Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / TreeWalker.cs / 1305600 / TreeWalker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TreeWalker class, allows client to walk custom views of // UIAutomation tree. // // History: // 02/05/2004 : BrendanM Created // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// TreeWalker - used to walk over a view of the UIAutomation tree /// #if (INTERNAL_COMPILE) internal sealed class TreeWalker #else public sealed class TreeWalker #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Create a tree walker that can be used to walk a specified /// view of the UIAutomation tree. /// /// Condition defining the view - nodes that do not satisfy this condition are skipped over public TreeWalker(Condition condition) { Misc.ValidateArgumentNonNull(condition, "condition"); _condition = condition; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ////// Predefined TreeWalker for walking the Raw view of the UIAutomation tree /// public static readonly TreeWalker RawViewWalker = new TreeWalker(Automation.RawViewCondition); ////// Predefined TreeWalker for walking the Control view of the UIAutomation tree /// public static readonly TreeWalker ControlViewWalker = new TreeWalker(Automation.ControlViewCondition); ////// Predefined TreeWalker for walking the Content view of the UIAutomation tree /// public static readonly TreeWalker ContentViewWalker = new TreeWalker(Automation.ContentViewCondition); #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Get the parent of the specified element, in the current view /// /// element to get the parent of ///The parent of the specified element; can be null if /// specified element was the root element ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetParent(AutomationElement element) { Misc.ValidateArgumentNonNull(element, "element"); return element.Navigate(NavigateDirection.Parent, _condition, null); } ////// Get the first child of the specified element, in the current view /// /// element to get the first child of ///The frst child of the specified element - or null if /// the specified element has no children ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetFirstChild(AutomationElement element) { Misc.ValidateArgumentNonNull(element, "element"); return element.Navigate(NavigateDirection.FirstChild, _condition, null); } ////// Get the last child of the specified element, in the current view /// /// element to get the last child of ///The last child of the specified element - or null if /// the specified element has no children ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetLastChild(AutomationElement element) { Misc.ValidateArgumentNonNull(element, "element"); return element.Navigate(NavigateDirection.LastChild, _condition, null); } ////// Get the next sibling of the specified element, in the current view /// /// element to get the next sibling of ///The next sibling of the specified element - or null if the /// specified element has no next sibling ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetNextSibling(AutomationElement element) { Misc.ValidateArgumentNonNull(element, "element"); return element.Navigate(NavigateDirection.NextSibling, _condition, null); } ////// Get the previous sibling of the specified element, in the current view /// /// element to get the previous sibling of ///The previous sibling of the specified element - or null if the /// specified element has no previous sibling ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetPreviousSibling(AutomationElement element) { Misc.ValidateArgumentNonNull(element, "element"); return element.Navigate(NavigateDirection.PreviousSibling, _condition, null); } ////// Return the element or the nearest ancestor which is present in /// the view of the tree used by this treewalker /// /// element to normalize ///The element or the nearest ancestor which satisfies the /// condition used by this TreeWalker ////// This method starts at the specified element and walks up the /// tree until it finds an element that satisfies the TreeWalker's /// condition. /// /// If the passed-in element itself satsifies the condition, it is /// returned as-is. /// /// If the process of walking up the tree hits the root node, then /// the root node is returned, regardless of whether it satisfies /// the condition or not. /// public AutomationElement Normalize(AutomationElement element) { Misc.ValidateArgumentNonNull(element, "element"); return element.Normalize(_condition, null); } ////// Get the parent of the specified element, in the current view, /// prefetching properties /// /// element to get the parent of /// CacheRequest specifying information to be prefetched ///The parent of the specified element; can be null if /// specified element was the root element ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetParent(AutomationElement element, CacheRequest request) { Misc.ValidateArgumentNonNull(element, "element"); Misc.ValidateArgumentNonNull(request, "request"); return element.Navigate(NavigateDirection.Parent, _condition, request); } ////// Get the first child of the specified element, in the current view, /// prefetching properties /// /// element to get the first child of /// CacheRequest specifying information to be prefetched ///The frst child of the specified element - or null if /// the specified element has no children ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetFirstChild(AutomationElement element, CacheRequest request) { Misc.ValidateArgumentNonNull(element, "element"); Misc.ValidateArgumentNonNull(request, "request"); return element.Navigate(NavigateDirection.FirstChild, _condition, request); } ////// Get the last child of the specified element, in