Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / IconConverter.cs / 1 / IconConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.IO; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.ComponentModel.Design.Serialization; using System.Diagnostics.CodeAnalysis; ////// /// IconConverter is a class that can be used to convert /// Icon from one data type to another. Access this /// class through the TypeDescriptor. /// public class IconConverter : ExpandableObjectConverter { ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(byte[])) { return true; } if(sourceType == typeof(InstanceDescriptor)){ return false; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(Image) || destinationType == typeof(Bitmap)) { return true; } if (destinationType == typeof(byte[])) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is byte[]) { MemoryStream ms = new MemoryStream((byte[])value); return new Icon(ms); } return base.ConvertFrom(context, culture, value); } ///Converts the given object to the converter's native type. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] [SuppressMessage("Microsoft.Reliability", "CA2000:DisposeObjectsBeforeLosingScope")] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(Image) || destinationType == typeof(Bitmap)) { Icon icon = value as Icon; if( icon != null ) { return icon.ToBitmap(); } } if (destinationType == typeof(string)) { if (value != null) { return value.ToString(); } else { return SR.GetString(SR.toStringNone); } } if (destinationType == typeof(byte[])) { if (value != null) { MemoryStream ms = null; try { ms = new MemoryStream(); Icon icon = value as Icon; if( icon != null ) { icon.Save(ms); } } finally { if (ms != null) { ms.Close(); } } if (ms != null) { return ms.ToArray(); } else { return null; } } else { return new byte[0]; } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.IO; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.ComponentModel.Design.Serialization; using System.Diagnostics.CodeAnalysis; ////// /// IconConverter is a class that can be used to convert /// Icon from one data type to another. Access this /// class through the TypeDescriptor. /// public class IconConverter : ExpandableObjectConverter { ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(byte[])) { return true; } if(sourceType == typeof(InstanceDescriptor)){ return false; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(Image) || destinationType == typeof(Bitmap)) { return true; } if (destinationType == typeof(byte[])) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is byte[]) { MemoryStream ms = new MemoryStream((byte[])value); return new Icon(ms); } return base.ConvertFrom(context, culture, value); } ///Converts the given object to the converter's native type. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] [SuppressMessage("Microsoft.Reliability", "CA2000:DisposeObjectsBeforeLosingScope")] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(Image) || destinationType == typeof(Bitmap)) { Icon icon = value as Icon; if( icon != null ) { return icon.ToBitmap(); } } if (destinationType == typeof(string)) { if (value != null) { return value.ToString(); } else { return SR.GetString(SR.toStringNone); } } if (destinationType == typeof(byte[])) { if (value != null) { MemoryStream ms = null; try { ms = new MemoryStream(); Icon icon = value as Icon; if( icon != null ) { icon.Save(ms); } } finally { if (ms != null) { ms.Close(); } } if (ms != null) { return ms.ToArray(); } else { return null; } } else { return new byte[0]; } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
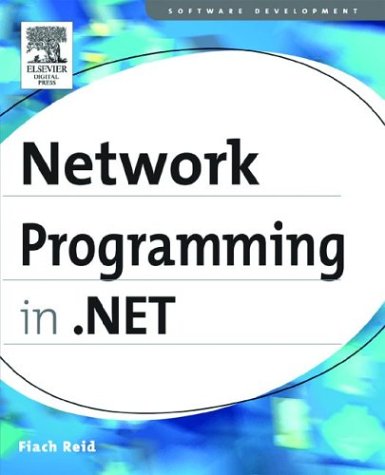
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerialReceived.cs
- RecordsAffectedEventArgs.cs
- DataContractSerializer.cs
- SecurityPermission.cs
- RowToParametersTransformer.cs
- WebPartHelpVerb.cs
- Thread.cs
- ComPlusDiagnosticTraceSchemas.cs
- DocumentPageTextView.cs
- TcpConnectionPoolSettings.cs
- TextLine.cs
- ConnectionPointGlyph.cs
- DataGridViewColumnConverter.cs
- GeneralTransform3DGroup.cs
- EvidenceTypeDescriptor.cs
- XsdDateTime.cs
- SelectingProviderEventArgs.cs
- MimeWriter.cs
- EditorAttribute.cs
- XPathDocumentIterator.cs
- Icon.cs
- WindowsBrush.cs
- CompilerGlobalScopeAttribute.cs
- ScriptControl.cs
- MembershipPasswordException.cs
- EditorZoneBase.cs
- DBBindings.cs
- initElementDictionary.cs
- BufferModesCollection.cs
- FolderBrowserDialog.cs
- OleDbCommandBuilder.cs
- DesignerEventService.cs
- TextSchema.cs
- PrePrepareMethodAttribute.cs
- DbReferenceCollection.cs
- DeploymentSection.cs
- QueryStringParameter.cs
- MenuTracker.cs
- TreeViewCancelEvent.cs
- ITextView.cs
- ExpressionParser.cs
- InnerItemCollectionView.cs
- CallbackHandler.cs
- ColumnWidthChangingEvent.cs
- ElementProxy.cs
- ProtectedConfiguration.cs
- SchemaMapping.cs
- CalendarItem.cs
- CssClassPropertyAttribute.cs
- RecognitionResult.cs
- Odbc32.cs
- VisualStyleTypesAndProperties.cs
- ThemeDirectoryCompiler.cs
- AudioStateChangedEventArgs.cs
- BitmapImage.cs
- SmiXetterAccessMap.cs
- ASCIIEncoding.cs
- ErrorsHelper.cs
- SwitchElementsCollection.cs
- Attribute.cs
- ObservableCollectionDefaultValueFactory.cs
- SecurityContextKeyIdentifierClause.cs
- ReadOnlyDataSource.cs
- CustomLineCap.cs
- FileDialog_Vista.cs
- CodeTypeOfExpression.cs
- LowerCaseStringConverter.cs
- StringExpressionSet.cs
- ButtonFieldBase.cs
- WebResourceUtil.cs
- ZipIOLocalFileHeader.cs
- UriTemplateMatchException.cs
- Track.cs
- MetadataArtifactLoaderResource.cs
- LocationUpdates.cs
- SystemDiagnosticsSection.cs
- DataControlLinkButton.cs
- BufferedReadStream.cs
- DetailsViewPagerRow.cs
- XmlElementAttributes.cs
- XmlImplementation.cs
- WorkflowDesigner.cs
- SuppressIldasmAttribute.cs
- mediaeventargs.cs
- RepeatButton.cs
- XmlSchemaNotation.cs
- ContextMenuStrip.cs
- FileDataSourceCache.cs
- EventEntry.cs
- SecurityKeyType.cs
- JsonEncodingStreamWrapper.cs
- XmlSchemaAnnotation.cs
- DataGridViewCellCancelEventArgs.cs
- Internal.cs
- HMACSHA1.cs
- ObjectDataSourceEventArgs.cs
- WebServiceEnumData.cs
- Container.cs
- PathFigureCollection.cs
- ComponentRenameEvent.cs