Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Error.cs / 1305376 / Error.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static error utility functions // //--------------------------------------------------------------------- namespace System.Data.Services { using System; ////// Strongly-typed and parameterized exception factory. /// internal static partial class Error { ////// create and trace a HttpHeaderFailure /// /// error code /// error message ///DataServiceException internal static DataServiceException HttpHeaderFailure(int errorCode, string message) { return Trace(new DataServiceException(errorCode, message)); } ///create exception when missing an expected batch boundary ///exception to throw internal static DataServiceException BatchStreamMissingBoundary() { return Trace(DataServiceException.CreateBadRequestError(Strings.DataService_InvalidContentTypeForBatchRequest)); } ///create exception when the expected content is missing /// http method operation ///exception to throw internal static DataServiceException BatchStreamContentExpected(BatchStreamState state) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_ContentExpected(state.ToString()))); } ///create exception when unexpected content is discovered /// http method operation ///exception to throw internal static DataServiceException BatchStreamContentUnexpected(BatchStreamState state) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_ContentUnexpected(state.ToString()))); } ///create exception when Get operation is specified in changeset ///exception to throw internal static DataServiceException BatchStreamGetMethodNotSupportInChangeset() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_GetMethodNotSupportedInChangeset)); } ///create exception when invalid batch request is specified ///exception to throw internal static DataServiceException BatchStreamInvalidBatchFormat() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidBatchFormat)); } ///create exception when boundary delimiter is not valid /// delimiter specified as specified in the request. ///exception to throw internal static DataServiceException BatchStreamInvalidDelimiter(string delimiter) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidDelimiter(delimiter))); } ///create exception when end changeset boundary delimiter is missing ///exception to throw internal static DataServiceException BatchStreamMissingEndChangesetDelimiter() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_MissingEndChangesetDelimiter)); } ///create exception when header value specified is not valid /// header value as specified in the request. ///exception to throw internal static DataServiceException BatchStreamInvalidHeaderValueSpecified(string headerValue) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidHeaderValueSpecified(headerValue))); } ///create exception when content length is not valid /// content length as specified in the request. ///exception to throw internal static DataServiceException BatchStreamInvalidContentLengthSpecified(string contentLength) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidContentLengthSpecified(contentLength))); } ///create exception when CUD operation is specified in batch ///exception to throw internal static DataServiceException BatchStreamOnlyGETOperationsCanBeSpecifiedInBatch() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_OnlyGETOperationsCanBeSpecifiedInBatch)); } ///create exception when operation header is specified in start of changeset ///exception to throw internal static DataServiceException BatchStreamInvalidOperationHeaderSpecified() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidOperationHeaderSpecified)); } ///create exception when http method name is not valid /// name of the http method as specified in the request. ///exception to throw internal static DataServiceException BatchStreamInvalidHttpMethodName(string methodName) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidHttpMethodName(methodName))); } ///create exception when more data is specified after end of batch delimiter. ///exception to throw internal static DataServiceException BatchStreamMoreDataAfterEndOfBatch() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_MoreDataAfterEndOfBatch)); } ///internal error where batch stream does do look ahead when read request is smaller than boundary delimiter ///exception to throw internal static InvalidOperationException BatchStreamInternalBufferRequestTooSmall() { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InternalBufferRequestTooSmall)); } ///internal error where the first request header is not of the form: 'MethodName' 'Url' 'Version' /// actual header value specified in the payload. ///exception to throw internal static InvalidOperationException BatchStreamInvalidMethodHeaderSpecified(string header) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidMethodHeaderSpecified(header))); } ///internal error when http version in batching request is not valid /// actual version as specified in the payload. /// expected version value. ///exception to throw internal static InvalidOperationException BatchStreamInvalidHttpVersionSpecified(string