Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapCodecInfo.cs / 1305600 / BitmapCodecInfo.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: BitmapCodecInfo.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.Windows.Media.Imaging; using System.Text; using MS.Internal.PresentationCore; // SecurityHelper namespace System.Windows.Media.Imaging { #region BitmapCodecInfo ////// Codec info for a given Encoder/Decoder /// public abstract class BitmapCodecInfo { #region Constructors ////// Constructor /// protected BitmapCodecInfo() { } ////// Internal Constructor /// internal BitmapCodecInfo(SafeMILHandle codecInfoHandle) { Debug.Assert(codecInfoHandle != null); _isBuiltIn = true; _codecInfoHandle = codecInfoHandle; } #endregion #region Public Properties ////// Container format /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual Guid ContainerFormat { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); Guid containerFormat; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetContainerFormat( _codecInfoHandle, out containerFormat )); return containerFormat; } } ////// Author /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string Author { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder author = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetAuthor( _codecInfoHandle, 0, author, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { author = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetAuthor( _codecInfoHandle, length, author, out length )); } if (author != null) return author.ToString(); else return String.Empty; } } ////// Version /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual System.Version Version { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder version = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetVersion( _codecInfoHandle, 0, version, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { version = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetVersion( _codecInfoHandle, length, version, out length )); } if (version != null) return new Version(version.ToString()); else return new Version(); } } ////// Spec Version /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual Version SpecificationVersion { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder specVersion = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetSpecVersion( _codecInfoHandle, 0, specVersion, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { specVersion = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetSpecVersion( _codecInfoHandle, length, specVersion, out length )); } if (specVersion != null) return new Version(specVersion.ToString()); else return new Version(); } } ////// Friendly Name /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string FriendlyName { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder friendlyName = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetFriendlyName( _codecInfoHandle, 0, friendlyName, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { friendlyName = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICComponentInfo.GetFriendlyName( _codecInfoHandle, length, friendlyName, out length )); } if (friendlyName != null) return friendlyName.ToString(); else return String.Empty; } } ////// Device Manufacturer /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string DeviceManufacturer { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder deviceManufacturer = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceManufacturer( _codecInfoHandle, 0, deviceManufacturer, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { deviceManufacturer = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceManufacturer( _codecInfoHandle, length, deviceManufacturer, out length )); } if (deviceManufacturer != null) return deviceManufacturer.ToString(); else return String.Empty; } } ////// Device Models /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string DeviceModels { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder deviceModels = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceModels( _codecInfoHandle, 0, deviceModels, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { deviceModels = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetDeviceModels( _codecInfoHandle, length, deviceModels, out length )); } if (deviceModels != null) return deviceModels.ToString(); else return String.Empty; } } ////// Mime types /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string MimeTypes { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder mimeTypes = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetMimeTypes( _codecInfoHandle, 0, mimeTypes, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { mimeTypes = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetMimeTypes( _codecInfoHandle, length, mimeTypes, out