Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / TextFormatting / ThousandthOfEmRealPoints.cs / 1 / ThousandthOfEmRealPoints.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: ThousandthOfEmRealPoints class // // History: // 1/13/2005: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// This is a fixed-size implementation of IList<Point>. It is aimed to reduce the double values storage /// while providing enough precision for glyph run operations. Current usage pattern suggests that there is no /// need to support resizing functionality (i.e. Add(), Insert(), Remove(), RemoveAt()). /// /// For each point being stored, it will store the X and Y coordinates of the point into two seperate ThousandthOfEmRealDoubles, /// which scales double to 16-bit integer if possible. /// /// internal sealed class ThousandthOfEmRealPoints : IList{ //---------------------------------- // Constructor //---------------------------------- internal ThousandthOfEmRealPoints( double emSize, int capacity ) { Debug.Assert(capacity >= 0); InitArrays(emSize, capacity); } internal ThousandthOfEmRealPoints( double emSize, IList pointValues ) { Debug.Assert(pointValues != null); InitArrays(emSize, pointValues.Count); // do the setting for (int i = 0; i < Count; i++) { _xArray[i] = pointValues[i].X; _yArray[i] = pointValues[i].Y; } } //------------------------------------- // Internal properties //------------------------------------- public int Count { get { return _xArray.Count; } } public bool IsReadOnly { get { return false; } } public Point this[int index] { get { // underlying array does boundary check return new Point(_xArray[index], _yArray[index]); } set { // underlying array does boundary check _xArray[index] = value.X; _yArray[index] = value.Y; } } //------------------------------------ // internal methods //------------------------------------ public int IndexOf(Point item) { // linear search for (int i = 0; i < Count; i++) { if (_xArray[i] == item.X && _yArray[i] == item.Y) { return i; } } return -1; } public void Clear() { _xArray.Clear(); _yArray.Clear(); } public bool Contains(Point item) { return IndexOf(item) >= 0; } public void CopyTo(Point[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } public IEnumerator GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } public void Add(Point value) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public bool Remove(Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } //--------------------------------------------- // Private methods //--------------------------------------------- private void InitArrays(double emSize, int capacity) { _xArray = new ThousandthOfEmRealDoubles(emSize, capacity); _yArray = new ThousandthOfEmRealDoubles(emSize, capacity); } //---------------------------------------- // Private members //---------------------------------------- private ThousandthOfEmRealDoubles _xArray; // scaled double array for X coordinates private ThousandthOfEmRealDoubles _yArray; // scaled double array for Y coordinates } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: ThousandthOfEmRealPoints class // // History: // 1/13/2005: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// This is a fixed-size implementation of IList<Point>. It is aimed to reduce the double values storage /// while providing enough precision for glyph run operations. Current usage pattern suggests that there is no /// need to support resizing functionality (i.e. Add(), Insert(), Remove(), RemoveAt()). /// /// For each point being stored, it will store the X and Y coordinates of the point into two seperate ThousandthOfEmRealDoubles, /// which scales double to 16-bit integer if possible. /// /// internal sealed class ThousandthOfEmRealPoints : IList{ //---------------------------------- // Constructor //---------------------------------- internal ThousandthOfEmRealPoints( double emSize, int capacity ) { Debug.Assert(capacity >= 0); InitArrays(emSize, capacity); } internal ThousandthOfEmRealPoints( double emSize, IList pointValues ) { Debug.Assert(pointValues != null); InitArrays(emSize, pointValues.Count); // do the setting for (int i = 0; i < Count; i++) { _xArray[i] = pointValues[i].X; _yArray[i] = pointValues[i].Y; } } //------------------------------------- // Internal