Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / IO / Packaging / PackageRelationshipCollection.cs / 1 / PackageRelationshipCollection.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a class for representing a PackageRelationshipCollection. This is a part of the // MMCF Packaging Layer. // // Details: // This class handles serialization to/from relationship parts, creation of those parts // and offers methods to create, delete and enumerate relationships. // // History: // 01/03/2004: SarjanaS: Initial creation. // 03/17/2004: BruceMac: Initial implementation // 04/26/2004: Sarjanas: Refactored some of the code to InternalRelationshipCollection.cs // and added support for filtered relationships // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; // For Exception strings - SRID using System.Collections.Generic; using System.Diagnostics; // For Debug.Assert using MS.Internal.IO.Packaging; namespace System.IO.Packaging { ////// Collection of all the relationships corresponding to a given source PackagePart /// public class PackageRelationshipCollection : IEnumerable{ //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region IEnumerable /// /// Returns an enumerator for all the relationships for a PackagePart /// ///IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// /// Returns an enumerator over all the relationships for a PackagePart /// ///public IEnumerator GetEnumerator() { List .Enumerator relationshipsEnumerator = _relationships.GetEnumerator(); if (_filter == null) return relationshipsEnumerator; else return new FilteredEnumerator(relationshipsEnumerator, _filter); } #endregion //------------------------------------------------------ // // Internal Members // //----------------------------------------------------- #region Internal Members /// /// Constructor /// ///For use by PackagePart internal PackageRelationshipCollection(InternalRelationshipCollection relationships, string filter) { Debug.Assert(relationships != null, "relationships parameter cannot be null"); _relationships = relationships; _filter = filter; } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ // None //----------------------------------------------------- // // Private Members // //------------------------------------------------------ #region Private Members private InternalRelationshipCollection _relationships; private string _filter; #endregion #region Private Class #region FilteredEnumerator Class ////// Internal class for the FilteredEnumerator /// private sealed class FilteredEnumerator : IEnumerator{ #region Constructor /// /// Constructs a FilteredEnumerator /// /// /// internal FilteredEnumerator(IEnumeratorenumerator, string filter) { Debug.Assert((enumerator != null), "Enumerator cannot be null"); Debug.Assert(filter != null, "PackageRelationship filter string cannot be null"); // Look for empty string or string with just spaces Debug.Assert(filter.Trim() != String.Empty, "RelationshipType filter cannot be empty string or a string with just spaces"); _enumerator = enumerator; _filter = filter; } #endregion Constructor #region IEnumerator Methods /// /// This method keeps moving the enumerator the the next position till /// a relationship is found with the matching Name /// ///Bool indicating if the enumerator successfully moved to the next position bool IEnumerator.MoveNext() { while (_enumerator.MoveNext()) { if (RelationshipTypeMatches()) return true; } return false; } ////// Gets the current object in the enumerator /// ///Object IEnumerator.Current { get { return _enumerator.Current; } } /// /// Resets the enumerator to the begining /// void IEnumerator.Reset() { _enumerator.Reset(); } #endregion IEnumerator Methods #region IEnumeratorMembers /// /// Gets the current object in the enumerator /// ///public PackageRelationship Current { get { return _enumerator.Current; } } #endregion IEnumerator Members #region IDisposable Members public void Dispose() { //Most enumerators have dispose as a no-op, we follow the same pattern. _enumerator.Dispose(); } #endregion IDisposable Members #region Private Methods private bool RelationshipTypeMatches() { PackageRelationship r = _enumerator.Current; //Case-sensitive comparison if (String.CompareOrdinal(r.RelationshipType, _filter)==0) return true; else return false; } #endregion Private Methods #region Private Members private IEnumerator _enumerator; private string _filter; #endregion Private Members } #endregion FilteredEnumerator Class #endregion Private Class } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a class for representing a PackageRelationshipCollection. This is a part of the // MMCF Packaging Layer. // // Details: // This class handles serialization to/from relationship parts, creation of those parts // and offers methods to create, delete and enumerate relationships. // // History: // 01/03/2004: SarjanaS: Initial creation. // 03/17/2004: BruceMac: Initial implementation // 04/26/2004: Sarjanas: Refactored some of the code to InternalRelationshipCollection.cs // and added support for filtered relationships // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; // For Exception strings - SRID using System.Collections.Generic; using System.Diagnostics; // For Debug.Assert using MS.Internal.IO.Packaging; namespace System.IO.Packaging { ////// Collection of all the relationships corresponding to a given source PackagePart /// public class PackageRelationshipCollection : IEnumerable{ //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region IEnumerable /// /// Returns an enumerator for all the relationships for a PackagePart /// ///IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// /// Returns