Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DrawListViewSubItemEventArgs.cs / 1 / DrawListViewSubItemEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView sub-items (Details view only). /// public class DrawListViewSubItemEventArgs : EventArgs { private readonly Graphics graphics; private readonly Rectangle bounds; private readonly ListViewItem item; private readonly ListViewItem.ListViewSubItem subItem; private readonly int itemIndex; private readonly int columnIndex; private readonly ColumnHeader header; private readonly ListViewItemStates itemState; private bool drawDefault; ////// /// Creates a new DrawListViewSubItemEventArgs with the given parameters. /// public DrawListViewSubItemEventArgs(Graphics graphics, Rectangle bounds, ListViewItem item, ListViewItem.ListViewSubItem subItem, int itemIndex, int columnIndex, ColumnHeader header, ListViewItemStates itemState) { this.graphics = graphics; this.bounds = bounds; this.item = item; this.subItem = subItem; this.itemIndex = itemIndex; this.columnIndex = columnIndex; this.header = header; this.itemState = itemState; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The parent item. /// public ListViewItem Item { get { return item; } } ////// /// The parent item. /// public ListViewItem.ListViewSubItem SubItem { get { return subItem; } } ////// /// The index in the ListView of the parent item. /// public int ItemIndex { get { return itemIndex; } } ////// /// The column index of this sub-item. /// public int ColumnIndex { get { return columnIndex; } } ////// /// The header of this sub-item's column /// public ColumnHeader Header { get { return header; } } ////// /// Miscellaneous state information pertaining to the parent item. /// public ListViewItemStates ItemState { get { return itemState; } } ////// /// Draws the sub-item's background. /// public void DrawBackground() { Color backColor = (itemIndex == -1) ? item.BackColor : subItem.BackColor; using (Brush backBrush = new SolidBrush(backColor)) { Graphics.FillRectangle(backBrush, bounds); } } ////// /// Draws a focus rectangle in the given bounds, if the item has focus. /// public void DrawFocusRectangle(Rectangle bounds) { if((itemState & ListViewItemStates.Focused) == ListViewItemStates.Focused) { ControlPaint.DrawFocusRectangle(graphics, Rectangle.Inflate(bounds, -1, -1), item.ForeColor, item.BackColor); } } ////// /// Draws the sub-item's text (overloaded) /// public void DrawText() { // Map the ColumnHeader::TextAlign to the TextFormatFlags. HorizontalAlignment hAlign = header.TextAlign; TextFormatFlags flags = (hAlign == HorizontalAlignment.Left) ? TextFormatFlags.Left : ((hAlign == HorizontalAlignment.Center) ? TextFormatFlags.HorizontalCenter : TextFormatFlags.Right); flags |= TextFormatFlags.WordEllipsis; DrawText(flags); } ////// /// Draws the sub-item's text (overloaded) - takes a TextFormatFlags argument. /// [ SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters") // We want to measure the size of blank space. // So we don't have to localize it. ] public void DrawText(TextFormatFlags flags) { string text = (itemIndex == -1) ? item.Text : subItem.Text; Font font = (itemIndex == -1) ? item.Font : subItem.Font; Color color = (itemIndex == -1) ? item.ForeColor : subItem.ForeColor; int padding = TextRenderer.MeasureText(" ", font).Width; Rectangle newBounds = Rectangle.Inflate(bounds, -padding, 0); TextRenderer.DrawText(graphics, text, font, newBounds, color, flags); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
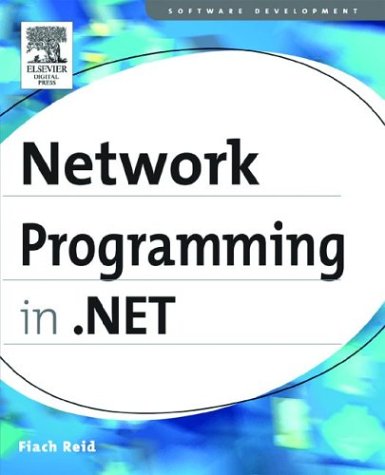
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLInt16.cs
- WebReferencesBuildProvider.cs
- ProjectedSlot.cs
- IncrementalHitTester.cs
- CryptoApi.cs
- XmlSchemaType.cs
- PropertyMetadata.cs
- BitmapDecoder.cs
- Int32Collection.cs
- PageEventArgs.cs
- OdbcPermission.cs
- MailMessageEventArgs.cs
- DesignTimeType.cs
- GlyphRunDrawing.cs
- MissingManifestResourceException.cs
- PageThemeBuildProvider.cs
- ListView.cs
- DataRowCollection.cs
- printdlgexmarshaler.cs
- WmiInstallComponent.cs
- HttpChannelBindingToken.cs
- ManagementQuery.cs
- PoisonMessageException.cs
- DependencyPropertyHelper.cs
- RelationalExpressions.cs
- SimpleBitVector32.cs
- TextFormatterContext.cs
- VisualTreeUtils.cs
- serverconfig.cs
- SecurityUniqueId.cs
- DataTablePropertyDescriptor.cs
- CollectionViewSource.cs
- BuildDependencySet.cs
- EditingCommands.cs
- InternalsVisibleToAttribute.cs
- DbTransaction.cs
- BitVector32.cs
- Update.cs
- SelectionRangeConverter.cs
- CompilerScope.cs
- IOThreadScheduler.cs
- DesignerProperties.cs
- SortQueryOperator.cs
- ECDiffieHellmanCngPublicKey.cs
- QueueAccessMode.cs
- FontUnit.cs
- WindowsSolidBrush.cs
- LinqTreeNodeEvaluator.cs
- IndexedString.cs
- ChooseAction.cs
- EDesignUtil.cs
- SapiAttributeParser.cs
- CursorConverter.cs
- CalloutQueueItem.cs
- PkcsMisc.cs
- RoleManagerSection.cs
- LinkConverter.cs
- XmlReflectionMember.cs
- TranslateTransform3D.cs
- SoapSchemaImporter.cs
- XmlSerializerNamespaces.cs
- TextEffectCollection.cs
- IPipelineRuntime.cs
- SplineKeyFrames.cs
- DataGridViewCellMouseEventArgs.cs
- WindowsRegion.cs
- SingleSelectRootGridEntry.cs
- glyphs.cs
- ErrorCodes.cs
- DynamicControlParameter.cs
- ProfileSection.cs
- PerformanceCounterPermissionEntryCollection.cs
- ListItemConverter.cs
- LoginUtil.cs
- DataGridPageChangedEventArgs.cs
- ParallelActivityDesigner.cs
- UrlPropertyAttribute.cs
- UInt32.cs
- mediapermission.cs
- DecimalConstantAttribute.cs
- HtmlWindow.cs
- AdRotator.cs
- AppSecurityManager.cs
- ErrorLog.cs
- ExcludeFromCodeCoverageAttribute.cs
- ISAPIWorkerRequest.cs
- WindowsStreamSecurityElement.cs
- ZipArchive.cs
- XmlAttributeCache.cs
- Scripts.cs
- DrawingCollection.cs
- Double.cs
- CryptoHandle.cs
- CompositeFontParser.cs
- RSAPKCS1SignatureDeformatter.cs
- ISAPIWorkerRequest.cs
- BindingsCollection.cs
- PathFigure.cs
- Substitution.cs
- NetPipeSection.cs