Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Mapping / StorageEntitySetMapping.cs / 2 / StorageEntitySetMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....],[....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Represents the Mapping metadata for an EnitytSet in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityTypeMapping /// --TableMappingFragment /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// This class represents the metadata for the EntitySetMapping elements in the /// above example. And it is possible to access the EntityTypeMaps underneath it. /// internal class StorageEntitySetMapping : StorageSetMapping { #region Constructors ////// Construct a EntitySet mapping object /// /// EntitySet metadata object /// The entity Container Mapping that contains this Set mapping internal StorageEntitySetMapping(EntitySet extent, StorageEntityContainerMapping entityContainerMapping) : base(extent, entityContainerMapping) { m_functionMappings = new List(); m_implicitlyMappedAssociationSetEnds = new List (); } #endregion #region Fields private readonly List m_functionMappings; private readonly List m_implicitlyMappedAssociationSetEnds; #endregion #region Properties /// /// Gets all function mappings for this entity set. /// internal IListFunctionMappings { get { return m_functionMappings.AsReadOnly(); } } /// /// Gets all association sets that are implicitly "covered" through function mappings. /// internal IListImplicitlyMappedAssociationSetEnds { get { return m_implicitlyMappedAssociationSetEnds.AsReadOnly(); } } /// /// Whether the EntitySetMapping has empty content /// Returns true if there are no Function Maps and no table Mapping fragments /// internal override bool HasNoContent { get { if (m_functionMappings.Count != 0) { return false; } return base.HasNoContent; } } #endregion #region Methods ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("EntitySetMapping"); sb.Append(" "); sb.Append("Name:"); sb.Append(this.Set.Name); if (this.QueryView != null) { sb.Append(" "); sb.Append("Query View:"); sb.Append(this.QueryView); } Console.WriteLine(sb.ToString()); foreach (StorageTypeMapping typeMapping in TypeMappings) { typeMapping.Print(index+5); } foreach (StorageEntityTypeFunctionMapping functionMappping in m_functionMappings) { functionMappping.Print(index + 10); } } ////// Requires: /// - Function mapping refers to a sub-type of this entity set's element type /// - Function mappings for types are not redundantly specified /// Adds a new function mapping for this class. /// /// Function mapping to add. May not be null. internal void AddFunctionMapping(StorageEntityTypeFunctionMapping functionMapping) { AssertFunctionMappingInvariants(functionMapping); m_functionMappings.Add(functionMapping); // check if any association sets are indirectly mapped within this function mapping // through association navigation bindings functionMapping.DeleteFunctionMapping.AddReferencedAssociationSetEnds((EntitySet)this.Set, m_implicitlyMappedAssociationSetEnds); functionMapping.InsertFunctionMapping.AddReferencedAssociationSetEnds((EntitySet)this.Set, m_implicitlyMappedAssociationSetEnds); functionMapping.UpdateFunctionMapping.AddReferencedAssociationSetEnds((EntitySet)this.Set, m_implicitlyMappedAssociationSetEnds); } [Conditional("DEBUG")] internal void AssertFunctionMappingInvariants(StorageEntityTypeFunctionMapping functionMapping) { Debug.Assert(null != functionMapping, "function mapping must not be null"); Debug.Assert(functionMapping.EntityType.Equals(this.Set.ElementType) || Helper.IsSubtypeOf(functionMapping.EntityType, this.Set.ElementType), "attempting to add a function mapping with the wrong entity type"); foreach (StorageEntityTypeFunctionMapping existingFunctionMapping in m_functionMappings) { Debug.Assert(!existingFunctionMapping.EntityType.Equals(functionMapping.EntityType), "function mapping already exists for this type"); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....],[....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Represents the Mapping metadata for an EnitytSet in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityTypeMapping /// --TableMappingFragment /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// This class represents the metadata for the EntitySetMapping elements in the /// above example. And it is possible to access the EntityTypeMaps underneath it. /// internal class StorageEntitySetMapping : StorageSetMapping { #region Constructors ////// Construct a EntitySet mapping object /// /// EntitySet metadata object /// The entity Container Mapping that contains this Set mapping internal StorageEntitySetMapping(EntitySet extent, StorageEntityContainerMapping entityContainerMapping) : base(extent, entityContainerMapping) { m_functionMappings = new List(); m_implicitlyMappedAssociationSetEnds = new List (); } #endregion #region Fields private readonly List m_functionMappings; private readonly List m_implicitlyMappedAssociationSetEnds; #endregion #region Properties /// /// Gets all function mappings for this entity set. /// internal IListFunctionMappings { get { return m_functionMappings.AsReadOnly(); } } /// /// Gets all association sets that are implicitly "covered" through function mappings. /// internal IListImplicitlyMappedAssociationSetEnds { get { return m_implicitlyMappedAssociationSetEnds.AsReadOnly(); } } /// /// Whether the EntitySetMapping has empty content /// Returns true if there are no Function Maps and no table Mapping fragments /// internal override bool HasNoContent { get { if (m_functionMappings.Count != 0) { return false; } return base.HasNoContent; } } #endregion #region Methods ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("EntitySetMapping"); sb.Append(" "); sb.Append("Name:"); sb.Append(this.Set.Name); if (this.QueryView != null) { sb.Append(" "); sb.Append("Query View:"); sb.Append(this.QueryView); } Console.WriteLine(sb.ToString()); foreach (StorageTypeMapping typeMapping in TypeMappings) { typeMapping.Print(index+5); } foreach (StorageEntityTypeFunctionMapping functionMappping in m_functionMappings) { functionMappping.Print(index + 10); } } ////// Requires: /// - Function mapping refers to a sub-type of this entity set's element type /// - Function mappings for types are not redundantly specified /// Adds a new function mapping for this class. /// /// Function mapping to add. May not be null. internal void AddFunctionMapping(StorageEntityTypeFunctionMapping functionMapping) { AssertFunctionMappingInvariants(functionMapping); m_functionMappings.Add(functionMapping); // check if any association sets are indirectly mapped within this function mapping // through association navigation bindings functionMapping.DeleteFunctionMapping.AddReferencedAssociationSetEnds((EntitySet)this.Set, m_implicitlyMappedAssociationSetEnds); functionMapping.InsertFunctionMapping.AddReferencedAssociationSetEnds((EntitySet)this.Set, m_implicitlyMappedAssociationSetEnds); functionMapping.UpdateFunctionMapping.AddReferencedAssociationSetEnds((EntitySet)this.Set, m_implicitlyMappedAssociationSetEnds); } [Conditional("DEBUG")] internal void AssertFunctionMappingInvariants(StorageEntityTypeFunctionMapping functionMapping) { Debug.Assert(null != functionMapping, "function mapping must not be null"); Debug.Assert(functionMapping.EntityType.Equals(this.Set.ElementType) || Helper.IsSubtypeOf(functionMapping.EntityType, this.Set.ElementType), "attempting to add a function mapping with the wrong entity type"); foreach (StorageEntityTypeFunctionMapping existingFunctionMapping in m_functionMappings) { Debug.Assert(!existingFunctionMapping.EntityType.Equals(functionMapping.EntityType), "function mapping already exists for this type"); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
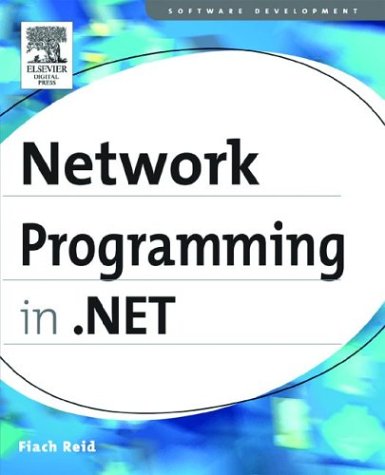
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PixelFormats.cs
- MessageQueueKey.cs
- XmlDictionary.cs
- WebContext.cs
- NegatedCellConstant.cs
- PermissionListSet.cs
- BmpBitmapDecoder.cs
- X509CertificateCollection.cs
- GlobalEventManager.cs
- ToolTipAutomationPeer.cs
- UIElementCollection.cs
- IPEndPointCollection.cs
- LayoutEvent.cs
- DataContractJsonSerializerOperationFormatter.cs
- InfoCardRSACryptoProvider.cs
- TableItemPattern.cs
- RowsCopiedEventArgs.cs
- RadioButtonFlatAdapter.cs
- CaretElement.cs
- KeyValueConfigurationElement.cs
- NativeCompoundFileAPIs.cs
- DataAdapter.cs
- UrlPath.cs
- ToolStripDropDownClosingEventArgs.cs
- ContextProperty.cs
- ValidationErrorCollection.cs
- ByteAnimationBase.cs
- ServiceDescriptions.cs
- GeneralTransform2DTo3D.cs
- WizardStepBase.cs
- UnionCodeGroup.cs
- AddInDeploymentState.cs
- DataBindingCollection.cs
- SuppressIldasmAttribute.cs
- DbParameterCollectionHelper.cs
- Win32Exception.cs
- versioninfo.cs
- DataSourceXmlTextReader.cs
- AnimationLayer.cs
- StyleXamlParser.cs
- VirtualPath.cs
- WebChannelFactory.cs
- RotateTransform3D.cs
- FrameworkContentElementAutomationPeer.cs
- WebPartMinimizeVerb.cs
- XmlDeclaration.cs
- StringSorter.cs
- FrugalList.cs
- Hyperlink.cs
- CorePropertiesFilter.cs
- StrongNameIdentityPermission.cs
- SetIndexBinder.cs
- XmlEventCache.cs
- VectorAnimationBase.cs
- ExcludeFromCodeCoverageAttribute.cs
- ConfigXmlAttribute.cs
- MaskDesignerDialog.cs
- WindowsRegion.cs
- XmlElementAttribute.cs
- X500Name.cs
- XDRSchema.cs
- RenderContext.cs
- XmlNamedNodeMap.cs
- MessageFormatterConverter.cs
- BufferBuilder.cs
- TextDecoration.cs
- MachineKey.cs
- _ListenerResponseStream.cs
- DataTemplateKey.cs
- ConfigXmlDocument.cs
- IndexedGlyphRun.cs
- ExpressionVisitor.cs
- _SpnDictionary.cs
- ModuleConfigurationInfo.cs
- CacheSection.cs
- ValuePatternIdentifiers.cs
- SchemaNotation.cs
- SwitchAttribute.cs
- XsdDateTime.cs
- ResourceDefaultValueAttribute.cs
- RIPEMD160Managed.cs
- VisualStyleInformation.cs
- RoleGroupCollection.cs
- EventLogPermissionAttribute.cs
- MobileControlPersister.cs
- ItemType.cs
- SiteOfOriginPart.cs
- CommandField.cs
- WizardForm.cs
- WorkflowValidationFailedException.cs
- SqlMethodTransformer.cs
- IisTraceWebEventProvider.cs
- MaskedTextBox.cs
- Attributes.cs
- SqlNodeAnnotations.cs
- CodeMemberProperty.cs
- BamlReader.cs
- DataStreams.cs
- PermissionAttributes.cs
- Exceptions.cs