actualVersion, string expectedVersion) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidHttpVersionSpecified(actualVersion, expectedVersion))); } ///internal error when number of headers at the start of each operation is not 2 /// First valid header name /// Second valid header name ///exception to throw internal static InvalidOperationException BatchStreamInvalidNumberOfHeadersAtOperationStart(string header1, string header2) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidNumberOfHeadersAtOperationStart(header1, header2))); } ///internal error Content-Transfer-Encoding is not specified or its value is not 'binary' /// name of the header /// expected value of the header ///exception to throw internal static InvalidOperationException BatchStreamMissingOrInvalidContentEncodingHeader(string headerName, string headerValue) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_MissingOrInvalidContentEncodingHeader(headerName, headerValue))); } ///internal error number of headers at the start of changeset is not correct /// First valid header name /// Second valid header name ///exception to throw internal static InvalidOperationException BatchStreamInvalidNumberOfHeadersAtChangeSetStart(string header1, string header2) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidNumberOfHeadersAtChangeSetStart(header1, header2))); } ///internal error when content type header is missing /// name of the missing header ///exception to throw internal static InvalidOperationException BatchStreamMissingContentTypeHeader(string headerName) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_MissingContentTypeHeader(headerName))); } ///internal error when content type header value is invalid. /// name of the header whose value is not correct. /// actual value as specified in the payload /// expected value 1 /// expected value 2 ///exception to throw internal static InvalidOperationException BatchStreamInvalidContentTypeSpecified(string headerName, string headerValue, string mime1, string mime2) { return Trace(DataServiceException.CreateBadRequestError(Strings.BatchStream_InvalidContentTypeSpecified(headerName, headerValue, mime1, mime2))); } ////// create and throw a ThrowObjectDisposed with a type name /// /// type being thrown on internal static void ThrowObjectDisposed(Type type) { throw Trace(new ObjectDisposedException(type.ToString())); } ////// Trace the exception /// ///type of the exception /// exception object to trace ///the exception parameter private static T Trace(T exception) where T : Exception { return exception; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
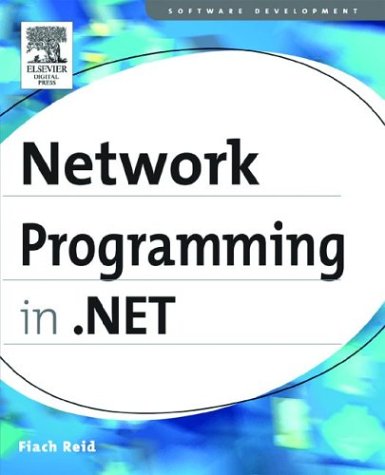
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeRsaProviderHandle.cs
- DriveNotFoundException.cs
- RelationshipEnd.cs
- BaseValidator.cs
- Debug.cs
- SecureStringHasher.cs
- ObjectPersistData.cs
- ByteKeyFrameCollection.cs
- StorageModelBuildProvider.cs
- EditorZoneBase.cs
- Blend.cs
- MemberRelationshipService.cs
- FormsAuthentication.cs
- TypeSemantics.cs
- WindowsFormsSynchronizationContext.cs
- StringHandle.cs
- DBDataPermissionAttribute.cs
- XmlEncodedRawTextWriter.cs
- SvcMapFileSerializer.cs
- ColumnWidthChangingEvent.cs
- InkCanvasSelection.cs
- Metadata.cs
- WindowsListViewItemCheckBox.cs
- InternalMappingException.cs
- HttpListenerContext.cs
- FileChangesMonitor.cs
- MessageLogTraceRecord.cs
- CssClassPropertyAttribute.cs
- DescendantBaseQuery.cs
- InternalDuplexChannelFactory.cs
- BuildProviderUtils.cs
- securitycriticaldataformultiplegetandset.cs
- SchemaImporterExtensionElement.cs
- BaseResourcesBuildProvider.cs
- QueueProcessor.cs
- DataRow.cs
- ImageListDesigner.cs
- RegexRunnerFactory.cs
- TdsValueSetter.cs
- TypeDescriptionProvider.cs
- RegistrationServices.cs
- HttpRequest.cs
- Image.cs
- ObjectDataSourceMethodEventArgs.cs
- StorageEntityContainerMapping.cs
- XmlEnumAttribute.cs
- ThreadAttributes.cs
- SimpleBitVector32.cs
- GroupBoxRenderer.cs
- ScriptManagerProxy.cs
- GroupBoxRenderer.cs
- WebConfigurationFileMap.cs
- WindowProviderWrapper.cs
- ServiceDescriptionReflector.cs
- SoapReflectionImporter.cs
- CharacterHit.cs
- MarginsConverter.cs
- SelectedCellsChangedEventArgs.cs
- EventItfInfo.cs
- SmtpReplyReaderFactory.cs
- SqlProfileProvider.cs
- WrappedIUnknown.cs
- BaseParser.cs
- GradientSpreadMethodValidation.cs
- PropertyMapper.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- FormCollection.cs
- MetadataItemSerializer.cs
- ThicknessAnimationUsingKeyFrames.cs
- WinFormsComponentEditor.cs
- SecureStringHasher.cs
- SqlFactory.cs
- XPathConvert.cs
- DebugView.cs
- COAUTHINFO.cs
- ConditionCollection.cs
- SkewTransform.cs
- MethodImplAttribute.cs
- Configuration.cs
- SmiGettersStream.cs
- Label.cs
- PointF.cs
- WindowsScrollBar.cs
- EpmTargetPathSegment.cs
- ContentDesigner.cs
- ConfigWriter.cs
- CreateUserWizard.cs
- ErrorFormatter.cs
- ContainerFilterService.cs
- SmtpSection.cs
- InstalledVoice.cs
- PreviewControlDesigner.cs
- QuaternionIndependentAnimationStorage.cs
- NotificationContext.cs
- SystemTcpConnection.cs
- ExtensionQuery.cs
- IntSecurity.cs
- ToolStripContentPanelDesigner.cs
- DrawListViewColumnHeaderEventArgs.cs
- Int64Storage.cs