Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapCodecInfo.cs / 1 / BitmapCodecInfo.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: BitmapCodecInfo.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.Windows.Media.Imaging; using System.Text; using MS.Internal.PresentationCore; // SecurityHelper namespace System.Windows.Media.Imaging { #region BitmapCodecInfo ////// Codec info for a given Encoder/Decoder /// public abstract class BitmapCodecInfo { #region Constructors ////// Constructor /// protected BitmapCodecInfo() { } ////// Internal Constructor /// internal BitmapCodecInfo(SafeMILHandle codecInfoHandle) { Debug.Assert(codecInfoHandle != null); _isBuiltIn = true; _codecInfoHandle = codecInfoHandle; } #endregion #region Public Properties ////// Container format /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual Guid ContainerFormat { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); Guid containerFormat; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetContainerFormat( _codecInfoHandle, out containerFormat )); return containerFormat; } } ////// Author /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string Author { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder author = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetAuthor( _codecInfoHandle, 0, author, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { author = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetAuthor( _codecInfoHandle, length, author, out length )); } if (author != null) return author.ToString(); else return String.Empty; } } ////// Version /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual System.Version Version { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder version = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetVersion( _codecInfoHandle, 0, version, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { version = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetVersion( _codecInfoHandle, length, version, out length )); } if (version != null) return new Version(version.ToString()); else return new Version(); } } ////// Spec Version /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual Version SpecificationVersion { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder specVersion = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetSpecVersion( _codecInfoHandle, 0, specVersion, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { specVersion = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetSpecVersion( _codecInfoHandle, length, specVersion, out length )); } if (specVersion != null) return new Version(specVersion.ToString()); else return new Version(); } } ////// Friendly Name /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string FriendlyName { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder friendlyName = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetFriendlyName( _codecInfoHandle, 0, friendlyName, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { friendlyName = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetFriendlyName( _codecInfoHandle, length, friendlyName, out length )); } if (friendlyName != null) return friendlyName.ToString(); else return String.Empty; } } ////// Device Manufacturer /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string DeviceManufacturer { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder deviceManufacturer = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceManufacturer( _codecInfoHandle, 0, deviceManufacturer, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { deviceManufacturer = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceManufacturer( _codecInfoHandle, length, deviceManufacturer, out length )); } if (deviceManufacturer != null) return deviceManufacturer.ToString(); else return String.Empty; } } ////// Device Models /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string DeviceModels { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder deviceModels = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceModels( _codecInfoHandle, 0, deviceModels, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { deviceModels = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceModels( _codecInfoHandle, length, deviceModels, out length )); } if (deviceModels != null) return deviceModels.ToString(); else return String.Empty; } } ////// Mime types /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string MimeTypes { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder mimeTypes = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetMimeTypes( _codecInfoHandle, 0, mimeTypes, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { mimeTypes = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetMimeTypes( _codecInfoHandle, length, mimeTypes, out length )); } if (mimeTypes != null) return mimeTypes.ToString(); else return String.Empty; } } ////// File extensions /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string FileExtensions { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder fileExtensions = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetFileExtensions( _codecInfoHandle, 0, fileExtensions, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { fileExtensions = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetFileExtensions( _codecInfoHandle, length, fileExtensions, out length )); } if (fileExtensions != null) return fileExtensions.ToString(); else return String.Empty; } } ////// Does Support Animation /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsAnimation { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsAnimation; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportAnimation( _codecInfoHandle, out supportsAnimation )); return supportsAnimation; } } ////// Does Support Lossless /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsLossless { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsLossless; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportLossless( _codecInfoHandle, out supportsLossless )); return supportsLossless; } } ////// Does Support Multiple Frames /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsMultipleFrames { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsMultiFrame; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportMultiframe( _codecInfoHandle, out supportsMultiFrame )); return supportsMultiFrame; } } #endregion #region Private Methods private void EnsureBuiltIn() { if (!_isBuiltIn) { throw new NotImplementedException(); } } #endregion #region Data Members /// is this a built in codec info? private bool _isBuiltIn; /// Codec info handle SafeMILHandle _codecInfoHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: BitmapCodecInfo.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.Windows.Media.Imaging; using System.Text; using MS.Internal.PresentationCore; // SecurityHelper namespace System.Windows.Media.Imaging { #region BitmapCodecInfo ////// Codec info for a given Encoder/Decoder /// public abstract class BitmapCodecInfo { #region Constructors ////// Constructor /// protected BitmapCodecInfo() { } ////// Internal Constructor /// internal BitmapCodecInfo(SafeMILHandle codecInfoHandle) { Debug.Assert(codecInfoHandle != null); _isBuiltIn = true; _codecInfoHandle = codecInfoHandle; } #endregion #region Public Properties ////// Container format /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual Guid ContainerFormat { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); Guid containerFormat; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetContainerFormat( _codecInfoHandle, out containerFormat )); return containerFormat; } } ////// Author /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string Author { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder author = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetAuthor( _codecInfoHandle, 0, author, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { author = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetAuthor( _codecInfoHandle, length, author, out length )); } if (author != null) return author.ToString(); else return String.Empty; } } ////// Version /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual System.Version Version { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder version = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetVersion( _codecInfoHandle, 0, version, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { version = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetVersion( _codecInfoHandle, length, version, out length )); } if (version != null) return new Version(version.ToString()); else return new Version(); } } ////// Spec Version /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual Version SpecificationVersion { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder specVersion = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetSpecVersion( _codecInfoHandle, 0, specVersion, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { specVersion = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetSpecVersion( _codecInfoHandle, length, specVersion, out length )); } if (specVersion != null) return new Version(specVersion.ToString()); else return new Version(); } } ////// Friendly Name /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string FriendlyName { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder friendlyName = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetFriendlyName( _codecInfoHandle, 0, friendlyName, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { friendlyName = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetFriendlyName( _codecInfoHandle, length, friendlyName, out length )); } if (friendlyName != null) return friendlyName.ToString(); else return String.Empty; } } ////// Device Manufacturer /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string DeviceManufacturer { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder deviceManufacturer = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceManufacturer( _codecInfoHandle, 0, deviceManufacturer, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { deviceManufacturer = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceManufacturer( _codecInfoHandle, length, deviceManufacturer, out length )); } if (deviceManufacturer != null) return deviceManufacturer.ToString(); else return String.Empty; } } ////// Device Models /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string DeviceModels { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder deviceModels = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceModels( _codecInfoHandle, 0, deviceModels, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { deviceModels = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceModels( _codecInfoHandle, length, deviceModels, out length )); } if (deviceModels != null) return deviceModels.ToString(); else return String.Empty; } } ////// Mime types /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string MimeTypes { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder mimeTypes = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetMimeTypes( _codecInfoHandle, 0, mimeTypes, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { mimeTypes = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetMimeTypes( _codecInfoHandle, length, mimeTypes, out length )); } if (mimeTypes != null) return mimeTypes.ToString(); else return String.Empty; } } ////// File extensions /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string FileExtensions { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder fileExtensions = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetFileExtensions( _codecInfoHandle, 0, fileExtensions, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { fileExtensions = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetFileExtensions( _codecInfoHandle, length, fileExtensions, out length )); } if (fileExtensions != null) return fileExtensions.ToString(); else return String.Empty; } } ////// Does Support Animation /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsAnimation { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsAnimation; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportAnimation( _codecInfoHandle, out supportsAnimation )); return supportsAnimation; } } ////// Does Support Lossless /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsLossless { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsLossless; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportLossless( _codecInfoHandle, out supportsLossless )); return supportsLossless; } } ////// Does Support Multiple Frames /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsMultipleFrames { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsMultiFrame; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportMultiframe( _codecInfoHandle, out supportsMultiFrame )); return supportsMultiFrame; } } #endregion #region Private Methods private void EnsureBuiltIn() { if (!_isBuiltIn) { throw new NotImplementedException(); } } #endregion #region Data Members /// is this a built in codec info? private bool _isBuiltIn; /// Codec info handle SafeMILHandle _codecInfoHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
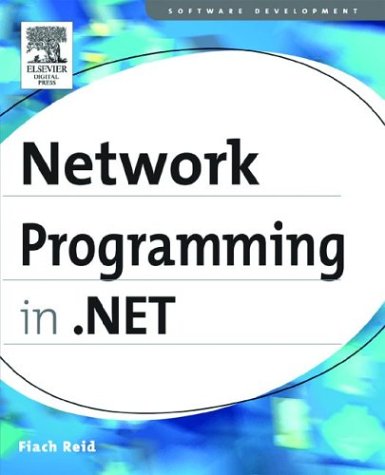
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Base64Encoder.cs
- DataViewListener.cs
- RoleGroupCollectionEditor.cs
- XmlParser.cs
- OperationCanceledException.cs
- TransactionChannelListener.cs
- MissingSatelliteAssemblyException.cs
- VersionedStreamOwner.cs
- ServiceRoute.cs
- DemultiplexingClientMessageFormatter.cs
- DatagridviewDisplayedBandsData.cs
- ServiceSettingsResponseInfo.cs
- WebConfigurationManager.cs
- WorkflowInlining.cs
- SafeNativeMethods.cs
- ErrorHandler.cs
- HttpProfileGroupBase.cs
- HttpResponseInternalWrapper.cs
- CopyNodeSetAction.cs
- Condition.cs
- CodeAttributeArgumentCollection.cs
- ComPlusInstanceContextInitializer.cs
- PngBitmapDecoder.cs
- InteropExecutor.cs
- EpmTargetPathSegment.cs
- KeyProperty.cs
- RemotingSurrogateSelector.cs
- EdmComplexPropertyAttribute.cs
- Assert.cs
- RenderData.cs
- SqlErrorCollection.cs
- BroadcastEventHelper.cs
- TextBox.cs
- OutOfMemoryException.cs
- WmfPlaceableFileHeader.cs
- ProxyElement.cs
- APCustomTypeDescriptor.cs
- RecordManager.cs
- GridItem.cs
- ResourceAttributes.cs
- ItemsChangedEventArgs.cs
- IdentitySection.cs
- MarshalDirectiveException.cs
- WindowsListViewGroupHelper.cs
- WindowsToolbarItemAsMenuItem.cs
- figurelength.cs
- Span.cs
- PageParser.cs
- DataGridViewCellLinkedList.cs
- HttpProxyCredentialType.cs
- CqlErrorHelper.cs
- WebProxyScriptElement.cs
- TextEndOfParagraph.cs
- LoginAutoFormat.cs
- SpStreamWrapper.cs
- NewItemsContextMenuStrip.cs
- Soap12ProtocolReflector.cs
- HostedElements.cs
- NotConverter.cs
- DebugView.cs
- ObjectStateFormatter.cs
- XmlSchemaComplexContentExtension.cs
- XmlSchemaAnnotation.cs
- MetadataItem_Static.cs
- _TransmitFileOverlappedAsyncResult.cs
- EnumBuilder.cs
- GregorianCalendarHelper.cs
- CodeGeneratorOptions.cs
- XmlLanguageConverter.cs
- ResetableIterator.cs
- DrawingGroup.cs
- WorkflowPageSetupDialog.cs
- TransactionChannelFaultConverter.cs
- Matrix.cs
- ParameterBuilder.cs
- DataMemberFieldEditor.cs
- WebPartConnectVerb.cs
- IPGlobalProperties.cs
- CellParaClient.cs
- UniqueConstraint.cs
- SqlDataReaderSmi.cs
- ImageMap.cs
- LinqDataSourceDeleteEventArgs.cs
- RequestStatusBarUpdateEventArgs.cs
- PropVariant.cs
- SoapBinding.cs
- TextPattern.cs
- Journal.cs
- LineInfo.cs
- StreamGeometryContext.cs
- DataListDesigner.cs
- FontStyles.cs
- ContentTextAutomationPeer.cs
- DetailsViewDeletedEventArgs.cs
- SystemIcmpV4Statistics.cs
- FileInfo.cs
- QuaternionRotation3D.cs
- EntityClassGenerator.cs
- LinqDataSourceEditData.cs
- ImpersonationContext.cs