the current view, /// prefetching properties /// /// element to get the last child of /// CacheRequest specifying information to be prefetched ///The last child of the specified element - or null if /// the specified element has no children ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetLastChild(AutomationElement element, CacheRequest request) { Misc.ValidateArgumentNonNull(element, "element"); Misc.ValidateArgumentNonNull(request, "request"); return element.Navigate(NavigateDirection.LastChild, _condition, request); } ////// Get the next sibling of the specified element, in the current view, /// prefetching properties /// /// element to get the next sibling of /// CacheRequest specifying information to be prefetched ///The next sibling of the specified element - or null if the /// specified element has no next sibling ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetNextSibling(AutomationElement element, CacheRequest request) { Misc.ValidateArgumentNonNull(element, "element"); Misc.ValidateArgumentNonNull(request, "request"); return element.Navigate(NavigateDirection.NextSibling, _condition, request); } ////// Get the previous sibling of the specified element, in the current view, /// prefetching properties /// /// element to get the previous sibling of /// CacheRequest specifying information to be prefetched ///The previous sibling of the specified element - or null if the /// specified element has no previous sibling ///The view used is determined by the condition passed to /// the constructor - elements that do not satisfy that condition /// are skipped over public AutomationElement GetPreviousSibling(AutomationElement element, CacheRequest request) { Misc.ValidateArgumentNonNull(element, "element"); Misc.ValidateArgumentNonNull(request, "request"); return element.Navigate(NavigateDirection.PreviousSibling, _condition, request); } ////// Return the element or the nearest ancestor which is present in /// the view of the tree used by this treewalker, prefetching properties /// for the returned node /// /// element to normalize /// CacheRequest specifying information to be prefetched ///The element or the nearest ancestor which satisfies the /// condition used by this TreeWalker ////// This method starts at the specified element and walks up the /// tree until it finds an element that satisfies the TreeWalker's /// condition. /// /// If the passed-in element itself satsifies the condition, it is /// returned as-is. /// /// If the process of walking up the tree hits the root node, then /// the root node is returned, regardless of whether it satisfies /// the condition or not. /// public AutomationElement Normalize(AutomationElement element, CacheRequest request) { Misc.ValidateArgumentNonNull(element, "element"); Misc.ValidateArgumentNonNull(request, "request"); return element.Normalize(_condition, request); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Returns the condition used by this TreeWalker. The TreeWalker /// skips over nodes that do not satisfy the condition. /// public Condition Condition { get { return _condition; } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private Condition _condition; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
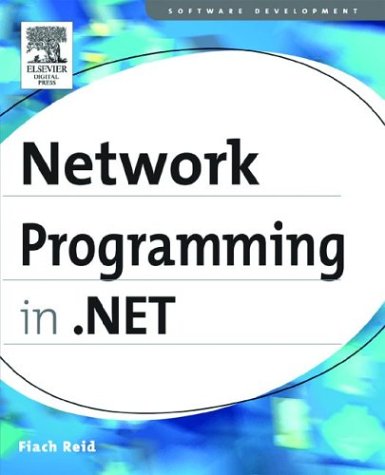
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewAutoSizeColumnModeEventArgs.cs
- GridPattern.cs
- InsufficientMemoryException.cs
- RawMouseInputReport.cs
- NativeMethodsOther.cs
- ThreadAbortException.cs
- input.cs
- NetworkInformationPermission.cs
- SafeRegistryKey.cs
- ExceptionHandlersDesigner.cs
- GridItemPatternIdentifiers.cs
- MemoryRecordBuffer.cs
- ApplicationContext.cs
- ColorAnimation.cs
- SchemaConstraints.cs
- TileBrush.cs
- ImageListUtils.cs
- TypeSystemHelpers.cs
- SafeProcessHandle.cs
- Crypto.cs
- SimpleType.cs
- ByteStreamGeometryContext.cs
- MaskedTextBox.cs
- CriticalFinalizerObject.cs
- SymDocumentType.cs
- EdgeModeValidation.cs
- XmlHelper.cs
- StringComparer.cs
- OdbcConnectionHandle.cs
- AsyncOperation.cs
- XPathDescendantIterator.cs
- DataGridAddNewRow.cs
- PackWebRequestFactory.cs
- HttpUnhandledOperationInvoker.cs
- DmlSqlGenerator.cs
- PKCS1MaskGenerationMethod.cs
- CompiledXpathExpr.cs
- GridViewColumnCollectionChangedEventArgs.cs
- EmptyQuery.cs
- TableCellCollection.cs
- ObjectTag.cs
- SafeEventLogWriteHandle.cs
- TreeViewImageKeyConverter.cs
- EventLogInformation.cs
- OpenTypeLayoutCache.cs
- RectAnimation.cs
- Delegate.cs
- RefExpr.cs
- DataRowCollection.cs
- EventLog.cs
- HttpCookieCollection.cs
- AssemblyHelper.cs
- MediaTimeline.cs
- InteropExecutor.cs
- ReadonlyMessageFilter.cs
- RecommendedAsConfigurableAttribute.cs
- Update.cs
- EmbossBitmapEffect.cs
- UserControlCodeDomTreeGenerator.cs
- BitmapDownload.cs
- MimeWriter.cs
- SqlExpander.cs
- EntityKey.cs
- StrokeNode.cs
- XpsManager.cs
- WSSecurityPolicy12.cs
- ManagedIStream.cs
- UnsafeCollabNativeMethods.cs
- SystemIcons.cs
- DbParameterCollection.cs
- CursorConverter.cs
- TrackBar.cs
- FlagsAttribute.cs
- ObjectReferenceStack.cs
- DesignBindingEditor.cs
- ResourcesChangeInfo.cs
- HwndProxyElementProvider.cs
- DataGridViewBindingCompleteEventArgs.cs
- SystemGatewayIPAddressInformation.cs
- FrameworkContentElementAutomationPeer.cs
- BaseTemplateBuildProvider.cs
- CharacterString.cs
- TrackingDataItemValue.cs
- DataGridViewCellCollection.cs
- OraclePermission.cs
- RuntimeCompatibilityAttribute.cs
- BuildTopDownAttribute.cs
- X509ChainElement.cs
- UInt64Converter.cs
- ObjectNavigationPropertyMapping.cs
- NamespaceInfo.cs
- OracleSqlParser.cs
- TraceHandler.cs
- DataControlPagerLinkButton.cs
- TreeView.cs
- SqlGenerator.cs
- CodeExpressionStatement.cs
- NonVisualControlAttribute.cs
- ConstNode.cs
- XmlElement.cs