Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartConnectionCollection.cs / 2 / WebPartConnectionCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Globalization; using System.Security.Permissions; using System.Web.Util; // [ Editor("System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartConnectionCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; private WebPartManager _webPartManager; internal WebPartConnectionCollection(WebPartManager webPartManager) { _webPartManager = webPartManager; } public bool IsReadOnly { get { return _readOnly; } } ////// Returns the WebPartConnection at a given index. /// public WebPartConnection this[int index] { get { return (WebPartConnection)List[index]; } set { List[index] = value; } } ////// Returns the WebPartConnection with the specified id, performing a case-insensitive comparison. /// Returns null if there are no matches. /// public WebPartConnection this[string id] { // PERF: Use a hashtable for lookup, instead of a linear search get { foreach (WebPartConnection connection in List) { if (String.Equals(connection.ID, id, StringComparison.OrdinalIgnoreCase)) { return connection; } } return null; } } ////// Adds a Connection to the collection. /// public int Add(WebPartConnection value) { return List.Add(value); } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } ////// True if the WebPartConnection is contained in the collection. /// public bool Contains(WebPartConnection value) { return List.Contains(value); } internal bool ContainsProvider(WebPart provider) { foreach (WebPartConnection connection in List) { if (connection.Provider == provider) { return true; } } return false; } public void CopyTo(WebPartConnection[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartConnection value) { return List.IndexOf(value); } ////// Inserts a WebPartConnection into the collection. /// public void Insert(int index, WebPartConnection value) { List.Insert(index, value); } ////// protected override void OnClear() { CheckReadOnly(); base.OnClear(); } protected override void OnInsert(int index, object value) { CheckReadOnly(); ((WebPartConnection)value).SetWebPartManager(_webPartManager); base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { CheckReadOnly(); ((WebPartConnection)value).SetWebPartManager(null); base.OnRemove(index, value); } protected override void OnSet(int index, object oldValue, object newValue) { CheckReadOnly(); ((WebPartConnection)oldValue).SetWebPartManager(null); ((WebPartConnection)newValue).SetWebPartManager(_webPartManager); base.OnSet(index, oldValue, newValue); } ////// Validates that an object is a WebPartConnection. /// protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartConnection)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartConnection"), "value"); } } ////// Removes a WebPartConnection from the collection. /// public void Remove(WebPartConnection value) { List.Remove(value); } ////// Marks the collection readonly. This is useful, because we assume that the list /// of static connections is known at Init time, and new connections are added /// through the WebPartManager. /// internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Globalization; using System.Security.Permissions; using System.Web.Util; // [ Editor("System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartConnectionCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; private WebPartManager _webPartManager; internal WebPartConnectionCollection(WebPartManager webPartManager) { _webPartManager = webPartManager; } public bool IsReadOnly { get { return _readOnly; } } ////// Returns the WebPartConnection at a given index. /// public WebPartConnection this[int index] { get { return (WebPartConnection)List[index]; } set { List[index] = value; } } ////// Returns the WebPartConnection with the specified id, performing a case-insensitive comparison. /// Returns null if there are no matches. /// public WebPartConnection this[string id] { // PERF: Use a hashtable for lookup, instead of a linear search get { foreach (WebPartConnection connection in List) { if (String.Equals(connection.ID, id, StringComparison.OrdinalIgnoreCase)) { return connection; } } return null; } } ////// Adds a Connection to the collection. /// public int Add(WebPartConnection value) { return