Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Objects / ObjectViewEntityCollectionData.cs / 1 / ObjectViewEntityCollectionData.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner jhutson // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Data.Objects.DataClasses; using System.Diagnostics; namespace System.Data.Objects { ////// Manages a binding list constructed from an EntityCollection. /// ////// Type of the elements in the binding list. /// ////// Type of element in the underlying EntityCollection. /// ////// The binding list is initialized from the EntityCollection, /// and is synchronized with changes made to the EntityCollection membership. /// This class always allows additions and removals from the binding list. /// internal sealed class ObjectViewEntityCollectionData: IObjectViewData where TItemElement : class, IEntityWithRelationships where TViewElement : TItemElement { private List _bindingList; private EntityCollection _entityCollection; private readonly bool _canEditItems; /// /// True if item that was added to binding list but not underlying entity collection /// is now being committed to the collection. /// Otherwise false. /// Used by CommitItemAt and OnCollectionChanged methods to coordinate addition /// of new item to underlying entity collection. /// private bool _itemCommitPending; ////// Construct a new instance of the ObjectViewEntityCollectionData class using the supplied entityCollection. /// /// /// EntityCollection used to populate the binding list. /// internal ObjectViewEntityCollectionData(EntityCollectionentityCollection) { _entityCollection = entityCollection; _canEditItems = typeof(IEntityWithChangeTracker).IsAssignableFrom(typeof(TViewElement)); _bindingList = new List (entityCollection.Count); foreach (TViewElement entity in entityCollection) { _bindingList.Add(entity); } } #region IObjectViewData Members public IList List { get { return _bindingList; } } public bool AllowNew { get { return !_entityCollection.IsReadOnly; } } public bool AllowEdit { get { return _canEditItems; } } public bool AllowRemove { get { return !_entityCollection.IsReadOnly; } } public bool FiresEventOnAdd { get { return true; } } public bool FiresEventOnRemove { get { return true; } } public bool FiresEventOnClear { get { return true; } } public void EnsureCanAddNew() { // nop } public int Add(TViewElement item, bool isAddNew) { if (isAddNew) { // Item is added to bindingList, but pending addition to entity collection. _bindingList.Add(item); } else { _entityCollection.Add(item); // OnCollectionChanged will be fired, where the binding list will be updated. } return _bindingList.Count - 1; } public void CommitItemAt(int index) { TViewElement item = _bindingList[index]; try { _itemCommitPending = true; _entityCollection.Add(item); // OnCollectionChanged will be fired, where the binding list will be updated. } finally { _itemCommitPending = false; } } public void Clear() { if (0 < _bindingList.Count) { List _deletionList = new List (); foreach (object item in _bindingList) { _deletionList.Add(item as IEntityWithRelationships); } _entityCollection.BulkDeleteAll(_deletionList); // EntityCollection will fire change event which this instance will use to clean up the binding list. } } public bool Remove(TViewElement item, bool isCancelNew) { bool removed; if (isCancelNew) { // Item was previously added to binding list, but not entity collection. removed = _bindingList.Remove(item); } else { removed = _entityCollection.Remove(item); // OnCollectionChanged will be fired, where the binding list will be updated. } return removed; } public ListChangedEventArgs OnCollectionChanged(object sender, CollectionChangeEventArgs e, ObjectViewListener listener) { ListChangedEventArgs changeArgs = null; switch (e.Action) { case CollectionChangeAction.Remove: // An Entity is being removed from entity collection, remove it from list. if (e.Element is TViewElement) { TViewElement removedItem = (TViewElement)e.Element; int oldIndex = _bindingList.IndexOf(removedItem); if (oldIndex != -1) { _bindingList.Remove(removedItem); // Unhook from events of removed entity. listener.UnregisterEntityEvents(removedItem); changeArgs = new ListChangedEventArgs(ListChangedType.ItemDeleted, oldIndex /* newIndex*/, -1 /* oldIndex*/); } } break; case CollectionChangeAction.Add: // Add the entity to our list. if (e.Element is TViewElement) { // Do not process Add events that fire as a result of committing an item to the entity collection. if (!