Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Runtime / Serialization / SerializationEventsCache.cs / 1 / SerializationEventsCache.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SerializationEventsCache ** ** ** Purpose: Caches the various serialization events such as On(De)Seriliz(ed)ing ** ** ============================================================*/ namespace System.Runtime.Serialization { using System; using System.Collections; using System.Collections.Generic; using System.Reflection; using System.Reflection.Cache; using System.Globalization; internal class SerializationEvents { private Listm_OnSerializingMethods = null; private List m_OnSerializedMethods = null; private List m_OnDeserializingMethods = null; private List m_OnDeserializedMethods = null; private List GetMethodsWithAttribute(Type attribute, Type t) { List mi = new List (); Type baseType = t; // Traverse the hierarchy to find all methods with the particular attribute while (baseType != null && baseType != typeof(Object)) { RuntimeType rt = (RuntimeType)baseType; // Get all methods which are declared on this type, instance and public or nonpublic MethodInfo[] mis = baseType.GetMethods(BindingFlags.DeclaredOnly | BindingFlags.Instance | BindingFlags.NonPublic | BindingFlags.Public); #if _DEBUG bool found = false; #endif foreach(MethodInfo m in mis) { // For each method find if attribute is present, the return type is void and the method is not virtual if (m.IsDefined(attribute, false)) { #if _DEBUG BCLDebug.Assert(m.ReturnType == typeof(void) && !m.IsVirtual, "serialization events methods cannot be virtual and need to have void return"); ParameterInfo[] paramInfo = m.GetParameters(); // Only add it if this method has one parameter of type StreamingContext if (paramInfo.Length == 1 && paramInfo[0].ParameterType == typeof(StreamingContext)) { if (found) BCLDebug.Assert(false, "Mutliple methods with same serialization attribute"); #endif mi.Add(m); #if _DEBUG found = true; } else BCLDebug.Assert(false, "Incorrect serialization event signature"); #endif } } #if _DEBUG found = false; #endif baseType = baseType.BaseType; } mi.Reverse(); // We should invoke the methods starting from base return (mi.Count == 0) ? null : mi; } internal SerializationEvents(Type t) { // Initialize all events m_OnSerializingMethods = GetMethodsWithAttribute(typeof(OnSerializingAttribute), t); m_OnSerializedMethods = GetMethodsWithAttribute(typeof(OnSerializedAttribute), t); m_OnDeserializingMethods = GetMethodsWithAttribute(typeof(OnDeserializingAttribute), t); m_OnDeserializedMethods = GetMethodsWithAttribute(typeof(OnDeserializedAttribute), t); } internal bool HasOnSerializingEvents { get { return m_OnSerializingMethods != null || m_OnSerializedMethods != null; } } internal void InvokeOnSerializing(Object obj, StreamingContext context) { BCLDebug.Assert(obj != null, "object should have been initialized"); // Invoke all OnSerializingMethods if (m_OnSerializingMethods != null) { Object[] p = new Object[] {context}; SerializationEventHandler handler = null; foreach(MethodInfo m in m_OnSerializingMethods) { SerializationEventHandler onSerializing = (SerializationEventHandler)Delegate.InternalCreateDelegate(typeof(SerializationEventHandler), obj, m); handler = (SerializationEventHandler)Delegate.Combine(handler, onSerializing); } handler(context); } } internal void InvokeOnDeserializing(Object obj, StreamingContext context) { BCLDebug.Assert(obj != null, "object should have been initialized"); // Invoke all OnDeserializingMethods if (m_OnDeserializingMethods != null) { Object[] p = new Object[] {context}; SerializationEventHandler handler = null; foreach(MethodInfo m in m_OnDeserializingMethods) { SerializationEventHandler onDeserializing = (SerializationEventHandler)Delegate.InternalCreateDelegate(typeof(SerializationEventHandler), obj, m); handler = (SerializationEventHandler)Delegate.Combine(handler, onDeserializing); } handler(context); } } internal void InvokeOnDeserialized(Object obj, StreamingContext context) { BCLDebug.Assert(obj != null, "object should have been initialized"); // Invoke all OnDeserializingMethods if (m_OnDeserializedMethods != null) { Object[] p = new Object[] {context}; SerializationEventHandler handler = null; foreach(MethodInfo m in m_OnDeserializedMethods) { SerializationEventHandler onDeserialized = (SerializationEventHandler)Delegate.InternalCreateDelegate(typeof(SerializationEventHandler), obj, m); handler = (SerializationEventHandler)Delegate.Combine(handler, onDeserialized); } handler(context); } } internal SerializationEventHandler AddOnSerialized(Object obj, SerializationEventHandler handler) { // Add all OnSerialized methods to a delegate if (m_OnSerializedMethods != null) { foreach(MethodInfo m in m_OnSerializedMethods) { SerializationEventHandler onSerialized = (SerializationEventHandler)Delegate.InternalCreateDelegate(typeof(SerializationEventHandler), obj, m); handler = (SerializationEventHandler)Delegate.Combine(handler, onSerialized); } } return handler; } internal SerializationEventHandler AddOnDeserialized(Object obj, SerializationEventHandler handler) { // Add all OnDeserialized methods to a delegate if (m_OnDeserializedMethods != null) { foreach(MethodInfo m in m_OnDeserializedMethods) { SerializationEventHandler onDeserialized = (SerializationEventHandler)Delegate.InternalCreateDelegate(typeof(SerializationEventHandler), obj, m); handler = (SerializationEventHandler)Delegate.Combine(handler, onDeserialized); } } return handler; } } internal static class SerializationEventsCache { private static Hashtable cache = new Hashtable(); internal static SerializationEvents GetSerializationEventsForType(Type t) { SerializationEvents events; if ((events = (SerializationEvents)cache[t]) == null) { events = new SerializationEvents(t); cache[t] = events; // Add this to the cache. } return events; } } }
Link Menu
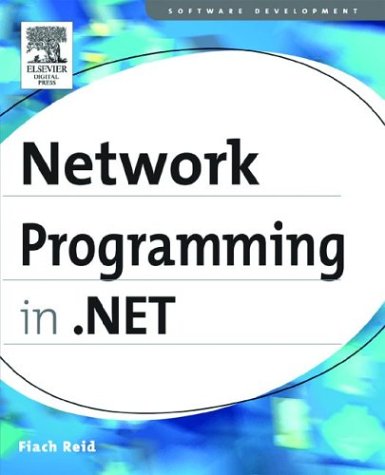
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseDataBoundControl.cs
- ConfigXmlSignificantWhitespace.cs
- Literal.cs
- WindowsTreeView.cs
- FastEncoderStatics.cs
- ScriptControlDescriptor.cs
- XmlDataCollection.cs
- ListViewPagedDataSource.cs
- CircleHotSpot.cs
- TraceHandlerErrorFormatter.cs
- SizeValueSerializer.cs
- Listener.cs
- VisualBasicDesignerHelper.cs
- RefreshPropertiesAttribute.cs
- DescendantBaseQuery.cs
- AutomationIdentifierGuids.cs
- InlineUIContainer.cs
- PKCS1MaskGenerationMethod.cs
- ManagedIStream.cs
- RoutedEventArgs.cs
- DataControlLinkButton.cs
- CommandID.cs
- TerminateDesigner.cs
- DragSelectionMessageFilter.cs
- ComponentResourceManager.cs
- SecurityCriticalDataForSet.cs
- EdmError.cs
- DbConnectionFactory.cs
- WindowsIdentity.cs
- OutOfProcStateClientManager.cs
- SoapAttributeAttribute.cs
- MethodBody.cs
- RightsManagementEncryptedStream.cs
- AspCompat.cs
- CallbackValidatorAttribute.cs
- KeyManager.cs
- ImageList.cs
- XamlTreeBuilder.cs
- TextContainer.cs
- ProfileGroupSettings.cs
- UserUseLicenseDictionaryLoader.cs
- Missing.cs
- EncodingInfo.cs
- TimeoutHelper.cs
- HtmlSelect.cs
- DbConnectionStringCommon.cs
- ArcSegment.cs
- ReversePositionQuery.cs
- CompatibleComparer.cs
- SafeProcessHandle.cs
- TextLine.cs
- _Rfc2616CacheValidators.cs
- MethodResolver.cs
- ShaperBuffers.cs
- DependentList.cs
- WebHeaderCollection.cs
- ComponentResourceKey.cs
- Object.cs
- XsltQilFactory.cs
- ButtonColumn.cs
- SharedPerformanceCounter.cs
- BamlResourceSerializer.cs
- FormView.cs
- TransformGroup.cs
- Span.cs
- TypeSource.cs
- ScriptingSectionGroup.cs
- RoleManagerModule.cs
- InlineCollection.cs
- ContractNamespaceAttribute.cs
- InternalControlCollection.cs
- SHA1CryptoServiceProvider.cs
- MessageUtil.cs
- BitStream.cs
- _RequestCacheProtocol.cs
- DrawingGroupDrawingContext.cs
- SiteMapPath.cs
- HelpExampleGenerator.cs
- CorePropertiesFilter.cs
- GridViewDeletedEventArgs.cs
- AggregateNode.cs
- GestureRecognitionResult.cs
- TransactionsSectionGroup.cs
- PreservationFileWriter.cs
- StackSpiller.Temps.cs
- ComUdtElementCollection.cs
- ServiceModelConfigurationSectionCollection.cs
- AnnotationStore.cs
- AssemblyResourceLoader.cs
- VirtualDirectoryMapping.cs
- MiniLockedBorderGlyph.cs
- UpdateRecord.cs
- LockCookie.cs
- WindowsEditBox.cs
- UnhandledExceptionEventArgs.cs
- NamespaceList.cs
- HtmlFormWrapper.cs
- SQLInt32Storage.cs
- TabletDeviceInfo.cs
- ProxyAttribute.cs