Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / DatePickerTextBox.cs / 1305600 / DatePickerTextBox.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Diagnostics; using System.Globalization; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Input; using System.Windows.Media; namespace System.Windows.Controls.Primitives { ////// DatePickerTextBox is a specialized form of TextBox which displays custom visuals when its contents are empty /// [TemplatePart(Name = DatePickerTextBox.ElementContentName, Type = typeof(ContentControl))] public sealed partial class DatePickerTextBox : TextBox { #region Constants private const string ElementContentName = "PART_Watermark"; #endregion #region Data private ContentControl elementContent; #endregion #region Constructor ////// Static constructor /// static DatePickerTextBox() { DefaultStyleKeyProperty.OverrideMetadata(typeof(DatePickerTextBox), new FrameworkPropertyMetadata(typeof(DatePickerTextBox))); TextProperty.OverrideMetadata(typeof(DatePickerTextBox), new FrameworkPropertyMetadata(OnVisualStatePropertyChanged)); } ////// Initializes a new instance of the public DatePickerTextBox() { this.SetCurrentValue(WatermarkProperty, SR.Get(SRID.DatePickerTextBox_DefaultWatermarkText)); this.Loaded += OnLoaded; this.IsEnabledChanged += new DependencyPropertyChangedEventHandler(OnDatePickerTextBoxIsEnabledChanged); } #endregion #region Public Properties #region Watermark ///class. /// /// Watermark dependency property /// internal static readonly DependencyProperty WatermarkProperty = DependencyProperty.Register( "Watermark", typeof(object), typeof(DatePickerTextBox), new PropertyMetadata(OnWatermarkPropertyChanged)); ////// Watermark content /// ///The watermark. internal object Watermark { get { return (object)GetValue(WatermarkProperty); } set { SetValue(WatermarkProperty, value); } } #endregion #endregion Public Properties #region Protected ////// Called when template is applied to the control. /// public override void OnApplyTemplate() { base.OnApplyTemplate(); elementContent = ExtractTemplatePart(ElementContentName); // We dont want to expose watermark property as public yet, because there // is a good chance in future that the implementation will change when // a WatermarkTextBox control gets implemented. This is mostly to // mimc SL. Hence setting the binding in code rather than in control template. if (elementContent != null) { Binding watermarkBinding = new Binding("Watermark"); watermarkBinding.Source = this; elementContent.SetBinding(ContentControl.ContentProperty, watermarkBinding); } OnWatermarkChanged(); } protected override void OnGotFocus(RoutedEventArgs e) { base.OnGotFocus(e); if (IsEnabled) { if (!string.IsNullOrEmpty(this.Text)) { Select(0, this.Text.Length); } } } #endregion Protected #region Private private void OnLoaded(object sender, RoutedEventArgs e) { ApplyTemplate(); } /// /// Change to the correct visual state for the textbox. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { base.ChangeVisualState(useTransitions); // Update the WatermarkStates group if (this.Watermark != null && string.IsNullOrEmpty(this.Text)) { VisualStates.GoToState(this, useTransitions, VisualStates.StateWatermarked, VisualStates.StateUnwatermarked); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateUnwatermarked); } } private T ExtractTemplatePart(string partName) where T : DependencyObject { DependencyObject obj = GetTemplateChild(partName); return ExtractTemplatePart (partName, obj); } private static T ExtractTemplatePart (string partName, DependencyObject obj) where T : DependencyObject { Debug.Assert( obj == null || typeof(T).IsInstanceOfType(obj), string.Format(CultureInfo.InvariantCulture, SR.Get(SRID.DatePickerTextBox_TemplatePartIsOfIncorrectType), partName, typeof(T).Name)); return obj as T; } /// /// Called when the IsEnabled property changes. /// /// Sender object /// Property changed args private void OnDatePickerTextBoxIsEnabledChanged(object sender, DependencyPropertyChangedEventArgs e) { Debug.Assert(e.NewValue is bool); bool isEnabled = (bool)e.NewValue; SetCurrentValueInternal(IsReadOnlyProperty, MS.Internal.KnownBoxes.BooleanBoxes.Box(!isEnabled)); } private void OnWatermarkChanged() { if (elementContent != null) { Control watermarkControl = this.Watermark as Control; if (watermarkControl != null) { watermarkControl.IsTabStop = false; watermarkControl.IsHitTestVisible = false; } } } ////// Called when watermark property is changed. /// /// The sender. /// Theinstance containing the event data. private static void OnWatermarkPropertyChanged(DependencyObject sender, DependencyPropertyChangedEventArgs args) { DatePickerTextBox datePickerTextBox = sender as DatePickerTextBox; Debug.Assert(datePickerTextBox != null, "The source is not an instance of a DatePickerTextBox!"); datePickerTextBox.OnWatermarkChanged(); datePickerTextBox.UpdateVisualState(); } #endregion Private } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Diagnostics; using System.Globalization; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Input; using System.Windows.Media; namespace System.Windows.Controls.Primitives { /// /// DatePickerTextBox is a specialized form of TextBox which displays custom visuals when its contents are empty /// [TemplatePart(Name = DatePickerTextBox.ElementContentName, Type = typeof(ContentControl))] public sealed partial class DatePickerTextBox : TextBox { #region Constants private const string ElementContentName = "PART_Watermark"; #endregion #region Data private ContentControl elementContent; #endregion #region Constructor ////// Static constructor /// static DatePickerTextBox() { DefaultStyleKeyProperty.OverrideMetadata(typeof(DatePickerTextBox), new FrameworkPropertyMetadata(typeof(DatePickerTextBox))); TextProperty.OverrideMetadata(typeof(DatePickerTextBox), new FrameworkPropertyMetadata(OnVisualStatePropertyChanged)); } ////// Initializes a new instance of the public DatePickerTextBox() { this.SetCurrentValue(WatermarkProperty, SR.Get(SRID.DatePickerTextBox_DefaultWatermarkText)); this.Loaded += OnLoaded; this.IsEnabledChanged += new DependencyPropertyChangedEventHandler(OnDatePickerTextBoxIsEnabledChanged); } #endregion #region Public Properties #region Watermark ///class. /// /// Watermark dependency property /// internal static readonly DependencyProperty WatermarkProperty = DependencyProperty.Register( "Watermark", typeof(object), typeof(DatePickerTextBox), new PropertyMetadata(OnWatermarkPropertyChanged)); ////// Watermark content /// ///The watermark. internal object Watermark { get { return (object)GetValue(WatermarkProperty); } set { SetValue(WatermarkProperty, value); } } #endregion #endregion Public Properties #region Protected ////// Called when template is applied to the control. /// public override void OnApplyTemplate() { base.OnApplyTemplate(); elementContent = ExtractTemplatePart(ElementContentName); // We dont want to expose watermark property as public yet, because there // is a good chance in future that the implementation will change when // a WatermarkTextBox control gets implemented. This is mostly to // mimc SL. Hence setting the binding in code rather than in control template. if (elementContent != null) { Binding watermarkBinding = new Binding("Watermark"); watermarkBinding.Source = this; elementContent.SetBinding(ContentControl.ContentProperty, watermarkBinding); } OnWatermarkChanged(); } protected override void OnGotFocus(RoutedEventArgs e) { base.OnGotFocus(e); if (IsEnabled) { if (!string.IsNullOrEmpty(this.Text)) { Select(0, this.Text.Length); } } } #endregion Protected #region Private private void OnLoaded(object sender, RoutedEventArgs e) { ApplyTemplate(); } /// /// Change to the correct visual state for the textbox. