Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / State / SessionStateContainer.cs / 1305376 / SessionStateContainer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HttpSessionState * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.SessionState { using System.Threading; using System.Runtime.InteropServices; using System.Web; using System.Web.Util; using System.Collections; using System.Collections.Specialized; using System.Text; using System.Globalization; using System.Security.Permissions; public class HttpSessionStateContainer : IHttpSessionState { String _id; ISessionStateItemCollection _sessionItems; HttpStaticObjectsCollection _staticObjects; int _timeout; bool _newSession; HttpCookieMode _cookieMode; SessionStateMode _mode; bool _abandon; bool _isReadonly; SessionStateModule _stateModule; // used for optimized InProc session id callback public HttpSessionStateContainer( String id, ISessionStateItemCollection sessionItems, HttpStaticObjectsCollection staticObjects, int timeout, bool newSession, HttpCookieMode cookieMode, SessionStateMode mode, bool isReadonly) : this(null, id, sessionItems, staticObjects, timeout, newSession, cookieMode, mode, isReadonly) { if (id == null) { throw new ArgumentNullException("id"); } } internal HttpSessionStateContainer( SessionStateModule stateModule, string id, ISessionStateItemCollection sessionItems, HttpStaticObjectsCollection staticObjects, int timeout, bool newSession, HttpCookieMode cookieMode, SessionStateMode mode, bool isReadonly) { _stateModule = stateModule; _id = id; // If null, it means we're delaying session id reading _sessionItems = sessionItems; _staticObjects = staticObjects; _timeout = timeout; _newSession = newSession; _cookieMode = cookieMode; _mode = mode; _isReadonly = isReadonly; } internal HttpSessionStateContainer() { } /* * The Id of the session. */ public String SessionID { get { if (_id == null) { Debug.Assert(_stateModule != null, "_stateModule != null"); _id = _stateModule.DelayedGetSessionId(); } return _id; } } /* * The length of a session before it times out, in minutes. */ public int Timeout { get {return _timeout;} set { if (value <= 0) { throw new ArgumentException(SR.GetString(SR.Timeout_must_be_positive)); } if (value > SessionStateModule.MAX_CACHE_BASED_TIMEOUT_MINUTES && (Mode == SessionStateMode.InProc || Mode == SessionStateMode.StateServer)) { throw new ArgumentException( SR.GetString(SR.Invalid_cache_based_session_timeout)); } _timeout = value; } } /* * Is this a new session? */ ////// public bool IsNewSession { get {return _newSession;} } /* * Is session state in a separate process */ ///[To be supplied.] ////// public SessionStateMode Mode { get {return _mode;} } /* * Is session state cookieless? */ public bool IsCookieless { get { if (_stateModule != null) { // See VSWhidbey 399907 return _stateModule.SessionIDManagerUseCookieless; } else { // For container created by custom session state module, // sorry, we currently don't have a way to tell and thus we rely blindly // on cookieMode. return CookieMode == HttpCookieMode.UseUri; } } } public HttpCookieMode CookieMode { get {return _cookieMode;} } /* * Abandon the session. * */ ///[To be supplied.] ////// public void Abandon() { _abandon = true; } ///[To be supplied.] ////// public int LCID { // get { return Thread.CurrentThread.CurrentCulture.LCID; } set { Thread.CurrentThread.CurrentCulture = HttpServerUtility.CreateReadOnlyCultureInfo(value); } } ///[To be supplied.] ////// public int CodePage { // get { if (HttpContext.Current != null) return HttpContext.Current.Response.ContentEncoding.CodePage; else return Encoding.Default.CodePage; } set { if (HttpContext.Current != null) HttpContext.Current.Response.ContentEncoding = Encoding.GetEncoding(value); } } public bool IsAbandoned { get {return _abandon;} } public HttpStaticObjectsCollection StaticObjects { get { return _staticObjects;} } public Object this[String