Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Generated / Brush.cs / 1 / Brush.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { [TypeConverter(typeof(BrushConverter))] [ValueSerializer(typeof(BrushValueSerializer))] // Used by MarkupWriter abstract partial class Brush : Animatable, IFormattable, DUCE.IResource { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Brush Clone() { return (Brush)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Brush CloneCurrentValue() { return (Brush)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Brush target = ((Brush) d); target.PropertyChanged(OpacityProperty); } private static void TransformPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Brush target = ((Brush) d); Transform oldV = (Transform) e.OldValue; Transform newV = (Transform) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(TransformProperty); } private static void RelativeTransformPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Brush target = ((Brush) d); Transform oldV = (Transform) e.OldValue; Transform newV = (Transform) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(RelativeTransformProperty); } #region Public Properties ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } ////// Transform - Transform. Default value is Transform.Identity. /// public Transform Transform { get { return (Transform) GetValue(TransformProperty); } set { SetValueInternal(TransformProperty, value); } } ////// RelativeTransform - Transform. Default value is Transform.Identity. /// public Transform RelativeTransform { get { return (Transform) GetValue(RelativeTransformProperty); } set { SetValueInternal(RelativeTransformProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal abstract DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel); ////// AddRefOnChannel /// DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { return AddRefOnChannelCore(channel); } } internal abstract void ReleaseOnChannelCore(DUCE.Channel channel); ////// ReleaseOnChannel /// void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { ReleaseOnChannelCore(channel); } } internal abstract DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel); ////// GetHandle /// DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle handle; using (CompositionEngineLock.Acquire()) { handle = GetHandleCore(channel); } return handle; } internal abstract int GetChannelCountCore(); ////// GetChannelCount /// int DUCE.IResource.GetChannelCount() { // must already be in composition lock here return GetChannelCountCore(); } internal abstract DUCE.Channel GetChannelCore(int index); ////// GetChannel /// DUCE.Channel DUCE.IResource.GetChannel(int index) { // must already be in composition lock here return GetChannelCore(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal virtual string ConvertToString(string format, IFormatProvider provider) { return base.ToString(); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the Brush.Opacity property. /// public static readonly DependencyProperty OpacityProperty; ////// The DependencyProperty for the Brush.Transform property. /// public static readonly DependencyProperty TransformProperty; ////// The DependencyProperty for the Brush.RelativeTransform property. /// public static readonly DependencyProperty RelativeTransformProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_Opacity = 1.0; internal static Transform s_Transform = Transform.Identity; internal static Transform s_RelativeTransform = Transform.Identity; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static Brush() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Transform == null || s_Transform.IsFrozen, "Detected context bound default value Brush.s_Transform (See OS Bug #947272)."); Debug.Assert(s_RelativeTransform == null || s_RelativeTransform.IsFrozen, "Detected context bound default value Brush.s_RelativeTransform (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(Brush); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); TransformProperty = RegisterProperty("Transform", typeof(Transform), typeofThis, Transform.Identity, new PropertyChangedCallback(TransformPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); RelativeTransformProperty = RegisterProperty("RelativeTransform", typeof(Transform), typeofThis, Transform.Identity, new PropertyChangedCallback(RelativeTransformPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { [TypeConverter(typeof(BrushConverter))] [ValueSerializer(typeof(BrushValueSerializer))] // Used by MarkupWriter abstract partial class Brush : Animatable, IFormattable, DUCE.IResource { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Brush Clone() { return (Brush)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Brush CloneCurrentValue() { return (Brush)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Brush target = ((Brush) d); target.PropertyChanged(OpacityProperty); } private static void TransformPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Brush target = ((Brush) d); Transform oldV = (Transform) e.OldValue; Transform newV = (Transform) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(TransformProperty); } private static void RelativeTransformPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } Brush target = ((Brush) d); Transform oldV = (Transform) e.OldValue; Transform newV = (Transform) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(RelativeTransformProperty); } #region Public Properties ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } ////// Transform - Transform. Default value is Transform.Identity. /// public