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { base.ChangeVisualState(useTransitions); // Update the WatermarkStates group if (this.Watermark != null && string.IsNullOrEmpty(this.Text)) { VisualStates.GoToState(this, useTransitions, VisualStates.StateWatermarked, VisualStates.StateUnwatermarked); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateUnwatermarked); } } private T ExtractTemplatePart(string partName) where T : DependencyObject { DependencyObject obj = GetTemplateChild(partName); return ExtractTemplatePart (partName, obj); } private static T ExtractTemplatePart (string partName, DependencyObject obj) where T : DependencyObject { Debug.Assert( obj == null || typeof(T).IsInstanceOfType(obj), string.Format(CultureInfo.InvariantCulture, SR.Get(SRID.DatePickerTextBox_TemplatePartIsOfIncorrectType), partName, typeof(T).Name)); return obj as T; } /// /// Called when the IsEnabled property changes. /// /// Sender object /// Property changed args private void OnDatePickerTextBoxIsEnabledChanged(object sender, DependencyPropertyChangedEventArgs e) { Debug.Assert(e.NewValue is bool); bool isEnabled = (bool)e.NewValue; SetCurrentValueInternal(IsReadOnlyProperty, MS.Internal.KnownBoxes.BooleanBoxes.Box(!isEnabled)); } private void OnWatermarkChanged() { if (elementContent != null) { Control watermarkControl = this.Watermark as Control; if (watermarkControl != null) { watermarkControl.IsTabStop = false; watermarkControl.IsHitTestVisible = false; } } } ////// Called when watermark property is changed. /// /// The sender. /// Theinstance containing the event data. private static void OnWatermarkPropertyChanged(DependencyObject sender, DependencyPropertyChangedEventArgs args) { DatePickerTextBox datePickerTextBox = sender as DatePickerTextBox; Debug.Assert(datePickerTextBox != null, "The source is not an instance of a DatePickerTextBox!"); datePickerTextBox.OnWatermarkChanged(); datePickerTextBox.UpdateVisualState(); } #endregion Private } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
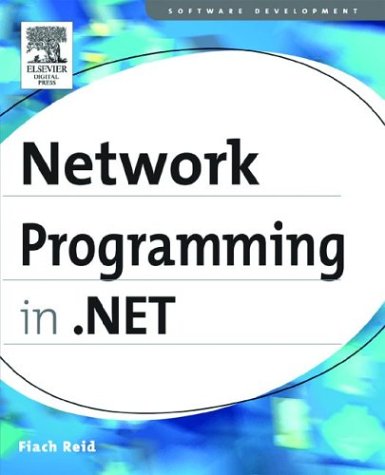
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TagPrefixAttribute.cs
- DataControlFieldCollection.cs
- FormView.cs
- HttpRuntimeSection.cs
- DataGridCaption.cs
- Transform3DGroup.cs
- DataBinder.cs
- IssuedTokenClientBehaviorsElement.cs
- CreateUserErrorEventArgs.cs
- EntityClientCacheKey.cs
- odbcmetadatacolumnnames.cs
- CodeChecksumPragma.cs
- PromptStyle.cs
- DataContract.cs
- CodeIdentifier.cs
- WindowsSolidBrush.cs
- shaperfactory.cs
- ScriptHandlerFactory.cs
- CallbackValidatorAttribute.cs
- WorkingDirectoryEditor.cs
- InvokeMethodActivity.cs
- ToolboxDataAttribute.cs
- FunctionDetailsReader.cs
- DecoderFallback.cs
- TextBoxView.cs
- Renderer.cs
- XmlAttributeAttribute.cs
- ProfileProvider.cs
- ModifiableIteratorCollection.cs
- SByteConverter.cs
- PasswordBox.cs
- Vector.cs
- ExpressionBindingCollection.cs
- CqlParserHelpers.cs
- NumberFormatInfo.cs
- WebServiceEndpoint.cs
- MDIClient.cs
- ControlBindingsCollection.cs
- ColorPalette.cs
- Pkcs7Signer.cs
- SemanticBasicElement.cs
- SafePEFileHandle.cs
- ThicknessAnimation.cs
- AnnotationDocumentPaginator.cs
- TypeNameConverter.cs
- SqlRowUpdatedEvent.cs
- ScrollPattern.cs
- ChangeTracker.cs
- WorkflowTransactionOptions.cs
- ConstantProjectedSlot.cs
- TextRange.cs
- RoutedEventHandlerInfo.cs
- ObjectDataSourceSelectingEventArgs.cs
- ManagedFilter.cs
- NestedContainer.cs
- CommonDialog.cs
- ICspAsymmetricAlgorithm.cs
- BitVector32.cs
- DbProviderServices.cs
- EventSinkHelperWriter.cs
- Quaternion.cs
- DownloadProgressEventArgs.cs
- DataBoundControl.cs
- ParameterElementCollection.cs
- CapiNative.cs
- DataGridViewSelectedCellCollection.cs
- ConcatQueryOperator.cs
- SpStreamWrapper.cs
- AlignmentYValidation.cs
- FontWeightConverter.cs
- SignedPkcs7.cs
- BitmapDecoder.cs
- CapabilitiesState.cs
- HwndSubclass.cs
- ObjectListFieldCollection.cs
- Frame.cs
- CultureSpecificStringDictionary.cs
- ArrangedElement.cs
- MatrixCamera.cs
- HttpCachePolicy.cs
- DataRelationPropertyDescriptor.cs
- EndOfStreamException.cs
- ServiceReference.cs
- Point3DCollectionValueSerializer.cs
- PageParserFilter.cs
- DataTableCollection.cs
- StoreConnection.cs
- AutomationElementCollection.cs
- SharedUtils.cs
- TextFormatterContext.cs
- PartitionedStreamMerger.cs
- ProfileModule.cs
- ViewStateException.cs
- TypeSystem.cs
- ILGenerator.cs
- DateTimeOffsetStorage.cs
- RemoteWebConfigurationHostStream.cs
- EntityModelSchemaGenerator.cs
- WebControlAdapter.cs
- StorageComplexPropertyMapping.cs