_itemCommitPending) { TViewElement addedItem = (TViewElement)e.Element; _bindingList.Add(addedItem); // Register to its events. listener.RegisterEntityEvents(addedItem); changeArgs = new ListChangedEventArgs(ListChangedType.ItemAdded, _bindingList.Count - 1 /* newIndex*/, -1 /* oldIndex*/); } } break; case CollectionChangeAction.Refresh: foreach (TViewElement entity in _bindingList) { Debug.Assert(entity is IEntityWithChangeTracker, "entity must be type IEntityWithChangeTracker"); listener.UnregisterEntityEvents(entity); } _bindingList.Clear(); foreach (TViewElement entity in _entityCollection) { _bindingList.Add(entity); Debug.Assert(entity is IEntityWithChangeTracker, "entity must be type IEntityWithChangeTracker"); listener.RegisterEntityEvents(entity); } changeArgs = new ListChangedEventArgs(ListChangedType.Reset, -1 /*newIndex*/, -1/*oldIndex*/); break; } return changeArgs; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner jhutson // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Data.Objects.DataClasses; using System.Diagnostics; namespace System.Data.Objects { ////// Manages a binding list constructed from an EntityCollection. /// ////// Type of the elements in the binding list. /// ////// Type of element in the underlying EntityCollection. /// ////// The binding list is initialized from the EntityCollection, /// and is synchronized with changes made to the EntityCollection membership. /// This class always allows additions and removals from the binding list. /// internal sealed class ObjectViewEntityCollectionData: IObjectViewData where TItemElement : class, IEntityWithRelationships where TViewElement : TItemElement { private List _bindingList; private EntityCollection _entityCollection; private readonly bool _canEditItems; /// /// True if item that was added to binding list but not underlying entity collection /// is now being committed to the collection. /// Otherwise false. /// Used by CommitItemAt and OnCollectionChanged methods to coordinate addition /// of new item to underlying entity collection. /// private bool _itemCommitPending; ////// Construct a new instance of the ObjectViewEntityCollectionData class using the supplied entityCollection. /// /// /// EntityCollection used to populate the binding list. /// internal ObjectViewEntityCollectionData(EntityCollectionentityCollection) { _entityCollection = entityCollection; _canEditItems = typeof(IEntityWithChangeTracker).IsAssignableFrom(typeof(TViewElement)); _bindingList = new List (entityCollection.Count); foreach (TViewElement entity in entityCollection) { _bindingList.Add(entity); } } #region IObjectViewData Members public IList List { get { return _bindingList; } } public bool AllowNew { get { return !_entityCollection.IsReadOnly; } } public bool AllowEdit { get { return _canEditItems; } } public bool AllowRemove { get { return !_entityCollection.IsReadOnly; } } public bool FiresEventOnAdd { get { return true; } } public bool FiresEventOnRemove { get { return true; } } public bool FiresEventOnClear { get { return true; } } public void EnsureCanAddNew() { // nop } public int Add(TViewElement item, bool isAddNew) { if (isAddNew) { // Item is added to bindingList, but pending addition to entity collection. _bindingList.Add(item); } else { _entityCollection.Add(item); // OnCollectionChanged will be fired, where the binding list will be updated. } return _bindingList.Count - 1; } public void CommitItemAt(int index) { TViewElement item = _bindingList[index]; try { _itemCommitPending = true; _entityCollection.Add(item); // OnCollectionChanged will be fired, where the binding list will be updated. } finally { _itemCommitPending = false; } } public void Clear() { if (0 < _bindingList.Count) { List _deletionList = new List (); foreach (object item in _bindingList) { _deletionList.Add(item as IEntityWithRelationships); } _entityCollection.BulkDeleteAll(_deletionList); // EntityCollection will fire change event which this instance will use to clean up the binding list. } } public bool Remove(TViewElement item, bool isCancelNew) { bool removed; if (isCancelNew) { // Item was previously added to binding list, but not entity collection. removed = _bindingList.Remove(item); } else { removed = _entityCollection.Remove(item); // OnCollectionChanged will be fired, where the binding list will be updated. } return removed; } public ListChangedEventArgs OnCollectionChanged(object sender, CollectionChangeEventArgs e, ObjectViewListener listener) { ListChangedEventArgs changeArgs = null; switch (e.Action) { case CollectionChangeAction.Remove: // An Entity is being removed from entity collection, remove it from list. if (e.Element is TViewElement) { TViewElement removedItem = (TViewElement)e.Element; int oldIndex = _bindingList.IndexOf(removedItem); if (oldIndex != -1) { _bindingList.Remove(removedItem); // Unhook from events of removed entity. listener.UnregisterEntityEvents(removedItem); changeArgs = new ListChangedEventArgs(ListChangedType.ItemDeleted, oldIndex /* newIndex*/, -1 /* oldIndex*/); } } break; case CollectionChangeAction.Add: // Add the entity to our list. if (e.Element is TViewElement) { // Do not process Add events that fire as a result of committing an item to the entity collection. if (!_itemCommitPending) { TViewElement addedItem = (TViewElement)e.Element; _bindingList.Add(addedItem); // Register to its events. listener.RegisterEntityEvents(addedItem); changeArgs = new ListChangedEventArgs(ListChangedType.ItemAdded, _bindingList.Count - 1 /* newIndex*/, -1 /* oldIndex*/); } } break; case CollectionChangeAction.Refresh: foreach (TViewElement entity in _bindingList) { Debug.Assert(entity is IEntityWithChangeTracker, "entity must be type IEntityWithChangeTracker"); listener.UnregisterEntityEvents(entity); } _bindingList.Clear(); foreach (TViewElement entity in _entityCollection) { _bindingList.Add(entity); Debug.Assert(entity is IEntityWithChangeTracker, "entity must be type IEntityWithChangeTracker"); listener.RegisterEntityEvents(entity); } changeArgs = new ListChangedEventArgs(ListChangedType.Reset, -1 /*newIndex*/, -1/*oldIndex*/); break; } return changeArgs; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
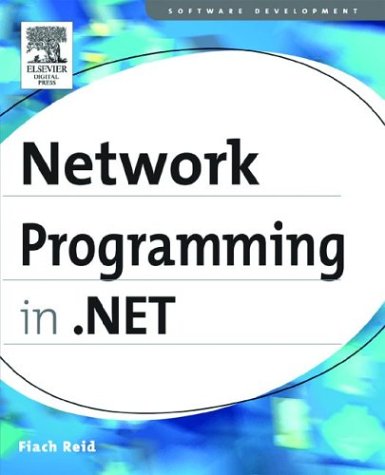
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventRoute.cs
- SettingsPropertyIsReadOnlyException.cs
- MonthChangedEventArgs.cs
- InheritedPropertyChangedEventArgs.cs
- RegistryKey.cs
- ActivityInfo.cs
- AsyncPostBackErrorEventArgs.cs
- SrgsText.cs
- MasterPageCodeDomTreeGenerator.cs
- ClientSettingsProvider.cs
- TimeEnumHelper.cs
- FormClosedEvent.cs
- UniqueID.cs
- PageThemeBuildProvider.cs
- StringComparer.cs
- SchemaCollectionCompiler.cs
- DocumentEventArgs.cs
- VirtualPath.cs
- UnaryNode.cs
- MeshGeometry3D.cs
- PathNode.cs
- Privilege.cs
- ViewgenGatekeeper.cs
- ExtendedPropertyCollection.cs
- OleDbWrapper.cs
- MulticastDelegate.cs
- TypeLoadException.cs
- OrderByBuilder.cs
- ProfileBuildProvider.cs
- PiiTraceSource.cs
- ConfigurationManagerHelper.cs
- DataControlImageButton.cs
- AdapterDictionary.cs
- TypedElement.cs
- RemoteWebConfigurationHostStream.cs
- AddToCollection.cs
- ExpanderAutomationPeer.cs
- PnrpPeerResolverElement.cs
- TimeSpanSecondsConverter.cs
- TableLayoutStyle.cs
- DockAndAnchorLayout.cs
- EditingCoordinator.cs
- WebPartConnectionCollection.cs
- TextEditorMouse.cs
- StringFunctions.cs
- XmlDataProvider.cs
- Geometry.cs
- HtmlInputText.cs
- JpegBitmapDecoder.cs
- TriState.cs
- DataGridColumn.cs
- PropertiesTab.cs
- SpellCheck.cs
- DesignerActionVerbItem.cs
- FileDialog_Vista.cs
- CodeCommentStatement.cs
- WebPartTracker.cs
- CryptoConfig.cs
- LinqDataSourceSelectEventArgs.cs
- DrawingAttributesDefaultValueFactory.cs
- Logging.cs
- TraceRecord.cs
- SqlProvider.cs
- RegisteredExpandoAttribute.cs
- LineBreak.cs
- PageClientProxyGenerator.cs
- StopStoryboard.cs
- PersianCalendar.cs
- UdpTransportSettings.cs
- DataGridComboBoxColumn.cs
- ZipFileInfo.cs
- ConcurrentDictionary.cs
- ActivityDesignerResources.cs
- Stylesheet.cs
- TemplatePartAttribute.cs
- GlyphRunDrawing.cs
- ItemList.cs
- BitStack.cs
- SubpageParagraph.cs
- TypeConverter.cs
- SendOperation.cs
- coordinatorscratchpad.cs
- FontNameConverter.cs
- WindowsFont.cs
- AdornerDecorator.cs
- PersonalizationAdministration.cs
- UDPClient.cs
- BuildProviderInstallComponent.cs
- MinMaxParagraphWidth.cs
- Serializer.cs
- CheckBoxField.cs
- XmlSchemaException.cs
- SearchForVirtualItemEventArgs.cs
- CredentialCache.cs
- HuffCodec.cs
- SHA1CryptoServiceProvider.cs
- DisplayInformation.cs
- XmlDataSourceNodeDescriptor.cs
- SafeNativeMethods.cs
- DrawingVisual.cs