Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlSortKeyAccumulator.cs / 1305376 / XmlSortKeyAccumulator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Accumulates a list of sort keys and stores them in an array. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct XmlSortKeyAccumulator { private XmlSortKey[] keys; private int pos; #if DEBUG private const int DefaultSortKeyCount = 4; #else private const int DefaultSortKeyCount = 64; #endif ////// Initialize the XmlSortKeyAccumulator. /// public void Create() { if (this.keys == null) this.keys = new XmlSortKey[DefaultSortKeyCount]; this.pos = 0; this.keys[0] = null; } ////// Create a new sort key and append it to the current run of sort keys. /// public void AddStringSortKey(XmlCollation collation, string value) { AppendSortKey(collation.CreateSortKey(value)); } public void AddDecimalSortKey(XmlCollation collation, decimal value) { AppendSortKey(new XmlDecimalSortKey(value, collation)); } public void AddIntegerSortKey(XmlCollation collation, long value) { AppendSortKey(new XmlIntegerSortKey(value, collation)); } public void AddIntSortKey(XmlCollation collation, int value) { AppendSortKey(new XmlIntSortKey(value, collation)); } public void AddDoubleSortKey(XmlCollation collation, double value) { AppendSortKey(new XmlDoubleSortKey(value, collation)); } public void AddDateTimeSortKey(XmlCollation collation, DateTime value) { AppendSortKey(new XmlDateTimeSortKey(value, collation)); } public void AddEmptySortKey(XmlCollation collation) { AppendSortKey(new XmlEmptySortKey(collation)); } ////// Finish creating the current run of sort keys and begin a new run. /// public void FinishSortKeys() { this.pos++; if (this.pos >= this.keys.Length) { XmlSortKey[] keysNew = new XmlSortKey[this.pos * 2]; Array.Copy(this.keys, 0, keysNew, 0, this.keys.Length); this.keys = keysNew; } this.keys[this.pos] = null; } ////// Append new sort key to the current run of sort keys. /// private void AppendSortKey(XmlSortKey key) { // Ensure that sort will be stable by setting index of key key.Priority = this.pos; if (this.keys[this.pos] == null) this.keys[this.pos] = key; else this.keys[this.pos].AddSortKey(key); } ////// Get array of sort keys that was constructed by this internal class. /// public Array Keys { get { return this.keys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
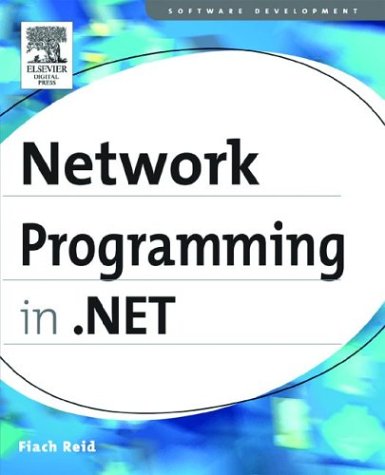
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LoginViewDesigner.cs
- PackagePartCollection.cs
- CodeNamespace.cs
- TextUtf8RawTextWriter.cs
- Separator.cs
- Assembly.cs
- SafeNativeMemoryHandle.cs
- TreeNodeClickEventArgs.cs
- InvokeGenerator.cs
- ObjectDataSourceView.cs
- Attachment.cs
- ChannelPoolSettings.cs
- keycontainerpermission.cs
- ServiceDescriptionSerializer.cs
- ExpressionTable.cs
- TableAutomationPeer.cs
- ClientSettingsProvider.cs
- FrameworkTextComposition.cs
- SqlProfileProvider.cs
- BounceEase.cs
- RepeaterDesigner.cs
- ClientBuildManagerCallback.cs
- CompilerParameters.cs
- ObjectStateEntry.cs
- TypeInitializationException.cs
- DataServiceException.cs
- TagMapInfo.cs
- CompositeScriptReference.cs
- WebServiceHostFactory.cs
- DataBindEngine.cs
- RuntimeIdentifierPropertyAttribute.cs
- WebEvents.cs
- StateManager.cs
- TimeSpan.cs
- _ConnectionGroup.cs
- OdbcUtils.cs
- DataGridViewToolTip.cs
- CultureData.cs
- CompositeControl.cs
- RIPEMD160.cs
- CategoryValueConverter.cs
- OdbcHandle.cs
- DataGridViewSelectedColumnCollection.cs
- Timer.cs
- ImpersonateTokenRef.cs
- DataSetMappper.cs
- X509Certificate.cs
- XsdValidatingReader.cs
- MachineKeyConverter.cs
- PreviewKeyDownEventArgs.cs
- OpCellTreeNode.cs
- DataGridRow.cs
- InstanceOwner.cs
- BinaryNode.cs
- Compiler.cs
- PropertyChangeTracker.cs
- sqlmetadatafactory.cs
- ControlBuilderAttribute.cs
- TextModifier.cs
- MetafileHeader.cs
- OleDbWrapper.cs
- RemotingSurrogateSelector.cs
- ToolStripLabel.cs
- ByteStorage.cs
- Thread.cs
- CqlWriter.cs
- XmlCharacterData.cs
- ScrollChangedEventArgs.cs
- CodeComment.cs
- ExpressionBuilderContext.cs
- UrlParameterReader.cs
- MatrixIndependentAnimationStorage.cs
- Directory.cs
- HtmlTernaryTree.cs
- Condition.cs
- WindowsRichEdit.cs
- Rotation3DAnimation.cs
- DocumentViewer.cs
- ReflectionHelper.cs
- Matrix3DConverter.cs
- OdbcError.cs
- ServiceMemoryGates.cs
- Rotation3DAnimation.cs
- FunctionImportMapping.cs
- TextProperties.cs
- _NetRes.cs
- HttpListenerException.cs
- DataGridViewCellValidatingEventArgs.cs
- SessionIDManager.cs
- TextFindEngine.cs
- BoundPropertyEntry.cs
- ColumnResult.cs
- SafeNativeMethods.cs
- BasicBrowserDialog.designer.cs
- LiteralControl.cs
- XmlNavigatorFilter.cs
- SkinBuilder.cs
- SetStoryboardSpeedRatio.cs
- AnnotationMap.cs
- FileFormatException.cs