Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / HierarchicalDataSourceControl.cs / 1 / HierarchicalDataSourceControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.ComponentModel; using System.Security.Permissions; [ Bindable(false), ControlBuilder(typeof(DataSourceControlBuilder)), Designer("System.Web.UI.Design.HierarchicalDataSourceDesigner, " + AssemblyRef.SystemDesign), NonVisualControl() ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class HierarchicalDataSourceControl : Control, IHierarchicalDataSource { private static readonly object EventDataSourceChanged = new object(); [ EditorBrowsable(EditorBrowsableState.Never), ] public override string ClientID { get { return base.ClientID; } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override ControlCollection Controls { get { return base.Controls; } } [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ Browsable(false), DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets a value that indicates whether a control should be rendered on /// the page. /// [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool Visible { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.ControlNonVisual, this.GetType().Name)); } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void ApplyStyleSheetSkin(Page page) { base.ApplyStyleSheetSkin(page); } ////// Overidden to prevent child controls from being added to this control. /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override Control FindControl(string id) { return base.FindControl(id); } ////// [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected abstract HierarchicalDataSourceView GetHierarchicalView(string viewPath); [ EditorBrowsable(EditorBrowsableState.Never), ] public override bool HasControls() { return base.HasControls(); } protected virtual void OnDataSourceChanged(EventArgs e) { EventHandler onDataSourceChangedHandler = (EventHandler)Events[EventDataSourceChanged]; if (onDataSourceChangedHandler != null) onDataSourceChangedHandler(this, e); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void RenderControl(HtmlTextWriter writer) { base.RenderControl(writer); } #region Implementation of IHierarchicalDataSource ////// Raised when the underlying data source has changed. The /// change may be due to a change in the control's properties, /// or a change in the data due to an edit action performed by /// the DataSourceControl. /// event EventHandler IHierarchicalDataSource.DataSourceChanged { add { Events.AddHandler(EventDataSourceChanged, value); } remove { Events.RemoveHandler(EventDataSourceChanged, value); } } ///HierarchicalDataSourceView IHierarchicalDataSource.GetHierarchicalView(string viewPath) { return GetHierarchicalView(viewPath); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.ComponentModel; using System.Security.Permissions; [ Bindable(false), ControlBuilder(typeof(DataSourceControlBuilder)), Designer("System.Web.UI.Design.HierarchicalDataSourceDesigner, " + AssemblyRef.SystemDesign), NonVisualControl() ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class HierarchicalDataSourceControl : Control, IHierarchicalDataSource { private static readonly object EventDataSourceChanged = new object(); [ EditorBrowsable(EditorBrowsableState.Never), ] public override string ClientID { get { return base.ClientID; } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override ControlCollection Controls { get { return base.Controls; } } [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ Browsable(false), DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets a value that indicates whether a control should be rendered on /// the page. /// [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool Visible { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.ControlNonVisual, this.GetType().Name)); } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void ApplyStyleSheetSkin(Page page) { base.ApplyStyleSheetSkin(page); } ////// Overidden