Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / BadImageFormatException.cs / 3 / BadImageFormatException.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: BadImageFormatException ** ** ** Purpose: Exception to an invalid dll or executable format. ** ** ===========================================================*/ namespace System { using System; using System.Runtime.Serialization; using FileLoadException = System.IO.FileLoadException; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; using System.Globalization; [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] public class BadImageFormatException : SystemException { private String _fileName; // The name of the corrupt PE file. private String _fusionLog; // fusion log (when applicable) public BadImageFormatException() : base(Environment.GetResourceString("Arg_BadImageFormatException")) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); } public BadImageFormatException(String message) : base(message) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); } public BadImageFormatException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); } public BadImageFormatException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); _fileName = fileName; } public BadImageFormatException(String message, String fileName, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) { if ((_fileName == null) && (HResult == System.__HResults.COR_E_EXCEPTION)) _message = Environment.GetResourceString("Arg_BadImageFormatException"); else _message = FileLoadException.FormatFileLoadExceptionMessage(_fileName, HResult); } } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected BadImageFormatException(SerializationInfo info, StreamingContext context) : base(info, context) { // Base class constructor will check info != null. _fileName = info.GetString("BadImageFormat_FileName"); try { _fusionLog = info.GetString("BadImageFormat_FusionLog"); } catch { _fusionLog = null; } } private BadImageFormatException(String fileName, String fusionLog, int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("BadImageFormat_FileName", _fileName, typeof(String)); try { info.AddValue("BadImageFormat_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: BadImageFormatException ** ** ** Purpose: Exception to an invalid dll or executable format. ** ** ===========================================================*/ namespace System { using System; using System.Runtime.Serialization; using FileLoadException = System.IO.FileLoadException; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; using System.Globalization; [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] public class BadImageFormatException : SystemException { private String _fileName; // The name of the corrupt PE file. private String _fusionLog; // fusion log (when applicable) public BadImageFormatException() : base(Environment.GetResourceString("Arg_BadImageFormatException")) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); } public BadImageFormatException(String message) : base(message) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); } public BadImageFormatException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); } public BadImageFormatException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); _fileName = fileName; } public BadImageFormatException(String message, String fileName, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_BADIMAGEFORMAT); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) { if ((_fileName == null) && (HResult == System.__HResults.COR_E_EXCEPTION)) _message = Environment.GetResourceString("Arg_BadImageFormatException"); else _message = FileLoadException.FormatFileLoadExceptionMessage(_fileName, HResult); } } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected BadImageFormatException(SerializationInfo info, StreamingContext context) : base(info, context) { // Base class constructor will check info != null. _fileName = info.GetString("BadImageFormat_FileName"); try { _fusionLog = info.GetString("BadImageFormat_FusionLog"); } catch { _fusionLog = null; } } private BadImageFormatException(String fileName, String fusionLog, int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("BadImageFormat_FileName", _fileName, typeof(String)); try { info.AddValue("BadImageFormat_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
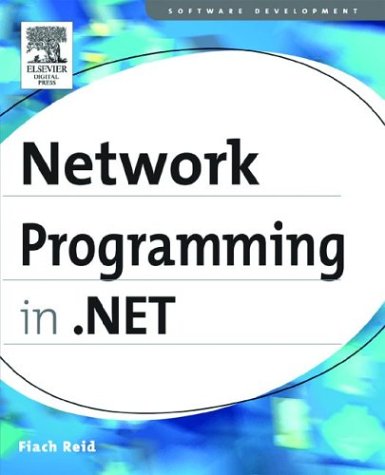
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaSubstitutionGroup.cs
- GeometryHitTestResult.cs
- UnsignedPublishLicense.cs
- ObjectStateEntry.cs
- X509AudioLogo.cs
- AccessControlList.cs
- WebHttpBehavior.cs
- DbConnectionPool.cs
- TabletCollection.cs
- TypeForwardedToAttribute.cs
- OdbcError.cs
- SQLInt64Storage.cs
- SmtpNegotiateAuthenticationModule.cs
- CacheOutputQuery.cs
- CompiledRegexRunnerFactory.cs
- WinInetCache.cs
- UTF8Encoding.cs
- DataRecord.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- Control.cs
- DatePickerTextBox.cs
- SqlProfileProvider.cs
- ToggleButton.cs
- ControlEvent.cs
- ClientBuildManagerCallback.cs
- DefaultHttpHandler.cs
- serverconfig.cs
- contentDescriptor.cs
- FtpWebResponse.cs
- Converter.cs
- ToolboxItemAttribute.cs
- WindowsToolbarAsMenu.cs
- URLIdentityPermission.cs
- XXXOnTypeBuilderInstantiation.cs
- HttpConfigurationSystem.cs
- WebGetAttribute.cs
- Memoizer.cs
- QueryResponse.cs
- ObjectContext.cs
- UpdateExpressionVisitor.cs
- DataGridViewDataErrorEventArgs.cs
- ValueUnavailableException.cs
- BamlWriter.cs
- SelectorItemAutomationPeer.cs
- UnsignedPublishLicense.cs
- EntityUtil.cs
- LineGeometry.cs
- ZipFileInfo.cs
- ChannelServices.cs
- ResourcesBuildProvider.cs
- ReflectionTypeLoadException.cs
- Point.cs
- OperationPerformanceCounters.cs
- LingerOption.cs
- UnauthorizedWebPart.cs
- AppDomainAttributes.cs
- AppSettingsReader.cs
- TextTreeRootTextBlock.cs
- Win32PrintDialog.cs
- CapabilitiesSection.cs
- ClickablePoint.cs
- ObjectStateManager.cs
- ZipFileInfoCollection.cs
- SetIndexBinder.cs
- SpotLight.cs
- DateTimeParse.cs
- RankException.cs
- HttpWriter.cs
- TableCellCollection.cs
- AuthenticationException.cs
- DrawingContextFlattener.cs
- ExitEventArgs.cs
- Guid.cs
- WindowsSecurityTokenAuthenticator.cs
- LazyTextWriterCreator.cs
- WebPartCatalogAddVerb.cs
- Size.cs
- UriTemplateLiteralQueryValue.cs
- updatecommandorderer.cs
- SqlDelegatedTransaction.cs
- AutomationElement.cs
- ScalarConstant.cs
- ValidationErrorEventArgs.cs
- ObjectFullSpanRewriter.cs
- ProviderBase.cs
- AutoScrollExpandMessageFilter.cs
- TextBounds.cs
- WebBrowserNavigatingEventHandler.cs
- XmlHelper.cs
- DeflateStreamAsyncResult.cs
- CompleteWizardStep.cs
- LinkedResource.cs
- RuleSettings.cs
- OutputScopeManager.cs
- PerfCounterSection.cs
- PageBreakRecord.cs
- SynchronizedCollection.cs
- NetworkInformationPermission.cs
- WindowShowOrOpenTracker.cs
- AutoGeneratedField.cs