length )); } if (mimeTypes != null) return mimeTypes.ToString(); else return String.Empty; } } ////// File extensions /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual string FileExtensions { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); StringBuilder fileExtensions = null; UInt32 length = 0; // Find the length of the string needed HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetFileExtensions( _codecInfoHandle, 0, fileExtensions, out length )); Debug.Assert(length >= 0); // get the string back if (length > 0) { fileExtensions = new StringBuilder((int)length); HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.GetFileExtensions( _codecInfoHandle, length, fileExtensions, out length )); } if (fileExtensions != null) return fileExtensions.ToString(); else return String.Empty; } } ////// Does Support Animation /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsAnimation { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsAnimation; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportAnimation( _codecInfoHandle, out supportsAnimation )); return supportsAnimation; } } ////// Does Support Lossless /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsLossless { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsLossless; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportLossless( _codecInfoHandle, out supportsLossless )); return supportsLossless; } } ////// Does Support Multiple Frames /// ////// Callers must have RegistryPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls unamanged code to retrieve data /// PublicOK - Demands registry permissions /// public virtual bool SupportsMultipleFrames { [SecurityCritical] get { SecurityHelper.DemandRegistryPermission(); EnsureBuiltIn(); bool supportsMultiFrame; HRESULT.Check(UnsafeNativeMethods.WICBitmapCodecInfo.DoesSupportMultiframe( _codecInfoHandle, out supportsMultiFrame )); return supportsMultiFrame; } } #endregion #region Private Methods private void EnsureBuiltIn() { if (!_isBuiltIn) { throw new NotImplementedException(); } } #endregion #region Data Members /// is this a built in codec info? private bool _isBuiltIn; /// Codec info handle SafeMILHandle _codecInfoHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
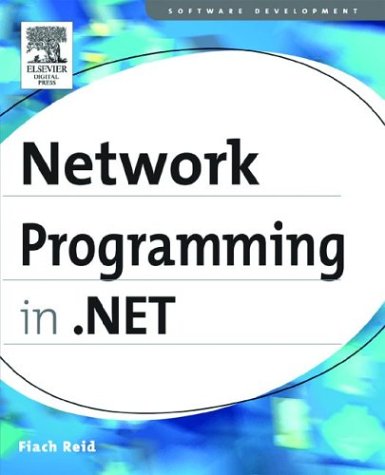
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeMultiPart.cs
- DelegatingConfigHost.cs
- ReliableSessionElement.cs
- SoapMessage.cs
- ExpandCollapseProviderWrapper.cs
- Imaging.cs
- DrawListViewSubItemEventArgs.cs
- DbExpressionRules.cs
- QueryAsyncResult.cs
- SimpleTableProvider.cs
- RouteParser.cs
- StoreContentChangedEventArgs.cs
- Region.cs
- CqlBlock.cs
- InputBinder.cs
- DescendantOverDescendantQuery.cs
- EntityContainerRelationshipSet.cs
- Listbox.cs
- DtdParser.cs
- FileInfo.cs
- XmlSchemaCompilationSettings.cs
- FunctionImportMapping.cs
- TdsParserSafeHandles.cs
- XmlNodeChangedEventArgs.cs
- IriParsingElement.cs
- XmlSubtreeReader.cs
- NodeLabelEditEvent.cs
- XmlSchemaSimpleTypeList.cs
- XmlWrappingReader.cs
- UrlPath.cs
- FormViewDeleteEventArgs.cs
- RoleService.cs
- IDQuery.cs
- PartialCachingControl.cs
- XMLSchema.cs
- BaseCodePageEncoding.cs
- DefaultBindingPropertyAttribute.cs
- Int32Collection.cs
- SoapTransportImporter.cs
- HttpModuleAction.cs
- XmlSchemaSimpleTypeRestriction.cs
- TagMapInfo.cs
- NamedPermissionSet.cs
- ComponentResourceKeyConverter.cs
- CompositeTypefaceMetrics.cs
- StreamInfo.cs
- PointConverter.cs
- UnknownWrapper.cs
- EntityDataSourceDesigner.cs
- ManifestSignedXml.cs
- UIElementAutomationPeer.cs
- ImageBrush.cs
- PropertyValueChangedEvent.cs
- XmlEntityReference.cs
- SQLRoleProvider.cs
- Pool.cs
- TrustManagerPromptUI.cs
- TypeSystem.cs
- XmlWriterSettings.cs
- DataPagerFieldCommandEventArgs.cs
- XmlWrappingWriter.cs
- SourceFileBuildProvider.cs
- XmlWhitespace.cs
- UnaryOperationBinder.cs
- DataPager.cs
- LoopExpression.cs
- ActivityTypeCodeDomSerializer.cs
- BaseParser.cs
- XmlSchemaSimpleTypeRestriction.cs
- VerificationException.cs
- ObjectViewEntityCollectionData.cs
- WindowsGraphicsWrapper.cs
- DetailsViewCommandEventArgs.cs
- OdbcReferenceCollection.cs
- ColumnReorderedEventArgs.cs
- SchemaElementDecl.cs
- PagesSection.cs
- MediaCommands.cs
- XmlReflectionMember.cs
- WindowsGraphics2.cs
- HtmlElement.cs
- SqlConnectionPoolGroupProviderInfo.cs
- UriParserTemplates.cs
- AutomationPatternInfo.cs
- ObjectItemAttributeAssemblyLoader.cs
- SHA512CryptoServiceProvider.cs
- TextControl.cs
- TimeEnumHelper.cs
- DataGridViewSelectedColumnCollection.cs
- XhtmlTextWriter.cs
- RewritingProcessor.cs
- TraceHandlerErrorFormatter.cs
- DataGridParentRows.cs
- CodeDomSerializerBase.cs
- Logging.cs
- DrawToolTipEventArgs.cs
- InstanceContextManager.cs
- TextBoxBase.cs
- RandomNumberGenerator.cs
- Operators.cs