Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / MediaContextNotificationWindow.cs / 1 / MediaContextNotificationWindow.cs
//------------------------------------------------------------------------------ // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // A wrapper for a top-level hidden window that is used to process // messages broadcasted to top-level windows only (such as DWM's // WM_DWMCOMPOSITIONCHANGED). If the WPF application doesn't have // a top-level window (as it is the case for XBAP applications), // such messages would have been ignored. // //----------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using Microsoft.Win32; using Microsoft.Internal; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; using SafeNativeMethods=MS.Win32.PresentationCore.SafeNativeMethods; namespace System.Windows.Media { ////// The MediaContextNotificationWindow structure provides its owner /// MediaContext with the ability to receive and forward window /// messages broadcasted to top-level windows. /// internal struct MediaContextNotificationWindow { //+--------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods ////// Sets the owner MediaContext and creates the notification window. /// ////// Critical - Creates an HwndWrapper and adds a hook. /// TreatAsSafe: The _hwndNotification window is critical and this function is safe to call /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateNotificationWindow(MediaContext ownerMediaContext) { // Remember the pointer to the owner MediaContext that we'll forward the broadcasts to. _ownerMediaContext = ownerMediaContext; // Create a top-level, invisible window so we can get the WM_DWMCOMPOSITIONCHANGED // and other DWM notifications that are broadcasted to top-level windows only. HwndWrapper hwndNotification; hwndNotification = new HwndWrapper(0, NativeMethods.WS_POPUP, 0, 0, 0, 0, 0, "MediaContextNotificationWindow", IntPtr.Zero, null); _hwndNotificationHook = new HwndWrapperHook(MessageFilter); _hwndNotification = new SecurityCriticalDataClass(hwndNotification); _hwndNotification.Value.AddHook(_hwndNotificationHook); } /// /// Critical - Calls dispose on the critical hwnd wrapper. /// TreatAsSafe: It is safe to dispose the wrapper /// [SecurityCritical, SecurityTreatAsSafe] internal void DisposeNotificationWindow() { if (_hwndNotification != null) _hwndNotification.Value.Dispose(); _hwndNotificationHook = null; _hwndNotification = null; _ownerMediaContext = null; } #endregion Internal Methods //+---------------------------------------------------------------------- // // Private Methods // //--------------------------------------------------------------------- #region Private Methods ////// If any of the interesting broadcast messages is seen, forward them to the owner MediaContext. /// private IntPtr MessageFilter(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { Debug.Assert(_ownerMediaContext != null); if (msg == NativeMethods.WM_DWMCOMPOSITIONCHANGED) { _ownerMediaContext.OnDWMCompositionChanged(); } return IntPtr.Zero; } #endregion Private Methods //+---------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields // The owner MediaContext private MediaContext _ownerMediaContext; // A top-level hidden window. private SecurityCriticalDataClass_hwndNotification; // The message filter hook for the top-level hidden window. private HwndWrapperHook _hwndNotificationHook; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // A wrapper for a top-level hidden window that is used to process // messages broadcasted to top-level windows only (such as DWM's // WM_DWMCOMPOSITIONCHANGED). If the WPF application doesn't have // a top-level window (as it is the case for XBAP applications), // such messages would have been ignored. // //----------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using Microsoft.Win32; using Microsoft.Internal; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; using SafeNativeMethods=MS.Win32.PresentationCore.SafeNativeMethods; namespace System.Windows.Media { ////// The MediaContextNotificationWindow structure provides its owner /// MediaContext with the ability to receive and forward window /// messages broadcasted to top-level windows. /// internal struct MediaContextNotificationWindow { //+--------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods ////// Sets the owner MediaContext and creates the notification window. /// ////// Critical - Creates an HwndWrapper and adds a hook. /// TreatAsSafe: The _hwndNotification window is critical and this function