Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / WebControls / MenuItemBindingCollection.cs / 1 / MenuItemBindingCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web; ////// Provides a collection of MenuItemBinding objects /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class MenuItemBindingCollection : StateManagedCollection { private static readonly Type[] knownTypes = new Type[] { typeof(MenuItemBinding) }; private Menu _owner; private MenuItemBinding _defaultBinding; private MenuItemBindingCollection() { } internal MenuItemBindingCollection(Menu owner) { _owner = owner; } ////// Gets the MenuItemBinding at the specified index /// public MenuItemBinding this[int i] { get { return (MenuItemBinding)((IList)this)[i]; } set { ((IList)this)[i] = value; } } ////// Adds a MenuItemBinding to the collection /// public int Add(MenuItemBinding binding) { return ((IList)this).Add(binding); } public bool Contains(MenuItemBinding binding) { return ((IList)this).Contains(binding); } public void CopyTo(MenuItemBinding[] array, int index) { ((IList)this).CopyTo(array, index); } protected override object CreateKnownType(int index) { return new MenuItemBinding(); } private void FindDefaultBinding() { _defaultBinding = null; // Look for another binding that would be a good default foreach (MenuItemBinding binding in this) { if (binding.Depth == -1 && binding.DataMember.Length == 0) { _defaultBinding = binding; break; } } } ////// Gets a MenuItemBinding data binding definition for the specified depth or datamember /// internal MenuItemBinding GetBinding(string dataMember, int depth) { MenuItemBinding bestMatch = null; int match = 0; if ((dataMember != null) && (dataMember.Length == 0)) { dataMember = null; } foreach (MenuItemBinding binding in this) { if ((binding.Depth == depth)) { if (String.Equals(binding.DataMember, dataMember, StringComparison.CurrentCultureIgnoreCase)) { return binding; } else if ((match < 1) && (binding.DataMember.Length == 0)) { bestMatch = binding; match = 1; } } else if (String.Equals(binding.DataMember, dataMember, StringComparison.CurrentCultureIgnoreCase) && (match < 2) && (binding.Depth == -1)) { bestMatch = binding; match = 2; } } if (bestMatch == null) { // Check that the default binding is still suitable (VSWhidbey 358817) if (_defaultBinding != null) { if (_defaultBinding.Depth != -1 || _defaultBinding.DataMember.Length != 0) { // Look for another binding that would be a good default FindDefaultBinding(); } bestMatch = _defaultBinding; } } return bestMatch; } protected override Type[] GetKnownTypes() { return knownTypes; } public int IndexOf(MenuItemBinding value) { return ((IList)this).IndexOf(value); } public void Insert(int index, MenuItemBinding binding) { ((IList)this).Insert(index, binding); } protected override void OnClear() { _defaultBinding = null; } protected override void OnRemoveComplete(int index, object value) { if (value == _defaultBinding) { FindDefaultBinding(); } } protected override void OnValidate(object value) { base.OnValidate(value); MenuItemBinding binding = value as MenuItemBinding; if ((binding != null) && (binding.DataMember.Length == 0) && (binding.Depth == -1)) { _defaultBinding = binding; } } ////// Removes a MenuItemBinding from the collection. /// public void Remove(MenuItemBinding binding) { ((IList)this).Remove(binding); } ////// Removes a MenuItemBinding from the collection at a given index. /// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } protected override void SetDirtyObject(object o) { if (o is MenuItemBinding) { ((MenuItemBinding)o).SetDirty(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web; ////// Provides a collection of MenuItemBinding objects /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class MenuItemBindingCollection : StateManagedCollection { private static readonly Type[] knownTypes = new Type[] { typeof(MenuItemBinding) }; private Menu _owner; private MenuItemBinding _defaultBinding; private MenuItemBindingCollection() { } internal MenuItemBindingCollection(Menu owner) { _owner = owner; } ////// Gets the MenuItemBinding at the specified index /// public MenuItemBinding this[int i] { get { return (MenuItemBinding)((IList)this)[i]; } set { ((IList)this)[i] = value; } } ////// Adds a MenuItemBinding to the collection /// public int Add(MenuItemBinding binding) { return ((IList)this).Add(binding); } public bool Contains(MenuItemBinding binding) { return ((IList)this).Contains(binding); } public void CopyTo(MenuItemBinding[] array, int index) { ((IList)this).CopyTo(array, index); } protected override object CreateKnownType(int index) { return new MenuItemBinding(); } private void FindDefaultBinding() { _defaultBinding = null; // Look for another binding that would be a good default foreach (MenuItemBinding binding in this) { if (binding.Depth == -1 && binding.DataMember.Length == 0) { _defaultBinding = binding; break; } } } ////// Gets a MenuItemBinding data binding definition for the specified depth or datamember /// internal MenuItemBinding GetBinding(string dataMember, int depth) { MenuItemBinding bestMatch = null; int match = 0; if ((dataMember != null) && (dataMember.Length == 0)) { dataMember = null; } foreach (MenuItemBinding binding in this) { if ((binding.Depth == depth)) { if (String.Equals(binding.DataMember, dataMember, StringComparison.CurrentCultureIgnoreCase)) { return binding; } else if ((match < 1) && (binding.DataMember.Length == 0)) { bestMatch = binding; match = 1; } } else if (String.Equals(binding.DataMember, dataMember, StringComparison.CurrentCultureIgnoreCase) && (match < 2) && (binding.Depth == -1)) { bestMatch = binding; match = 2; } } if (bestMatch == null) { // Check that the default binding is still suitable (VSWhidbey 358817) if (_defaultBinding != null) { if (_defaultBinding.Depth != -1 || _defaultBinding.DataMember.Length != 0) { // Look for another binding that would be a good default FindDefaultBinding(); } bestMatch = _defaultBinding; } } return bestMatch; } protected override Type[] GetKnownTypes() { return knownTypes; } public int IndexOf(MenuItemBinding value) { return ((IList)this).IndexOf(value); } public void Insert(int index, MenuItemBinding binding) { ((IList)this).Insert(index, binding); } protected override void OnClear() { _defaultBinding = null; } protected override void OnRemoveComplete(int index, object value) { if (value == _defaultBinding) { FindDefaultBinding(); } } protected override void OnValidate(object value) { base.OnValidate(value); MenuItemBinding binding = value as MenuItemBinding; if ((binding != null) && (binding.DataMember.Length == 0) && (binding.Depth == -1)) { _defaultBinding = binding; } } ////// Removes a MenuItemBinding from the collection. /// public void Remove(MenuItemBinding binding) { ((IList)this).Remove(binding); } ////// Removes a MenuItemBinding from the collection at a given index. /// public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } protected override void SetDirtyObject(object o) { if (o is MenuItemBinding) { ((MenuItemBinding)o).SetDirty(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
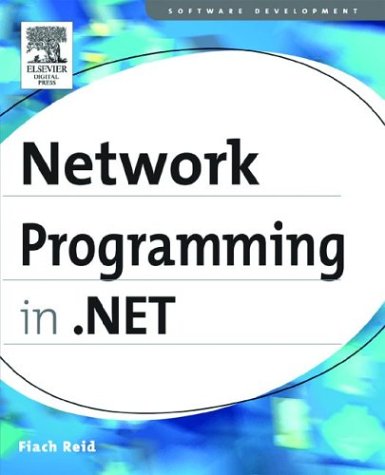
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpProfileGroupBase.cs
- PointCollectionConverter.cs
- WS2007FederationHttpBinding.cs
- ArrayWithOffset.cs
- TypeExtensionSerializer.cs
- SerializerDescriptor.cs
- Pool.cs
- TypeValidationEventArgs.cs
- CustomSignedXml.cs
- DecimalAnimation.cs
- PropertyTab.cs
- MouseActionValueSerializer.cs
- SourceFileBuildProvider.cs
- CaseInsensitiveOrdinalStringComparer.cs
- graph.cs
- CommandValueSerializer.cs
- LiteralControl.cs
- SmtpCommands.cs
- AdvancedBindingEditor.cs
- XmlWriter.cs
- CommandPlan.cs
- MD5.cs
- DataBindingHandlerAttribute.cs
- SignalGate.cs
- Model3D.cs
- DictionarySectionHandler.cs
- DataServiceStreamResponse.cs
- FormCollection.cs
- FlowDocumentReader.cs
- Stacktrace.cs
- XsdBuildProvider.cs
- LinqDataSourceDisposeEventArgs.cs
- SelectedGridItemChangedEvent.cs
- ArrayListCollectionBase.cs
- CheckBoxList.cs
- XmlChildNodes.cs
- SqlLiftWhereClauses.cs
- documentsequencetextcontainer.cs
- DbFunctionCommandTree.cs
- RegexRunnerFactory.cs
- SimpleType.cs
- PropertyInfoSet.cs
- FunctionDescription.cs
- SmtpFailedRecipientsException.cs
- baseaxisquery.cs
- ConsumerConnectionPoint.cs
- SqlTypeConverter.cs
- LocalizeDesigner.cs
- propertyentry.cs
- ValueQuery.cs
- PrintPreviewGraphics.cs
- HitTestParameters3D.cs
- SeekStoryboard.cs
- CompilationUnit.cs
- DataGridViewRowPrePaintEventArgs.cs
- EventLogPermissionEntry.cs
- DateTime.cs
- HtmlInputControl.cs
- ReferentialConstraint.cs
- UInt64Storage.cs
- ScaleTransform3D.cs
- querybuilder.cs
- CheckBoxRenderer.cs
- CanonicalFontFamilyReference.cs
- BroadcastEventHelper.cs
- QilNode.cs
- DuplicateDetector.cs
- SoapFormatter.cs
- HandlerFactoryCache.cs
- RoleManagerModule.cs
- GeneralTransform3D.cs
- AttachInfo.cs
- CFGGrammar.cs
- HtmlTableRow.cs
- ChannelSinkStacks.cs
- StyleBamlTreeBuilder.cs
- ImageMapEventArgs.cs
- ExclusiveCanonicalizationTransform.cs
- DataIdProcessor.cs
- ExpressionPrefixAttribute.cs
- SystemFonts.cs
- FontTypeConverter.cs
- QueryCacheEntry.cs
- OutputCacheProfileCollection.cs
- XsdBuildProvider.cs
- WorkerRequest.cs
- SessionStateSection.cs
- FamilyMap.cs
- ToolBar.cs
- CalendarSelectionChangedEventArgs.cs
- DecoratedNameAttribute.cs
- EventPropertyMap.cs
- ChangesetResponse.cs
- TreeNodeStyle.cs
- StorageModelBuildProvider.cs
- SchemaExporter.cs
- KeyGestureValueSerializer.cs
- SqlGenerator.cs
- SpellerInterop.cs
- EntitySqlQueryCacheEntry.cs