an enumerator over all the relationships for a PackagePart /// ///public IEnumerator GetEnumerator() { List .Enumerator relationshipsEnumerator = _relationships.GetEnumerator(); if (_filter == null) return relationshipsEnumerator; else return new FilteredEnumerator(relationshipsEnumerator, _filter); } #endregion //------------------------------------------------------ // // Internal Members // //----------------------------------------------------- #region Internal Members /// /// Constructor /// ///For use by PackagePart internal PackageRelationshipCollection(InternalRelationshipCollection relationships, string filter) { Debug.Assert(relationships != null, "relationships parameter cannot be null"); _relationships = relationships; _filter = filter; } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ // None //----------------------------------------------------- // // Private Members // //------------------------------------------------------ #region Private Members private InternalRelationshipCollection _relationships; private string _filter; #endregion #region Private Class #region FilteredEnumerator Class ////// Internal class for the FilteredEnumerator /// private sealed class FilteredEnumerator : IEnumerator{ #region Constructor /// /// Constructs a FilteredEnumerator /// /// /// internal FilteredEnumerator(IEnumeratorenumerator, string filter) { Debug.Assert((enumerator != null), "Enumerator cannot be null"); Debug.Assert(filter != null, "PackageRelationship filter string cannot be null"); // Look for empty string or string with just spaces Debug.Assert(filter.Trim() != String.Empty, "RelationshipType filter cannot be empty string or a string with just spaces"); _enumerator = enumerator; _filter = filter; } #endregion Constructor #region IEnumerator Methods /// /// This method keeps moving the enumerator the the next position till /// a relationship is found with the matching Name /// ///Bool indicating if the enumerator successfully moved to the next position bool IEnumerator.MoveNext() { while (_enumerator.MoveNext()) { if (RelationshipTypeMatches()) return true; } return false; } ////// Gets the current object in the enumerator /// ///Object IEnumerator.Current { get { return _enumerator.Current; } } /// /// Resets the enumerator to the begining /// void IEnumerator.Reset() { _enumerator.Reset(); } #endregion IEnumerator Methods #region IEnumeratorMembers /// /// Gets the current object in the enumerator /// ///public PackageRelationship Current { get { return _enumerator.Current; } } #endregion IEnumerator Members #region IDisposable Members public void Dispose() { //Most enumerators have dispose as a no-op, we follow the same pattern. _enumerator.Dispose(); } #endregion IDisposable Members #region Private Methods private bool RelationshipTypeMatches() { PackageRelationship r = _enumerator.Current; //Case-sensitive comparison if (String.CompareOrdinal(r.RelationshipType, _filter)==0) return true; else return false; } #endregion Private Methods #region Private Members private IEnumerator _enumerator; private string _filter; #endregion Private Members } #endregion FilteredEnumerator Class #endregion Private Class } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
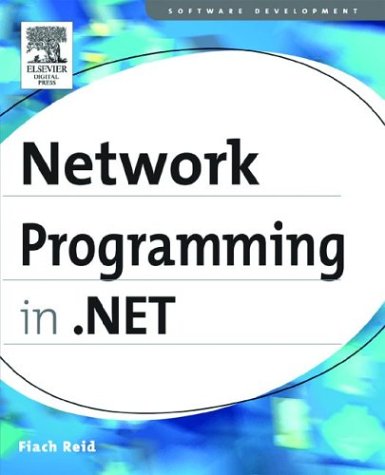
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MediaSystem.cs
- XmlSerializationGeneratedCode.cs
- Rect3D.cs
- FlagsAttribute.cs
- BoundField.cs
- EventSinkHelperWriter.cs
- BackgroundFormatInfo.cs
- TreePrinter.cs
- BitmapData.cs
- XmlSchemaAnnotated.cs
- PopOutPanel.cs
- TypeDescriptorFilterService.cs
- UrlMappingCollection.cs
- XsltSettings.cs
- UdpTransportSettingsElement.cs
- ContextMenuAutomationPeer.cs
- Package.cs
- DataObjectEventArgs.cs
- XmlSchemaChoice.cs
- UtilityExtension.cs
- Identifier.cs
- ACE.cs
- AbsoluteQuery.cs
- BatchParser.cs
- FastEncoder.cs
- SqlDataAdapter.cs
- XmlDataCollection.cs
- TableLayoutPanel.cs
- FormViewActionList.cs
- GeometryValueSerializer.cs
- DataGridRowHeaderAutomationPeer.cs
- SpeechUI.cs
- PasswordBoxAutomationPeer.cs
- PreApplicationStartMethodAttribute.cs
- EmptyReadOnlyDictionaryInternal.cs
- ServiceConfigurationTraceRecord.cs
- SchemaCreator.cs
- ProtocolReflector.cs
- SuppressedPackageProperties.cs
- OrderedDictionary.cs
- DataPagerCommandEventArgs.cs
- StringValidatorAttribute.cs
- ResourceAssociationSetEnd.cs
- WindowsSlider.cs
- SkinIDTypeConverter.cs
- Color.cs
- Int64Animation.cs
- CngProperty.cs
- Freezable.cs
- UserNameSecurityTokenAuthenticator.cs
- HandleRef.cs
- ExpressionVisitorHelpers.cs
- CryptoApi.cs
- ProtocolException.cs
- CompatibleComparer.cs
- Point4DValueSerializer.cs
- _CacheStreams.cs
- GZipDecoder.cs
- XmlArrayAttribute.cs
- ObjectSet.cs
- SecurityPermission.cs
- Zone.cs
- ExtractorMetadata.cs
- CompressStream.cs
- MimePart.cs
- DataGridBoundColumn.cs
- XamlPoint3DCollectionSerializer.cs
- Size3DConverter.cs
- ButtonBase.cs
- TextSearch.cs
- DateTimeOffsetAdapter.cs
- EventHandlersStore.cs
- Vector3dCollection.cs
- SourceFileBuildProvider.cs
- Crc32.cs
- _HeaderInfo.cs
- EpmContentSerializerBase.cs
- SuppressMessageAttribute.cs
- OracleDataAdapter.cs
- AsyncCompletedEventArgs.cs
- _BaseOverlappedAsyncResult.cs
- TablePatternIdentifiers.cs
- recordstatescratchpad.cs
- ObsoleteAttribute.cs
- Regex.cs
- PropVariant.cs
- PointUtil.cs
- WebResourceUtil.cs
- MetadataAssemblyHelper.cs
- externdll.cs
- SchemaImporterExtension.cs
- EventPropertyMap.cs
- documentsequencetextpointer.cs
- HitTestWithGeometryDrawingContextWalker.cs
- PermissionToken.cs
- ToolZone.cs
- ResizeGrip.cs
- StrongNameIdentityPermission.cs
- Crc32.cs
- UpdateCommandGenerator.cs