Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Configuration / RemoteWebConfigurationHostServer.cs / 4 / RemoteWebConfigurationHostServer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Collections; using System.Configuration; using System.Security; using System.IO; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Reflection; using System.Web.Util; using System.Collections.Specialized; using System.Xml; using System.Security.Cryptography; #if !FEATURE_PAL // FEATURE_PAL does not enable access control using System.Security.AccessControl; #endif // !FEATURE_PAL using System.Security.Permissions; #if !FEATURE_PAL // FEATURE_PAL does not enable COM [ComVisible(true), ClassInterface(ClassInterfaceType.AutoDual), #if WIN64 Guid("CE6ED9D0-3BF2-4243-B0AB-919BAC47542B"), ProgId("System.Web.Configuration.RemoteWebConfigurationHostServer_64")] #else Guid("8DEC0FA2-CC19-494F-8613-1F6221C0C5AB"), ProgId("System.Web.Configuration.RemoteWebConfigurationHostServer_32")] #endif #endif // FEATURE_PAL does not enable COM [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RemoteWebConfigurationHostServer : IRemoteWebConfigurationHostServer { internal const char FilePathsSeparatorChar = '<'; static internal readonly char[] FilePathsSeparatorParams = new char[] {FilePathsSeparatorChar}; public byte[] GetData(string fileName, bool getReadTimeOnly, out long readTime) { if (!fileName.ToLowerInvariant().EndsWith(".config", StringComparison.Ordinal)) throw new Exception(SR.GetString(SR.Can_not_access_files_other_than_config)); byte [] buf; if (File.Exists(fileName)) { if (getReadTimeOnly) { buf = new byte[0]; } else { buf = File.ReadAllBytes(fileName); } DateTime lastWrite = File.GetLastWriteTimeUtc(fileName); readTime = (DateTime.UtcNow > lastWrite ? DateTime.UtcNow.Ticks : lastWrite.Ticks); } else { buf = new byte[0]; readTime = DateTime.UtcNow.Ticks; } return buf; } public void WriteData(string fileName, string templateFileName, byte[] data, ref long readTime) { if (!fileName.ToLowerInvariant().EndsWith(".config", StringComparison.Ordinal)) throw new Exception(SR.GetString(SR.Can_not_access_files_other_than_config)); bool fileExists = File.Exists(fileName); FileInfo fileInfo = null; FileAttributes fileAttributes = FileAttributes.Normal; string tempFile = null; Exception createStreamExcep = null; FileStream tempFileStream = null; long lastWriteTicks = 0; long utcNowTicks = 0; ///////////////////////////////////////////////////////////////////// // Step 1: If the file exists, then make sure it hasn't been written to since it was read if (fileExists && File.GetLastWriteTimeUtc(fileName).Ticks > readTime) { throw new Exception(SR.GetString(SR.File_changed_since_read, fileName)); } ///////////////////////////////////////////////////////////////////// // Step 2: Get the security-descriptor and attributes of the file if (fileExists) { try { fileInfo = new FileInfo(fileName); fileAttributes = fileInfo.Attributes; } catch { } if (((int)(fileAttributes & (FileAttributes.ReadOnly | FileAttributes.Hidden))) != 0) throw new Exception(SR.GetString(SR.File_is_read_only, fileName)); } ///////////////////////////////////////////////////////////////////// // Step 3: Generate a temp file name. Make sure that the temp file doesn't exist tempFile = fileName + "." + GetRandomFileExt() + ".tmp"; for (int iter = 0; File.Exists(tempFile); iter++) { // if it exists, then use a different random name if (iter > 100) // don't try more than 100 times throw