name] { get { return _sessionItems[name]; } set { _sessionItems[name] = value; } } ///[To be supplied.] ////// public Object this[int index] { get {return _sessionItems[index];} set {_sessionItems[index] = value;} } ///[To be supplied.] ////// public void Add(String name, Object value) { _sessionItems[name] = value; } ///[To be supplied.] ////// public void Remove(String name) { _sessionItems.Remove(name); } ///[To be supplied.] ////// public void RemoveAt(int index) { _sessionItems.RemoveAt(index); } ///[To be supplied.] ////// public void Clear() { _sessionItems.Clear(); } ///[To be supplied.] ////// public void RemoveAll() { Clear(); } ///[To be supplied.] ////// public int Count { get {return _sessionItems.Count;} } ///[To be supplied.] ////// public NameObjectCollectionBase.KeysCollection Keys { get {return _sessionItems.Keys;} } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return _sessionItems.GetEnumerator(); } ///[To be supplied.] ////// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///[To be supplied.] ////// public Object SyncRoot { get { return this;} } ///[To be supplied.] ////// public bool IsReadOnly { get { return _isReadonly;} } ///[To be supplied.] ////// public bool IsSynchronized { get { return false;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
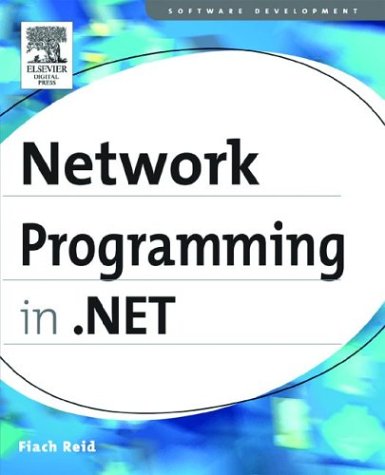
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTrigger.cs
- ChannelTerminatedException.cs
- XPathPatternParser.cs
- VirtualDirectoryMapping.cs
- ElementHost.cs
- ToolStripItemTextRenderEventArgs.cs
- ScaleTransform.cs
- WithStatement.cs
- BinHexDecoder.cs
- GuidTagList.cs
- ToolTipAutomationPeer.cs
- HttpChannelBindingToken.cs
- XmlNamespaceDeclarationsAttribute.cs
- Expander.cs
- GregorianCalendarHelper.cs
- WebDisplayNameAttribute.cs
- EditorPartCollection.cs
- _CommandStream.cs
- ZipArchive.cs
- XmlSchemaSimpleContentRestriction.cs
- VectorCollectionConverter.cs
- EntitySqlQueryCacheEntry.cs
- LiteralLink.cs
- PropertyDescriptorComparer.cs
- OperatingSystemVersionCheck.cs
- CharacterMetrics.cs
- PointHitTestResult.cs
- UnsafeNativeMethods.cs
- GorillaCodec.cs
- ChildDocumentBlock.cs
- DataKeyCollection.cs
- LicenseException.cs
- CodeCatchClauseCollection.cs
- SortableBindingList.cs
- ImageSourceValueSerializer.cs
- SerialPort.cs
- UnsafeNativeMethods.cs
- SmtpException.cs
- SqlDataSourceView.cs
- EnumerableCollectionView.cs
- DataTableClearEvent.cs
- DefaultBinder.cs
- RichTextBoxDesigner.cs
- DesignerVerb.cs
- DbConnectionOptions.cs
- ObjectComplexPropertyMapping.cs
- MatrixAnimationUsingPath.cs
- TempFiles.cs
- MatrixAnimationBase.cs
- BulletedList.cs
- FormatException.cs
- PageContentAsyncResult.cs
- ObjectComplexPropertyMapping.cs
- ThreadStartException.cs
- FileNotFoundException.cs
- XmlCharType.cs
- TextEffect.cs
- TrailingSpaceComparer.cs
- NamedObject.cs
- InputMethodStateTypeInfo.cs
- RegexNode.cs
- MetadataPropertyvalue.cs
- XPathSelfQuery.cs
- DataGridTextBox.cs
- ToolStripDropTargetManager.cs
- COM2IPerPropertyBrowsingHandler.cs
- CodeTypeDeclaration.cs
- SapiInterop.cs
- WebBrowserProgressChangedEventHandler.cs
- BasicBrowserDialog.designer.cs
- Button.cs
- TdsRecordBufferSetter.cs
- CatalogZoneBase.cs
- ConfigurationStrings.cs
- IncrementalCompileAnalyzer.cs
- XmlDataLoader.cs
- ConnectionConsumerAttribute.cs
- TreeViewTemplateSelector.cs
- XmlReflectionMember.cs
- RSAProtectedConfigurationProvider.cs
- GiveFeedbackEvent.cs
- SmtpSection.cs
- AddInPipelineAttributes.cs
- HandoffBehavior.cs
- RuntimeConfigLKG.cs
- PropertyDescriptor.cs
- ReadOnlyNameValueCollection.cs
- FunctionImportElement.cs
- DeadCharTextComposition.cs
- LogManagementAsyncResult.cs
- UserValidatedEventArgs.cs
- SecurityUtils.cs
- ElementProxy.cs
- DrawToolTipEventArgs.cs
- _BaseOverlappedAsyncResult.cs
- LinqDataSourceView.cs
- ConstraintEnumerator.cs
- EventLogPermissionEntryCollection.cs
- RectAnimationClockResource.cs
- X509ChainPolicy.cs