Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / HtmlToClrEventProxy.cs / 1 / HtmlToClrEventProxy.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Globalization; using System.Collections; using System.Reflection; namespace System.Windows.Forms { ////// This class is here for IHTML*3.AttachHandler style eventing. /// We need a way of routing requests for DISPID(0) to a particular CLR event without creating /// a public class. In order to accomplish this we implement IReflect and handle InvokeMethod /// to call back on a CLR event handler. /// internal class HtmlToClrEventProxy : IReflect { private EventHandler eventHandler; private IReflect typeIReflectImplementation; private object sender = null; private string eventName; public HtmlToClrEventProxy(object sender, string eventName, EventHandler eventHandler) { this.eventHandler = eventHandler; this.eventName = eventName; Type htmlToClrEventProxyType = typeof(HtmlToClrEventProxy); typeIReflectImplementation = htmlToClrEventProxyType as IReflect; } public string EventName { get { return eventName; } } [DispId(0)] public void OnHtmlEvent() { InvokeClrEvent(); } private void InvokeClrEvent() { if (eventHandler != null) { eventHandler(sender, EventArgs.Empty); } } #region IReflect Type IReflect.UnderlyingSystemType { get { return typeIReflectImplementation.UnderlyingSystemType; } } // Methods System.Reflection.FieldInfo IReflect.GetField(string name, System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetField(name, bindingAttr); } System.Reflection.FieldInfo[] IReflect.GetFields(System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetFields(bindingAttr); } System.Reflection.MemberInfo[] IReflect.GetMember(string name, System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetMember(name, bindingAttr); } System.Reflection.MemberInfo[] IReflect.GetMembers(System.Reflection.BindingFlags bindingAttr){ return typeIReflectImplementation.GetMembers(bindingAttr); } System.Reflection.MethodInfo IReflect.GetMethod(string name, System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetMethod(name, bindingAttr); } System.Reflection.MethodInfo IReflect.GetMethod(string name, System.Reflection.BindingFlags bindingAttr, System.Reflection.Binder binder, Type[] types, System.Reflection.ParameterModifier[] modifiers) { return typeIReflectImplementation.GetMethod(name, bindingAttr, binder, types, modifiers); } System.Reflection.MethodInfo[] IReflect.GetMethods(System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetMethods(bindingAttr); } System.Reflection.PropertyInfo[] IReflect.GetProperties(System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetProperties(bindingAttr); } System.Reflection.PropertyInfo IReflect.GetProperty(string name, System.Reflection.BindingFlags bindingAttr) { return typeIReflectImplementation.GetProperty(name, bindingAttr); } System.Reflection.PropertyInfo IReflect.GetProperty(string name, System.Reflection.BindingFlags bindingAttr, System.Reflection.Binder binder, Type returnType, Type[] types, System.Reflection.ParameterModifier[] modifiers) { return typeIReflectImplementation.GetProperty(name, bindingAttr, binder, returnType, types, modifiers); } // InvokeMember: // If we get a call for DISPID=0, fire the CLR event. // object IReflect.InvokeMember(string name, System.Reflection.BindingFlags invokeAttr, System.Reflection.Binder binder, object target, object[] args, System.Reflection.ParameterModifier[] modifiers, System.Globalization.CultureInfo culture, string[] namedParameters) { // if (name == "[DISPID=0]") { // we know we're getting called back to fire the event - translate this now into a CLR event. OnHtmlEvent(); // since there's no return value for void, return null. return null; } else { return typeIReflectImplementation.InvokeMember(name, invokeAttr, binder, target, args, modifiers, culture, namedParameters); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
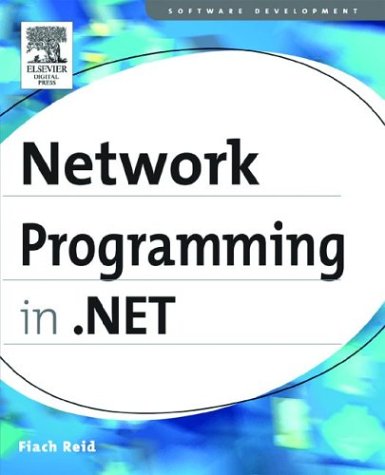
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SamlDoNotCacheCondition.cs
- DockProviderWrapper.cs
- SymbolEqualComparer.cs
- SchemaCollectionPreprocessor.cs
- SqlProviderServices.cs
- StyleXamlParser.cs
- ListView.cs
- COM2ExtendedBrowsingHandler.cs
- CqlGenerator.cs
- BuildDependencySet.cs
- TcpAppDomainProtocolHandler.cs
- SynchronizationFilter.cs
- GeneralTransform3DGroup.cs
- DataSetSchema.cs
- WindowsFont.cs
- ChannelManager.cs
- RtfControls.cs
- ReferenceEqualityComparer.cs
- MSG.cs
- FontStyles.cs
- NetworkInformationException.cs
- RegisteredExpandoAttribute.cs
- ObjectParameterCollection.cs
- SqlAliaser.cs
- PropertyValueUIItem.cs
- NamespaceExpr.cs
- CssStyleCollection.cs
- TableCellCollection.cs
- Regex.cs
- RemoveStoryboard.cs
- SqlDelegatedTransaction.cs
- Schema.cs
- HttpRequestMessageProperty.cs
- CompensationExtension.cs
- ConnectivityStatus.cs
- DbProviderFactories.cs
- CapabilitiesSection.cs
- XPathAxisIterator.cs
- dataprotectionpermission.cs
- ControlParameter.cs
- PnrpPeerResolverElement.cs
- SingleKeyFrameCollection.cs
- QueryOutputWriter.cs
- _NestedSingleAsyncResult.cs
- ComplexBindingPropertiesAttribute.cs
- TreeViewDataItemAutomationPeer.cs
- PagerSettings.cs
- XmlSchemaAnyAttribute.cs
- GeometryModel3D.cs
- AsymmetricSecurityBindingElement.cs
- SafeNativeMemoryHandle.cs
- SessionState.cs
- basevalidator.cs
- BaseDataListDesigner.cs
- AddInProcess.cs
- ProgressChangedEventArgs.cs
- CancellationTokenSource.cs
- Int64AnimationBase.cs
- ToolStripRenderEventArgs.cs
- SourceSwitch.cs
- ReadOnlyMetadataCollection.cs
- ReadContentAsBinaryHelper.cs
- OutputCacheSettingsSection.cs
- KernelTypeValidation.cs
- SqlDataSourceConfigureSelectPanel.cs
- Journaling.cs
- HopperCache.cs
- SerializationStore.cs
- RowUpdatedEventArgs.cs
- InfocardClientCredentials.cs
- Model3DGroup.cs
- SqlCharStream.cs
- lengthconverter.cs
- CalendarKeyboardHelper.cs
- ObjectNotFoundException.cs
- TypedLocationWrapper.cs
- ModelVisual3D.cs
- isolationinterop.cs
- login.cs
- CodeGroup.cs
- AggregateException.cs
- InputQueue.cs
- PackageFilter.cs
- SupportsPreviewControlAttribute.cs
- TextTreeNode.cs
- FlowDocumentReaderAutomationPeer.cs
- FixedHyperLink.cs
- GridItemCollection.cs
- SimpleTypeResolver.cs
- SessionStateSection.cs
- DataGridLinkButton.cs
- NegationPusher.cs
- StorageTypeMapping.cs
- MailMessage.cs
- Stack.cs
- SQLDateTimeStorage.cs
- DefaultBindingPropertyAttribute.cs
- RectAnimationClockResource.cs
- HandlerBase.cs
- OleDbException.cs