Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / DataGridBoundColumn.cs / 1305600 / DataGridBoundColumn.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.ObjectModel; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Data; using System.Windows.Input; namespace System.Windows.Controls { ////// A base class for specifying column definitions for certain standard /// types that do not allow arbitrary templates. /// public abstract class DataGridBoundColumn : DataGridColumn { #region Constructors static DataGridBoundColumn() { SortMemberPathProperty.OverrideMetadata(typeof(DataGridBoundColumn), new FrameworkPropertyMetadata(null, OnCoerceSortMemberPath)); } #endregion #region Binding private static object OnCoerceSortMemberPath(DependencyObject d, object baseValue) { var column = (DataGridBoundColumn)d; var sortMemberPath = (string)baseValue; if (string.IsNullOrEmpty(sortMemberPath)) { var bindingSortMemberPath = DataGridHelper.GetPathFromBinding(column.Binding as Binding); if (!string.IsNullOrEmpty(bindingSortMemberPath)) { sortMemberPath = bindingSortMemberPath; } } return sortMemberPath; } ////// The binding that will be applied to the generated element. /// ////// This isn't a DP because if it were getting the value would evaluate the binding. /// public virtual BindingBase Binding { get { return _binding; } set { if (_binding != value) { BindingBase oldBinding = _binding; _binding = value; CoerceValue(IsReadOnlyProperty); CoerceValue(SortMemberPathProperty); OnBindingChanged(oldBinding, _binding); } } } protected override bool OnCoerceIsReadOnly(bool baseValue) { if (DataGridHelper.IsOneWay(_binding)) { return true; } // only call the base if we dont want to force IsReadOnly true return base.OnCoerceIsReadOnly(baseValue); } ////// Called when Binding changes. /// ////// Default implementation notifies the DataGrid and its subtree about the change. /// /// The old binding. /// The new binding. protected virtual void OnBindingChanged(BindingBase oldBinding, BindingBase newBinding) { NotifyPropertyChanged("Binding"); } ////// Assigns the Binding to the desired property on the target object. /// internal void ApplyBinding(DependencyObject target, DependencyProperty property) { BindingBase binding = Binding; if (binding != null) { BindingOperations.SetBinding(target, property, binding); } else { BindingOperations.ClearBinding(target, property); } } #endregion #region Styling ////// A style that is applied to the generated element when not editing. /// The TargetType of the style depends on the derived column class. /// public Style ElementStyle { get { return (Style)GetValue(ElementStyleProperty); } set { SetValue(ElementStyleProperty, value); } } ////// The DependencyProperty for the ElementStyle property. /// public static readonly DependencyProperty ElementStyleProperty = DependencyProperty.Register( "ElementStyle", typeof(Style), typeof(DataGridBoundColumn), new FrameworkPropertyMetadata(null, new PropertyChangedCallback(DataGridColumn.NotifyPropertyChangeForRefreshContent))); ////// A style that is applied to the generated element when editing. /// The TargetType of the style depends on the derived column class. /// public Style EditingElementStyle { get { return (Style)GetValue(EditingElementStyleProperty); } set { SetValue(EditingElementStyleProperty, value); } } ////// The DependencyProperty for the EditingElementStyle property. /// public static readonly DependencyProperty EditingElementStyleProperty = DependencyProperty.Register( "EditingElementStyle", typeof(Style), typeof(DataGridBoundColumn), new FrameworkPropertyMetadata(null, new PropertyChangedCallback(DataGridColumn.NotifyPropertyChangeForRefreshContent))); ////// Assigns the ElementStyle to the desired property on the given element. /// internal void ApplyStyle(bool isEditing, bool defaultToElementStyle, FrameworkElement element) { Style style = PickStyle(isEditing, defaultToElementStyle); if (style != null) { element.Style = style; } } private Style PickStyle(bool isEditing, bool defaultToElementStyle) { Style style = isEditing ? EditingElementStyle : ElementStyle; if (isEditing && defaultToElementStyle && (style == null)) { style = ElementStyle; } return style; } #endregion #region Clipboard Copy/Paste ////// If base ClipboardContentBinding is not set we use Binding. /// public override BindingBase ClipboardContentBinding { get { return base.ClipboardContentBinding ?? Binding; } set { base.ClipboardContentBinding = value; } } #endregion #region Property Changed Handler ////// Override which rebuilds the cell's visual tree for Binding change /// /// /// protected internal override void RefreshCellContent(FrameworkElement element, string propertyName) { DataGridCell cell = element as DataGridCell; if (cell != null) { bool isCellEditing = cell.IsEditing; if ((string.Compare(propertyName, "Binding", StringComparison.Ordinal) == 0) || (string.Compare(propertyName, "ElementStyle", StringComparison.Ordinal) == 0 && !isCellEditing) || (string.Compare(propertyName, "EditingElementStyle", StringComparison.Ordinal) == 0 && isCellEditing)) { cell.BuildVisualTree(); return; } } base.RefreshCellContent(element, propertyName); } #endregion #region Data private BindingBase _binding; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
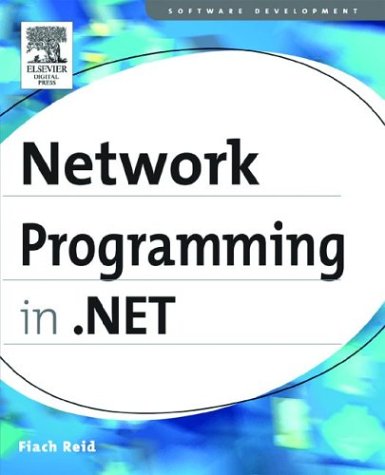
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListDesigner.cs
- FontEmbeddingManager.cs
- DesignerVerbCollection.cs
- VScrollBar.cs
- XmlBinaryWriterSession.cs
- ArithmeticException.cs
- DataContractSerializerSection.cs
- ProcessHost.cs
- WebException.cs
- ParsedAttributeCollection.cs
- SynchronizedInputProviderWrapper.cs
- Point3DCollection.cs
- COM2PictureConverter.cs
- SocketPermission.cs
- FormsAuthentication.cs
- PiiTraceSource.cs
- SchemaUtility.cs
- DataGridViewCellEventArgs.cs
- SQLResource.cs
- listitem.cs
- CodeExpressionCollection.cs
- MoveSizeWinEventHandler.cs
- DBDataPermissionAttribute.cs
- DoubleAnimationUsingPath.cs
- DoubleConverter.cs
- XmlDataSourceDesigner.cs
- ClientSideProviderDescription.cs
- WebPartHelpVerb.cs
- ComplexType.cs
- _DigestClient.cs
- TrackBar.cs
- IndexedWhereQueryOperator.cs
- QilValidationVisitor.cs
- EmbeddedMailObject.cs
- BamlLocalizableResourceKey.cs
- TreeNodeCollection.cs
- PointF.cs
- WebBrowserBase.cs
- CopyNamespacesAction.cs
- InArgumentConverter.cs
- CompositeCollectionView.cs
- TextReader.cs
- TypeElement.cs
- Propagator.ExtentPlaceholderCreator.cs
- CharacterBuffer.cs
- SqlDataSourceParameterParser.cs
- HtmlValidationSummaryAdapter.cs
- SqlBuilder.cs
- XmlHierarchicalEnumerable.cs
- ObjectHandle.cs
- ComplexLine.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ThrowOnMultipleAssignment.cs
- ValidatorUtils.cs
- HtmlInputImage.cs
- TrackingLocation.cs
- TabControl.cs
- PersonalizationProviderCollection.cs
- StatusStrip.cs
- StreamUpdate.cs
- DataServiceBehavior.cs
- CancellationTokenRegistration.cs
- HttpModulesSection.cs
- SpellerError.cs
- LineServicesCallbacks.cs
- DbConnectionStringCommon.cs
- SplashScreenNativeMethods.cs
- SQLBinaryStorage.cs
- DesignerActionListCollection.cs
- XmlnsDictionary.cs
- TaskExtensions.cs
- Compiler.cs
- OleDbRowUpdatedEvent.cs
- HwndProxyElementProvider.cs
- VirtualPathUtility.cs
- RadioButton.cs
- HostedTransportConfigurationBase.cs
- infer.cs
- TreeNodeCollection.cs
- MasterPageParser.cs
- ControllableStoryboardAction.cs
- MonthChangedEventArgs.cs
- TiffBitmapDecoder.cs
- SafeEventHandle.cs
- ColorBlend.cs
- ProcessThread.cs
- RuntimeConfigurationRecord.cs
- TextServicesLoader.cs
- DbException.cs
- CompilerScopeManager.cs
- CLRBindingWorker.cs
- SecureEnvironment.cs
- NavigationExpr.cs
- DefaultAssemblyResolver.cs
- DeviceOverridableAttribute.cs
- LinkGrep.cs
- ConnectionsZone.cs
- SQLInt16Storage.cs
- KnownTypesHelper.cs
- LiteralText.cs