Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / ColumnCollection.cs / 1 / ColumnCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataGridColumnCollection : ICollection, IStateManager { private DataGrid owner; private ArrayList columns; private bool marked; ///Represents the collection of columns to be displayed in /// a ////// control. /// public DataGridColumnCollection(DataGrid owner, ArrayList columns) { this.owner = owner; this.columns = columns; } ///Initializes a new instance of ///class. /// [ Browsable(false) ] public int Count { get { return columns.Count; } } ///Gets the number of columns in the collection. This property is read-only. ////// [ Browsable(false) ] public bool IsReadOnly { get { return false; } } ///Gets a value that specifies whether items in the ///can be /// modified. This property is read-only. /// [ Browsable(false) ] public bool IsSynchronized { get { return false; } } ///Gets a value that indicates whether the ///is thread-safe. This property is read-only. /// [ Browsable(false) ] public Object SyncRoot { get { return this; } } ///Gets the object used to synchronize access to the collection. This property is read-only. ////// [ Browsable(false) ] public DataGridColumn this[int index] { get { return (DataGridColumn)columns[index]; } } ///Gets a ///at the specified index in the /// collection. /// public void Add(DataGridColumn column) { AddAt(-1, column); } ///Appends a ///to the collection. /// public void AddAt(int index, DataGridColumn column) { if (column == null) { throw new ArgumentNullException("column"); } if (index == -1) { columns.Add(column); } else { columns.Insert(index, column); } column.SetOwner(owner); if (marked) ((IStateManager)column).TrackViewState(); OnColumnsChanged(); } ///Inserts a ///to the collection /// at the specified index. /// public void Clear() { columns.Clear(); OnColumnsChanged(); } ///Empties the collection of all ///objects. /// public void CopyTo(Array array, int index) { if (array == null) { throw new ArgumentNullException("array"); } for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies the contents of the entire collection into an ///appending at /// the specified index of the . /// public IEnumerator GetEnumerator() { return columns.GetEnumerator(); } ///Creates an enumerator for the ///used to iterate through the collection. /// public int IndexOf(DataGridColumn column) { if (column != null) { return columns.IndexOf(column); } return -1; } ///Returns the index of the first occurrence of a value in a ///. /// private void OnColumnsChanged() { if (owner != null) { owner.OnColumnsChanged(); } } ////// public void RemoveAt(int index) { if ((index >= 0) && (index < Count)) { columns.RemoveAt(index); OnColumnsChanged(); } else { throw new ArgumentOutOfRangeException("index"); } } ///Removes a ///from the collection at the specified /// index. /// public void Remove(DataGridColumn column) { int index = IndexOf(column); if (index >= 0) { RemoveAt(index); } } ///Removes the specified ///from the collection. /// /// Return true if tracking state changes. /// bool IStateManager.IsTrackingViewState { get { return marked; } } ////// /// Load previously saved state. /// void IStateManager.LoadViewState(object savedState) { if (savedState != null) { object[] columnsState = (object[])savedState; if (columnsState.Length == columns.Count) { for (int i = 0; i < columnsState.Length; i++) { if (columnsState[i] != null) { ((IStateManager)columns[i]).LoadViewState(columnsState[i]); } } } } } ////// /// Start tracking state changes. /// void IStateManager.TrackViewState() { marked = true; int columnCount = columns.Count; for (int i = 0; i < columnCount; i++) { ((IStateManager)columns[i]).TrackViewState(); } } ////// /// Return object containing state changes. /// object IStateManager.SaveViewState() { int columnCount = columns.Count; object[] columnsState = new object[columnCount]; bool savedState = false; for (int i = 0; i < columnCount; i++) { columnsState[i] = ((IStateManager)columns[i]).SaveViewState(); if (columnsState[i] != null) savedState = true; } return savedState ? columnsState : null; } } }
Link Menu
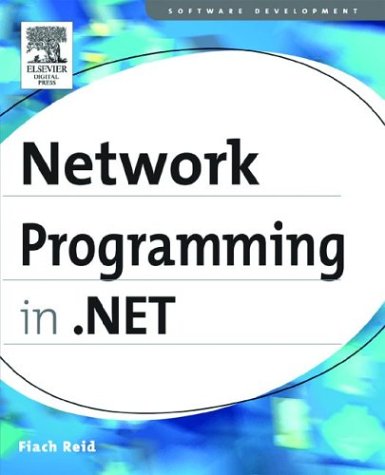
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewDeletedEventArgs.cs
- FacetChecker.cs
- FixedNode.cs
- SerializationObjectManager.cs
- ActivityExecutionFilter.cs
- OdbcHandle.cs
- DateTimeOffsetConverter.cs
- CultureTable.cs
- MasterPageBuildProvider.cs
- DetailsViewUpdateEventArgs.cs
- StreamGeometry.cs
- COAUTHINFO.cs
- ObjectKeyFrameCollection.cs
- LineUtil.cs
- SettingsPropertyNotFoundException.cs
- CaseInsensitiveHashCodeProvider.cs
- ListViewHitTestInfo.cs
- ReachUIElementCollectionSerializer.cs
- CommandHelper.cs
- LockedActivityGlyph.cs
- PageBuildProvider.cs
- StorageInfo.cs
- UniqueTransportManagerRegistration.cs
- EpmSourceTree.cs
- AppSettingsReader.cs
- ModuleConfigurationInfo.cs
- EmptyEnumerator.cs
- StickyNoteAnnotations.cs
- SchemaAttDef.cs
- ZipPackagePart.cs
- CoTaskMemUnicodeSafeHandle.cs
- DataGridViewImageCell.cs
- XmlWhitespace.cs
- KeysConverter.cs
- BitConverter.cs
- DataTemplateKey.cs
- AsymmetricCryptoHandle.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- AssemblyFilter.cs
- XmlUnspecifiedAttribute.cs
- WebRequest.cs
- IDispatchConstantAttribute.cs
- HMACSHA512.cs
- HMACRIPEMD160.cs
- PenThread.cs
- TextComposition.cs
- Transactions.cs
- COM2EnumConverter.cs
- DesignerAutoFormatCollection.cs
- SqlDataSourceCommandEventArgs.cs
- DashStyles.cs
- DetailsView.cs
- SingleTagSectionHandler.cs
- DuplexChannelFactory.cs
- ProgressBarAutomationPeer.cs
- BindingWorker.cs
- ValidatorCompatibilityHelper.cs
- DetailsViewModeEventArgs.cs
- ApplicationHost.cs
- ObjectSecurity.cs
- Point3DKeyFrameCollection.cs
- AuthenticationService.cs
- Helper.cs
- UseLicense.cs
- ArgumentOutOfRangeException.cs
- PartialList.cs
- MessageSmuggler.cs
- TextBox.cs
- DesignerActionUI.cs
- TagElement.cs
- WebPartZoneCollection.cs
- AssertSection.cs
- ComPlusInstanceContextInitializer.cs
- XPathDocument.cs
- WebPartZoneBase.cs
- URL.cs
- ConstructorArgumentAttribute.cs
- QueryStringParameter.cs
- WindowsStartMenu.cs
- MessageBox.cs
- BindingCollection.cs
- SpanIndex.cs
- DataColumnMappingCollection.cs
- CellParaClient.cs
- WebEventTraceProvider.cs
- HtmlTextBoxAdapter.cs
- LayoutTable.cs
- KeyValueConfigurationCollection.cs
- BinaryUtilClasses.cs
- XamlPathDataSerializer.cs
- DataControlFieldHeaderCell.cs
- ClipboardData.cs
- StdRegProviderWrapper.cs
- MaskedTextBoxTextEditorDropDown.cs
- RegionIterator.cs
- RenderDataDrawingContext.cs
- ProcessModuleCollection.cs
- SqlServer2KCompatibilityCheck.cs
- SimplePropertyEntry.cs
- IChannel.cs