Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewComboBoxColumn.cs / 1 / DataGridViewComboBoxColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Text; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Globalization; ///[ Designer("System.Windows.Forms.Design.DataGridViewComboBoxColumnDesigner, " + AssemblyRef.SystemDesign), ToolboxBitmapAttribute(typeof(DataGridViewComboBoxColumn), "DataGridViewComboBoxColumn.bmp") ] public class DataGridViewComboBoxColumn : DataGridViewColumn { private static Type columnType = typeof(DataGridViewComboBoxColumn); /// public DataGridViewComboBoxColumn() : base(new DataGridViewComboBoxCell()) { ((DataGridViewComboBoxCell)base.CellTemplate).TemplateComboBoxColumn = this; } /// [ Browsable(true), DefaultValue(true), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnAutoCompleteDescr) ] public bool AutoComplete { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.AutoComplete; } set { if (this.AutoComplete != value) { this.ComboBoxCellTemplate.AutoComplete = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.AutoComplete = value; } } } } } } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override DataGridViewCell CellTemplate { get { return base.CellTemplate; } set { DataGridViewComboBoxCell dataGridViewComboBoxCell = value as DataGridViewComboBoxCell; if (value != null && dataGridViewComboBoxCell == null) { throw new InvalidCastException(SR.GetString(SR.DataGridViewTypeColumn_WrongCellTemplateType, "System.Windows.Forms.DataGridViewComboBoxCell")); } base.CellTemplate = value; if (value != null) { dataGridViewComboBoxCell.TemplateComboBoxColumn = this; } } } private DataGridViewComboBoxCell ComboBoxCellTemplate { get { return (DataGridViewComboBoxCell) this.CellTemplate; } } /// [ DefaultValue(null), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnDataSourceDescr), RefreshProperties(RefreshProperties.Repaint), AttributeProvider(typeof(IListSource)), ] public object DataSource { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DataSource; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DataSource = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DataSource = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } /// [ DefaultValue(""), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnDisplayMemberDescr), TypeConverterAttribute("System.Windows.Forms.Design.DataMemberFieldConverter, " + AssemblyRef.SystemDesign), Editor("System.Windows.Forms.Design.DataMemberFieldEditor, " + AssemblyRef.SystemDesign, typeof(System.Drawing.Design.UITypeEditor)) ] public string DisplayMember { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DisplayMember; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DisplayMember = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DisplayMember = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } /// [ DefaultValue(DataGridViewComboBoxDisplayStyle.DropDownButton), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_ComboBoxColumnDisplayStyleDescr) ] public DataGridViewComboBoxDisplayStyle DisplayStyle { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DisplayStyle; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DisplayStyle = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DisplayStyleInternal = value; } } // Calling InvalidateColumn instead of OnColumnCommonChange because DisplayStyle does not affect preferred size. this.DataGridView.InvalidateColumn(this.Index); } } } /// [ DefaultValue(false), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_ComboBoxColumnDisplayStyleForCurrentCellOnlyDescr) ] public bool DisplayStyleForCurrentCellOnly { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DisplayStyleForCurrentCellOnly; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DisplayStyleForCurrentCellOnly = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DisplayStyleForCurrentCellOnlyInternal = value; } } // Calling InvalidateColumn instead of OnColumnCommonChange because DisplayStyleForCurrentCellOnly does not affect preferred size. this.DataGridView.InvalidateColumn(this.Index); } } } /// [ DefaultValue(1), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnDropDownWidthDescr), ] public int DropDownWidth { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DropDownWidth; } set { if (this.DropDownWidth != value) { this.ComboBoxCellTemplate.DropDownWidth = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DropDownWidth = value; } } } } } } /// [ DefaultValue(FlatStyle.Standard), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_ComboBoxColumnFlatStyleDescr), ] public FlatStyle FlatStyle { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewComboBoxCell) this.CellTemplate).FlatStyle; } set { if (this.FlatStyle != value) { ((DataGridViewComboBoxCell)this.CellTemplate).FlatStyle = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.FlatStyleInternal = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } } /// [ Editor("System.Windows.Forms.Design.StringCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnItemsDescr) ] public DataGridViewComboBoxCell.ObjectCollection Items { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.GetItems(this.DataGridView); } } /// [ DefaultValue(""), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnValueMemberDescr), TypeConverterAttribute("System.Windows.Forms.Design.DataMemberFieldConverter, " + AssemblyRef.SystemDesign), Editor("System.Windows.Forms.Design.DataMemberFieldEditor, " + AssemblyRef.SystemDesign, typeof(System.Drawing.Design.UITypeEditor)) ] public string ValueMember { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.ValueMember; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.ValueMember = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.ValueMember = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } /// [ DefaultValue(DataGridViewComboBoxCell.DATAGRIDVIEWCOMBOBOXCELL_defaultMaxDropDownItems), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnMaxDropDownItemsDescr) ] public int MaxDropDownItems { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.MaxDropDownItems; } set { if (this.MaxDropDownItems != value) { this.ComboBoxCellTemplate.MaxDropDownItems = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.MaxDropDownItems = value; } } } } } } /// [ DefaultValue(false), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnSortedDescr) ] public bool Sorted { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.Sorted; } set { if (this.Sorted != value) { this.ComboBoxCellTemplate.Sorted = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.Sorted = value; } } } } } } /// public override object Clone() { DataGridViewComboBoxColumn dataGridViewColumn; Type thisType = this.GetType(); if (thisType == columnType) //performance improvement { dataGridViewColumn = new DataGridViewComboBoxColumn(); } else { // SECREVIEW : Late-binding does not represent a security thread, see bug#411899 for more info.. // dataGridViewColumn = (DataGridViewComboBoxColumn)System.Activator.CreateInstance(thisType); } if (dataGridViewColumn != null) { base.CloneInternal(dataGridViewColumn); ((DataGridViewComboBoxCell) dataGridViewColumn.CellTemplate).TemplateComboBoxColumn = dataGridViewColumn; } return dataGridViewColumn; } internal void OnItemsCollectionChanged() { // Items collection of the CellTemplate was changed. // Update the items collection of each existing DataGridViewComboBoxCell in the column. if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; object[] items = ((DataGridViewComboBoxCell)this.CellTemplate).Items.InnerArray.ToArray(); for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.Items.ClearInternal(); dataGridViewCell.Items.AddRangeInternal(items); } } this.DataGridView.OnColumnCommonChange(this.Index); } } /// /// /// public override string ToString() { StringBuilder sb = new StringBuilder(64); sb.Append("DataGridViewComboBoxColumn { Name="); sb.Append(this.Name); sb.Append(", Index="); sb.Append(this.Index.ToString(CultureInfo.CurrentCulture)); sb.Append(" }"); return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Text; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Globalization; ///[ Designer("System.Windows.Forms.Design.DataGridViewComboBoxColumnDesigner, " + AssemblyRef.SystemDesign), ToolboxBitmapAttribute(typeof(DataGridViewComboBoxColumn), "DataGridViewComboBoxColumn.bmp") ] public class DataGridViewComboBoxColumn : DataGridViewColumn { private static Type columnType = typeof(DataGridViewComboBoxColumn); /// public DataGridViewComboBoxColumn() : base(new DataGridViewComboBoxCell()) { ((DataGridViewComboBoxCell)base.CellTemplate).TemplateComboBoxColumn = this; } /// [ Browsable(true), DefaultValue(true), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnAutoCompleteDescr) ] public bool AutoComplete { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.AutoComplete; } set { if (this.AutoComplete != value) { this.ComboBoxCellTemplate.AutoComplete = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.AutoComplete = value; } } } } } } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override DataGridViewCell CellTemplate { get { return base.CellTemplate; } set { DataGridViewComboBoxCell dataGridViewComboBoxCell = value as DataGridViewComboBoxCell; if (value != null && dataGridViewComboBoxCell == null) { throw new InvalidCastException(SR.GetString(SR.DataGridViewTypeColumn_WrongCellTemplateType, "System.Windows.Forms.DataGridViewComboBoxCell")); } base.CellTemplate = value; if (value != null) { dataGridViewComboBoxCell.TemplateComboBoxColumn = this; } } } private DataGridViewComboBoxCell ComboBoxCellTemplate { get { return (DataGridViewComboBoxCell) this.CellTemplate; } } /// [ DefaultValue(null), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnDataSourceDescr), RefreshProperties(RefreshProperties.Repaint), AttributeProvider(typeof(IListSource)), ] public object DataSource { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DataSource; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DataSource = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DataSource = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } /// [ DefaultValue(""), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnDisplayMemberDescr), TypeConverterAttribute("System.Windows.Forms.Design.DataMemberFieldConverter, " + AssemblyRef.SystemDesign), Editor("System.Windows.Forms.Design.DataMemberFieldEditor, " + AssemblyRef.SystemDesign, typeof(System.Drawing.Design.UITypeEditor)) ] public string DisplayMember { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DisplayMember; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DisplayMember = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DisplayMember = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } /// [ DefaultValue(DataGridViewComboBoxDisplayStyle.DropDownButton), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_ComboBoxColumnDisplayStyleDescr) ] public DataGridViewComboBoxDisplayStyle DisplayStyle { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DisplayStyle; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DisplayStyle = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DisplayStyleInternal = value; } } // Calling InvalidateColumn instead of OnColumnCommonChange because DisplayStyle does not affect preferred size. this.DataGridView.InvalidateColumn(this.Index); } } } /// [ DefaultValue(false), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_ComboBoxColumnDisplayStyleForCurrentCellOnlyDescr) ] public bool DisplayStyleForCurrentCellOnly { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DisplayStyleForCurrentCellOnly; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.DisplayStyleForCurrentCellOnly = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DisplayStyleForCurrentCellOnlyInternal = value; } } // Calling InvalidateColumn instead of OnColumnCommonChange because DisplayStyleForCurrentCellOnly does not affect preferred size. this.DataGridView.InvalidateColumn(this.Index); } } } /// [ DefaultValue(1), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnDropDownWidthDescr), ] public int DropDownWidth { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.DropDownWidth; } set { if (this.DropDownWidth != value) { this.ComboBoxCellTemplate.DropDownWidth = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.DropDownWidth = value; } } } } } } /// [ DefaultValue(FlatStyle.Standard), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_ComboBoxColumnFlatStyleDescr), ] public FlatStyle FlatStyle { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewComboBoxCell) this.CellTemplate).FlatStyle; } set { if (this.FlatStyle != value) { ((DataGridViewComboBoxCell)this.CellTemplate).FlatStyle = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.FlatStyleInternal = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } } /// [ Editor("System.Windows.Forms.Design.StringCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnItemsDescr) ] public DataGridViewComboBoxCell.ObjectCollection Items { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.GetItems(this.DataGridView); } } /// [ DefaultValue(""), SRCategory(SR.CatData), SRDescription(SR.DataGridView_ComboBoxColumnValueMemberDescr), TypeConverterAttribute("System.Windows.Forms.Design.DataMemberFieldConverter, " + AssemblyRef.SystemDesign), Editor("System.Windows.Forms.Design.DataMemberFieldEditor, " + AssemblyRef.SystemDesign, typeof(System.Drawing.Design.UITypeEditor)) ] public string ValueMember { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.ValueMember; } set { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } this.ComboBoxCellTemplate.ValueMember = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.ValueMember = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } /// [ DefaultValue(DataGridViewComboBoxCell.DATAGRIDVIEWCOMBOBOXCELL_defaultMaxDropDownItems), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnMaxDropDownItemsDescr) ] public int MaxDropDownItems { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.MaxDropDownItems; } set { if (this.MaxDropDownItems != value) { this.ComboBoxCellTemplate.MaxDropDownItems = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.MaxDropDownItems = value; } } } } } } /// [ DefaultValue(false), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_ComboBoxColumnSortedDescr) ] public bool Sorted { get { if (this.ComboBoxCellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return this.ComboBoxCellTemplate.Sorted; } set { if (this.Sorted != value) { this.ComboBoxCellTemplate.Sorted = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.Sorted = value; } } } } } } /// public override object Clone() { DataGridViewComboBoxColumn dataGridViewColumn; Type thisType = this.GetType(); if (thisType == columnType) //performance improvement { dataGridViewColumn = new DataGridViewComboBoxColumn(); } else { // SECREVIEW : Late-binding does not represent a security thread, see bug#411899 for more info.. // dataGridViewColumn = (DataGridViewComboBoxColumn)System.Activator.CreateInstance(thisType); } if (dataGridViewColumn != null) { base.CloneInternal(dataGridViewColumn); ((DataGridViewComboBoxCell) dataGridViewColumn.CellTemplate).TemplateComboBoxColumn = dataGridViewColumn; } return dataGridViewColumn; } internal void OnItemsCollectionChanged() { // Items collection of the CellTemplate was changed. // Update the items collection of each existing DataGridViewComboBoxCell in the column. if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; object[] items = ((DataGridViewComboBoxCell)this.CellTemplate).Items.InnerArray.ToArray(); for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewComboBoxCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewComboBoxCell; if (dataGridViewCell != null) { dataGridViewCell.Items.ClearInternal(); dataGridViewCell.Items.AddRangeInternal(items); } } this.DataGridView.OnColumnCommonChange(this.Index); } } /// /// /// public override string ToString() { StringBuilder sb = new StringBuilder(64); sb.Append("DataGridViewComboBoxColumn { Name="); sb.Append(this.Name); sb.Append(", Index="); sb.Append(this.Index.ToString(CultureInfo.CurrentCulture)); sb.Append(" }"); return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.///
Link Menu
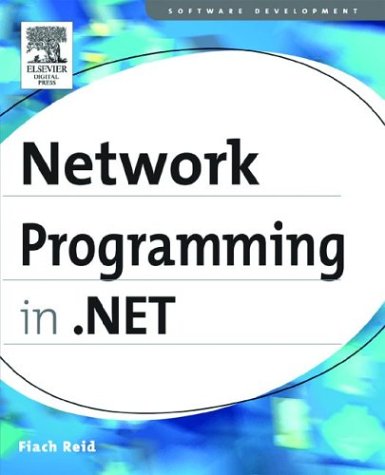
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FlowThrottle.cs
- SortedSetDebugView.cs
- DynamicMethod.cs
- X509UI.cs
- SHA512.cs
- SqlTriggerAttribute.cs
- DbFunctionCommandTree.cs
- SrgsDocument.cs
- SplitterPanel.cs
- XmlChildEnumerator.cs
- XmlTextReaderImpl.cs
- X509Utils.cs
- FileDataSourceCache.cs
- ISAPIApplicationHost.cs
- IRCollection.cs
- ContentDisposition.cs
- DataServiceHost.cs
- StatusBarAutomationPeer.cs
- UIPermission.cs
- SchemaTableOptionalColumn.cs
- StringReader.cs
- IntSecurity.cs
- PathNode.cs
- TimeoutValidationAttribute.cs
- DataControlCommands.cs
- DynamicActivity.cs
- DataGridViewCellStyleChangedEventArgs.cs
- ToolstripProfessionalRenderer.cs
- TreeWalkHelper.cs
- SortedSet.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- ServiceOperationInfoTypeConverter.cs
- JsonReader.cs
- RootBrowserWindowAutomationPeer.cs
- RegexStringValidator.cs
- WeakReference.cs
- RangeValidator.cs
- NonBatchDirectoryCompiler.cs
- HWStack.cs
- SerializationSectionGroup.cs
- KeyValueConfigurationCollection.cs
- TransactionManager.cs
- HtmlWindow.cs
- IgnoreSection.cs
- TextEditorLists.cs
- DataRow.cs
- BulletedList.cs
- Point4D.cs
- HttpCachePolicy.cs
- XNodeValidator.cs
- ActionFrame.cs
- OAVariantLib.cs
- MetadataCache.cs
- CodeGroup.cs
- HtmlGenericControl.cs
- LogEntry.cs
- OracleParameter.cs
- DefaultSection.cs
- InputBuffer.cs
- DataGridTextColumn.cs
- KeySpline.cs
- DragDrop.cs
- RadioButtonBaseAdapter.cs
- KeyEventArgs.cs
- DataGridViewDataErrorEventArgs.cs
- MarkupCompilePass2.cs
- BinaryMethodMessage.cs
- VisualStateManager.cs
- Brush.cs
- FrameworkContentElement.cs
- TemplateColumn.cs
- Constant.cs
- SoapSchemaMember.cs
- OracleString.cs
- ThreadSafeList.cs
- StylusPointPropertyInfo.cs
- DataGridrowEditEndingEventArgs.cs
- TempEnvironment.cs
- ModelUIElement3D.cs
- MetabaseReader.cs
- Array.cs
- WebPartDeleteVerb.cs
- MimeMapping.cs
- StatusBarPanelClickEvent.cs
- StorageRoot.cs
- FontInfo.cs
- FixedSOMPageConstructor.cs
- CodeEventReferenceExpression.cs
- UdpSocket.cs
- Control.cs
- ForEachAction.cs
- LinearKeyFrames.cs
- PropertyPathWorker.cs
- DragEvent.cs
- DataBoundControl.cs
- TrackingParameters.cs
- BaseProcessor.cs
- GatewayDefinition.cs
- ButtonColumn.cs
- StateBag.cs