is safe to call /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateNotificationWindow(MediaContext ownerMediaContext) { // Remember the pointer to the owner MediaContext that we'll forward the broadcasts to. _ownerMediaContext = ownerMediaContext; // Create a top-level, invisible window so we can get the WM_DWMCOMPOSITIONCHANGED // and other DWM notifications that are broadcasted to top-level windows only. HwndWrapper hwndNotification; hwndNotification = new HwndWrapper(0, NativeMethods.WS_POPUP, 0, 0, 0, 0, 0, "MediaContextNotificationWindow", IntPtr.Zero, null); _hwndNotificationHook = new HwndWrapperHook(MessageFilter); _hwndNotification = new SecurityCriticalDataClass(hwndNotification); _hwndNotification.Value.AddHook(_hwndNotificationHook); } /// /// Critical - Calls dispose on the critical hwnd wrapper. /// TreatAsSafe: It is safe to dispose the wrapper /// [SecurityCritical, SecurityTreatAsSafe] internal void DisposeNotificationWindow() { if (_hwndNotification != null) _hwndNotification.Value.Dispose(); _hwndNotificationHook = null; _hwndNotification = null; _ownerMediaContext = null; } #endregion Internal Methods //+---------------------------------------------------------------------- // // Private Methods // //--------------------------------------------------------------------- #region Private Methods ////// If any of the interesting broadcast messages is seen, forward them to the owner MediaContext. /// private IntPtr MessageFilter(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { Debug.Assert(_ownerMediaContext != null); if (msg == NativeMethods.WM_DWMCOMPOSITIONCHANGED) { _ownerMediaContext.OnDWMCompositionChanged(); } return IntPtr.Zero; } #endregion Private Methods //+---------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields // The owner MediaContext private MediaContext _ownerMediaContext; // A top-level hidden window. private SecurityCriticalDataClass_hwndNotification; // The message filter hook for the top-level hidden window. private HwndWrapperHook _hwndNotificationHook; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
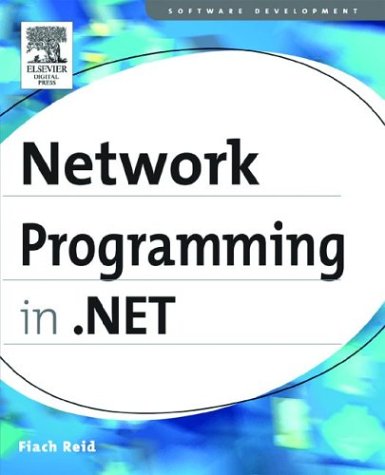
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewGroup.cs
- Light.cs
- ResourceLoader.cs
- NamedElement.cs
- XmlSchemaObjectTable.cs
- _SafeNetHandles.cs
- IdentityValidationException.cs
- WinFormsComponentEditor.cs
- DataGridLinkButton.cs
- HtmlTable.cs
- DbProviderFactories.cs
- DrawingVisualDrawingContext.cs
- SBCSCodePageEncoding.cs
- DBConnectionString.cs
- CellLabel.cs
- InheritedPropertyChangedEventArgs.cs
- FloatUtil.cs
- EmptyReadOnlyDictionaryInternal.cs
- DefaultWorkflowTransactionService.cs
- DocumentViewer.cs
- ObjectStateFormatter.cs
- AudioFormatConverter.cs
- StringConverter.cs
- FacetDescriptionElement.cs
- JsonDataContract.cs
- GiveFeedbackEvent.cs
- path.cs
- PenLineJoinValidation.cs
- TransformCollection.cs
- BitmapCache.cs
- ObjectKeyFrameCollection.cs
- BamlTreeUpdater.cs
- AndCondition.cs
- M3DUtil.cs
- FontStyles.cs
- TextElement.cs
- ApplicationInterop.cs
- DataGridItemCollection.cs
- ResourceAttributes.cs
- FontFamily.cs
- VoiceObjectToken.cs
- DesignerOptionService.cs
- ImageSourceValueSerializer.cs
- SerializationException.cs
- TypedElement.cs
- WindowsFormsSynchronizationContext.cs
- XhtmlConformanceSection.cs
- TranslateTransform.cs
- WebResourceUtil.cs
- XmlSchemaChoice.cs
- StateBag.cs
- ControlBuilder.cs
- InstanceDataCollection.cs
- Trace.cs
- CompModHelpers.cs
- Enlistment.cs
- ScalarConstant.cs
- PagedDataSource.cs
- CompositionAdorner.cs
- EmbeddedMailObject.cs
- AdRotatorDesigner.cs
- WebPartConnectionsCancelEventArgs.cs
- DesignerLoader.cs
- ProtocolsSection.cs
- FaultPropagationRecord.cs
- Cursor.cs
- HtmlUtf8RawTextWriter.cs
- GridPattern.cs
- LockRecursionException.cs
- StreamHelper.cs
- TextSelectionHighlightLayer.cs
- NegationPusher.cs
- GeometryConverter.cs
- HistoryEventArgs.cs
- ObjectDataSourceMethodEventArgs.cs
- MorphHelpers.cs
- _ShellExpression.cs
- UserInitiatedRoutedEventPermission.cs
- AttributeQuery.cs
- FieldToken.cs
- TableRowCollection.cs
- RegexInterpreter.cs
- CalendarData.cs
- XamlTypeMapper.cs
- EndpointPerformanceCounters.cs
- LinkedResourceCollection.cs
- StyleModeStack.cs
- XmlSchemaType.cs
- ChangeToolStripParentVerb.cs
- ExceptionUtility.cs
- DataGridViewRowEventArgs.cs
- EventToken.cs
- TextContainerChangeEventArgs.cs
- HttpChannelFactory.cs
- Soap12ServerProtocol.cs
- coordinatorfactory.cs
- updatecommandorderer.cs
- WebBrowserPermission.cs
- WSTransactionSection.cs
- HttpWebRequest.cs