Transform Transform { get { return (Transform) GetValue(TransformProperty); } set { SetValueInternal(TransformProperty, value); } } ////// RelativeTransform - Transform. Default value is Transform.Identity. /// public Transform RelativeTransform { get { return (Transform) GetValue(RelativeTransformProperty); } set { SetValueInternal(RelativeTransformProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal abstract DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel); ////// AddRefOnChannel /// DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { return AddRefOnChannelCore(channel); } } internal abstract void ReleaseOnChannelCore(DUCE.Channel channel); ////// ReleaseOnChannel /// void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { ReleaseOnChannelCore(channel); } } internal abstract DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel); ////// GetHandle /// DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle handle; using (CompositionEngineLock.Acquire()) { handle = GetHandleCore(channel); } return handle; } internal abstract int GetChannelCountCore(); ////// GetChannelCount /// int DUCE.IResource.GetChannelCount() { // must already be in composition lock here return GetChannelCountCore(); } internal abstract DUCE.Channel GetChannelCore(int index); ////// GetChannel /// DUCE.Channel DUCE.IResource.GetChannel(int index) { // must already be in composition lock here return GetChannelCore(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal virtual string ConvertToString(string format, IFormatProvider provider) { return base.ToString(); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the Brush.Opacity property. /// public static readonly DependencyProperty OpacityProperty; ////// The DependencyProperty for the Brush.Transform property. /// public static readonly DependencyProperty TransformProperty; ////// The DependencyProperty for the Brush.RelativeTransform property. /// public static readonly DependencyProperty RelativeTransformProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_Opacity = 1.0; internal static Transform s_Transform = Transform.Identity; internal static Transform s_RelativeTransform = Transform.Identity; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static Brush() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Transform == null || s_Transform.IsFrozen, "Detected context bound default value Brush.s_Transform (See OS Bug #947272)."); Debug.Assert(s_RelativeTransform == null || s_RelativeTransform.IsFrozen, "Detected context bound default value Brush.s_RelativeTransform (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(Brush); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); TransformProperty = RegisterProperty("Transform", typeof(Transform), typeofThis, Transform.Identity, new PropertyChangedCallback(TransformPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); RelativeTransformProperty = RegisterProperty("RelativeTransform", typeof(Transform), typeofThis, Transform.Identity, new PropertyChangedCallback(RelativeTransformPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
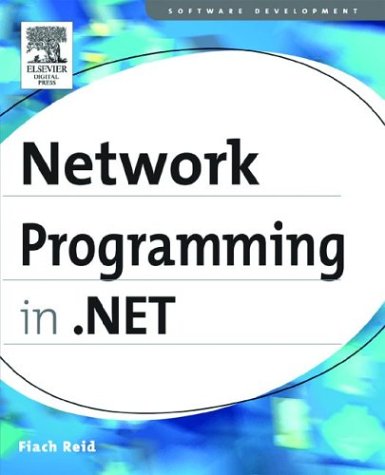
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MeasureData.cs
- PerspectiveCamera.cs
- XmlSchemaSimpleTypeRestriction.cs
- MatrixUtil.cs
- SynchronizingStream.cs
- ObjectStateManager.cs
- Operators.cs
- ZoneButton.cs
- SqlDataReaderSmi.cs
- XmlImplementation.cs
- HandlerFactoryCache.cs
- SqlFacetAttribute.cs
- WrappedIUnknown.cs
- SafeProcessHandle.cs
- DeploymentSection.cs
- Debugger.cs
- DeviceContext.cs
- XmlSchemaAnyAttribute.cs
- TraceEventCache.cs
- WebMessageEncodingBindingElement.cs
- HtmlControlPersistable.cs
- DesignerDataTableBase.cs
- FormViewDeleteEventArgs.cs
- SQLBinaryStorage.cs
- DataGridViewSelectedCellCollection.cs
- ReadOnlyCollection.cs
- Base64Decoder.cs
- Adorner.cs
- BlockCollection.cs
- DBDataPermission.cs
- XmlSchemaAttribute.cs
- LocatorGroup.cs
- DomainUpDown.cs
- OletxTransactionManager.cs
- GACMembershipCondition.cs
- MailWebEventProvider.cs
- LostFocusEventManager.cs
- LineBreakRecord.cs
- GridLengthConverter.cs
- CodeDirectoryCompiler.cs
- PtsHelper.cs
- Slider.cs
- BooleanToVisibilityConverter.cs
- TimestampInformation.cs
- TemplateColumn.cs
- CellTreeNodeVisitors.cs
- TextTreeNode.cs
- TokenBasedSetEnumerator.cs
- ClassicBorderDecorator.cs
- Visual.cs
- ErrorBehavior.cs
- ObjectHelper.cs
- AllowedAudienceUriElement.cs
- DbConnectionOptions.cs
- Wizard.cs
- TextContainerChangedEventArgs.cs
- ListViewSortEventArgs.cs
- Helper.cs
- StateRuntime.cs
- IImplicitResourceProvider.cs
- Mutex.cs
- XmlWellformedWriter.cs
- FileSystemEnumerable.cs
- X509ThumbprintKeyIdentifierClause.cs
- EntityConnectionStringBuilder.cs
- ErrorRuntimeConfig.cs
- ViewStateModeByIdAttribute.cs
- HwndAppCommandInputProvider.cs
- ProfileManager.cs
- ServiceActivationException.cs
- DataControlFieldCollection.cs
- ConnectionManagementSection.cs
- XmlSchemaParticle.cs
- ZipIOLocalFileDataDescriptor.cs
- CertificateElement.cs
- ViewPort3D.cs
- ReservationCollection.cs
- BaseComponentEditor.cs
- SqlCommand.cs
- CqlParser.cs
- SymmetricCryptoHandle.cs
- DataGridClipboardCellContent.cs
- AppSecurityManager.cs
- XsdBuildProvider.cs
- ObjectManager.cs
- SqlFunctions.cs
- FocusWithinProperty.cs
- KeysConverter.cs
- PageThemeBuildProvider.cs
- HttpUnhandledOperationInvoker.cs
- Int64.cs
- AstNode.cs
- ObjectDataSourceFilteringEventArgs.cs
- TextMetrics.cs
- RegistrationServices.cs
- RequestContext.cs
- XmlAttributeAttribute.cs
- LocalizableResourceBuilder.cs
- And.cs
- TableParaClient.cs