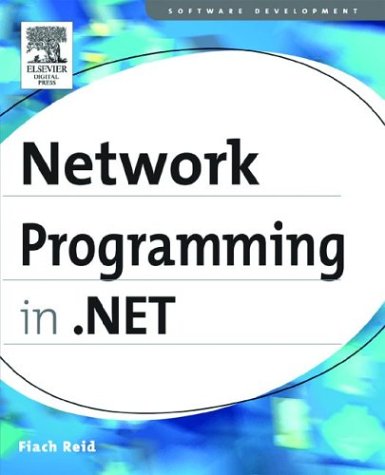
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultPrintController.cs
- StateMachineDesignerPaint.cs
- HighlightVisual.cs
- ActiveDocumentEvent.cs
- ArraySortHelper.cs
- FolderBrowserDialogDesigner.cs
- FontSourceCollection.cs
- TypeExtensions.cs
- SmtpTransport.cs
- InteropBitmapSource.cs
- IDReferencePropertyAttribute.cs
- FontNameEditor.cs
- ArgumentsParser.cs
- StandardToolWindows.cs
- ToolStripContainerActionList.cs
- SectionInput.cs
- X509Utils.cs
- EventMappingSettingsCollection.cs
- ToolStripOverflow.cs
- QueryContinueDragEventArgs.cs
- OdbcHandle.cs
- SparseMemoryStream.cs
- EntityDesignerUtils.cs
- SqlEnums.cs
- OleAutBinder.cs
- mactripleDES.cs
- Nullable.cs
- Monitor.cs
- SafeNativeMethods.cs
- SafeSystemMetrics.cs
- Style.cs
- PageParser.cs
- InOutArgumentConverter.cs
- BufferAllocator.cs
- TextEditorTyping.cs
- TreeNodeMouseHoverEvent.cs
- XmlSchemaSubstitutionGroup.cs
- TextParagraphProperties.cs
- Gdiplus.cs
- RSAPKCS1KeyExchangeFormatter.cs
- ConfigXmlText.cs
- Line.cs
- MemberRelationshipService.cs
- BitConverter.cs
- TemplatedAdorner.cs
- WebResponse.cs
- UTF8Encoding.cs
- MergeFailedEvent.cs
- XmlComment.cs
- TraceHandler.cs
- WebPartConnectionsCancelEventArgs.cs
- Block.cs
- MethodInfo.cs
- PageCatalogPart.cs
- DocumentProperties.cs
- TabRenderer.cs
- Expression.cs
- DataRowChangeEvent.cs
- Journal.cs
- HwndSource.cs
- FixedSOMTable.cs
- DbTransaction.cs
- wgx_sdk_version.cs
- Invariant.cs
- FixedDSBuilder.cs
- SqlNodeAnnotations.cs
- WindowsListViewGroupSubsetLink.cs
- BufferedOutputStream.cs
- HttpHandlerAction.cs
- ColumnCollection.cs
- GlyphingCache.cs
- ReversePositionQuery.cs
- PageThemeCodeDomTreeGenerator.cs
- AttachedAnnotation.cs
- OracleString.cs
- InputDevice.cs
- SQLByte.cs
- ListSortDescriptionCollection.cs
- sqlstateclientmanager.cs
- CellQuery.cs
- RemotingService.cs
- ProjectionPlanCompiler.cs
- FacetDescriptionElement.cs
- HotSpot.cs
- ViewManager.cs
- CodeTypeParameter.cs
- PlanCompilerUtil.cs
- TemplatePagerField.cs
- ListViewSortEventArgs.cs
- Int32RectConverter.cs
- ConfigurationCollectionAttribute.cs
- XmlBindingWorker.cs
- AtomMaterializerLog.cs
- RuntimeVariableList.cs
- ClientType.cs
- PasswordBox.cs
- BufferBuilder.cs
- PreviewPrintController.cs
- ClientFormsAuthenticationCredentials.cs
- CacheHelper.cs