List.Add(value); } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } ////// True if the WebPartConnection is contained in the collection. /// public bool Contains(WebPartConnection value) { return List.Contains(value); } internal bool ContainsProvider(WebPart provider) { foreach (WebPartConnection connection in List) { if (connection.Provider == provider) { return true; } } return false; } public void CopyTo(WebPartConnection[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartConnection value) { return List.IndexOf(value); } ////// Inserts a WebPartConnection into the collection. /// public void Insert(int index, WebPartConnection value) { List.Insert(index, value); } ////// protected override void OnClear() { CheckReadOnly(); base.OnClear(); } protected override void OnInsert(int index, object value) { CheckReadOnly(); ((WebPartConnection)value).SetWebPartManager(_webPartManager); base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { CheckReadOnly(); ((WebPartConnection)value).SetWebPartManager(null); base.OnRemove(index, value); } protected override void OnSet(int index, object oldValue, object newValue) { CheckReadOnly(); ((WebPartConnection)oldValue).SetWebPartManager(null); ((WebPartConnection)newValue).SetWebPartManager(_webPartManager); base.OnSet(index, oldValue, newValue); } ////// Validates that an object is a WebPartConnection. /// protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartConnection)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartConnection"), "value"); } } ////// Removes a WebPartConnection from the collection. /// public void Remove(WebPartConnection value) { List.Remove(value); } ////// Marks the collection readonly. This is useful, because we assume that the list /// of static connections is known at Init time, and new connections are added /// through the WebPartManager. /// internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
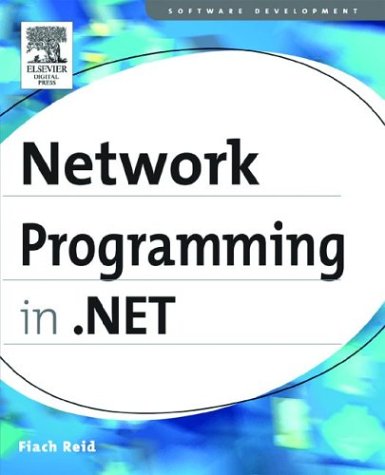
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BackgroundWorker.cs
- TouchesOverProperty.cs
- StringExpressionSet.cs
- PeerTransportListenAddressConverter.cs
- TransformConverter.cs
- __Filters.cs
- ReliabilityContractAttribute.cs
- Event.cs
- FileInfo.cs
- RegexCaptureCollection.cs
- DiscoveryViaBehavior.cs
- WebPartDescriptionCollection.cs
- ConstantExpression.cs
- NativeObjectSecurity.cs
- SoapEnvelopeProcessingElement.cs
- Filter.cs
- ConstantExpression.cs
- ResourcePermissionBaseEntry.cs
- DesignBindingPicker.cs
- DeviceContext.cs
- InvalidEnumArgumentException.cs
- PackWebResponse.cs
- RawAppCommandInputReport.cs
- EntitySqlQueryCacheKey.cs
- EventMappingSettings.cs
- TextElementEnumerator.cs
- Int16.cs
- metadatamappinghashervisitor.cs
- MD5CryptoServiceProvider.cs
- EqualityComparer.cs
- MatchAllMessageFilter.cs
- HtmlInputReset.cs
- StructuredTypeInfo.cs
- DocumentsTrace.cs
- SymmetricAlgorithm.cs
- DataSourceXmlSerializationAttribute.cs
- RuntimeHandles.cs
- SystemUdpStatistics.cs
- SafeNativeMethods.cs
- DataRowView.cs
- WhitespaceRule.cs
- CmsInterop.cs
- UnmanagedHandle.cs
- PaperSize.cs
- Overlapped.cs
- BamlRecordWriter.cs
- ColumnCollection.cs
- DeleteWorkflowOwnerCommand.cs
- CancellationToken.cs
- hwndwrapper.cs
- LinkClickEvent.cs
- ExpressionLexer.cs
- EventManager.cs
- TdsParserStateObject.cs
- UserControlAutomationPeer.cs
- MeasurementDCInfo.cs
- SimpleWebHandlerParser.cs
- MetafileHeaderWmf.cs
- TransactionManager.cs
- EDesignUtil.cs
- SimpleRecyclingCache.cs
- XsltContext.cs
- processwaithandle.cs
- X509Certificate.cs
- PublisherMembershipCondition.cs
- GACMembershipCondition.cs
- InternalTypeHelper.cs
- OleDbErrorCollection.cs
- WebPartEditorApplyVerb.cs
- DetailsView.cs
- DirectoryInfo.cs
- ProjectionCamera.cs
- Compilation.cs
- PassportPrincipal.cs
- Empty.cs
- DataGridViewRowConverter.cs
- DependencyPropertyChangedEventArgs.cs
- JsonFaultDetail.cs
- ReadOnlyCollection.cs
- PowerStatus.cs
- EdmSchemaAttribute.cs
- XmlNodeComparer.cs
- CodeDOMUtility.cs
- CompiledXpathExpr.cs
- DbConnectionOptions.cs
- GroupByExpressionRewriter.cs
- SettingsPropertyValue.cs
- RIPEMD160Managed.cs
- Missing.cs
- StringUtil.cs
- ModulesEntry.cs
- CapiHashAlgorithm.cs
- PropertyCondition.cs
- PropertyChangeTracker.cs
- BrushMappingModeValidation.cs
- WebHttpSecurityElement.cs
- WSHttpBinding.cs
- ChildTable.cs
- ManagementOptions.cs
- Material.cs