Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / DefaultHttpHandler.cs / 3 / DefaultHttpHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Text; using System.Web.Util; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class DefaultHttpHandler : IHttpAsyncHandler { private HttpContext _context; private NameValueCollection _executeUrlHeaders; public DefaultHttpHandler() { } protected HttpContext Context { get { return _context; } } // headers to provide to execute url protected NameValueCollection ExecuteUrlHeaders { get { if (_executeUrlHeaders == null && _context != null) { _executeUrlHeaders = new NameValueCollection(_context.Request.Headers); } return _executeUrlHeaders; } } // called when we know a precondition for calling // execute URL has been violated public virtual void OnExecuteUrlPreconditionFailure() { // do nothing - the derived class might throw } // add a virtual method that provides the request target for the EXECUTE_URL call // if return null, the default calls EXECUTE_URL for the default target public virtual String OverrideExecuteUrlPath() { return null; } internal static bool IsClassicAspRequest(String filePath) { return StringUtil.StringEndsWithIgnoreCase(filePath, ".asp"); } public virtual IAsyncResult BeginProcessRequest(HttpContext context, AsyncCallback callback, Object state) { // DDB 168193: DefaultHttpHandler is obsolate in integrated mode if (HttpRuntime.UseIntegratedPipeline) { throw new PlatformNotSupportedException(SR.GetString(SR.Method_Not_Supported_By_Iis_Integrated_Mode, "DefaultHttpHandler.BeginProcessRequest")); } _context = context; HttpResponse response = _context.Response; if (response.CanExecuteUrlForEntireResponse) { // use ExecuteUrl String path = OverrideExecuteUrlPath(); if (path != null && !HttpRuntime.IsFullTrust) { // validate that an untrusted derived classes (not in GAC) // didn't set the path to a place that CAS wouldn't let it access if (!this.GetType().Assembly.GlobalAssemblyCache) { HttpRuntime.CheckFilePermission(context.Request.MapPath(path)); } } return response.BeginExecuteUrlForEntireResponse(path, _executeUrlHeaders, callback, state); } else { // let the base class throw if it doesn't want static files handler OnExecuteUrlPreconditionFailure(); // use static file handler _context = null; // don't keep request data alive in sync case HttpRequest request = context.Request; // refuse POST requests if (request.HttpVerb == HttpVerb.POST) { throw new HttpException(405, SR.GetString(SR.Method_not_allowed, request.HttpMethod, request.Path)); } // refuse .asp requests if (IsClassicAspRequest(request.FilePath)) { throw new HttpException(403, SR.GetString(SR.Path_forbidden, request.Path)); } // default to static file handler StaticFileHandler.ProcessRequestInternal(context); // return async result indicating completion return new HttpAsyncResult(callback, state, true, null, null); } } public virtual void EndProcessRequest(IAsyncResult result) { if (_context != null) { HttpResponse response = _context.Response; _context = null; response.EndExecuteUrlForEntireResponse(result); } } public virtual void ProcessRequest(HttpContext context) { // this handler should never be called synchronously throw new InvalidOperationException(SR.GetString(SR.Cannot_call_defaulthttphandler_sync)); } public virtual bool IsReusable { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
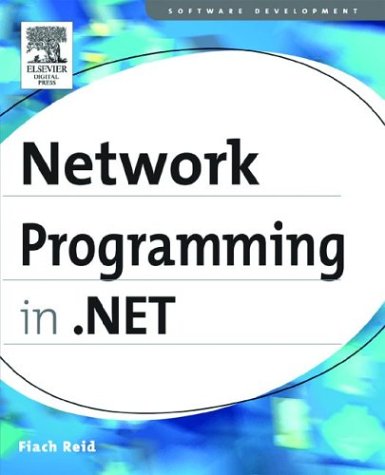
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- URL.cs
- ZoneIdentityPermission.cs
- FormsAuthenticationTicket.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- DetailsViewInsertEventArgs.cs
- DoubleAnimationBase.cs
- TextRangeEditTables.cs
- EdmItemCollection.OcAssemblyCache.cs
- ListBoxItemAutomationPeer.cs
- OfTypeExpression.cs
- VideoDrawing.cs
- SerializerWriterEventHandlers.cs
- GeneralTransform.cs
- DrawingVisual.cs
- NativeMethods.cs
- XmlSignificantWhitespace.cs
- TransactionFlowBindingElement.cs
- RelatedImageListAttribute.cs
- MapPathBasedVirtualPathProvider.cs
- RunClient.cs
- BmpBitmapEncoder.cs
- RegexGroupCollection.cs
- GeometryHitTestParameters.cs
- FormatConvertedBitmap.cs
- PartialList.cs
- WorkflowRuntimeServiceElementCollection.cs
- ErrorRuntimeConfig.cs
- objectresult_tresulttype.cs
- RuleSet.cs
- StylusButton.cs
- LongPath.cs
- PathParser.cs
- FSWPathEditor.cs
- TextFormatterHost.cs
- HtmlInputText.cs
- XPathNodeList.cs
- AlphabeticalEnumConverter.cs
- SingleAnimation.cs
- CellLabel.cs
- ParseNumbers.cs
- InfoCardArgumentException.cs
- DataGridParentRows.cs
- CompositeCollection.cs
- BitmapPalettes.cs
- MaskedTextBox.cs
- DataPagerField.cs
- DesignerVerbCollection.cs
- ParserStreamGeometryContext.cs
- VectorAnimationBase.cs
- SqlClientFactory.cs
- RightsManagementPermission.cs
- TextEditorThreadLocalStore.cs
- PriorityQueue.cs
- PathData.cs
- nulltextcontainer.cs
- Component.cs
- SQLInt16Storage.cs
- InternalConfigHost.cs
- IBuiltInEvidence.cs
- WindowsPrincipal.cs
- DbParameterCollectionHelper.cs
- CssClassPropertyAttribute.cs
- DeclarativeExpressionConditionDeclaration.cs
- TracingConnectionListener.cs
- OledbConnectionStringbuilder.cs
- ReadOnlyHierarchicalDataSource.cs
- DataSourceNameHandler.cs
- EasingFunctionBase.cs
- DataSvcMapFileSerializer.cs
- UrlParameterWriter.cs
- Random.cs
- MediaPlayerState.cs
- SystemFonts.cs
- IntPtr.cs
- ExpressionDumper.cs
- AppDomainUnloadedException.cs
- TableRow.cs
- Rule.cs
- StoragePropertyMapping.cs
- FormatVersion.cs
- ALinqExpressionVisitor.cs
- ChameleonKey.cs
- XmlSchemaCompilationSettings.cs
- ScriptControlDescriptor.cs
- relpropertyhelper.cs
- InternalPermissions.cs
- DetailsViewRowCollection.cs
- IriParsingElement.cs
- EdmScalarPropertyAttribute.cs
- UriTemplateClientFormatter.cs
- PrintDialog.cs
- BulletedList.cs
- ParallelEnumerableWrapper.cs
- ExceptionUtil.cs
- DataGridViewTextBoxEditingControl.cs
- SkewTransform.cs
- WebPartDescriptionCollection.cs
- LinearGradientBrush.cs
- QueryStringParameter.cs
- XmlEncodedRawTextWriter.cs