Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / ContainerTracking.cs / 1305600 / ContainerTracking.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Input; namespace System.Windows.Controls { ////// Represents a node in a linked list used to track active containers. /// Containers should instantiate and references these. /// Parents hold onto the linked list. /// /// The list is iterated in order to call a variety of methods on containers /// in response to changes on the parent. /// internal class ContainerTracking{ internal ContainerTracking(T container) { _container = container; } /// /// The row container that this object represents. /// internal T Container { get { return _container; } } ////// The next node in the list. /// internal ContainerTrackingNext { get { return _next; } } /// /// The previous node in the list. /// internal ContainerTrackingPrevious { get { return _previous; } } /// /// Adds this tracker to the list of active containers. /// /// The root of the list. internal void StartTracking(ref ContainerTrackingroot) { // Add the node to the root if (root != null) { root._previous = this; } _next = root; root = this; } /// /// Removes this tracker from the list of active containers. /// /// The root of the list. internal void StopTracking(ref ContainerTrackingroot) { // Unhook the node from the list if (_previous != null) { _previous._next = _next; } if (_next != null) { _next._previous = _previous; } // Update the root reference if (root == this) { root = _next; } // Clear the node's references _previous = null; _next = null; } #region Debugging Helpers /// /// Asserts that the container represented by this tracker is in the list represented by the given root. /// [Conditional("DEBUG")] internal void Debug_AssertIsInList(ContainerTrackingroot) { #if DEBUG Debug.Assert(IsInList(root), "This container should be in the tracking list."); #endif } /// /// Asserts that the container represented by this tracker is not in the list represented by the given root. /// [Conditional("DEBUG")] internal void Debug_AssertNotInList(ContainerTrackingroot) { #if DEBUG Debug.Assert(!IsInList(root), "This container shouldn't be in our tracking list"); #endif } #if DEBUG /// /// Checks that this tracker is present in the list starting with root. It's a linear walk through the list, so should be used /// mostly for debugging /// private bool IsInList(ContainerTrackingroot) { ContainerTracking node = root; while (node != null) { if (node == this) { return true; } node = node._next; } return false; } #endif #endregion private T _container; private ContainerTracking _next; private ContainerTracking _previous; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
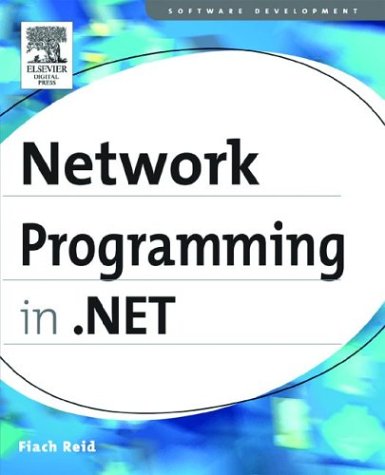
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeUtils.cs
- DataGridHeaderBorder.cs
- ReadOnlyTernaryTree.cs
- ApplicationCommands.cs
- FixedSOMSemanticBox.cs
- ThemeableAttribute.cs
- WebPartUserCapability.cs
- LineBreak.cs
- ApplicationSecurityManager.cs
- initElementDictionary.cs
- MembershipSection.cs
- StorageBasedPackageProperties.cs
- PictureBox.cs
- AssociationType.cs
- XmlSchemaObjectCollection.cs
- HttpConfigurationContext.cs
- DiagnosticsConfigurationHandler.cs
- CodeCatchClauseCollection.cs
- XmlSchemaProviderAttribute.cs
- WindowHideOrCloseTracker.cs
- SchemaNames.cs
- ConnectionPoint.cs
- HtmlMeta.cs
- SessionPageStateSection.cs
- IERequestCache.cs
- TextMessageEncoder.cs
- TreeNodeBinding.cs
- Metafile.cs
- HtmlControl.cs
- AssemblyHash.cs
- LinearKeyFrames.cs
- PlainXmlWriter.cs
- DateTimeConstantAttribute.cs
- DetailsViewCommandEventArgs.cs
- StatusStrip.cs
- SemanticResultKey.cs
- SafeSecurityHelper.cs
- AVElementHelper.cs
- PropertyValidationContext.cs
- HiddenFieldPageStatePersister.cs
- InheritanceRules.cs
- NoPersistHandle.cs
- ToolStripProgressBar.cs
- __TransparentProxy.cs
- EntityCommand.cs
- XmlDataSourceView.cs
- Separator.cs
- BuildManager.cs
- DataGridColumnHeadersPresenter.cs
- ObjectNavigationPropertyMapping.cs
- CreateUserWizardStep.cs
- EventLevel.cs
- OptionalColumn.cs
- InlineCollection.cs
- IISMapPath.cs
- TreeViewCancelEvent.cs
- Sequence.cs
- CodeAttachEventStatement.cs
- AccessedThroughPropertyAttribute.cs
- GuidelineSet.cs
- FileCodeGroup.cs
- FindCriteriaCD1.cs
- QueueProcessor.cs
- TraceShell.cs
- XmlName.cs
- NestPullup.cs
- RefreshPropertiesAttribute.cs
- Compiler.cs
- PeerNameRecordCollection.cs
- Error.cs
- AssemblyAssociatedContentFileAttribute.cs
- Literal.cs
- InputLanguageEventArgs.cs
- HtmlGenericControl.cs
- XmlSignificantWhitespace.cs
- BooleanConverter.cs
- AuthorizationRule.cs
- SqlColumnizer.cs
- EndOfStreamException.cs
- ConfigXmlSignificantWhitespace.cs
- ContentElementAutomationPeer.cs
- ResourceProperty.cs
- CodeMemberEvent.cs
- SystemTcpStatistics.cs
- DataGridTablesFactory.cs
- ProtocolReflector.cs
- DispatchRuntime.cs
- ReliableChannelFactory.cs
- OleCmdHelper.cs
- NotifyInputEventArgs.cs
- PropertySourceInfo.cs
- EntityFrameworkVersions.cs
- UnhandledExceptionEventArgs.cs
- DynamicQueryableWrapper.cs
- FileUpload.cs
- StatusBarItem.cs
- WebPartMinimizeVerb.cs
- Thread.cs
- ReflectionServiceProvider.cs
- MemberHolder.cs