to prevent child controls from being added to this control. /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override Control FindControl(string id) { return base.FindControl(id); } ////// [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected abstract HierarchicalDataSourceView GetHierarchicalView(string viewPath); [ EditorBrowsable(EditorBrowsableState.Never), ] public override bool HasControls() { return base.HasControls(); } protected virtual void OnDataSourceChanged(EventArgs e) { EventHandler onDataSourceChangedHandler = (EventHandler)Events[EventDataSourceChanged]; if (onDataSourceChangedHandler != null) onDataSourceChangedHandler(this, e); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void RenderControl(HtmlTextWriter writer) { base.RenderControl(writer); } #region Implementation of IHierarchicalDataSource ////// Raised when the underlying data source has changed. The /// change may be due to a change in the control's properties, /// or a change in the data due to an edit action performed by /// the DataSourceControl. /// event EventHandler IHierarchicalDataSource.DataSourceChanged { add { Events.AddHandler(EventDataSourceChanged, value); } remove { Events.RemoveHandler(EventDataSourceChanged, value); } } ///HierarchicalDataSourceView IHierarchicalDataSource.GetHierarchicalView(string viewPath) { return GetHierarchicalView(viewPath); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
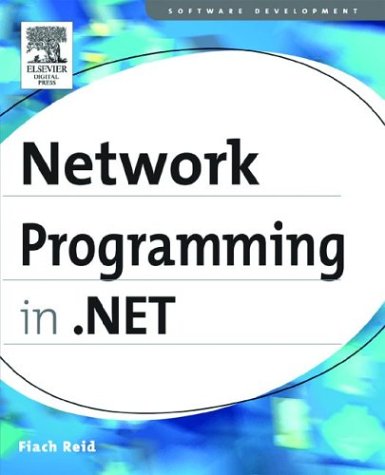
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputControl.cs
- GPRECT.cs
- Span.cs
- Boolean.cs
- BroadcastEventHelper.cs
- DropShadowBitmapEffect.cs
- InputChannelAcceptor.cs
- ObjectQuery_EntitySqlExtensions.cs
- TableLayoutPanelCellPosition.cs
- Publisher.cs
- PerfCounterSection.cs
- TemplateLookupAction.cs
- PackageRelationshipSelector.cs
- CodeArrayCreateExpression.cs
- ColorBlend.cs
- CmsInterop.cs
- DesignerHelpers.cs
- ChoiceConverter.cs
- BindingFormattingDialog.cs
- TreeNodeCollection.cs
- ListDataBindEventArgs.cs
- HttpStreamFormatter.cs
- BamlTreeUpdater.cs
- CultureMapper.cs
- MarkupProperty.cs
- XsltException.cs
- DecimalAnimation.cs
- XmlObjectSerializerContext.cs
- GridViewSelectEventArgs.cs
- CompositeControl.cs
- DataSourceControl.cs
- ToolboxDataAttribute.cs
- SharedDp.cs
- SecurityKeyIdentifierClause.cs
- DocumentGridContextMenu.cs
- LogEntrySerializationException.cs
- ExtentKey.cs
- AttributeTable.cs
- UIElementHelper.cs
- TraceHandlerErrorFormatter.cs
- ContentFileHelper.cs
- CodeThrowExceptionStatement.cs
- SQLByte.cs
- BlobPersonalizationState.cs
- Typeface.cs
- _HeaderInfoTable.cs
- KnownTypesHelper.cs
- ContextQuery.cs
- Preprocessor.cs
- COMException.cs
- SiteMapNodeItemEventArgs.cs
- DesignerOptions.cs
- FormViewRow.cs
- DynamicValidatorEventArgs.cs
- OrderedEnumerableRowCollection.cs
- BaseParser.cs
- DataDocumentXPathNavigator.cs
- QuadraticBezierSegment.cs
- CompilationPass2Task.cs
- Panel.cs
- FormCollection.cs
- DropDownHolder.cs
- EdmMember.cs
- IPPacketInformation.cs
- NegatedConstant.cs
- DesignTimeParseData.cs
- Interlocked.cs
- COM2ExtendedBrowsingHandler.cs
- DataError.cs
- VisualCollection.cs
- DoubleCollection.cs
- SessionConnectionReader.cs
- HiddenField.cs
- cookiecontainer.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- ZipIOLocalFileHeader.cs
- UrlPath.cs
- SpnegoTokenProvider.cs
- HttpRequest.cs
- TextCollapsingProperties.cs
- XMLUtil.cs
- ResourceReferenceExpressionConverter.cs
- FragmentQuery.cs
- MutexSecurity.cs
- StoreItemCollection.Loader.cs
- XmlAttributeAttribute.cs
- RowToFieldTransformer.cs
- PropertySegmentSerializationProvider.cs
- SendMailErrorEventArgs.cs
- RandomDelaySendsAsyncResult.cs
- Color.cs
- FixedPageAutomationPeer.cs
- TreeNodeStyleCollection.cs
- GridItem.cs
- UncommonField.cs
- StyleXamlTreeBuilder.cs
- BamlLocalizableResource.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- SpotLight.cs
- AvTraceDetails.cs