Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / SharedStatics.cs / 1 / SharedStatics.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: SharedStatics ** ** ** Purpose: Container for statics that are shared across AppDomains. ** ** =============================================================================*/ namespace System { using System.Threading; using System.Runtime.Remoting; using System.Security; using System.Security.Util; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using StringMaker = System.Security.Util.Tokenizer.StringMaker; internal sealed class SharedStatics { // this is declared static but is actually forced to be the same object // for each AppDomain at AppDomain create time. internal static SharedStatics _sharedStatics; // when we create the single object we can construct anything we will need // here. If not too many, then just create them all in the constructor, otherwise // can have the property check & create. Need to be aware of threading issues // when do so though. // Note: This ctor is not called when we setup _sharedStatics via AppDomain::SetupSharedStatics SharedStatics() { _Remoting_Identity_IDGuid = null; _Remoting_Identity_IDSeqNum = 0x40; // Reserve initial numbers for well known objects. _maker = null; } private String _Remoting_Identity_IDGuid; public static String Remoting_Identity_IDGuid { get { if (_sharedStatics._Remoting_Identity_IDGuid == null) { bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._Remoting_Identity_IDGuid == null) { _sharedStatics._Remoting_Identity_IDGuid = Guid.NewGuid().ToString().Replace('-', '_'); } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } BCLDebug.Assert(_sharedStatics._Remoting_Identity_IDGuid != null, "_sharedStatics._Remoting_Identity_IDGuid != null"); return _sharedStatics._Remoting_Identity_IDGuid; } } private StringMaker _maker; static public StringMaker GetSharedStringMaker() { StringMaker maker = null; bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._maker != null) { maker = _sharedStatics._maker; _sharedStatics._maker = null; } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } if (maker == null) { maker = new StringMaker(); } return maker; } static public void ReleaseSharedStringMaker(ref StringMaker maker) { // save this stringmaker so someone else can use it bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); _sharedStatics._maker = maker; maker = null; } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } // Note this may not need to be process-wide. private int _Remoting_Identity_IDSeqNum; internal static int Remoting_Identity_GetNextSeqNum() { return Interlocked.Increment(ref _sharedStatics._Remoting_Identity_IDSeqNum); } // This is the total amount of memory currently "reserved" via // all MemoryFailPoints allocated within the process. // Stored as a long because we need to use Interlocked.Add. private long _memFailPointReservedMemory; [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static long AddMemoryFailPointReservation(long size) { // Size can legitimately be negative - see Dispose. return Interlocked.Add(ref _sharedStatics._memFailPointReservedMemory, (long) size); } internal static ulong MemoryFailPointReservedMemory { get { BCLDebug.Assert(_sharedStatics._memFailPointReservedMemory >= 0, "Process-wide MemoryFailPoint reserved memory was negative!"); return (ulong) _sharedStatics._memFailPointReservedMemory; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: SharedStatics ** ** ** Purpose: Container for statics that are shared across AppDomains. ** ** =============================================================================*/ namespace System { using System.Threading; using System.Runtime.Remoting; using System.Security; using System.Security.Util; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using StringMaker = System.Security.Util.Tokenizer.StringMaker; internal sealed class SharedStatics { // this is declared static but is actually forced to be the same object // for each AppDomain at AppDomain create time. internal static SharedStatics _sharedStatics; // when we create the single object we can construct anything we will need // here. If not too many, then just create them all in the constructor, otherwise // can have the property check & create. Need to be aware of threading issues // when do so though. // Note: This ctor is not called when we setup _sharedStatics via AppDomain::SetupSharedStatics SharedStatics() { _Remoting_Identity_IDGuid = null; _Remoting_Identity_IDSeqNum = 0x40; // Reserve initial numbers for well known objects. _maker = null; } private String _Remoting_Identity_IDGuid; public static String Remoting_Identity_IDGuid { get { if (_sharedStatics._Remoting_Identity_IDGuid == null) { bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._Remoting_Identity_IDGuid == null) { _sharedStatics._Remoting_Identity_IDGuid = Guid.NewGuid().ToString().Replace('-', '_'); } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } BCLDebug.Assert(_sharedStatics._Remoting_Identity_IDGuid != null, "_sharedStatics._Remoting_Identity_IDGuid != null"); return _sharedStatics._Remoting_Identity_IDGuid; } } private StringMaker _maker; static public StringMaker GetSharedStringMaker() { StringMaker maker = null; bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); if (_sharedStatics._maker != null) { maker = _sharedStatics._maker; _sharedStatics._maker = null; } } finally { if (tookLock) Monitor.Exit(_sharedStatics); } if (maker == null) { maker = new StringMaker(); } return maker; } static public void ReleaseSharedStringMaker(ref StringMaker maker) { // save this stringmaker so someone else can use it bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(_sharedStatics, ref tookLock); _sharedStatics._maker = maker; maker = null; } finally { if (tookLock) Monitor.Exit(_sharedStatics); } } // Note this may not need to be process-wide. private int _Remoting_Identity_IDSeqNum; internal static int Remoting_Identity_GetNextSeqNum() { return Interlocked.Increment(ref _sharedStatics._Remoting_Identity_IDSeqNum); } // This is the total amount of memory currently "reserved" via // all MemoryFailPoints allocated within the process. // Stored as a long because we need to use Interlocked.Add. private long _memFailPointReservedMemory; [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static long AddMemoryFailPointReservation(long size) { // Size can legitimately be negative - see Dispose. return Interlocked.Add(ref _sharedStatics._memFailPointReservedMemory, (long) size); } internal static ulong MemoryFailPointReservedMemory { get { BCLDebug.Assert(_sharedStatics._memFailPointReservedMemory >= 0, "Process-wide MemoryFailPoint reserved memory was negative!"); return (ulong) _sharedStatics._memFailPointReservedMemory; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
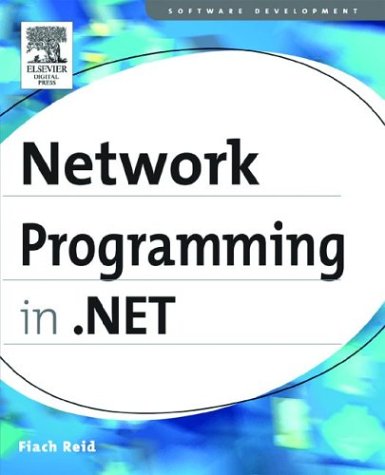
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigXmlAttribute.cs
- Process.cs
- XmlSchemaComplexContent.cs
- TriggerActionCollection.cs
- DeviceFilterEditorDialog.cs
- IImplicitResourceProvider.cs
- DayRenderEvent.cs
- DispatchOperation.cs
- TextServicesPropertyRanges.cs
- DbCommandDefinition.cs
- FlowLayoutPanel.cs
- WebBaseEventKeyComparer.cs
- MenuItem.cs
- Method.cs
- RuleDefinitions.cs
- CodeMemberField.cs
- NameSpaceExtractor.cs
- FunctionImportMapping.ReturnTypeRenameMapping.cs
- ColumnTypeConverter.cs
- HtmlTextViewAdapter.cs
- ReflectPropertyDescriptor.cs
- SafeUserTokenHandle.cs
- GridViewSortEventArgs.cs
- DesignerActionGlyph.cs
- XmlValueConverter.cs
- CommunicationObjectFaultedException.cs
- BinHexEncoding.cs
- Rotation3DKeyFrameCollection.cs
- MimeTypeAttribute.cs
- BooleanExpr.cs
- CurrentChangingEventArgs.cs
- PerformanceCounterPermissionEntryCollection.cs
- DecoderNLS.cs
- AliasedSlot.cs
- StickyNoteAnnotations.cs
- InvokeMethodActivityDesigner.cs
- BroadcastEventHelper.cs
- FileNotFoundException.cs
- XmlParser.cs
- AssemblyHash.cs
- XXXInfos.cs
- TemplateManager.cs
- DataGridViewMethods.cs
- BitArray.cs
- RestHandler.cs
- XXXInfos.cs
- HttpCacheVary.cs
- JournalEntryListConverter.cs
- Rotation3DAnimationBase.cs
- DispatcherExceptionFilterEventArgs.cs
- ReadOnlyDataSource.cs
- VerificationException.cs
- HttpConfigurationSystem.cs
- RegionInfo.cs
- AspNetSynchronizationContext.cs
- DocumentPage.cs
- Sql8ExpressionRewriter.cs
- TextInfo.cs
- PrinterUnitConvert.cs
- Visitor.cs
- VScrollProperties.cs
- Pts.cs
- TextChange.cs
- XmlSignatureProperties.cs
- BamlCollectionHolder.cs
- RightsManagementManager.cs
- XsltException.cs
- ConnectorRouter.cs
- CollectionConverter.cs
- DataGridPageChangedEventArgs.cs
- oledbmetadatacolumnnames.cs
- MetadataWorkspace.cs
- SortDescriptionCollection.cs
- Point.cs
- TypeUtils.cs
- ReferentialConstraint.cs
- DetailsViewInsertEventArgs.cs
- HTMLTextWriter.cs
- MetadataException.cs
- BaseParser.cs
- DataBoundControlHelper.cs
- ScrollBarAutomationPeer.cs
- DrawToolTipEventArgs.cs
- GridViewPageEventArgs.cs
- ColumnMapCopier.cs
- DateTimeValueSerializerContext.cs
- DataGridViewMethods.cs
- DependencyObjectType.cs
- DefaultValueTypeConverter.cs
- PropertyTabAttribute.cs
- IndentTextWriter.cs
- JoinGraph.cs
- GradientBrush.cs
- DeploymentExceptionMapper.cs
- ReadOnlyMetadataCollection.cs
- Condition.cs
- PaperSize.cs
- RepeaterCommandEventArgs.cs
- SecurityIdentifierElementCollection.cs
- ComplexTypeEmitter.cs