Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / Facet.cs / 1 / Facet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Class for representing a Facet object /// This object is Immutable (not just set to readonly) and /// some parts of the system are depending on that behavior /// [DebuggerDisplay("{Name,nq}={Value}")] public sealed class Facet : MetadataItem { #region Constructors ////// The constructor for constructing a Facet object with the facet description and a value /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null private Facet(FacetDescription facetDescription, object value) :base(MetadataFlags.Readonly) { EntityUtil.GenericCheckArgumentNull(facetDescription, "facetDescription"); _facetDescription = facetDescription; _value = value; } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value) { return Create(facetDescription, value, false); } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet /// true to bypass caching and known values; false otherwise. ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value, bool bypassKnownValues) { EntityUtil.CheckArgumentNull(facetDescription, "facetDescription"); if (!bypassKnownValues) { // Reuse facets with a null value. if (object.ReferenceEquals(value, null)) { return facetDescription.NullValueFacet; } // Reuse facets with a default value. if (object.Equals(facetDescription.DefaultValue, value)) { return facetDescription.DefaultValueFacet; } // Special case boolean facets. if (facetDescription.FacetType.Identity == "Edm.Boolean") { bool boolValue = (bool)value; return facetDescription.GetBooleanFacet(boolValue); } } Facet result = new Facet(facetDescription, value); // Check the type of the value only if we know what the correct CLR type is if (value != null && !Helper.IsUnboundedFacetValue(result) && result.FacetType.ClrType != null) { Type valueType = value.GetType(); if (valueType != result.FacetType.ClrType && !result.FacetType.ClrType.IsAssignableFrom(valueType)) { throw EntityUtil.FacetValueHasIncorrectType(result.Name, result.FacetType.ClrType, valueType, "value"); } } return result; } #endregion #region Fields ///The object describing this facet. private readonly FacetDescription _facetDescription; ///The value assigned to this facet. private readonly object _value; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.Facet; } } ////// Gets the description object for describing the facet /// public FacetDescription Description { get { return _facetDescription; } } ////// Gets/Sets the name of the facet /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _facetDescription.FacetName; } } ////// Gets/Sets the type of the facet /// [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType FacetType { get { return _facetDescription.FacetType; } } ////// Gets/Sets the value of the facet /// ///Thrown if the Facet instance is in ReadOnly state [MetadataProperty(typeof(Object), false)] public Object Value { get { return _value; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return _facetDescription.FacetName; } } ////// Indicates whether the value of the facet is unbounded /// public bool IsUnbounded { get { return object.ReferenceEquals(Value, EdmConstants.UnboundedValue); } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Class for representing a Facet object /// This object is Immutable (not just set to readonly) and /// some parts of the system are depending on that behavior /// [DebuggerDisplay("{Name,nq}={Value}")] public sealed class Facet : MetadataItem { #region Constructors ////// The constructor for constructing a Facet object with the facet description and a value /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null private Facet(FacetDescription facetDescription, object value) :base(MetadataFlags.Readonly) { EntityUtil.GenericCheckArgumentNull(facetDescription, "facetDescription"); _facetDescription = facetDescription; _value = value; } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value) { return Create(facetDescription, value, false); } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet /// true to bypass caching and known values; false otherwise. ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value, bool bypassKnownValues) { EntityUtil.CheckArgumentNull(facetDescription, "facetDescription"); if (!bypassKnownValues) { // Reuse facets with a null value. if (object.ReferenceEquals(value, null)) { return facetDescription.NullValueFacet; } // Reuse facets with a default value. if (object.Equals(facetDescription.DefaultValue, value)) { return facetDescription.DefaultValueFacet; } // Special case boolean facets. if (facetDescription.FacetType.Identity == "Edm.Boolean") { bool boolValue = (bool)value; return facetDescription.GetBooleanFacet(boolValue); } } Facet result = new Facet(facetDescription, value); // Check the type of the value only if we know what the correct CLR type is if (value != null && !Helper.IsUnboundedFacetValue(result) && result.FacetType.ClrType != null) { Type valueType = value.GetType(); if (valueType != result.FacetType.ClrType && !result.FacetType.ClrType.IsAssignableFrom(valueType)) { throw EntityUtil.FacetValueHasIncorrectType(result.Name, result.FacetType.ClrType, valueType, "value"); } } return result; } #endregion #region Fields ///The object describing this facet. private readonly FacetDescription _facetDescription; ///The value assigned to this facet. private readonly object _value; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.Facet; } } ////// Gets the description object for describing the facet /// public FacetDescription Description { get { return _facetDescription; } } ////// Gets/Sets the name of the facet /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _facetDescription.FacetName; } } ////// Gets/Sets the type of the facet /// [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType FacetType { get { return _facetDescription.FacetType; } } ////// Gets/Sets the value of the facet /// ///Thrown if the Facet instance is in ReadOnly state [MetadataProperty(typeof(Object), false)] public Object Value { get { return _value; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return _facetDescription.FacetName; } } ////// Indicates whether the value of the facet is unbounded /// public bool IsUnbounded { get { return object.ReferenceEquals(Value, EdmConstants.UnboundedValue); } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
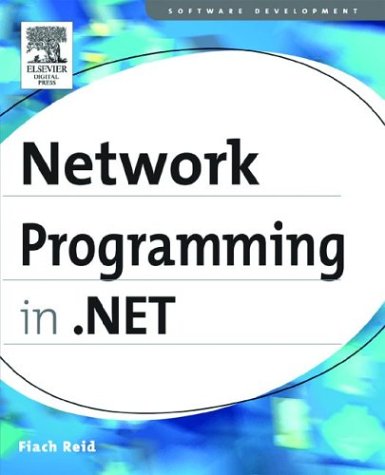
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextSegment.cs
- HostingEnvironmentWrapper.cs
- RuntimeHelpers.cs
- DnsPermission.cs
- GridViewCancelEditEventArgs.cs
- StylusLogic.cs
- MarginCollapsingState.cs
- Sentence.cs
- OdbcDataAdapter.cs
- MatrixTransform.cs
- WebConfigurationFileMap.cs
- TypeBrowserDialog.cs
- FunctionUpdateCommand.cs
- QilValidationVisitor.cs
- PluggableProtocol.cs
- JavaScriptString.cs
- BindingElement.cs
- ServiceElement.cs
- ThreadSafeList.cs
- ClientApiGenerator.cs
- CodeNamespace.cs
- TcpClientChannel.cs
- PerfProviderCollection.cs
- HttpListenerContext.cs
- StaticSiteMapProvider.cs
- XLinq.cs
- MessageSecurityTokenVersion.cs
- MsmqChannelListenerBase.cs
- PolyLineSegmentFigureLogic.cs
- _AuthenticationState.cs
- MouseEvent.cs
- BoolExpr.cs
- EntityClassGenerator.cs
- BitConverter.cs
- ControlBuilderAttribute.cs
- KeyedQueue.cs
- ObjectToken.cs
- SafeWaitHandle.cs
- XPathNodeHelper.cs
- AppSecurityManager.cs
- StatusBarPanelClickEvent.cs
- RowTypeElement.cs
- EndpointInstanceProvider.cs
- QilTernary.cs
- WebControl.cs
- NonParentingControl.cs
- AsynchronousChannel.cs
- LinearQuaternionKeyFrame.cs
- IntermediatePolicyValidator.cs
- OutputCacheProfile.cs
- CommandBindingCollection.cs
- JapaneseLunisolarCalendar.cs
- NeutralResourcesLanguageAttribute.cs
- NativeMethodsOther.cs
- CommonProperties.cs
- RTLAwareMessageBox.cs
- UriExt.cs
- ControlEvent.cs
- PreloadedPackages.cs
- OverflowException.cs
- DbProviderManifest.cs
- Clock.cs
- HijriCalendar.cs
- XmlRawWriterWrapper.cs
- CollectionMarkupSerializer.cs
- DrawingVisualDrawingContext.cs
- ProcessModule.cs
- InstalledFontCollection.cs
- DBConcurrencyException.cs
- SchemaTableOptionalColumn.cs
- CqlGenerator.cs
- QueryCursorEventArgs.cs
- PeerToPeerException.cs
- MatchSingleFxEngineOpcode.cs
- PersianCalendar.cs
- SpecialFolderEnumConverter.cs
- ChineseLunisolarCalendar.cs
- ThreadStateException.cs
- DataGridView.cs
- XmlNode.cs
- MemberDescriptor.cs
- DataGridClipboardCellContent.cs
- SaveLedgerEntryRequest.cs
- RadioButton.cs
- ToggleProviderWrapper.cs
- XmlSchemaAnyAttribute.cs
- CodeCompileUnit.cs
- DataTableReader.cs
- EvidenceTypeDescriptor.cs
- ConnectionStringSettingsCollection.cs
- _NativeSSPI.cs
- RegisteredArrayDeclaration.cs
- Pkcs7Signer.cs
- DynamicResourceExtension.cs
- Function.cs
- ParallelTimeline.cs
- Win32NamedPipes.cs
- SecureConversationSecurityTokenParameters.cs
- OdbcFactory.cs
- contentDescriptor.cs