new Exception(SR.GetString(SR.Unable_to_create_temp_file)); else tempFile = fileName + "." + GetRandomFileExt() + ".tmp"; } ///////////////////////////////////////////////////////////////////// // Step 4: Write the buffer to the temp file, and move it to the actual file try { tempFileStream = new FileStream(tempFile, FileMode.CreateNew, FileAccess.Write, FileShare.ReadWrite, data.Length, FileOptions.WriteThrough); tempFileStream.Write(data, 0, data.Length); } catch (Exception e) { createStreamExcep = e; } finally { if (tempFileStream != null) tempFileStream.Close(); } if (createStreamExcep != null) { try { File.Delete(tempFile); } catch { } throw createStreamExcep; } if (fileExists) { try { DuplicateFileAttributes(fileName, tempFile); } catch { } } else if ( templateFileName != null ) { try { DuplicateTemplateAttributes(fileName, templateFileName); } catch { } } ///////////////////////////////////////////////////////////////////// // Step 4: Move the temp filt to the actual file if (!UnsafeNativeMethods.MoveFileEx(tempFile, fileName, MOVEFILE_COPY_ALLOWED | MOVEFILE_REPLACE_EXISTING | MOVEFILE_WRITE_THROUGH)) { try { File.Delete(tempFile); } catch { } Marshal.ThrowExceptionForHR(Marshal.GetHRForLastWin32Error()); } ///////////////////////////////////////////////////////////////////// // Step 5: Set the attributes of the file if (fileExists) { fileInfo = new FileInfo(fileName); fileInfo.Attributes = fileAttributes; } ///////////////////////////////////////////////////////////////////// // Step 6: Record the current time as the read-time lastWriteTicks = File.GetLastWriteTimeUtc(fileName).Ticks; utcNowTicks = DateTime.UtcNow.Ticks; readTime = (utcNowTicks > lastWriteTicks ? utcNowTicks : lastWriteTicks); } public string GetFilePaths(int webLevelAsInt, string path, string site, string locationSubPath) { WebLevel webLevel = (WebLevel) webLevelAsInt; IConfigMapPath configMapPath = IISMapPath.GetInstance(); // Get the configuration paths and application information string appSiteName, appSiteID; VirtualPath appPath; string configPath, locationConfigPath; WebConfigurationHost.GetConfigPaths(configMapPath, webLevel, VirtualPath.CreateNonRelativeAllowNull(path), site, locationSubPath, out appPath, out appSiteName, out appSiteID, out configPath, out locationConfigPath); // // Format of filePaths: // appPath < appSiteName < appSiteID < configPath < locationConfigPath [< configPath < fileName]+ // ArrayList filePaths = new ArrayList(); filePaths.Add(VirtualPath.GetVirtualPathString(appPath)); filePaths.Add(appSiteName); filePaths.Add(appSiteID); filePaths.Add(configPath); filePaths.Add(locationConfigPath); string dummySiteID; VirtualPath virtualPath; WebConfigurationHost.GetSiteIDAndVPathFromConfigPath(configPath, out dummySiteID, out virtualPath); // pathmap for machine.config filePaths.Add(WebConfigurationHost.MachineConfigPath); filePaths.Add(HttpConfigurationSystem.MachineConfigurationFilePath); // pathmap for root web.config if (webLevel != WebLevel.Machine) { filePaths.Add(WebConfigurationHost.RootWebConfigPath); filePaths.Add(HttpConfigurationSystem.RootWebConfigurationFilePath); // pathmap for other paths for (VirtualPath currentVirtualPath = virtualPath; currentVirtualPath != null; currentVirtualPath = currentVirtualPath.Parent) { string currentConfigPath = WebConfigurationHost.GetConfigPathFromSiteIDAndVPath(appSiteID, currentVirtualPath); string currentFilePath = configMapPath.MapPath(appSiteID, currentVirtualPath.VirtualPathString); currentFilePath = System.IO.Path.Combine(currentFilePath, HttpConfigurationSystem.WebConfigFileName); filePaths.Add(currentConfigPath); filePaths.Add(currentFilePath); } } // join into a single string StringBuilder sb = new StringBuilder(); for (int i = 0; i < filePaths.Count; i++) { if (i > 0) { sb.Append(FilePathsSeparatorChar); } string part = (string) filePaths[i]; sb.Append(part); } return sb.ToString(); } public string DoEncryptOrDecrypt(bool doEncrypt, string xmlString, string protectionProviderName, string protectionProviderType, string[] paramKeys, string[] paramValues) { Type t = Type.GetType(protectionProviderType, true); if (!typeof(ProtectedConfigurationProvider).IsAssignableFrom(t)) { throw new Exception(SR.GetString(SR.WrongType_of_Protected_provider)); } ProtectedConfigurationProvider provider = (ProtectedConfigurationProvider)Activator.CreateInstance(t); NameValueCollection cloneParams = new NameValueCollection(paramKeys.Length); XmlNode node; for(int iter=0; iter
Link Menu
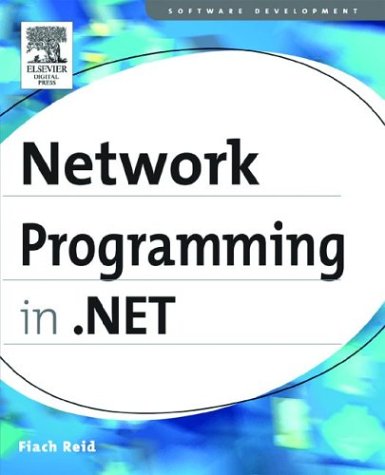
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GlyphsSerializer.cs
- XPathNodePointer.cs
- TypeDependencyAttribute.cs
- FixedPageProcessor.cs
- SmtpSection.cs
- ConnectionProviderAttribute.cs
- ToolboxItemAttribute.cs
- QueuedDeliveryRequirementsMode.cs
- BinarySerializer.cs
- Stream.cs
- ListSortDescriptionCollection.cs
- BaseDataList.cs
- ComponentConverter.cs
- DefaultTextStore.cs
- InstanceKeyView.cs
- NetSectionGroup.cs
- SecurityAlgorithmSuiteConverter.cs
- coordinatorfactory.cs
- Thread.cs
- NameSpaceEvent.cs
- FaultDescriptionCollection.cs
- KeyTimeConverter.cs
- SuppressIldasmAttribute.cs
- XmlSchemaSubstitutionGroup.cs
- SymbolEqualComparer.cs
- TableRow.cs
- ProcessModule.cs
- DisplayNameAttribute.cs
- PermissionSet.cs
- ServiceModelExtensionCollectionElement.cs
- CacheChildrenQuery.cs
- StyleHelper.cs
- RelatedImageListAttribute.cs
- XmlSchemaGroup.cs
- KeyEvent.cs
- PermissionAttributes.cs
- HtmlInputImage.cs
- DurableEnlistmentState.cs
- AuthenticationModuleElement.cs
- CompilerGlobalScopeAttribute.cs
- UInt16Storage.cs
- ProtocolProfile.cs
- WindowsListViewItemCheckBox.cs
- bindurihelper.cs
- PolyQuadraticBezierSegment.cs
- CssTextWriter.cs
- BuildProvider.cs
- SortedDictionary.cs
- ResourceDescriptionAttribute.cs
- RegionInfo.cs
- RepeatButton.cs
- TypeConverterHelper.cs
- DataFieldConverter.cs
- AspProxy.cs
- Material.cs
- ResourceDescriptionAttribute.cs
- Opcode.cs
- PropertyReferenceSerializer.cs
- DocumentPageHost.cs
- ImageMapEventArgs.cs
- PropertyPathWorker.cs
- Misc.cs
- DataSysAttribute.cs
- KeyFrames.cs
- TraceHandler.cs
- ScrollProviderWrapper.cs
- MonikerSyntaxException.cs
- FlowLayoutPanel.cs
- SerializationStore.cs
- RegexBoyerMoore.cs
- Stream.cs
- OpenTypeCommon.cs
- TextViewBase.cs
- StrokeNodeOperations.cs
- StylusPointProperties.cs
- peernodestatemanager.cs
- StyleHelper.cs
- HttpRequestCacheValidator.cs
- CompilerErrorCollection.cs
- DeclaredTypeElementCollection.cs
- AppDomainInfo.cs
- HostedTcpTransportManager.cs
- ViewLoader.cs
- IgnorePropertiesAttribute.cs
- ModifierKeysConverter.cs
- ContextDataSourceContextData.cs
- IDispatchConstantAttribute.cs
- RtfControls.cs
- ColumnResizeAdorner.cs
- XPathSelfQuery.cs
- Shape.cs
- ListBox.cs
- CreateWorkflowOwnerCommand.cs
- SqlBulkCopy.cs
- PaintValueEventArgs.cs
- HideDisabledControlAdapter.cs
- ColumnResizeAdorner.cs
- CryptoApi.cs
- CertificateManager.cs
- SecurityIdentifierElementCollection.cs