Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Synthesis / InstalledVoice.cs / 1 / InstalledVoice.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.IO; using System.Speech.AudioFormat; using System.Speech.Internal; using System.Speech.Internal.ObjectTokens; using System.Speech.Internal.Synthesis; using System.Speech.Synthesis.TtsEngine; using System.Threading; using RegistryDataKey = System.Speech.Internal.ObjectTokens.RegistryDataKey; using RegistryEntry = System.Collections.Generic.KeyValuePair; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Synthesis { /// /// TODOC /// [DebuggerDisplay ("{VoiceInfo.Name} [{Enabled ? \"Enabled\" : \"Disabled\"}]")] public class InstalledVoice { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal InstalledVoice (VoiceSynthesis voiceSynthesizer, VoiceInfo voice) { _voiceSynthesizer = voiceSynthesizer; _voice = voice; _enabled = true; } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties ////// TODOC /// public VoiceInfo VoiceInfo { get { return _voice; } } ////// TODOC /// public bool Enabled { get { return _enabled; } set { SetEnabledFlag (value, true); } } #endregion Events //******************************************************************** // // Public Methods // //******************************************************************** #region public Methods /// TODOC public override bool Equals (object obj) { InstalledVoice ti2 = obj as InstalledVoice; if (ti2 == null) { return false; } return VoiceInfo.Name == ti2.VoiceInfo.Name && VoiceInfo.Age == ti2.VoiceInfo.Age && VoiceInfo.Gender == ti2.VoiceInfo.Gender && VoiceInfo.Culture.Equals (ti2.VoiceInfo.Culture); } /// TODOC public override int GetHashCode () { return VoiceInfo.Name.GetHashCode (); } #endregion Events //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods internal static InstalledVoice Find (Listlist, VoiceInfo voiceId) { foreach (InstalledVoice ti in list) { if (ti.Enabled && ti.VoiceInfo.Equals (voiceId)) { return ti; } } return null; } internal static InstalledVoice FirstEnabled (List list, CultureInfo culture) { InstalledVoice voiceFirst = null; foreach (InstalledVoice ti in list) { if (ti.Enabled) { if (Helpers.CompareInvariantCulture (ti.VoiceInfo.Culture, culture)) { return ti; } if (voiceFirst == null) { voiceFirst = ti; } } } return voiceFirst; } internal void SetEnabledFlag (bool value, bool switchContext) { try { if (_enabled != value) { _enabled = value; if (_enabled == false) { // reset the default voice if necessary if (_voice.Equals (_voiceSynthesizer.CurrentVoice (switchContext).VoiceInfo)) { _voiceSynthesizer.Voice = null; } } else { // reset the default voice if necessary. This new voice could be the default _voiceSynthesizer.Voice = null; } } } // If no voice can be set, ignore the error catch (InvalidOperationException) { // reset to the default voice. _voiceSynthesizer.Voice = null; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private VoiceInfo _voice; private bool _enabled; #pragma warning disable 6524 // The voice synthesizer cannot be disposed when this object is deleted. private VoiceSynthesis _voiceSynthesizer; #pragma warning restore 6524 #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.IO; using System.Speech.AudioFormat; using System.Speech.Internal; using System.Speech.Internal.ObjectTokens; using System.Speech.Internal.Synthesis; using System.Speech.Synthesis.TtsEngine; using System.Threading; using RegistryDataKey = System.Speech.Internal.ObjectTokens.RegistryDataKey; using RegistryEntry = System.Collections.Generic.KeyValuePair; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Synthesis { /// /// TODOC /// [DebuggerDisplay ("{VoiceInfo.Name} [{Enabled ? \"Enabled\" : \"Disabled\"}]")] public class InstalledVoice { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal InstalledVoice (VoiceSynthesis voiceSynthesizer, VoiceInfo voice) { _voiceSynthesizer = voiceSynthesizer; _voice = voice; _enabled = true; } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties ////// TODOC /// public VoiceInfo VoiceInfo { get { return _voice; } } ////// TODOC /// public bool Enabled { get { return _enabled; } set { SetEnabledFlag (value, true); } } #endregion Events //******************************************************************** // // Public Methods // //******************************************************************** #region public Methods /// TODOC public override bool Equals (object obj) { InstalledVoice ti2 = obj as InstalledVoice; if (ti2 == null) { return false; } return VoiceInfo.Name == ti2.VoiceInfo.Name && VoiceInfo.Age == ti2.VoiceInfo.Age && VoiceInfo.Gender == ti2.VoiceInfo.Gender && VoiceInfo.Culture.Equals (ti2.VoiceInfo.Culture); } /// TODOC public override int GetHashCode () { return VoiceInfo.Name.GetHashCode (); } #endregion Events //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods internal static InstalledVoice Find (Listlist, VoiceInfo voiceId) { foreach (InstalledVoice ti in list) { if (ti.Enabled && ti.VoiceInfo.Equals (voiceId)) { return ti; } } return null; } internal static InstalledVoice FirstEnabled (List list, CultureInfo culture) { InstalledVoice voiceFirst = null; foreach (InstalledVoice ti in list) { if (ti.Enabled) { if (Helpers.CompareInvariantCulture (ti.VoiceInfo.Culture, culture)) { return ti; } if (voiceFirst == null) { voiceFirst = ti; } } } return voiceFirst; } internal void SetEnabledFlag (bool value, bool switchContext) { try { if (_enabled != value) { _enabled = value; if (_enabled == false) { // reset the default voice if necessary if (_voice.Equals (_voiceSynthesizer.CurrentVoice (switchContext).VoiceInfo)) { _voiceSynthesizer.Voice = null; } } else { // reset the default voice if necessary. This new voice could be the default _voiceSynthesizer.Voice = null; } } } // If no voice can be set, ignore the error catch (InvalidOperationException) { // reset to the default voice. _voiceSynthesizer.Voice = null; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private VoiceInfo _voice; private bool _enabled; #pragma warning disable 6524 // The voice synthesizer cannot be disposed when this object is deleted. private VoiceSynthesis _voiceSynthesizer; #pragma warning restore 6524 #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
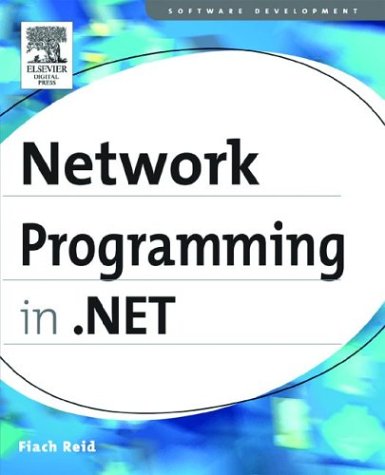
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlUrlResolver.cs
- ToolStripRendererSwitcher.cs
- ControlsConfig.cs
- Win32SafeHandles.cs
- OdbcConnectionStringbuilder.cs
- SystemWebSectionGroup.cs
- RowSpanVector.cs
- Vector3DAnimationBase.cs
- ToolStripDropTargetManager.cs
- GroupLabel.cs
- ContainerParaClient.cs
- HtmlAnchor.cs
- Utils.cs
- FileUtil.cs
- DesignerTransactionCloseEvent.cs
- Floater.cs
- Page.cs
- IgnoreSection.cs
- AddValidationError.cs
- ProgressBarRenderer.cs
- CodeCompiler.cs
- BinaryObjectInfo.cs
- AssemblyInfo.cs
- DocumentAutomationPeer.cs
- TextRunProperties.cs
- QilCloneVisitor.cs
- Site.cs
- Lease.cs
- DoubleCollectionValueSerializer.cs
- ControlCachePolicy.cs
- CodeAttachEventStatement.cs
- BaseValidatorDesigner.cs
- ClosureBinding.cs
- RegionData.cs
- SmtpNtlmAuthenticationModule.cs
- Misc.cs
- CustomPopupPlacement.cs
- StrongNamePublicKeyBlob.cs
- WebPartAuthorizationEventArgs.cs
- ScrollChrome.cs
- CatalogPart.cs
- MachineKeySection.cs
- FixedSOMFixedBlock.cs
- ListBindingHelper.cs
- IOException.cs
- ObjectCacheSettings.cs
- XhtmlCssHandler.cs
- PackageRelationship.cs
- TraceShell.cs
- ColumnResizeAdorner.cs
- TableColumn.cs
- DataPager.cs
- PlatformNotSupportedException.cs
- TargetInvocationException.cs
- HtmlEmptyTagControlBuilder.cs
- MeasurementDCInfo.cs
- XmlSequenceWriter.cs
- WebConvert.cs
- ReturnType.cs
- Size.cs
- ClonableStack.cs
- XmlChoiceIdentifierAttribute.cs
- ColorConvertedBitmap.cs
- SafeFileMapViewHandle.cs
- Debug.cs
- TextWriterEngine.cs
- CreateUserWizardStep.cs
- RegexWriter.cs
- SqlInternalConnection.cs
- AsymmetricSignatureDeformatter.cs
- ToolStripItemClickedEventArgs.cs
- ScrollItemProviderWrapper.cs
- RootAction.cs
- FunctionDetailsReader.cs
- SafeViewOfFileHandle.cs
- Crypto.cs
- TimeEnumHelper.cs
- GenericsInstances.cs
- AmbientProperties.cs
- CustomWebEventKey.cs
- DynamicPropertyHolder.cs
- TimelineGroup.cs
- AbstractExpressions.cs
- QilTargetType.cs
- PagerSettings.cs
- BoundingRectTracker.cs
- ClockGroup.cs
- TreeNodeCollection.cs
- DesignerOptionService.cs
- CqlErrorHelper.cs
- CacheChildrenQuery.cs
- ProfileGroupSettings.cs
- COM2ICategorizePropertiesHandler.cs
- PathData.cs
- RegexTree.cs
- BindingWorker.cs
- Itemizer.cs
- SkewTransform.cs
- MultiByteCodec.cs
- AsymmetricSignatureDeformatter.cs