properties //------------------------------------- public int Count { get { return _xArray.Count; } } public bool IsReadOnly { get { return false; } } public Point this[int index] { get { // underlying array does boundary check return new Point(_xArray[index], _yArray[index]); } set { // underlying array does boundary check _xArray[index] = value.X; _yArray[index] = value.Y; } } //------------------------------------ // internal methods //------------------------------------ public int IndexOf(Point item) { // linear search for (int i = 0; i < Count; i++) { if (_xArray[i] == item.X && _yArray[i] == item.Y) { return i; } } return -1; } public void Clear() { _xArray.Clear(); _yArray.Clear(); } public bool Contains(Point item) { return IndexOf(item) >= 0; } public void CopyTo(Point[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } public IEnumerator GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } public void Add(Point value) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public bool Remove(Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } //--------------------------------------------- // Private methods //--------------------------------------------- private void InitArrays(double emSize, int capacity) { _xArray = new ThousandthOfEmRealDoubles(emSize, capacity); _yArray = new ThousandthOfEmRealDoubles(emSize, capacity); } //---------------------------------------- // Private members //---------------------------------------- private ThousandthOfEmRealDoubles _xArray; // scaled double array for X coordinates private ThousandthOfEmRealDoubles _yArray; // scaled double array for Y coordinates } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
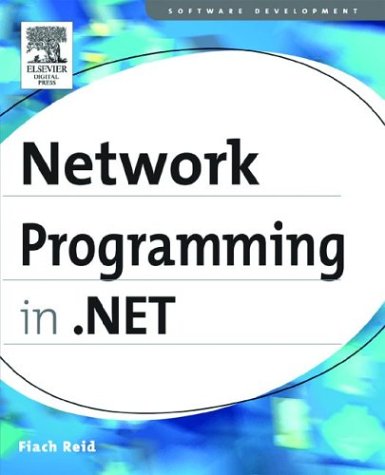
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintDialogException.cs
- ManualResetEvent.cs
- EditableLabelControl.cs
- DBConnectionString.cs
- MailDefinition.cs
- DataGridComboBoxColumn.cs
- _Rfc2616CacheValidators.cs
- cache.cs
- DeviceContexts.cs
- DoubleLinkList.cs
- FormatStringEditor.cs
- HostingPreferredMapPath.cs
- TextViewDesigner.cs
- SqlCacheDependencySection.cs
- DesignerLoader.cs
- GroupBox.cs
- DateTimeConstantAttribute.cs
- Rect3DConverter.cs
- ScriptResourceAttribute.cs
- MulticastIPAddressInformationCollection.cs
- Visual3D.cs
- CheckBox.cs
- ComplexBindingPropertiesAttribute.cs
- RowTypeElement.cs
- ComponentResourceKey.cs
- SystemWebCachingSectionGroup.cs
- WindowsSpinner.cs
- SpeakInfo.cs
- ThrowHelper.cs
- ReliableSessionBindingElement.cs
- PagerSettings.cs
- CollectionBase.cs
- EndPoint.cs
- ObjectReferenceStack.cs
- RowParagraph.cs
- Freezable.cs
- PngBitmapEncoder.cs
- ControlCachePolicy.cs
- SchemaRegistration.cs
- Effect.cs
- ComponentResourceKeyConverter.cs
- SchemaNamespaceManager.cs
- Int32KeyFrameCollection.cs
- UnmanagedMemoryStream.cs
- MultipartContentParser.cs
- RawTextInputReport.cs
- DataRelationPropertyDescriptor.cs
- DesignerForm.cs
- StreamBodyWriter.cs
- TextFormatterImp.cs
- DataSet.cs
- TextLineResult.cs
- XPathScanner.cs
- ConfigurationValidatorAttribute.cs
- ProtocolViolationException.cs
- ProfileServiceManager.cs
- ExecutionScope.cs
- _FixedSizeReader.cs
- SqlRecordBuffer.cs
- Marshal.cs
- HandlerBase.cs
- FixedDocumentPaginator.cs
- XmlSchemaProviderAttribute.cs
- MarginsConverter.cs
- compensatingcollection.cs
- RegistryHandle.cs
- Page.cs
- EntityTypeEmitter.cs
- CryptoApi.cs
- MenuAutomationPeer.cs
- CommentEmitter.cs
- UserPreferenceChangedEventArgs.cs
- CorrelationValidator.cs
- AuthenticationSection.cs
- FrameworkElement.cs
- AttributeEmitter.cs
- TypeDescriptionProviderAttribute.cs
- ValidationPropertyAttribute.cs
- MetadataPropertyAttribute.cs
- Pair.cs
- AggregatePushdown.cs
- OptionalColumn.cs
- _DisconnectOverlappedAsyncResult.cs
- SplitterPanel.cs
- QilReplaceVisitor.cs
- ExpressionPrefixAttribute.cs
- MetafileHeaderEmf.cs
- WebPartCatalogCloseVerb.cs
- SmtpFailedRecipientsException.cs
- NetNamedPipeSecurityMode.cs
- ButtonChrome.cs
- ConfigXmlCDataSection.cs
- sqlmetadatafactory.cs
- TextEditorParagraphs.cs
- ItemsPanelTemplate.cs
- RequestUriProcessor.cs
- DataSourceView.cs
- Trace.cs
- FormClosedEvent.cs
- TextDataBindingHandler.cs