Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / Oletx / OletxCommittableTransaction.cs / 1305376 / OletxCommittableTransaction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions.Oletx { using System; using System.Configuration; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.Remoting.Messaging; using System.Runtime.Serialization; using System.Security.Permissions; using System.Threading; using System.Transactions.Diagnostics; ////// A Transaction object represents a single transaction. It is created by TransactionManager /// objects through CreateTransaction or UnmarshalTransaction. Alternatively, the static Create /// methodis provided, which creates a "default" TransactionManager and requests that it create /// a new transaction with default values. A transaction can only be committed by /// the client application that created the transaction. If a client application wishes to allow /// access to the transaction by multiple threads, but wants to prevent those other threads from /// committing the transaction, the application can make a "clone" of the transaction. Transaction /// clones have the same capabilities as the original transaction, except for the ability to commit /// the transaction. /// [Serializable] internal class OletxCommittableTransaction : OletxTransaction { bool commitCalled = false; ////// Constructor for the Transaction object. Specifies the TransactionManager instance that is /// creating the transaction. /// /// /// Specifies the TransactionManager instance that is creating the transaction. /// internal OletxCommittableTransaction( RealOletxTransaction realOletxTransaction ) : base( realOletxTransaction ) { realOletxTransaction.committableTransaction = this; } internal bool CommitCalled { get { return this.commitCalled; } } internal void BeginCommit( InternalTransaction internalTransaction ) { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), "CommittableTransaction.BeginCommit" ); TransactionCommitCalledTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), this.TransactionTraceId ); } Debug.Assert( ( 0 == this.disposed ), "OletxTransction object is disposed" ); this.realOletxTransaction.InternalTransaction = internalTransaction; this.commitCalled = true; this.realOletxTransaction.Commit(); if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), "CommittableTransaction.BeginCommit" ); } return; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
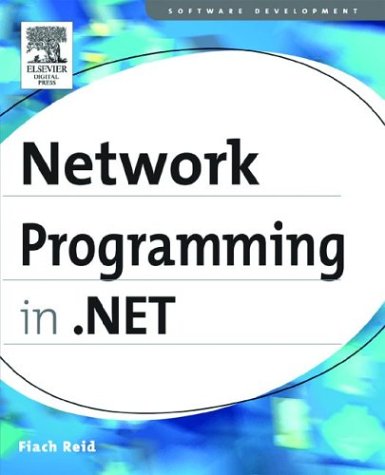
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PlainXmlWriter.cs
- Attributes.cs
- SettingsPropertyWrongTypeException.cs
- XmlCharCheckingWriter.cs
- PersistChildrenAttribute.cs
- serverconfig.cs
- WebPartEventArgs.cs
- ByteArrayHelperWithString.cs
- SR.cs
- HMAC.cs
- PropertyMappingExceptionEventArgs.cs
- DeleteIndexBinder.cs
- ToolStripScrollButton.cs
- DesignerVerb.cs
- SQLInt64.cs
- BuilderInfo.cs
- Decorator.cs
- IteratorFilter.cs
- DbTransaction.cs
- ListManagerBindingsCollection.cs
- CachedBitmap.cs
- MeshGeometry3D.cs
- URLBuilder.cs
- MultiViewDesigner.cs
- BamlLocalizer.cs
- sitestring.cs
- DataGridViewCellConverter.cs
- PartialClassGenerationTask.cs
- InfoCardSymmetricCrypto.cs
- WmfPlaceableFileHeader.cs
- WebSysDescriptionAttribute.cs
- UnaryExpressionHelper.cs
- CodeCatchClauseCollection.cs
- Shape.cs
- ViewStateChangedEventArgs.cs
- ChannelAcceptor.cs
- safelink.cs
- ToolStripArrowRenderEventArgs.cs
- sqlcontext.cs
- OleDbConnection.cs
- ClientUrlResolverWrapper.cs
- DynamicActivity.cs
- ListViewGroupConverter.cs
- HtmlInputCheckBox.cs
- GridItemPattern.cs
- NullToBooleanConverter.cs
- HttpApplicationFactory.cs
- TriggerActionCollection.cs
- TrailingSpaceComparer.cs
- TextSearch.cs
- SettingsPropertyValue.cs
- UnsafeNativeMethodsPenimc.cs
- PropertyChangedEventManager.cs
- FactoryMaker.cs
- XmlArrayItemAttributes.cs
- DataGrid.cs
- EmptyStringExpandableObjectConverter.cs
- Translator.cs
- EntityType.cs
- nulltextnavigator.cs
- SaveFileDialog.cs
- Application.cs
- Fx.cs
- InputEventArgs.cs
- ObjectListTitleAttribute.cs
- TextSimpleMarkerProperties.cs
- XPathNodeIterator.cs
- PersonalizationState.cs
- XmlILTrace.cs
- SizeFConverter.cs
- ProcessModelSection.cs
- ColorBlend.cs
- ContentIterators.cs
- MoveSizeWinEventHandler.cs
- Error.cs
- XmlHierarchicalEnumerable.cs
- TreeNodeStyleCollection.cs
- PageStatePersister.cs
- XmlUnspecifiedAttribute.cs
- NativeCompoundFileAPIs.cs
- ProxyHwnd.cs
- BamlRecordReader.cs
- TableLayoutStyleCollection.cs
- GenerateHelper.cs
- FileFormatException.cs
- ObjectListDataBindEventArgs.cs
- SafeFileHandle.cs
- TypeExtension.cs
- RightNameExpirationInfoPair.cs
- DisplayMemberTemplateSelector.cs
- XmlSchemaExporter.cs
- SafeHandle.cs
- UnsafeNativeMethods.cs
- TraceRecord.cs
- CodeThrowExceptionStatement.cs
- ISO2022Encoding.cs
- ServiceOperationListItem.cs
- ProgressChangedEventArgs.cs
- UnsafeNativeMethods.cs
- DataGridSortCommandEventArgs.cs